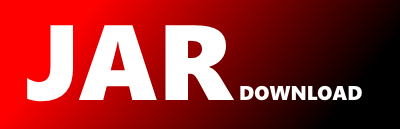
com.helger.html.hc.config.HCSettings Maven / Gradle / Ivy
/**
* Copyright (C) 2014-2016 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.html.hc.config;
import java.nio.charset.Charset;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.GuardedBy;
import javax.annotation.concurrent.ThreadSafe;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.helger.commons.CGlobal;
import com.helger.commons.ValueEnforcer;
import com.helger.commons.annotation.ReturnsMutableObject;
import com.helger.commons.concurrent.SimpleReadWriteLock;
import com.helger.commons.debug.GlobalDebug;
import com.helger.commons.lang.ServiceLoaderHelper;
import com.helger.commons.system.ENewLineMode;
import com.helger.html.EHTMLVersion;
import com.helger.html.hc.IHCConversionSettings;
/**
* Global HC settings
*
* @author Philip Helger
*/
@ThreadSafe
public final class HCSettings
{
/** Default auto-complete for password fields: only in debug mode */
public static final boolean DEFAULT_AUTO_COMPLETE_OFF_FOR_PASSWORD_EDITS = !GlobalDebug.isDebugMode ();
/** By default inline scripts are emitted in mode "wrap in comment" */
public static final EHCScriptInlineMode DEFAULT_SCRIPT_INLINE_MODE = EHCScriptInlineMode.PLAIN_TEXT_WRAPPED_IN_COMMENT;
/** By default plain text without escape is used */
public static final EHCStyleInlineMode DEFAULT_STYLE_MODE = EHCStyleInlineMode.PLAIN_TEXT_NO_ESCAPE;
private static final Logger s_aLogger = LoggerFactory.getLogger (HCSettings.class);
private static final SimpleReadWriteLock s_aRWLock = new SimpleReadWriteLock ();
/** HC to MicroNode converter settings */
@GuardedBy ("s_aRWLock")
private static HCConversionSettings s_aConversionSettings = new HCConversionSettings (EHTMLVersion.DEFAULT);
/**
* For security reasons, the password should not be auto-filled by the browser
* in the release-version
*/
@GuardedBy ("s_aRWLock")
private static boolean s_bAutoCompleteOffForPasswordEdits = DEFAULT_AUTO_COMPLETE_OFF_FOR_PASSWORD_EDITS;
@GuardedBy ("s_aRWLock")
private static int s_nTextAreaDefaultRows = CGlobal.ILLEGAL_UINT;
/** The "on document ready" code provider */
@GuardedBy ("s_aRWLock")
private static IHCOnDocumentReadyProvider s_aOnDocumentReadyProvider = new DefaultHCOnDocumentReadyProvider ();
@GuardedBy ("s_aRWLock")
private static EHCScriptInlineMode s_eScriptInlineMode = DEFAULT_SCRIPT_INLINE_MODE;
@GuardedBy ("s_aRWLock")
private static EHCStyleInlineMode s_eStyleInlineMode = DEFAULT_STYLE_MODE;
@GuardedBy ("s_aRWLock")
private static ENewLineMode s_eNewLineMode = ENewLineMode.DEFAULT;
@GuardedBy ("s_aRWLock")
private static boolean s_bOOBDebugging = false;
@GuardedBy ("s_aRWLock")
private static boolean s_bScriptsInBody = true;
@GuardedBy ("s_aRWLock")
private static boolean s_bUseRegularResources = GlobalDebug.isDebugMode ();
static
{
// Apply all SPI settings providers
for (final IHCSettingsProviderSPI aSPI : ServiceLoaderHelper.getAllSPIImplementations (IHCSettingsProviderSPI.class))
aSPI.initHCSettings ();
}
private HCSettings ()
{}
/**
* Set the global conversion settings.
*
* @param aConversionSettings
* The object to be used. May not be null
.
*/
public static void setConversionSettings (@Nonnull final HCConversionSettings aConversionSettings)
{
ValueEnforcer.notNull (aConversionSettings, "ConversionSettings");
s_aRWLock.writeLocked ( () -> s_aConversionSettings = aConversionSettings);
}
/**
* @return The global mutable conversion settings. Never null
.
*/
@Nonnull
@ReturnsMutableObject ("design")
public static HCConversionSettings getMutableConversionSettings ()
{
return s_aRWLock.readLocked ( () -> s_aConversionSettings);
}
/**
* @return The global read-only non-null
conversion settings
*/
@Nonnull
public static IHCConversionSettings getConversionSettings ()
{
return getMutableConversionSettings ();
}
/**
* @return The global read-only non-null
conversion settings with
* XML namespaces disabled
*/
@Nonnull
public static HCConversionSettings getConversionSettingsWithoutNamespaces ()
{
// Create a copy!!
final HCConversionSettings aCS = getMutableConversionSettings ().getClone ();
// And modify the copied XML settings
aCS.getXMLWriterSettings ().setEmitNamespaces (false);
return aCS;
}
/**
* Get the {@link Charset} that is used to create the HTML code.
*
* @return The non-null
Charset object
*/
@Nonnull
public static Charset getHTMLCharset ()
{
return getConversionSettings ().getXMLWriterSettings ().getCharsetObj ();
}
/**
* Set the default HTML version to use. This sets the HTML version in the
* {@link HCSettings} class and performs some additional modifications
* depending on the chosen version.
*
* @param eHTMLVersion
* The HTML version to use. May not be null
.
*/
public static void setDefaultHTMLVersion (@Nonnull final EHTMLVersion eHTMLVersion)
{
ValueEnforcer.notNull (eHTMLVersion, "HTMLVersion");
// Update the HCSettings
getMutableConversionSettings ().setHTMLVersion (eHTMLVersion);
// Update the XMLWriterSettings
getMutableConversionSettings ().setXMLWriterSettings (HCConversionSettings.createDefaultXMLWriterSettings (eHTMLVersion));
if (eHTMLVersion.isAtLeastHTML5 ())
{
// No need to put anything in a comment
setScriptInlineMode (EHCScriptInlineMode.PLAIN_TEXT_NO_ESCAPE);
}
else
{
// Use default mode
setScriptInlineMode (DEFAULT_SCRIPT_INLINE_MODE);
}
}
public static boolean isAutoCompleteOffForPasswordEdits ()
{
return s_aRWLock.readLocked ( () -> s_bAutoCompleteOffForPasswordEdits);
}
public static void setAutoCompleteOffForPasswordEdits (final boolean bAutoCompleteOffForPasswordEdits)
{
s_aRWLock.writeLocked ( () -> s_bAutoCompleteOffForPasswordEdits = bAutoCompleteOffForPasswordEdits);
}
public static int getTextAreaDefaultRows ()
{
return s_aRWLock.readLocked ( () -> s_nTextAreaDefaultRows);
}
public static void setTextAreaDefaultRows (final int nTextAreaDefaultRows)
{
s_aRWLock.writeLocked ( () -> s_nTextAreaDefaultRows = nTextAreaDefaultRows);
}
@Nonnull
public static IHCOnDocumentReadyProvider getOnDocumentReadyProvider ()
{
return s_aRWLock.readLocked ( () -> s_aOnDocumentReadyProvider);
}
public static void setOnDocumentReadyProvider (@Nonnull final IHCOnDocumentReadyProvider aOnDocumentReadyProvider)
{
ValueEnforcer.notNull (aOnDocumentReadyProvider, "OnDocumentReadyProvider");
s_aRWLock.writeLocked ( () -> s_aOnDocumentReadyProvider = aOnDocumentReadyProvider);
}
/**
* @return The default masking mode to emit script content. Never
* null
.
*/
@Nonnull
public static EHCScriptInlineMode getScriptInlineMode ()
{
return s_aRWLock.readLocked ( () -> s_eScriptInlineMode);
}
/**
* Set how the content of script elements should be emitted. This only affects
* new built objects, and does not alter existing objects! The default mode is
* {@link #DEFAULT_SCRIPT_INLINE_MODE}.
*
* @param eMode
* The new masking mode to set. May not be null
.
*/
public static void setScriptInlineMode (@Nonnull final EHCScriptInlineMode eMode)
{
ValueEnforcer.notNull (eMode, "Mode");
s_aRWLock.writeLocked ( () -> {
if (s_eScriptInlineMode != eMode)
{
s_eScriptInlineMode = eMode;
s_aLogger.info ("Default