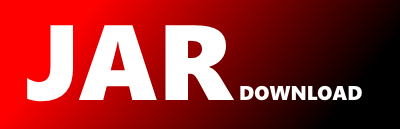
com.helger.schematron.pure.bound.xpath.PSXPathBoundAssertReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-schematron Show documentation
Show all versions of ph-schematron Show documentation
Library for validating XML documents with Schematron
/**
* Copyright (C) 2014-2020 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.schematron.pure.bound.xpath;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.concurrent.Immutable;
import javax.xml.xpath.XPathExpression;
import com.helger.commons.ValueEnforcer;
import com.helger.commons.annotation.ReturnsMutableCopy;
import com.helger.commons.collection.impl.ICommonsList;
import com.helger.commons.collection.impl.ICommonsMap;
import com.helger.commons.string.ToStringGenerator;
import com.helger.schematron.pure.model.PSAssertReport;
/**
* This class represents a single XPath-bound assert- or report-element.
*
* @author Philip Helger
*/
@Immutable
public class PSXPathBoundAssertReport
{
private final PSAssertReport m_aAssertReport;
private final String m_sTestExpression;
private final XPathExpression m_aBoundTestExpression;
private final ICommonsList m_aBoundContent;
private final ICommonsMap m_aBoundDiagnostics;
public PSXPathBoundAssertReport (@Nonnull final PSAssertReport aAssertReport,
@Nonnull final String sTestExpression,
@Nonnull final XPathExpression aBoundTestExpression,
@Nonnull final ICommonsList aBoundContent,
@Nonnull final ICommonsMap aBoundDiagnostics)
{
ValueEnforcer.notNull (aAssertReport, "AssertReport");
ValueEnforcer.notNull (sTestExpression, "TestExpression");
ValueEnforcer.notNull (aBoundTestExpression, "BoundTestExpression");
ValueEnforcer.notNull (aBoundContent, "BoundContent");
ValueEnforcer.notNull (aBoundDiagnostics, "BoundDiagnostics");
m_aAssertReport = aAssertReport;
m_sTestExpression = sTestExpression;
m_aBoundTestExpression = aBoundTestExpression;
m_aBoundContent = aBoundContent;
m_aBoundDiagnostics = aBoundDiagnostics;
}
/**
* @return The original assert/report element. Never null
.
*/
@Nonnull
public final PSAssertReport getAssertReport ()
{
return m_aAssertReport;
}
/**
* @return The source XPath expression that was compiled. Never
* null
.
*/
@Nonnull
public final String getTestExpression ()
{
return m_sTestExpression;
}
/**
* @return The pre-compiled XPath expression. Never null
.
*/
@Nonnull
public final XPathExpression getBoundTestExpression ()
{
return m_aBoundTestExpression;
}
/**
* @return All contained bound elements. It has the same amount of elements as
* the source assert/report.
*/
@Nonnull
@ReturnsMutableCopy
public final ICommonsList getAllBoundContentElements ()
{
return m_aBoundContent.getClone ();
}
/**
* Get the bound diagnostic matching the passed ID
*
* @param sID
* The ID to be resolved. May be null
.
* @return null
if the passed ID could not be resolved.
*/
@Nullable
public final PSXPathBoundDiagnostic getBoundDiagnosticOfID (@Nullable final String sID)
{
return m_aBoundDiagnostics.get (sID);
}
/**
* Get all bound diagnostics
*
* @return A copy of all bound diagnostics. Never null
but maybe
* empty.
*/
@Nullable
public final ICommonsMap getAllBoundDiagnostics ()
{
return m_aBoundDiagnostics.getClone ();
}
@Override
public String toString ()
{
return new ToStringGenerator (this).append ("assertReport", m_aAssertReport)
.append ("testExpression", m_sTestExpression)
.append ("boundTestExpression", m_aBoundTestExpression)
.append ("boundContent", m_aBoundContent)
.append ("boundDiagnostics", m_aBoundDiagnostics)
.getToString ();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy