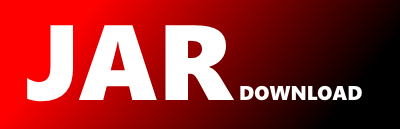
org.unece.cefact.namespaces.sbdh.StandardBusinessDocumentHeader Maven / Gradle / Ivy
Show all versions of ph-sbdh Show documentation
package org.unece.cefact.namespaces.sbdh;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.commons.annotation.ReturnsMutableCopy;
import com.helger.commons.annotation.ReturnsMutableObject;
import com.helger.commons.equals.EqualsHelper;
import com.helger.commons.hashcode.HashCodeGenerator;
import com.helger.commons.lang.IExplicitlyCloneable;
import com.helger.commons.string.ToStringGenerator;
/**
* Java class for StandardBusinessDocumentHeader complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="StandardBusinessDocumentHeader">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="HeaderVersion" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Sender" type="{http://www.unece.org/cefact/namespaces/StandardBusinessDocumentHeader}Partner" maxOccurs="unbounded"/>
* <element name="Receiver" type="{http://www.unece.org/cefact/namespaces/StandardBusinessDocumentHeader}Partner" maxOccurs="unbounded"/>
* <element name="DocumentIdentification" type="{http://www.unece.org/cefact/namespaces/StandardBusinessDocumentHeader}DocumentIdentification"/>
* <element name="Manifest" type="{http://www.unece.org/cefact/namespaces/StandardBusinessDocumentHeader}Manifest" minOccurs="0"/>
* <element name="BusinessScope" type="{http://www.unece.org/cefact/namespaces/StandardBusinessDocumentHeader}BusinessScope" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
* This class was annotated by ph-jaxb22-plugin -Xph-annotate
* This class contains methods created by ph-jaxb22-plugin -Xph-equalshashcode
* This class contains methods created by ph-jaxb22-plugin -Xph-tostring
* This class contains methods created by ph-jaxb22-plugin -Xph-list-extension
* This class contains methods created by ph-jaxb22-plugin -Xph-cloneable2
* This class contains methods created by ph-jaxb22-plugin -Xph-value-extender
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "StandardBusinessDocumentHeader", propOrder = {
"headerVersion",
"sender",
"receiver",
"documentIdentification",
"manifest",
"businessScope"
})
@CodingStyleguideUnaware
public class StandardBusinessDocumentHeader implements Serializable, IExplicitlyCloneable
{
@XmlElement(name = "HeaderVersion", required = true)
private String headerVersion;
@XmlElement(name = "Sender", required = true)
private List sender;
@XmlElement(name = "Receiver", required = true)
private List receiver;
@XmlElement(name = "DocumentIdentification", required = true)
private DocumentIdentification documentIdentification;
@XmlElement(name = "Manifest")
private Manifest manifest;
@XmlElement(name = "BusinessScope")
private BusinessScope businessScope;
/**
* Default constructor
* Note: automatically created by ph-jaxb22-plugin -Xph-value-extender
*
*/
public StandardBusinessDocumentHeader() {
}
/**
* Gets the value of the headerVersion property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getHeaderVersion() {
return headerVersion;
}
/**
* Sets the value of the headerVersion property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHeaderVersion(
@Nullable
String value) {
this.headerVersion = value;
}
/**
* Gets the value of the sender property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the sender property.
*
*
* For example, to add a new item, do as follows:
*
* getSender().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Partner }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getSender() {
if (sender == null) {
sender = new ArrayList();
}
return this.sender;
}
/**
* Gets the value of the receiver property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the receiver property.
*
*
* For example, to add a new item, do as follows:
*
* getReceiver().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Partner }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getReceiver() {
if (receiver == null) {
receiver = new ArrayList();
}
return this.receiver;
}
/**
* Gets the value of the documentIdentification property.
*
* @return
* possible object is
* {@link DocumentIdentification }
*
*/
@Nullable
public DocumentIdentification getDocumentIdentification() {
return documentIdentification;
}
/**
* Sets the value of the documentIdentification property.
*
* @param value
* allowed object is
* {@link DocumentIdentification }
*
*/
public void setDocumentIdentification(
@Nullable
DocumentIdentification value) {
this.documentIdentification = value;
}
/**
* Gets the value of the manifest property.
*
* @return
* possible object is
* {@link Manifest }
*
*/
@Nullable
public Manifest getManifest() {
return manifest;
}
/**
* Sets the value of the manifest property.
*
* @param value
* allowed object is
* {@link Manifest }
*
*/
public void setManifest(
@Nullable
Manifest value) {
this.manifest = value;
}
/**
* Gets the value of the businessScope property.
*
* @return
* possible object is
* {@link BusinessScope }
*
*/
@Nullable
public BusinessScope getBusinessScope() {
return businessScope;
}
/**
* Sets the value of the businessScope property.
*
* @param value
* allowed object is
* {@link BusinessScope }
*
*/
public void setBusinessScope(
@Nullable
BusinessScope value) {
this.businessScope = value;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public boolean equals(final Object o) {
if (o == this) {
return true;
}
if ((o == null)||(!getClass().equals(o.getClass()))) {
return false;
}
final StandardBusinessDocumentHeader rhs = ((StandardBusinessDocumentHeader) o);
if (!EqualsHelper.equals(businessScope, rhs.businessScope)) {
return false;
}
if (!EqualsHelper.equals(documentIdentification, rhs.documentIdentification)) {
return false;
}
if (!EqualsHelper.equals(headerVersion, rhs.headerVersion)) {
return false;
}
if (!EqualsHelper.equals(manifest, rhs.manifest)) {
return false;
}
if (!EqualsHelper.equalsCollection(receiver, rhs.receiver)) {
return false;
}
if (!EqualsHelper.equalsCollection(sender, rhs.sender)) {
return false;
}
return true;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public int hashCode() {
return new HashCodeGenerator(this).append(businessScope).append(documentIdentification).append(headerVersion).append(manifest).append(receiver).append(sender).getHashCode();
}
/**
* Created by ph-jaxb22-plugin -Xph-tostring
*
*/
@Override
public String toString() {
return new ToStringGenerator(this).append("businessScope", businessScope).append("documentIdentification", documentIdentification).append("headerVersion", headerVersion).append("manifest", manifest).append("receiver", receiver).append("sender", sender).getToString();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setSender(
@Nullable
final List aList) {
sender = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setReceiver(
@Nullable
final List aList) {
receiver = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasSenderEntries() {
return (!getSender().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoSenderEntries() {
return getSender().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getSenderCount() {
return getSender().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public Partner getSenderAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getSender().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addSender(
@Nonnull
final Partner elem) {
getSender().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasReceiverEntries() {
return (!getReceiver().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoReceiverEntries() {
return getReceiver().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getReceiverCount() {
return getReceiver().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public Partner getReceiverAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getReceiver().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addReceiver(
@Nonnull
final Partner elem) {
getReceiver().add(elem);
}
/**
* This method clones all values from this
to the passed object. All data in the parameter object is overwritten!Created by ph-jaxb22-plugin -Xph-cloneable2
*
* @param ret
* The target object to clone to. May not be null
.
*/
public void cloneTo(
@Nonnull
StandardBusinessDocumentHeader ret) {
ret.businessScope = ((businessScope == null)?null:businessScope.clone());
ret.documentIdentification = ((documentIdentification == null)?null:documentIdentification.clone());
ret.headerVersion = headerVersion;
ret.manifest = ((manifest == null)?null:manifest.clone());
if (receiver == null) {
ret.receiver = null;
} else {
List retReceiver = new ArrayList();
for (Partner aItem: getReceiver()) {
retReceiver.add(((aItem == null)?null:aItem.clone()));
}
ret.receiver = retReceiver;
}
if (sender == null) {
ret.sender = null;
} else {
List retSender = new ArrayList();
for (Partner aItem: getSender()) {
retSender.add(((aItem == null)?null:aItem.clone()));
}
ret.sender = retSender;
}
}
/**
* Created by ph-jaxb22-plugin -Xph-cloneable2
*
* @return
* The cloned object. Never null
.
*/
@Nonnull
@ReturnsMutableCopy
@Override
public StandardBusinessDocumentHeader clone() {
StandardBusinessDocumentHeader ret = new StandardBusinessDocumentHeader();
cloneTo(ret);
return ret;
}
}