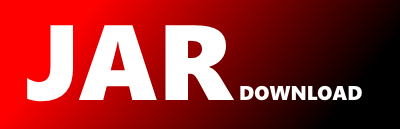
com.helger.schematron.svrl.jaxb.FailedAssert Maven / Gradle / Ivy
Show all versions of ph-schematron Show documentation
package com.helger.schematron.svrl.jaxb;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.commons.annotation.ReturnsMutableCopy;
import com.helger.commons.annotation.ReturnsMutableObject;
import com.helger.commons.equals.EqualsHelper;
import com.helger.commons.hashcode.HashCodeGenerator;
import com.helger.commons.lang.IExplicitlyCloneable;
import com.helger.commons.string.ToStringGenerator;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://purl.oclc.org/dsdl/svrl}diagnostic-reference" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://purl.oclc.org/dsdl/svrl}property-reference" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://purl.oclc.org/dsdl/svrl}text"/>
* </sequence>
* <attGroup ref="{http://purl.oclc.org/dsdl/svrl}attlist.assert-and-report"/>
* </restriction>
* </complexContent>
* </complexType>
*
* This class was annotated by ph-jaxb22-plugin -Xph-annotate
* This class contains methods created by ph-jaxb22-plugin -Xph-equalshashcode
* This class contains methods created by ph-jaxb22-plugin -Xph-tostring
* This class contains methods created by ph-jaxb22-plugin -Xph-list-extension
* This class contains methods created by ph-jaxb22-plugin -Xph-cloneable2
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"diagnosticReference",
"propertyReference",
"text"
})
@XmlRootElement(name = "failed-assert")
@CodingStyleguideUnaware
public class FailedAssert implements Serializable, IExplicitlyCloneable
{
@XmlElement(name = "diagnostic-reference")
private List diagnosticReference;
@XmlElement(name = "property-reference")
private List propertyReference;
@XmlElement(required = true)
private Text text;
@XmlAttribute(name = "id")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NCName")
private String id;
@XmlAttribute(name = "location", required = true)
@XmlSchemaType(name = "anySimpleType")
private String location;
@XmlAttribute(name = "test", required = true)
@XmlSchemaType(name = "anySimpleType")
private String test;
@XmlAttribute(name = "role")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
private String role;
@XmlAttribute(name = "flag")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
private String flag;
/**
* Gets the value of the diagnosticReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the diagnosticReference property.
*
*
* For example, to add a new item, do as follows:
*
* getDiagnosticReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DiagnosticReference }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getDiagnosticReference() {
if (diagnosticReference == null) {
diagnosticReference = new ArrayList();
}
return this.diagnosticReference;
}
/**
* Gets the value of the propertyReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the propertyReference property.
*
*
* For example, to add a new item, do as follows:
*
* getPropertyReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PropertyReference }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getPropertyReference() {
if (propertyReference == null) {
propertyReference = new ArrayList();
}
return this.propertyReference;
}
/**
* Gets the value of the text property.
*
* @return
* possible object is
* {@link Text }
*
*/
@Nullable
public Text getText() {
return text;
}
/**
* Sets the value of the text property.
*
* @param value
* allowed object is
* {@link Text }
*
*/
public void setText(
@Nullable
Text value) {
this.text = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(
@Nullable
String value) {
this.id = value;
}
/**
* Gets the value of the location property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getLocation() {
return location;
}
/**
* Sets the value of the location property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLocation(
@Nullable
String value) {
this.location = value;
}
/**
* Gets the value of the test property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getTest() {
return test;
}
/**
* Sets the value of the test property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTest(
@Nullable
String value) {
this.test = value;
}
/**
* Gets the value of the role property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getRole() {
return role;
}
/**
* Sets the value of the role property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRole(
@Nullable
String value) {
this.role = value;
}
/**
* Gets the value of the flag property.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public String getFlag() {
return flag;
}
/**
* Sets the value of the flag property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFlag(
@Nullable
String value) {
this.flag = value;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public boolean equals(final Object o) {
if (o == this) {
return true;
}
if ((o == null)||(!getClass().equals(o.getClass()))) {
return false;
}
final FailedAssert rhs = ((FailedAssert) o);
if (!EqualsHelper.equalsCollection(diagnosticReference, rhs.diagnosticReference)) {
return false;
}
if (!EqualsHelper.equals(flag, rhs.flag)) {
return false;
}
if (!EqualsHelper.equals(id, rhs.id)) {
return false;
}
if (!EqualsHelper.equals(location, rhs.location)) {
return false;
}
if (!EqualsHelper.equalsCollection(propertyReference, rhs.propertyReference)) {
return false;
}
if (!EqualsHelper.equals(role, rhs.role)) {
return false;
}
if (!EqualsHelper.equals(test, rhs.test)) {
return false;
}
if (!EqualsHelper.equals(text, rhs.text)) {
return false;
}
return true;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public int hashCode() {
return new HashCodeGenerator(this).append(diagnosticReference).append(flag).append(id).append(location).append(propertyReference).append(role).append(test).append(text).getHashCode();
}
/**
* Created by ph-jaxb22-plugin -Xph-tostring
*
*/
@Override
public String toString() {
return new ToStringGenerator(this).append("diagnosticReference", diagnosticReference).append("flag", flag).append("id", id).append("location", location).append("propertyReference", propertyReference).append("role", role).append("test", test).append("text", text).getToString();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setDiagnosticReference(
@Nullable
final List aList) {
diagnosticReference = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setPropertyReference(
@Nullable
final List aList) {
propertyReference = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasDiagnosticReferenceEntries() {
return (!getDiagnosticReference().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoDiagnosticReferenceEntries() {
return getDiagnosticReference().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getDiagnosticReferenceCount() {
return getDiagnosticReference().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public DiagnosticReference getDiagnosticReferenceAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getDiagnosticReference().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addDiagnosticReference(
@Nonnull
final DiagnosticReference elem) {
getDiagnosticReference().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasPropertyReferenceEntries() {
return (!getPropertyReference().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoPropertyReferenceEntries() {
return getPropertyReference().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getPropertyReferenceCount() {
return getPropertyReference().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public PropertyReference getPropertyReferenceAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getPropertyReference().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addPropertyReference(
@Nonnull
final PropertyReference elem) {
getPropertyReference().add(elem);
}
/**
* This method clones all values from this
to the passed object. All data in the parameter object is overwritten!Created by ph-jaxb22-plugin -Xph-cloneable2
*
* @param ret
* The target object to clone to. May not be null
.
*/
public void cloneTo(
@Nonnull
FailedAssert ret) {
if (diagnosticReference == null) {
ret.diagnosticReference = null;
} else {
List retDiagnosticReference = new ArrayList();
for (DiagnosticReference aItem: getDiagnosticReference()) {
retDiagnosticReference.add(((aItem == null)?null:aItem.clone()));
}
ret.diagnosticReference = retDiagnosticReference;
}
ret.flag = flag;
ret.id = id;
ret.location = location;
if (propertyReference == null) {
ret.propertyReference = null;
} else {
List retPropertyReference = new ArrayList();
for (PropertyReference aItem: getPropertyReference()) {
retPropertyReference.add(((aItem == null)?null:aItem.clone()));
}
ret.propertyReference = retPropertyReference;
}
ret.role = role;
ret.test = test;
ret.text = ((text == null)?null:text.clone());
}
/**
* Created by ph-jaxb22-plugin -Xph-cloneable2
*
* @return
* The cloned object. Never null
.
*/
@Nonnull
@ReturnsMutableCopy
@Override
public FailedAssert clone() {
FailedAssert ret = new FailedAssert();
cloneTo(ret);
return ret;
}
}