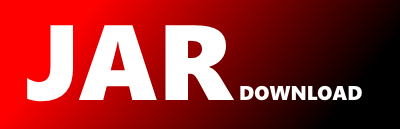
com.helger.xsds.bdxr.smp1.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-xsds-bdxr-smp1 Show documentation
Show all versions of ph-xsds-bdxr-smp1 Show documentation
OASIS BDXR SMP v1 wrapped in JAXB
package com.helger.xsds.bdxr.smp1;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.xsds.bdxr.smp1 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _ServiceGroup_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "ServiceGroup");
public final static QName _ServiceMetadata_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "ServiceMetadata");
public final static QName _SignedServiceMetadata_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "SignedServiceMetadata");
public final static QName _ParticipantIdentifier_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "ParticipantIdentifier");
public final static QName _DocumentIdentifier_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "DocumentIdentifier");
public final static QName _ProcessIdentifier_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "ProcessIdentifier");
public final static QName _RecipientIdentifier_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "RecipientIdentifier");
public final static QName _SenderIdentifier_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "SenderIdentifier");
public final static QName _ServiceGroupReferenceList_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "ServiceGroupReferenceList");
public final static QName _CompleteServiceGroup_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", "CompleteServiceGroup");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.xsds.bdxr.smp1
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ServiceGroupType }
*
* @return
* The created ServiceGroupType object and never null
.
*/
@Nonnull
public ServiceGroupType createServiceGroupType() {
return new ServiceGroupType();
}
/**
* Create an instance of {@link ServiceMetadataType }
*
* @return
* The created ServiceMetadataType object and never null
.
*/
@Nonnull
public ServiceMetadataType createServiceMetadataType() {
return new ServiceMetadataType();
}
/**
* Create an instance of {@link SignedServiceMetadataType }
*
* @return
* The created SignedServiceMetadataType object and never null
.
*/
@Nonnull
public SignedServiceMetadataType createSignedServiceMetadataType() {
return new SignedServiceMetadataType();
}
/**
* Create an instance of {@link ParticipantIdentifierType }
*
* @return
* The created ParticipantIdentifierType object and never null
.
*/
@Nonnull
public ParticipantIdentifierType createParticipantIdentifierType() {
return new ParticipantIdentifierType();
}
/**
* Create an instance of {@link DocumentIdentifierType }
*
* @return
* The created DocumentIdentifierType object and never null
.
*/
@Nonnull
public DocumentIdentifierType createDocumentIdentifierType() {
return new DocumentIdentifierType();
}
/**
* Create an instance of {@link ProcessIdentifierType }
*
* @return
* The created ProcessIdentifierType object and never null
.
*/
@Nonnull
public ProcessIdentifierType createProcessIdentifierType() {
return new ProcessIdentifierType();
}
/**
* Create an instance of {@link ServiceGroupReferenceListType }
*
* @return
* The created ServiceGroupReferenceListType object and never null
.
*/
@Nonnull
public ServiceGroupReferenceListType createServiceGroupReferenceListType() {
return new ServiceGroupReferenceListType();
}
/**
* Create an instance of {@link CompleteServiceGroupType }
*
* @return
* The created CompleteServiceGroupType object and never null
.
*/
@Nonnull
public CompleteServiceGroupType createCompleteServiceGroupType() {
return new CompleteServiceGroupType();
}
/**
* Create an instance of {@link ServiceInformationType }
*
* @return
* The created ServiceInformationType object and never null
.
*/
@Nonnull
public ServiceInformationType createServiceInformationType() {
return new ServiceInformationType();
}
/**
* Create an instance of {@link ProcessListType }
*
* @return
* The created ProcessListType object and never null
.
*/
@Nonnull
public ProcessListType createProcessListType() {
return new ProcessListType();
}
/**
* Create an instance of {@link ProcessType }
*
* @return
* The created ProcessType object and never null
.
*/
@Nonnull
public ProcessType createProcessType() {
return new ProcessType();
}
/**
* Create an instance of {@link ServiceEndpointList }
*
* @return
* The created ServiceEndpointList object and never null
.
*/
@Nonnull
public ServiceEndpointList createServiceEndpointList() {
return new ServiceEndpointList();
}
/**
* Create an instance of {@link EndpointType }
*
* @return
* The created EndpointType object and never null
.
*/
@Nonnull
public EndpointType createEndpointType() {
return new EndpointType();
}
/**
* Create an instance of {@link ServiceMetadataReferenceCollectionType }
*
* @return
* The created ServiceMetadataReferenceCollectionType object and never null
.
*/
@Nonnull
public ServiceMetadataReferenceCollectionType createServiceMetadataReferenceCollectionType() {
return new ServiceMetadataReferenceCollectionType();
}
/**
* Create an instance of {@link ServiceMetadataReferenceType }
*
* @return
* The created ServiceMetadataReferenceType object and never null
.
*/
@Nonnull
public ServiceMetadataReferenceType createServiceMetadataReferenceType() {
return new ServiceMetadataReferenceType();
}
/**
* Create an instance of {@link RedirectType }
*
* @return
* The created RedirectType object and never null
.
*/
@Nonnull
public RedirectType createRedirectType() {
return new RedirectType();
}
/**
* Create an instance of {@link ExtensionType }
*
* @return
* The created ExtensionType object and never null
.
*/
@Nonnull
public ExtensionType createExtensionType() {
return new ExtensionType();
}
/**
* Create an instance of {@link ServiceGroupReferenceType }
*
* @return
* The created ServiceGroupReferenceType object and never null
.
*/
@Nonnull
public ServiceGroupReferenceType createServiceGroupReferenceType() {
return new ServiceGroupReferenceType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ServiceGroupType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ServiceGroupType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "ServiceGroup")
@Nonnull
public JAXBElement createServiceGroup(
@Nullable
final ServiceGroupType value) {
return new JAXBElement(_ServiceGroup_QNAME, ServiceGroupType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ServiceMetadataType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ServiceMetadataType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "ServiceMetadata")
@Nonnull
public JAXBElement createServiceMetadata(
@Nullable
final ServiceMetadataType value) {
return new JAXBElement(_ServiceMetadata_QNAME, ServiceMetadataType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SignedServiceMetadataType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SignedServiceMetadataType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "SignedServiceMetadata")
@Nonnull
public JAXBElement createSignedServiceMetadata(
@Nullable
final SignedServiceMetadataType value) {
return new JAXBElement(_SignedServiceMetadata_QNAME, SignedServiceMetadataType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ParticipantIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ParticipantIdentifierType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "ParticipantIdentifier")
@Nonnull
public JAXBElement createParticipantIdentifier(
@Nullable
final ParticipantIdentifierType value) {
return new JAXBElement(_ParticipantIdentifier_QNAME, ParticipantIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DocumentIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DocumentIdentifierType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "DocumentIdentifier")
@Nonnull
public JAXBElement createDocumentIdentifier(
@Nullable
final DocumentIdentifierType value) {
return new JAXBElement(_DocumentIdentifier_QNAME, DocumentIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ProcessIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ProcessIdentifierType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "ProcessIdentifier")
@Nonnull
public JAXBElement createProcessIdentifier(
@Nullable
final ProcessIdentifierType value) {
return new JAXBElement(_ProcessIdentifier_QNAME, ProcessIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ParticipantIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ParticipantIdentifierType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "RecipientIdentifier")
@Nonnull
public JAXBElement createRecipientIdentifier(
@Nullable
final ParticipantIdentifierType value) {
return new JAXBElement(_RecipientIdentifier_QNAME, ParticipantIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ParticipantIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ParticipantIdentifierType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "SenderIdentifier")
@Nonnull
public JAXBElement createSenderIdentifier(
@Nullable
final ParticipantIdentifierType value) {
return new JAXBElement(_SenderIdentifier_QNAME, ParticipantIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ServiceGroupReferenceListType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ServiceGroupReferenceListType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "ServiceGroupReferenceList")
@Nonnull
public JAXBElement createServiceGroupReferenceList(
@Nullable
final ServiceGroupReferenceListType value) {
return new JAXBElement(_ServiceGroupReferenceList_QNAME, ServiceGroupReferenceListType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link CompleteServiceGroupType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link CompleteServiceGroupType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2016/05", name = "CompleteServiceGroup")
@Nonnull
public JAXBElement createCompleteServiceGroup(
@Nullable
final CompleteServiceGroupType value) {
return new JAXBElement(_CompleteServiceGroup_QNAME, CompleteServiceGroupType.class, null, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy