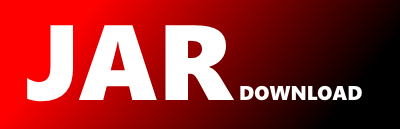
com.helger.html.markdown.IMarkdownDecorator Maven / Gradle / Ivy
/**
* Copyright (C) 2014-2016 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.html.markdown;
import javax.annotation.Nonnull;
import com.helger.html.hc.html.IHCElementWithChildren;
import com.helger.html.hc.html.embedded.HCImg;
import com.helger.html.hc.html.grouping.HCLI;
import com.helger.html.hc.html.textlevel.HCA;
import com.helger.html.hc.html.textlevel.HCCode;
/**
* Decorator interface.
*
* @author René Jeschke <[email protected]>
*/
public interface IMarkdownDecorator
{
/**
* Called when a paragraph is opened.
*
* Default implementation is:
*
*
*
* out.append("<p>");
*
*
* @param out
* The StringBuilder to write to.
*/
void openParagraph (@Nonnull MarkdownHCStack out);
/**
* Called when a paragraph is closed.
*
* Default implementation is:
*
*
*
* out.append("</p>\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeParagraph (@Nonnull MarkdownHCStack out);
/**
* Called when a blockquote is opened. Default implementation is:
*
*
* out.append("<blockquote>");
*
*
* @param out
* The StringBuilder to write to.
*/
void openBlockquote (@Nonnull MarkdownHCStack out);
/**
* Called when a blockquote is closed.
*
* Default implementation is:
*
*
*
* out.append("</blockquote>\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeBlockquote (@Nonnull MarkdownHCStack out);
/**
* Called when a code block is opened.
*
* Default implementation is:
*
*
*
* out.append("<pre><code>");
*
*
* @param out
* The StringBuilder to write to.
*/
void openCodeBlock (@Nonnull MarkdownHCStack out);
/**
* Called when a code block is closed.
*
* Default implementation is:
*
*
*
* out.append("</code></pre>\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeCodeBlock (@Nonnull MarkdownHCStack out);
/**
* Called when a code span is opened.
*
* Default implementation is:
*
*
*
* out.append("<code>");
*
*
* @param out
* The StringBuilder to write to.
* @return code element
*/
HCCode openCodeSpan (@Nonnull MarkdownHCStack out);
/**
* Called when a code span is closed.
*
* Default implementation is:
*
*
*
* out.append("</code>");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeCodeSpan (@Nonnull MarkdownHCStack out);
/**
* Called when a headline is opened.
*
* Note: Don't close the HTML tag!
*
*
* Default implementation is:
*
*
*
* out.append("<h");
* out.append(level);
*
*
* @param out
* The StringBuilder to write to.
* @param level
* Headline level
* @return Headline element
*/
@Nonnull
IHCElementWithChildren > openHeadline (@Nonnull MarkdownHCStack out, int level);
/**
* Called when a headline is closed.
*
* Default implementation is:
*
*
*
* out.append("</h");
* out.append(level);
* out.append(">\n");
*
*
* @param out
* The StringBuilder to write to.
* @param level
* Headline level
*/
void closeHeadline (@Nonnull MarkdownHCStack out, int level);
/**
* Called when a strong span is opened.
*
* Default implementation is:
*
*
*
* out.append("<strong>");
*
*
* @param out
* The StringBuilder to write to.
*/
void openStrong (@Nonnull MarkdownHCStack out);
/**
* Called when a strong span is closed.
*
* Default implementation is:
*
*
*
* out.append("</strong>");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeStrong (@Nonnull MarkdownHCStack out);
/**
* Called when a strike span is opened.
*
* Default implementation is:
*
*
*
* out.append("<s>");
*
*
* @param out
* The StringBuilder to write to.
*/
void openStrike (@Nonnull MarkdownHCStack out);
/**
* Called when a strike span is closed.
*
* Default implementation is:
*
*
*
* out.append("</s>");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeStrike (@Nonnull MarkdownHCStack out);
/**
* Called when an emphasis span is opened.
*
* Default implementation is:
*
*
*
* out.append("<em>");
*
*
* @param out
* The StringBuilder to write to.
*/
void openEmphasis (@Nonnull MarkdownHCStack out);
/**
* Called when an emphasis span is closed.
*
* Default implementation is:
*
*
*
* out.append("</em>");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeEmphasis (@Nonnull MarkdownHCStack out);
/**
* Called when a superscript span is opened.
*
* Default implementation is:
*
*
*
* out.append("<sup>");
*
*
* @param out
* The StringBuilder to write to.
*/
void openSuper (@Nonnull MarkdownHCStack out);
/**
* Called when a superscript span is closed.
*
* Default implementation is:
*
*
*
* out.append("</sup>");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeSuper (@Nonnull MarkdownHCStack out);
/**
* Called when an ordered list is opened.
*
* Default implementation is:
*
*
*
* out.append("<ol>\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void openOrderedList (@Nonnull MarkdownHCStack out);
/**
* Called when an ordered list is closed.
*
* Default implementation is:
*
*
*
* out.append("</ol>\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeOrderedList (@Nonnull MarkdownHCStack out);
/**
* Called when an unordered list is opened.
*
* Default implementation is:
*
*
*
* out.append("<ul>\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void openUnorderedList (@Nonnull MarkdownHCStack out);
/**
* Called when an unordered list is closed.
*
* Default implementation is:
*
*
*
* out.append("</ul>\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeUnorderedList (@Nonnull MarkdownHCStack out);
/**
* Called when a list item is opened.
*
* Note: Don't close the HTML tag!
*
*
* Default implementation is:
*
*
*
* out.append("<li");
*
*
* @param out
* The StringBuilder to write to.
* @return List item element
*/
@Nonnull
HCLI openListItem (@Nonnull MarkdownHCStack out);
/**
* Called when a list item is closed.
*
* Default implementation is:
*
*
*
* out.append("</li>\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void closeListItem (@Nonnull MarkdownHCStack out);
/**
* Called when a horizontal ruler is encountered.
*
* Default implementation is:
*
*
*
* out.append("<hr />\n");
*
*
* @param out
* The StringBuilder to write to.
*/
void appendHorizontalRuler (@Nonnull MarkdownHCStack out);
/**
* Called when a link is opened.
*
* Note: Don't close the HTML tag!
*
*
* Default implementation is:
*
*
*
* out.append("<a");
*
*
* @param out
* The stack to write to.
* @return Link element
*/
@Nonnull
HCA openLink (@Nonnull MarkdownHCStack out);
void closeLink (@Nonnull MarkdownHCStack out);
/**
* Called when an image is opened.
*
* Note: Don't close the HTML tag!
*
*
* Default implementation is:
*
*
*
* out.append("<img");
*
*
* @param out
* The StringBuilder to write to.
* @return Image element
*/
@Nonnull
HCImg appendImage (@Nonnull MarkdownHCStack out);
}