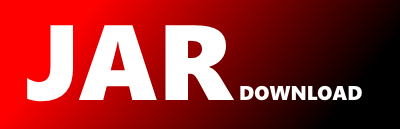
com.helger.schematron.svrl.jaxb.ActivePattern Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-schematron Show documentation
Show all versions of ph-schematron Show documentation
Library for validating XML documents with Schematron
package com.helger.schematron.svrl.jaxb;
import java.io.Serializable;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.commons.annotation.ReturnsMutableCopy;
import com.helger.commons.equals.EqualsHelper;
import com.helger.commons.hashcode.HashCodeGenerator;
import com.helger.commons.lang.IExplicitlyCloneable;
import com.helger.commons.string.ToStringGenerator;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://purl.oclc.org/dsdl/svrl}text" minOccurs="0"/>
* </sequence>
* <attribute name="id" type="{http://www.w3.org/2001/XMLSchema}NCName" />
* <attribute name="documents" type="{http://www.w3.org/2001/XMLSchema}anySimpleType" />
* <attribute name="name" type="{http://www.w3.org/2001/XMLSchema}anySimpleType" />
* <attribute name="role" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" />
* <attribute name="document" type="{http://www.w3.org/2001/XMLSchema}anySimpleType" />
* </restriction>
* </complexContent>
* </complexType>
*
* This class was annotated by ph-jaxb22-plugin -Xph-annotate
* This class contains methods created by ph-jaxb22-plugin -Xph-equalshashcode
* This class contains methods created by ph-jaxb22-plugin -Xph-tostring
* This class contains methods created by ph-jaxb22-plugin -Xph-cloneable2
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"text"
})
@XmlRootElement(name = "active-pattern")
@CodingStyleguideUnaware
public class ActivePattern implements Serializable, IExplicitlyCloneable
{
private Text text;
@XmlAttribute(name = "id")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NCName")
private String id;
@XmlAttribute(name = "documents")
@XmlSchemaType(name = "anySimpleType")
private String documents;
@XmlAttribute(name = "name")
@XmlSchemaType(name = "anySimpleType")
private String name;
@XmlAttribute(name = "role")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
private String role;
@XmlAttribute(name = "document")
@XmlSchemaType(name = "anySimpleType")
private String document;
/**
* Gets the value of the text property.
*
* @return
* possible object is
* {@link Text }
*
*/
@Nullable
public Text getText() {
return text;
}
/**
* Sets the value of the text property.
*
* @param value
* allowed object is
* {@link Text }
*
*/
public void setText(
@Nullable
Text value) {
this.text = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(
@Nullable
String value) {
this.id = value;
}
/**
* Gets the value of the documents property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getDocuments() {
return documents;
}
/**
* Sets the value of the documents property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDocuments(
@Nullable
String value) {
this.documents = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(
@Nullable
String value) {
this.name = value;
}
/**
* Gets the value of the role property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getRole() {
return role;
}
/**
* Sets the value of the role property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRole(
@Nullable
String value) {
this.role = value;
}
/**
* Gets the value of the document property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getDocument() {
return document;
}
/**
* Sets the value of the document property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDocument(
@Nullable
String value) {
this.document = value;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public boolean equals(final Object o) {
if (o == this) {
return true;
}
if ((o == null)||(!getClass().equals(o.getClass()))) {
return false;
}
final ActivePattern rhs = ((ActivePattern) o);
if (!EqualsHelper.equals(document, rhs.document)) {
return false;
}
if (!EqualsHelper.equals(documents, rhs.documents)) {
return false;
}
if (!EqualsHelper.equals(id, rhs.id)) {
return false;
}
if (!EqualsHelper.equals(name, rhs.name)) {
return false;
}
if (!EqualsHelper.equals(role, rhs.role)) {
return false;
}
if (!EqualsHelper.equals(text, rhs.text)) {
return false;
}
return true;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public int hashCode() {
return new HashCodeGenerator(this).append(document).append(documents).append(id).append(name).append(role).append(text).getHashCode();
}
/**
* Created by ph-jaxb22-plugin -Xph-tostring
*
*/
@Override
public String toString() {
return new ToStringGenerator(this).append("document", document).append("documents", documents).append("id", id).append("name", name).append("role", role).append("text", text).getToString();
}
/**
* This method clones all values from this
to the passed object. All data in the parameter object is overwritten!Created by ph-jaxb22-plugin -Xph-cloneable2
*
* @param ret
* The target object to clone to. May not be null
.
*/
public void cloneTo(
@Nonnull
ActivePattern ret) {
ret.document = document;
ret.documents = documents;
ret.id = id;
ret.name = name;
ret.role = role;
ret.text = ((text == null)?null:text.clone());
}
/**
* Created by ph-jaxb22-plugin -Xph-cloneable2
*
* @return
* The cloned object. Never null
.
*/
@Nonnull
@ReturnsMutableCopy
@Override
public ActivePattern clone() {
ActivePattern ret = new ActivePattern();
cloneTo(ret);
return ret;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy