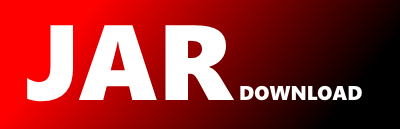
com.helger.xsds.bdxr.smp2.bc.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-xsds-bdxr-smp2 Show documentation
Show all versions of ph-xsds-bdxr-smp2 Show documentation
OASIS BDXR SMP v1 wrapped in JAXB
package com.helger.xsds.bdxr.smp2.bc;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.xsds.bdxr.smp2.bc package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _ActivationDate_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "ActivationDate");
public final static QName _AddressURI_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "AddressURI");
public final static QName _Contact_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "Contact");
public final static QName _ContentBinaryObject_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "ContentBinaryObject");
public final static QName _Description_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "Description");
public final static QName _ExpirationDate_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "ExpirationDate");
public final static QName _ID_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "ID");
public final static QName _ParticipantID_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "ParticipantID");
public final static QName _PublisherURI_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "PublisherURI");
public final static QName _RoleID_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "RoleID");
public final static QName _SMPVersionID_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "SMPVersionID");
public final static QName _TransportProfileID_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "TransportProfileID");
public final static QName _TypeCode_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", "TypeCode");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.xsds.bdxr.smp2.bc
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ActivationDateType }
*
* @return
* The created ActivationDateType object and never null
.
*/
@Nonnull
public ActivationDateType createActivationDateType() {
return new ActivationDateType();
}
/**
* Create an instance of {@link AddressURIType }
*
* @return
* The created AddressURIType object and never null
.
*/
@Nonnull
public AddressURIType createAddressURIType() {
return new AddressURIType();
}
/**
* Create an instance of {@link ContactType }
*
* @return
* The created ContactType object and never null
.
*/
@Nonnull
public ContactType createContactType() {
return new ContactType();
}
/**
* Create an instance of {@link ContentBinaryObjectType }
*
* @return
* The created ContentBinaryObjectType object and never null
.
*/
@Nonnull
public ContentBinaryObjectType createContentBinaryObjectType() {
return new ContentBinaryObjectType();
}
/**
* Create an instance of {@link DescriptionType }
*
* @return
* The created DescriptionType object and never null
.
*/
@Nonnull
public DescriptionType createDescriptionType() {
return new DescriptionType();
}
/**
* Create an instance of {@link ExpirationDateType }
*
* @return
* The created ExpirationDateType object and never null
.
*/
@Nonnull
public ExpirationDateType createExpirationDateType() {
return new ExpirationDateType();
}
/**
* Create an instance of {@link IDType }
*
* @return
* The created IDType object and never null
.
*/
@Nonnull
public IDType createIDType() {
return new IDType();
}
/**
* Create an instance of {@link ParticipantIDType }
*
* @return
* The created ParticipantIDType object and never null
.
*/
@Nonnull
public ParticipantIDType createParticipantIDType() {
return new ParticipantIDType();
}
/**
* Create an instance of {@link PublisherURIType }
*
* @return
* The created PublisherURIType object and never null
.
*/
@Nonnull
public PublisherURIType createPublisherURIType() {
return new PublisherURIType();
}
/**
* Create an instance of {@link RoleIDType }
*
* @return
* The created RoleIDType object and never null
.
*/
@Nonnull
public RoleIDType createRoleIDType() {
return new RoleIDType();
}
/**
* Create an instance of {@link SMPVersionIDType }
*
* @return
* The created SMPVersionIDType object and never null
.
*/
@Nonnull
public SMPVersionIDType createSMPVersionIDType() {
return new SMPVersionIDType();
}
/**
* Create an instance of {@link TransportProfileIDType }
*
* @return
* The created TransportProfileIDType object and never null
.
*/
@Nonnull
public TransportProfileIDType createTransportProfileIDType() {
return new TransportProfileIDType();
}
/**
* Create an instance of {@link TypeCodeType }
*
* @return
* The created TypeCodeType object and never null
.
*/
@Nonnull
public TypeCodeType createTypeCodeType() {
return new TypeCodeType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ActivationDateType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ActivationDateType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "ActivationDate")
@Nonnull
public JAXBElement createActivationDate(
@Nullable
final ActivationDateType value) {
return new JAXBElement(_ActivationDate_QNAME, ActivationDateType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AddressURIType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AddressURIType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "AddressURI")
@Nonnull
public JAXBElement createAddressURI(
@Nullable
final AddressURIType value) {
return new JAXBElement(_AddressURI_QNAME, AddressURIType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ContactType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ContactType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "Contact")
@Nonnull
public JAXBElement createContact(
@Nullable
final ContactType value) {
return new JAXBElement(_Contact_QNAME, ContactType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ContentBinaryObjectType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ContentBinaryObjectType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "ContentBinaryObject")
@Nonnull
public JAXBElement createContentBinaryObject(
@Nullable
final ContentBinaryObjectType value) {
return new JAXBElement(_ContentBinaryObject_QNAME, ContentBinaryObjectType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DescriptionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DescriptionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "Description")
@Nonnull
public JAXBElement createDescription(
@Nullable
final DescriptionType value) {
return new JAXBElement(_Description_QNAME, DescriptionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExpirationDateType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExpirationDateType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "ExpirationDate")
@Nonnull
public JAXBElement createExpirationDate(
@Nullable
final ExpirationDateType value) {
return new JAXBElement(_ExpirationDate_QNAME, ExpirationDateType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IDType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link IDType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "ID")
@Nonnull
public JAXBElement createID(
@Nullable
final IDType value) {
return new JAXBElement(_ID_QNAME, IDType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ParticipantIDType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ParticipantIDType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "ParticipantID")
@Nonnull
public JAXBElement createParticipantID(
@Nullable
final ParticipantIDType value) {
return new JAXBElement(_ParticipantID_QNAME, ParticipantIDType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PublisherURIType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link PublisherURIType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "PublisherURI")
@Nonnull
public JAXBElement createPublisherURI(
@Nullable
final PublisherURIType value) {
return new JAXBElement(_PublisherURI_QNAME, PublisherURIType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link RoleIDType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link RoleIDType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "RoleID")
@Nonnull
public JAXBElement createRoleID(
@Nullable
final RoleIDType value) {
return new JAXBElement(_RoleID_QNAME, RoleIDType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SMPVersionIDType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SMPVersionIDType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "SMPVersionID")
@Nonnull
public JAXBElement createSMPVersionID(
@Nullable
final SMPVersionIDType value) {
return new JAXBElement(_SMPVersionID_QNAME, SMPVersionIDType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TransportProfileIDType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TransportProfileIDType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "TransportProfileID")
@Nonnull
public JAXBElement createTransportProfileID(
@Nullable
final TransportProfileIDType value) {
return new JAXBElement(_TransportProfileID_QNAME, TransportProfileIDType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TypeCodeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TypeCodeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/BasicComponents", name = "TypeCode")
@Nonnull
public JAXBElement createTypeCode(
@Nullable
final TypeCodeType value) {
return new JAXBElement(_TypeCode_QNAME, TypeCodeType.class, null, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy