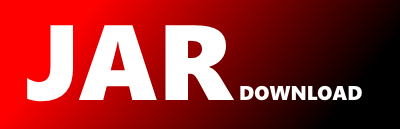
com.helger.jaxb.IJAXBWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-jaxb Show documentation
Show all versions of ph-jaxb Show documentation
Special Java 1.8+ Library with extended JAXB support
/**
* Copyright (C) 2014-2016 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.jaxb;
import java.io.File;
import java.io.OutputStream;
import java.io.Writer;
import java.nio.BufferOverflowException;
import java.nio.ByteBuffer;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.WillClose;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.stream.XMLStreamException;
import javax.xml.transform.Result;
import org.w3c.dom.Document;
import com.helger.commons.io.resource.IWritableResource;
import com.helger.commons.io.stream.ByteBufferOutputStream;
import com.helger.commons.io.stream.NonBlockingStringWriter;
import com.helger.commons.io.stream.StreamHelper;
import com.helger.commons.state.ESuccess;
import com.helger.xml.XMLFactory;
import com.helger.xml.microdom.IMicroDocument;
import com.helger.xml.microdom.serialize.MicroSAXHandler;
import com.helger.xml.transform.TransformResultFactory;
/**
* Interface for writing JAXB documents to various destinations.
*
* @author Philip Helger
* @param
* The JAXB type to be written
*/
public interface IJAXBWriter
{
/**
* A special bi-consumer that additionally can throw a {@link JAXBException}
*
* @author Philip Helger
* @param
* The JAXB type to be written
*/
@FunctionalInterface
public interface IJAXBMarshaller
{
void doMarshal (@Nonnull Marshaller aMarshaller, @Nonnull JAXBElement aJAXBElement) throws JAXBException;
}
/**
* Write the passed object to a {@link File}.
*
* @param aObject
* The object to be written. May not be null
.
* @param aResultFile
* The result file to be written to. May not be null
.
* @return {@link ESuccess}
*/
@Nonnull
default ESuccess write (@Nonnull final JAXBTYPE aObject, @Nonnull final File aResultFile)
{
return write (aObject, TransformResultFactory.create (aResultFile));
}
/**
* Write the passed object to an {@link OutputStream}.
*
* @param aObject
* The object to be written. May not be null
.
* @param aOS
* The output stream to write to. Will always be closed. May not be
* null
.
* @return {@link ESuccess}
*/
@Nonnull
default ESuccess write (@Nonnull final JAXBTYPE aObject, @Nonnull @WillClose final OutputStream aOS)
{
try
{
return write (aObject, TransformResultFactory.create (aOS));
}
finally
{
// Needs to be manually closed
StreamHelper.close (aOS);
}
}
/**
* Write the passed object to a {@link Writer}.
*
* @param aObject
* The object to be written. May not be null
.
* @param aWriter
* The writer to write to. Will always be closed. May not be
* null
.
* @return {@link ESuccess}
*/
@Nonnull
default ESuccess write (@Nonnull final JAXBTYPE aObject, @Nonnull @WillClose final Writer aWriter)
{
try
{
return write (aObject, TransformResultFactory.create (aWriter));
}
finally
{
// Needs to be manually closed
StreamHelper.close (aWriter);
}
}
/**
* Write the passed object to a {@link ByteBuffer}.
*
* @param aObject
* The object to be written. May not be null
.
* @param aBuffer
* The byte buffer to write to. If the buffer is too small, it is
* automatically extended. May not be null
.
* @return {@link ESuccess}
* @throws BufferOverflowException
* If the ByteBuffer is too small
*/
@Nonnull
default ESuccess write (@Nonnull final JAXBTYPE aObject, @Nonnull final ByteBuffer aBuffer)
{
return write (aObject, new ByteBufferOutputStream (aBuffer, false));
}
/**
* Write the passed object to an {@link IWritableResource}.
*
* @param aObject
* The object to be written. May not be null
.
* @param aResource
* The result resource to be written to. May not be null
.
* @return {@link ESuccess}
*/
@Nonnull
default ESuccess write (@Nonnull final JAXBTYPE aObject, @Nonnull final IWritableResource aResource)
{
return write (aObject, TransformResultFactory.create (aResource));
}
/**
* Convert the passed object to XML.
*
* @param aObject
* The object to be converted. May not be null
.
* @param aMarshallerFunc
* The marshalling function. May not be null
.
* @return {@link ESuccess}
*/
@Nonnull
ESuccess write (@Nonnull JAXBTYPE aObject, @Nonnull IJAXBMarshaller aMarshallerFunc);
/**
* Convert the passed object to XML.
*
* @param aObject
* The object to be converted. May not be null
.
* @param aResult
* The result object holder. May not be null
.
* @return {@link ESuccess}
*/
@Nonnull
default ESuccess write (@Nonnull final JAXBTYPE aObject, @Nonnull final Result aResult)
{
return write (aObject, (m, e) -> m.marshal (e, aResult));
}
/**
* Convert the passed object to XML.
*
* @param aObject
* The object to be converted. May not be null
.
* @param aHandler
* XML will be sent to this handler as SAX2 events. May not be
* null
.
* @return {@link ESuccess}
*/
@Nonnull
default ESuccess write (@Nonnull final JAXBTYPE aObject, @Nonnull final org.xml.sax.ContentHandler aHandler)
{
return write (aObject, (m, e) -> m.marshal (e, aHandler));
}
/**
* Convert the passed object to XML.
*
* @param aObject
* The object to be converted. May not be null
.
* @param aWriter
* XML will be sent to this writer. May not be null
.
* @return {@link ESuccess}
*/
@Nonnull
default ESuccess write (@Nonnull final JAXBTYPE aObject,
@Nonnull @WillClose final javax.xml.stream.XMLStreamWriter aWriter)
{
try
{
return write (aObject, (m, e) -> m.marshal (e, aWriter));
}
finally
{
// Needs to be manually closed
try
{
aWriter.flush ();
aWriter.close ();
}
catch (final XMLStreamException ex)
{
throw new IllegalStateException ("Failed to close XMLStreamWriter", ex);
}
}
}
/**
* Convert the passed object to a new DOM document.
*
* @param aObject
* The object to be converted. May not be null
.
* @return null
if converting the document failed.
*/
@Nullable
default Document getAsDocument (@Nonnull final JAXBTYPE aObject)
{
final Document aDoc = XMLFactory.newDocument ();
return write (aObject, TransformResultFactory.create (aDoc)).isSuccess () ? aDoc : null;
}
/**
* Convert the passed object to a new micro document.
*
* @param aObject
* The object to be converted. May not be null
.
* @return null
if converting the document failed.
*/
@Nullable
default IMicroDocument getAsMicroDocument (@Nonnull final JAXBTYPE aObject)
{
final MicroSAXHandler aHandler = new MicroSAXHandler (false, null);
return write (aObject, aHandler).isSuccess () ? aHandler.getDocument () : null;
}
/**
* Utility method to directly convert the passed domain object to an XML
* string.
*
* @param aObject
* The domain object to be converted. May not be null
.
* @return null
if the passed domain object could not be
* converted because of validation errors.
*/
@Nullable
default String getAsString (@Nonnull final JAXBTYPE aObject)
{
final NonBlockingStringWriter aSW = new NonBlockingStringWriter ();
return write (aObject, TransformResultFactory.create (aSW)).isSuccess () ? aSW.getAsString () : null;
}
/**
* Write the passed object to a {@link ByteBuffer} and return it.
*
* @param aObject
* The object to be written. May not be null
.
* @return null
if the passed domain object could not be
* converted because of validation errors.
*/
@Nullable
default ByteBuffer getAsByteBuffer (@Nonnull final JAXBTYPE aObject)
{
final ByteBufferOutputStream aBBOS = new ByteBufferOutputStream ();
return write (aObject, aBBOS).isFailure () ? null : aBBOS.getBuffer ();
}
/**
* Write the passed object to a byte array and return the created byte array.
*
* @param aObject
* The object to be written. May not be null
.
* @return null
if the passed domain object could not be
* converted because of validation errors.
*/
@Nullable
default byte [] getAsBytes (@Nonnull final JAXBTYPE aObject)
{
final ByteBufferOutputStream aBBOS = new ByteBufferOutputStream ();
return write (aObject, aBBOS).isFailure () ? null : aBBOS.getAsByteArray ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy