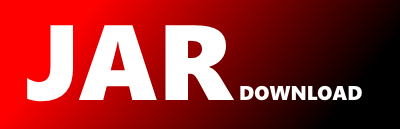
com.helger.xsds.bdxr.smp2.ec.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-xsds-bdxr-smp2 Show documentation
Show all versions of ph-xsds-bdxr-smp2 Show documentation
OASIS BDXR SMP v1 wrapped in JAXB
package com.helger.xsds.bdxr.smp2.ec;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.xsds.bdxr.smp2.ec package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _SMPExtensions_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "SMPExtensions");
public final static QName _SMPExtension_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "SMPExtension");
public final static QName _Name_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "Name");
public final static QName _ExtensionAgencyID_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "ExtensionAgencyID");
public final static QName _ExtensionAgencyName_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "ExtensionAgencyName");
public final static QName _ExtensionAgencyURI_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "ExtensionAgencyURI");
public final static QName _ExtensionContent_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "ExtensionContent");
public final static QName _ExtensionReason_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "ExtensionReason");
public final static QName _ExtensionReasonCode_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "ExtensionReasonCode");
public final static QName _ExtensionURI_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "ExtensionURI");
public final static QName _ExtensionVersionID_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", "ExtensionVersionID");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.xsds.bdxr.smp2.ec
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link SMPExtensionsType }
*
* @return
* The created SMPExtensionsType object and never null
.
*/
@Nonnull
public SMPExtensionsType createSMPExtensionsType() {
return new SMPExtensionsType();
}
/**
* Create an instance of {@link SMPExtensionType }
*
* @return
* The created SMPExtensionType object and never null
.
*/
@Nonnull
public SMPExtensionType createSMPExtensionType() {
return new SMPExtensionType();
}
/**
* Create an instance of {@link NameType }
*
* @return
* The created NameType object and never null
.
*/
@Nonnull
public NameType createNameType() {
return new NameType();
}
/**
* Create an instance of {@link ExtensionAgencyIDType }
*
* @return
* The created ExtensionAgencyIDType object and never null
.
*/
@Nonnull
public ExtensionAgencyIDType createExtensionAgencyIDType() {
return new ExtensionAgencyIDType();
}
/**
* Create an instance of {@link ExtensionAgencyNameType }
*
* @return
* The created ExtensionAgencyNameType object and never null
.
*/
@Nonnull
public ExtensionAgencyNameType createExtensionAgencyNameType() {
return new ExtensionAgencyNameType();
}
/**
* Create an instance of {@link ExtensionAgencyURIType }
*
* @return
* The created ExtensionAgencyURIType object and never null
.
*/
@Nonnull
public ExtensionAgencyURIType createExtensionAgencyURIType() {
return new ExtensionAgencyURIType();
}
/**
* Create an instance of {@link ExtensionContentType }
*
* @return
* The created ExtensionContentType object and never null
.
*/
@Nonnull
public ExtensionContentType createExtensionContentType() {
return new ExtensionContentType();
}
/**
* Create an instance of {@link ExtensionReasonType }
*
* @return
* The created ExtensionReasonType object and never null
.
*/
@Nonnull
public ExtensionReasonType createExtensionReasonType() {
return new ExtensionReasonType();
}
/**
* Create an instance of {@link ExtensionReasonCodeType }
*
* @return
* The created ExtensionReasonCodeType object and never null
.
*/
@Nonnull
public ExtensionReasonCodeType createExtensionReasonCodeType() {
return new ExtensionReasonCodeType();
}
/**
* Create an instance of {@link ExtensionURIType }
*
* @return
* The created ExtensionURIType object and never null
.
*/
@Nonnull
public ExtensionURIType createExtensionURIType() {
return new ExtensionURIType();
}
/**
* Create an instance of {@link ExtensionVersionIDType }
*
* @return
* The created ExtensionVersionIDType object and never null
.
*/
@Nonnull
public ExtensionVersionIDType createExtensionVersionIDType() {
return new ExtensionVersionIDType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SMPExtensionsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SMPExtensionsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "SMPExtensions")
@Nonnull
public JAXBElement createSMPExtensions(
@Nullable
final SMPExtensionsType value) {
return new JAXBElement(_SMPExtensions_QNAME, SMPExtensionsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SMPExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SMPExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "SMPExtension")
@Nonnull
public JAXBElement createSMPExtension(
@Nullable
final SMPExtensionType value) {
return new JAXBElement(_SMPExtension_QNAME, SMPExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link NameType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link NameType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "Name")
@Nonnull
public JAXBElement createName(
@Nullable
final NameType value) {
return new JAXBElement(_Name_QNAME, NameType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtensionAgencyIDType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExtensionAgencyIDType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "ExtensionAgencyID")
@Nonnull
public JAXBElement createExtensionAgencyID(
@Nullable
final ExtensionAgencyIDType value) {
return new JAXBElement(_ExtensionAgencyID_QNAME, ExtensionAgencyIDType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtensionAgencyNameType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExtensionAgencyNameType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "ExtensionAgencyName")
@Nonnull
public JAXBElement createExtensionAgencyName(
@Nullable
final ExtensionAgencyNameType value) {
return new JAXBElement(_ExtensionAgencyName_QNAME, ExtensionAgencyNameType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtensionAgencyURIType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExtensionAgencyURIType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "ExtensionAgencyURI")
@Nonnull
public JAXBElement createExtensionAgencyURI(
@Nullable
final ExtensionAgencyURIType value) {
return new JAXBElement(_ExtensionAgencyURI_QNAME, ExtensionAgencyURIType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtensionContentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExtensionContentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "ExtensionContent")
@Nonnull
public JAXBElement createExtensionContent(
@Nullable
final ExtensionContentType value) {
return new JAXBElement(_ExtensionContent_QNAME, ExtensionContentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtensionReasonType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExtensionReasonType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "ExtensionReason")
@Nonnull
public JAXBElement createExtensionReason(
@Nullable
final ExtensionReasonType value) {
return new JAXBElement(_ExtensionReason_QNAME, ExtensionReasonType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtensionReasonCodeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExtensionReasonCodeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "ExtensionReasonCode")
@Nonnull
public JAXBElement createExtensionReasonCode(
@Nullable
final ExtensionReasonCodeType value) {
return new JAXBElement(_ExtensionReasonCode_QNAME, ExtensionReasonCodeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtensionURIType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExtensionURIType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "ExtensionURI")
@Nonnull
public JAXBElement createExtensionURI(
@Nullable
final ExtensionURIType value) {
return new JAXBElement(_ExtensionURI_QNAME, ExtensionURIType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExtensionVersionIDType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExtensionVersionIDType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/SMP/2/ExtensionComponents", name = "ExtensionVersionID")
@Nonnull
public JAXBElement createExtensionVersionID(
@Nullable
final ExtensionVersionIDType value) {
return new JAXBElement(_ExtensionVersionID_QNAME, ExtensionVersionIDType.class, null, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy