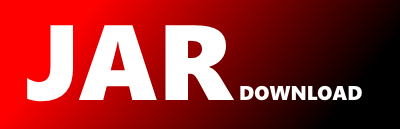
com.helger.html.jscode.JSCommentPart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-html-jscode Show documentation
Show all versions of ph-html-jscode Show documentation
Java library to programmtically create JavaScript in a structured way
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy