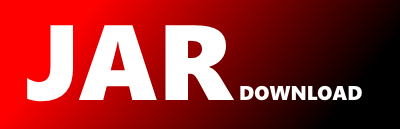
com.helger.http.basicauth.BasicAuthClientCredentials Maven / Gradle / Ivy
/**
* Copyright (C) 2014-2020 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.http.basicauth;
import java.io.Serializable;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.concurrent.Immutable;
import com.helger.commons.ValueEnforcer;
import com.helger.commons.annotation.Nonempty;
import com.helger.commons.base64.Base64;
import com.helger.commons.equals.EqualsHelper;
import com.helger.commons.hashcode.HashCodeGenerator;
import com.helger.commons.string.StringHelper;
import com.helger.commons.string.ToStringGenerator;
/**
* Credentials for HTTP basic authentication
*
* @author Philip Helger
*/
@Immutable
public class BasicAuthClientCredentials implements Serializable
{
private final String m_sUserName;
private final String m_sPassword;
/**
* Create credentials with a user name only and no password.
*
* @param sUserName
* The user name to use. May neither be null
nor empty.
*/
public BasicAuthClientCredentials (@Nonnull @Nonempty final String sUserName)
{
this (sUserName, null);
}
/**
* Create credentials with a user name and a password.
*
* @param sUserName
* The user name to use. May neither be null
nor empty.
* @param sPassword
* The password to use. May be null
or empty to indicate
* that no password is present.
*/
public BasicAuthClientCredentials (@Nonnull @Nonempty final String sUserName, @Nullable final String sPassword)
{
m_sUserName = ValueEnforcer.notEmpty (sUserName, "UserName");
// No difference between null and empty string
m_sPassword = StringHelper.hasNoText (sPassword) ? null : sPassword;
}
/**
* @return The user name. Neither null
nor empty.
*/
@Nonnull
@Nonempty
public String getUserName ()
{
return m_sUserName;
}
/**
* @return The password. May be null
or empty.
*/
@Nullable
public String getPassword ()
{
return m_sPassword;
}
/**
* @return true
if a non-null
non-empty password is
* present.
*/
public boolean hasPassword ()
{
return m_sPassword != null;
}
/**
* Create the request HTTP header value for use with the
* {@link com.helger.commons.http.CHttpHeader#AUTHORIZATION} header name.
*
* @return The HTTP header value to use. Neither null
nor empty.
*/
@Nonnull
@Nonempty
public String getRequestValue ()
{
final String sCombined = StringHelper.getConcatenatedOnDemand (m_sUserName,
HttpBasicAuth.USERNAME_PASSWORD_SEPARATOR,
m_sPassword);
return HttpBasicAuth.HEADER_VALUE_PREFIX_BASIC + " " + Base64.safeEncode (sCombined, HttpBasicAuth.CHARSET);
}
@Override
public boolean equals (final Object o)
{
if (o == this)
return true;
if (o == null || !getClass ().equals (o.getClass ()))
return false;
final BasicAuthClientCredentials rhs = (BasicAuthClientCredentials) o;
return m_sUserName.equals (rhs.m_sUserName) && EqualsHelper.equals (m_sPassword, rhs.m_sPassword);
}
@Override
public int hashCode ()
{
return new HashCodeGenerator (this).append (m_sUserName).append (m_sPassword).getHashCode ();
}
@Override
public String toString ()
{
return new ToStringGenerator (this).append ("userName", m_sUserName).appendPassword ("password").getToString ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy