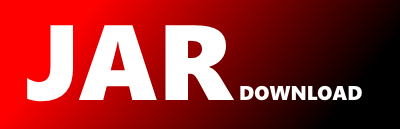
com.hellosign.openapi.model.BulkSendJobGetResponseSignatureRequests Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hellosign-java-sdk Show documentation
Show all versions of hellosign-java-sdk Show documentation
Use the HelloSign Java SDK to connect your Java app to HelloSign's service in microseconds!
The newest version!
/*
* HelloSign API
* HelloSign v3 API
*
* The version of the OpenAPI document: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.hellosign.openapi.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.hellosign.openapi.model.SignatureRequestResponse;
import com.hellosign.openapi.model.SignatureRequestResponseAttachment;
import com.hellosign.openapi.model.SignatureRequestResponseCustomFieldBase;
import com.hellosign.openapi.model.SignatureRequestResponseDataBase;
import com.hellosign.openapi.model.SignatureRequestResponseSignatures;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.hellosign.openapi.JSON;
import com.hellosign.openapi.ApiException;
/**
* BulkSendJobGetResponseSignatureRequests
*/
@JsonPropertyOrder({
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_TEST_MODE,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_SIGNATURE_REQUEST_ID,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_REQUESTER_EMAIL_ADDRESS,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_TITLE,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_ORIGINAL_TITLE,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_SUBJECT,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_MESSAGE,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_METADATA,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_CREATED_AT,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_IS_COMPLETE,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_IS_DECLINED,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_HAS_ERROR,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_FILES_URL,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_SIGNING_URL,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_DETAILS_URL,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_CC_EMAIL_ADDRESSES,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_SIGNING_REDIRECT_URL,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_TEMPLATE_IDS,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_CUSTOM_FIELDS,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_ATTACHMENTS,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_RESPONSE_DATA,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_SIGNATURES,
BulkSendJobGetResponseSignatureRequests.JSON_PROPERTY_BULK_SEND_JOB_ID
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class BulkSendJobGetResponseSignatureRequests {
public static final String JSON_PROPERTY_TEST_MODE = "test_mode";
private Boolean testMode = false;
public static final String JSON_PROPERTY_SIGNATURE_REQUEST_ID = "signature_request_id";
private String signatureRequestId;
public static final String JSON_PROPERTY_REQUESTER_EMAIL_ADDRESS = "requester_email_address";
private String requesterEmailAddress;
public static final String JSON_PROPERTY_TITLE = "title";
private String title;
public static final String JSON_PROPERTY_ORIGINAL_TITLE = "original_title";
private String originalTitle;
public static final String JSON_PROPERTY_SUBJECT = "subject";
private String subject;
public static final String JSON_PROPERTY_MESSAGE = "message";
private String message;
public static final String JSON_PROPERTY_METADATA = "metadata";
private Object metadata;
public static final String JSON_PROPERTY_CREATED_AT = "created_at";
private Integer createdAt;
public static final String JSON_PROPERTY_IS_COMPLETE = "is_complete";
private Boolean isComplete;
public static final String JSON_PROPERTY_IS_DECLINED = "is_declined";
private Boolean isDeclined;
public static final String JSON_PROPERTY_HAS_ERROR = "has_error";
private Boolean hasError;
public static final String JSON_PROPERTY_FILES_URL = "files_url";
private String filesUrl;
public static final String JSON_PROPERTY_SIGNING_URL = "signing_url";
private String signingUrl;
public static final String JSON_PROPERTY_DETAILS_URL = "details_url";
private String detailsUrl;
public static final String JSON_PROPERTY_CC_EMAIL_ADDRESSES = "cc_email_addresses";
private List ccEmailAddresses = null;
public static final String JSON_PROPERTY_SIGNING_REDIRECT_URL = "signing_redirect_url";
private String signingRedirectUrl;
public static final String JSON_PROPERTY_TEMPLATE_IDS = "template_ids";
private List templateIds = null;
public static final String JSON_PROPERTY_CUSTOM_FIELDS = "custom_fields";
private List customFields = null;
public static final String JSON_PROPERTY_ATTACHMENTS = "attachments";
private List attachments = null;
public static final String JSON_PROPERTY_RESPONSE_DATA = "response_data";
private List responseData = null;
public static final String JSON_PROPERTY_SIGNATURES = "signatures";
private List signatures = null;
public static final String JSON_PROPERTY_BULK_SEND_JOB_ID = "bulk_send_job_id";
private String bulkSendJobId;
public BulkSendJobGetResponseSignatureRequests() {
}
public BulkSendJobGetResponseSignatureRequests testMode(Boolean testMode) {
this.testMode = testMode;
return this;
}
/**
* Whether this is a test signature request. Test requests have no legal value. Defaults to `false`.
* @return testMode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether this is a test signature request. Test requests have no legal value. Defaults to `false`.")
@JsonProperty(JSON_PROPERTY_TEST_MODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getTestMode() {
return testMode;
}
@JsonProperty(JSON_PROPERTY_TEST_MODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTestMode(Boolean testMode) {
this.testMode = testMode;
}
public BulkSendJobGetResponseSignatureRequests signatureRequestId(String signatureRequestId) {
this.signatureRequestId = signatureRequestId;
return this;
}
/**
* The id of the SignatureRequest.
* @return signatureRequestId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The id of the SignatureRequest.")
@JsonProperty(JSON_PROPERTY_SIGNATURE_REQUEST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSignatureRequestId() {
return signatureRequestId;
}
@JsonProperty(JSON_PROPERTY_SIGNATURE_REQUEST_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSignatureRequestId(String signatureRequestId) {
this.signatureRequestId = signatureRequestId;
}
public BulkSendJobGetResponseSignatureRequests requesterEmailAddress(String requesterEmailAddress) {
this.requesterEmailAddress = requesterEmailAddress;
return this;
}
/**
* The email address of the initiator of the SignatureRequest.
* @return requesterEmailAddress
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The email address of the initiator of the SignatureRequest.")
@JsonProperty(JSON_PROPERTY_REQUESTER_EMAIL_ADDRESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRequesterEmailAddress() {
return requesterEmailAddress;
}
@JsonProperty(JSON_PROPERTY_REQUESTER_EMAIL_ADDRESS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRequesterEmailAddress(String requesterEmailAddress) {
this.requesterEmailAddress = requesterEmailAddress;
}
public BulkSendJobGetResponseSignatureRequests title(String title) {
this.title = title;
return this;
}
/**
* The title the specified Account uses for the SignatureRequest.
* @return title
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The title the specified Account uses for the SignatureRequest.")
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTitle(String title) {
this.title = title;
}
public BulkSendJobGetResponseSignatureRequests originalTitle(String originalTitle) {
this.originalTitle = originalTitle;
return this;
}
/**
* Default Label for account.
* @return originalTitle
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Default Label for account.")
@JsonProperty(JSON_PROPERTY_ORIGINAL_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOriginalTitle() {
return originalTitle;
}
@JsonProperty(JSON_PROPERTY_ORIGINAL_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOriginalTitle(String originalTitle) {
this.originalTitle = originalTitle;
}
public BulkSendJobGetResponseSignatureRequests subject(String subject) {
this.subject = subject;
return this;
}
/**
* The subject in the email that was initially sent to the signers.
* @return subject
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The subject in the email that was initially sent to the signers.")
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSubject() {
return subject;
}
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSubject(String subject) {
this.subject = subject;
}
public BulkSendJobGetResponseSignatureRequests message(String message) {
this.message = message;
return this;
}
/**
* The custom message in the email that was initially sent to the signers.
* @return message
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom message in the email that was initially sent to the signers.")
@JsonProperty(JSON_PROPERTY_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMessage() {
return message;
}
@JsonProperty(JSON_PROPERTY_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMessage(String message) {
this.message = message;
}
public BulkSendJobGetResponseSignatureRequests metadata(Object metadata) {
this.metadata = metadata;
return this;
}
/**
* The metadata attached to the signature request.
* @return metadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The metadata attached to the signature request.")
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Object getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(Object metadata) {
this.metadata = metadata;
}
public BulkSendJobGetResponseSignatureRequests createdAt(Integer createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Time the signature request was created.
* @return createdAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Time the signature request was created.")
@JsonProperty(JSON_PROPERTY_CREATED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getCreatedAt() {
return createdAt;
}
@JsonProperty(JSON_PROPERTY_CREATED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCreatedAt(Integer createdAt) {
this.createdAt = createdAt;
}
public BulkSendJobGetResponseSignatureRequests isComplete(Boolean isComplete) {
this.isComplete = isComplete;
return this;
}
/**
* Whether or not the SignatureRequest has been fully executed by all signers.
* @return isComplete
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether or not the SignatureRequest has been fully executed by all signers.")
@JsonProperty(JSON_PROPERTY_IS_COMPLETE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsComplete() {
return isComplete;
}
@JsonProperty(JSON_PROPERTY_IS_COMPLETE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsComplete(Boolean isComplete) {
this.isComplete = isComplete;
}
public BulkSendJobGetResponseSignatureRequests isDeclined(Boolean isDeclined) {
this.isDeclined = isDeclined;
return this;
}
/**
* Whether or not the SignatureRequest has been declined by a signer.
* @return isDeclined
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether or not the SignatureRequest has been declined by a signer.")
@JsonProperty(JSON_PROPERTY_IS_DECLINED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsDeclined() {
return isDeclined;
}
@JsonProperty(JSON_PROPERTY_IS_DECLINED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsDeclined(Boolean isDeclined) {
this.isDeclined = isDeclined;
}
public BulkSendJobGetResponseSignatureRequests hasError(Boolean hasError) {
this.hasError = hasError;
return this;
}
/**
* Whether or not an error occurred (either during the creation of the SignatureRequest or during one of the signings).
* @return hasError
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether or not an error occurred (either during the creation of the SignatureRequest or during one of the signings).")
@JsonProperty(JSON_PROPERTY_HAS_ERROR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getHasError() {
return hasError;
}
@JsonProperty(JSON_PROPERTY_HAS_ERROR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHasError(Boolean hasError) {
this.hasError = hasError;
}
public BulkSendJobGetResponseSignatureRequests filesUrl(String filesUrl) {
this.filesUrl = filesUrl;
return this;
}
/**
* The URL where a copy of the request's documents can be downloaded.
* @return filesUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL where a copy of the request's documents can be downloaded.")
@JsonProperty(JSON_PROPERTY_FILES_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFilesUrl() {
return filesUrl;
}
@JsonProperty(JSON_PROPERTY_FILES_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFilesUrl(String filesUrl) {
this.filesUrl = filesUrl;
}
public BulkSendJobGetResponseSignatureRequests signingUrl(String signingUrl) {
this.signingUrl = signingUrl;
return this;
}
/**
* The URL where a signer, after authenticating, can sign the documents. This should only be used by users with existing HelloSign accounts as they will be required to log in before signing.
* @return signingUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL where a signer, after authenticating, can sign the documents. This should only be used by users with existing HelloSign accounts as they will be required to log in before signing.")
@JsonProperty(JSON_PROPERTY_SIGNING_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSigningUrl() {
return signingUrl;
}
@JsonProperty(JSON_PROPERTY_SIGNING_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSigningUrl(String signingUrl) {
this.signingUrl = signingUrl;
}
public BulkSendJobGetResponseSignatureRequests detailsUrl(String detailsUrl) {
this.detailsUrl = detailsUrl;
return this;
}
/**
* The URL where the requester and the signers can view the current status of the SignatureRequest.
* @return detailsUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL where the requester and the signers can view the current status of the SignatureRequest.")
@JsonProperty(JSON_PROPERTY_DETAILS_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDetailsUrl() {
return detailsUrl;
}
@JsonProperty(JSON_PROPERTY_DETAILS_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDetailsUrl(String detailsUrl) {
this.detailsUrl = detailsUrl;
}
public BulkSendJobGetResponseSignatureRequests ccEmailAddresses(List ccEmailAddresses) {
this.ccEmailAddresses = ccEmailAddresses;
return this;
}
public BulkSendJobGetResponseSignatureRequests addCcEmailAddressesItem(String ccEmailAddressesItem) {
if (this.ccEmailAddresses == null) {
this.ccEmailAddresses = new ArrayList<>();
}
this.ccEmailAddresses.add(ccEmailAddressesItem);
return this;
}
/**
* A list of email addresses that were CCed on the SignatureRequest. They will receive a copy of the final PDF once all the signers have signed.
* @return ccEmailAddresses
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of email addresses that were CCed on the SignatureRequest. They will receive a copy of the final PDF once all the signers have signed.")
@JsonProperty(JSON_PROPERTY_CC_EMAIL_ADDRESSES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCcEmailAddresses() {
return ccEmailAddresses;
}
@JsonProperty(JSON_PROPERTY_CC_EMAIL_ADDRESSES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCcEmailAddresses(List ccEmailAddresses) {
this.ccEmailAddresses = ccEmailAddresses;
}
public BulkSendJobGetResponseSignatureRequests signingRedirectUrl(String signingRedirectUrl) {
this.signingRedirectUrl = signingRedirectUrl;
return this;
}
/**
* The URL you want the signer redirected to after they successfully sign.
* @return signingRedirectUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL you want the signer redirected to after they successfully sign.")
@JsonProperty(JSON_PROPERTY_SIGNING_REDIRECT_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSigningRedirectUrl() {
return signingRedirectUrl;
}
@JsonProperty(JSON_PROPERTY_SIGNING_REDIRECT_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSigningRedirectUrl(String signingRedirectUrl) {
this.signingRedirectUrl = signingRedirectUrl;
}
public BulkSendJobGetResponseSignatureRequests templateIds(List templateIds) {
this.templateIds = templateIds;
return this;
}
public BulkSendJobGetResponseSignatureRequests addTemplateIdsItem(String templateIdsItem) {
if (this.templateIds == null) {
this.templateIds = new ArrayList<>();
}
this.templateIds.add(templateIdsItem);
return this;
}
/**
* Templates IDs used in this SignatureRequest (if any).
* @return templateIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Templates IDs used in this SignatureRequest (if any).")
@JsonProperty(JSON_PROPERTY_TEMPLATE_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTemplateIds() {
return templateIds;
}
@JsonProperty(JSON_PROPERTY_TEMPLATE_IDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTemplateIds(List templateIds) {
this.templateIds = templateIds;
}
public BulkSendJobGetResponseSignatureRequests customFields(List customFields) {
this.customFields = customFields;
return this;
}
public BulkSendJobGetResponseSignatureRequests addCustomFieldsItem(SignatureRequestResponseCustomFieldBase customFieldsItem) {
if (this.customFields == null) {
this.customFields = new ArrayList<>();
}
this.customFields.add(customFieldsItem);
return this;
}
/**
* An array of Custom Field objects containing the name and type of each custom field. * Text Field uses `SignatureRequestResponseCustomFieldText` * Checkbox Field uses `SignatureRequestResponseCustomFieldCheckbox`
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of Custom Field objects containing the name and type of each custom field. * Text Field uses `SignatureRequestResponseCustomFieldText` * Checkbox Field uses `SignatureRequestResponseCustomFieldCheckbox`")
@JsonProperty(JSON_PROPERTY_CUSTOM_FIELDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCustomFields() {
return customFields;
}
@JsonProperty(JSON_PROPERTY_CUSTOM_FIELDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCustomFields(List customFields) {
this.customFields = customFields;
}
public BulkSendJobGetResponseSignatureRequests attachments(List attachments) {
this.attachments = attachments;
return this;
}
public BulkSendJobGetResponseSignatureRequests addAttachmentsItem(SignatureRequestResponseAttachment attachmentsItem) {
if (this.attachments == null) {
this.attachments = new ArrayList<>();
}
this.attachments.add(attachmentsItem);
return this;
}
/**
* Signer attachments.
* @return attachments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Signer attachments.")
@JsonProperty(JSON_PROPERTY_ATTACHMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAttachments() {
return attachments;
}
@JsonProperty(JSON_PROPERTY_ATTACHMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAttachments(List attachments) {
this.attachments = attachments;
}
public BulkSendJobGetResponseSignatureRequests responseData(List responseData) {
this.responseData = responseData;
return this;
}
public BulkSendJobGetResponseSignatureRequests addResponseDataItem(SignatureRequestResponseDataBase responseDataItem) {
if (this.responseData == null) {
this.responseData = new ArrayList<>();
}
this.responseData.add(responseDataItem);
return this;
}
/**
* An array of form field objects containing the name, value, and type of each textbox or checkmark field filled in by the signers.
* @return responseData
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of form field objects containing the name, value, and type of each textbox or checkmark field filled in by the signers.")
@JsonProperty(JSON_PROPERTY_RESPONSE_DATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getResponseData() {
return responseData;
}
@JsonProperty(JSON_PROPERTY_RESPONSE_DATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResponseData(List responseData) {
this.responseData = responseData;
}
public BulkSendJobGetResponseSignatureRequests signatures(List signatures) {
this.signatures = signatures;
return this;
}
public BulkSendJobGetResponseSignatureRequests addSignaturesItem(SignatureRequestResponseSignatures signaturesItem) {
if (this.signatures == null) {
this.signatures = new ArrayList<>();
}
this.signatures.add(signaturesItem);
return this;
}
/**
* An array of signature objects, 1 for each signer.
* @return signatures
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of signature objects, 1 for each signer.")
@JsonProperty(JSON_PROPERTY_SIGNATURES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSignatures() {
return signatures;
}
@JsonProperty(JSON_PROPERTY_SIGNATURES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSignatures(List signatures) {
this.signatures = signatures;
}
public BulkSendJobGetResponseSignatureRequests bulkSendJobId(String bulkSendJobId) {
this.bulkSendJobId = bulkSendJobId;
return this;
}
/**
* The id of the BulkSendJob.
* @return bulkSendJobId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The id of the BulkSendJob.")
@JsonProperty(JSON_PROPERTY_BULK_SEND_JOB_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getBulkSendJobId() {
return bulkSendJobId;
}
@JsonProperty(JSON_PROPERTY_BULK_SEND_JOB_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBulkSendJobId(String bulkSendJobId) {
this.bulkSendJobId = bulkSendJobId;
}
/**
* Return true if this BulkSendJobGetResponseSignatureRequests object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BulkSendJobGetResponseSignatureRequests bulkSendJobGetResponseSignatureRequests = (BulkSendJobGetResponseSignatureRequests) o;
return Objects.equals(this.testMode, bulkSendJobGetResponseSignatureRequests.testMode) &&
Objects.equals(this.signatureRequestId, bulkSendJobGetResponseSignatureRequests.signatureRequestId) &&
Objects.equals(this.requesterEmailAddress, bulkSendJobGetResponseSignatureRequests.requesterEmailAddress) &&
Objects.equals(this.title, bulkSendJobGetResponseSignatureRequests.title) &&
Objects.equals(this.originalTitle, bulkSendJobGetResponseSignatureRequests.originalTitle) &&
Objects.equals(this.subject, bulkSendJobGetResponseSignatureRequests.subject) &&
Objects.equals(this.message, bulkSendJobGetResponseSignatureRequests.message) &&
Objects.equals(this.metadata, bulkSendJobGetResponseSignatureRequests.metadata) &&
Objects.equals(this.createdAt, bulkSendJobGetResponseSignatureRequests.createdAt) &&
Objects.equals(this.isComplete, bulkSendJobGetResponseSignatureRequests.isComplete) &&
Objects.equals(this.isDeclined, bulkSendJobGetResponseSignatureRequests.isDeclined) &&
Objects.equals(this.hasError, bulkSendJobGetResponseSignatureRequests.hasError) &&
Objects.equals(this.filesUrl, bulkSendJobGetResponseSignatureRequests.filesUrl) &&
Objects.equals(this.signingUrl, bulkSendJobGetResponseSignatureRequests.signingUrl) &&
Objects.equals(this.detailsUrl, bulkSendJobGetResponseSignatureRequests.detailsUrl) &&
Objects.equals(this.ccEmailAddresses, bulkSendJobGetResponseSignatureRequests.ccEmailAddresses) &&
Objects.equals(this.signingRedirectUrl, bulkSendJobGetResponseSignatureRequests.signingRedirectUrl) &&
Objects.equals(this.templateIds, bulkSendJobGetResponseSignatureRequests.templateIds) &&
Objects.equals(this.customFields, bulkSendJobGetResponseSignatureRequests.customFields) &&
Objects.equals(this.attachments, bulkSendJobGetResponseSignatureRequests.attachments) &&
Objects.equals(this.responseData, bulkSendJobGetResponseSignatureRequests.responseData) &&
Objects.equals(this.signatures, bulkSendJobGetResponseSignatureRequests.signatures) &&
Objects.equals(this.bulkSendJobId, bulkSendJobGetResponseSignatureRequests.bulkSendJobId);
}
@Override
public int hashCode() {
return Objects.hash(testMode, signatureRequestId, requesterEmailAddress, title, originalTitle, subject, message, metadata, createdAt, isComplete, isDeclined, hasError, filesUrl, signingUrl, detailsUrl, ccEmailAddresses, signingRedirectUrl, templateIds, customFields, attachments, responseData, signatures, bulkSendJobId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BulkSendJobGetResponseSignatureRequests {\n");
sb.append(" testMode: ").append(toIndentedString(testMode)).append("\n");
sb.append(" signatureRequestId: ").append(toIndentedString(signatureRequestId)).append("\n");
sb.append(" requesterEmailAddress: ").append(toIndentedString(requesterEmailAddress)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" originalTitle: ").append(toIndentedString(originalTitle)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" isComplete: ").append(toIndentedString(isComplete)).append("\n");
sb.append(" isDeclined: ").append(toIndentedString(isDeclined)).append("\n");
sb.append(" hasError: ").append(toIndentedString(hasError)).append("\n");
sb.append(" filesUrl: ").append(toIndentedString(filesUrl)).append("\n");
sb.append(" signingUrl: ").append(toIndentedString(signingUrl)).append("\n");
sb.append(" detailsUrl: ").append(toIndentedString(detailsUrl)).append("\n");
sb.append(" ccEmailAddresses: ").append(toIndentedString(ccEmailAddresses)).append("\n");
sb.append(" signingRedirectUrl: ").append(toIndentedString(signingRedirectUrl)).append("\n");
sb.append(" templateIds: ").append(toIndentedString(templateIds)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" attachments: ").append(toIndentedString(attachments)).append("\n");
sb.append(" responseData: ").append(toIndentedString(responseData)).append("\n");
sb.append(" signatures: ").append(toIndentedString(signatures)).append("\n");
sb.append(" bulkSendJobId: ").append(toIndentedString(bulkSendJobId)).append("\n");
sb.append("}");
return sb.toString();
}
public Map createFormData() throws ApiException {
Map map = new HashMap<>();
boolean fileTypeFound = false;
try {
if (testMode != null) {
if (isFileTypeOrListOfFiles(testMode)) {
fileTypeFound = true;
}
if (testMode.getClass().equals(java.io.File.class) ||
testMode.getClass().equals(Integer.class) ||
testMode.getClass().equals(String.class) ) {
map.put("test_mode", testMode);
} else if (isListOfFile(testMode)) {
for(int i = 0; i< getListSize(testMode); i++) {
map.put("test_mode[" + i + "]", getFromList(testMode, i));
}
}
else {
map.put("test_mode", JSON.getDefault().getMapper().writeValueAsString(testMode));
}
}
if (signatureRequestId != null) {
if (isFileTypeOrListOfFiles(signatureRequestId)) {
fileTypeFound = true;
}
if (signatureRequestId.getClass().equals(java.io.File.class) ||
signatureRequestId.getClass().equals(Integer.class) ||
signatureRequestId.getClass().equals(String.class) ) {
map.put("signature_request_id", signatureRequestId);
} else if (isListOfFile(signatureRequestId)) {
for(int i = 0; i< getListSize(signatureRequestId); i++) {
map.put("signature_request_id[" + i + "]", getFromList(signatureRequestId, i));
}
}
else {
map.put("signature_request_id", JSON.getDefault().getMapper().writeValueAsString(signatureRequestId));
}
}
if (requesterEmailAddress != null) {
if (isFileTypeOrListOfFiles(requesterEmailAddress)) {
fileTypeFound = true;
}
if (requesterEmailAddress.getClass().equals(java.io.File.class) ||
requesterEmailAddress.getClass().equals(Integer.class) ||
requesterEmailAddress.getClass().equals(String.class) ) {
map.put("requester_email_address", requesterEmailAddress);
} else if (isListOfFile(requesterEmailAddress)) {
for(int i = 0; i< getListSize(requesterEmailAddress); i++) {
map.put("requester_email_address[" + i + "]", getFromList(requesterEmailAddress, i));
}
}
else {
map.put("requester_email_address", JSON.getDefault().getMapper().writeValueAsString(requesterEmailAddress));
}
}
if (title != null) {
if (isFileTypeOrListOfFiles(title)) {
fileTypeFound = true;
}
if (title.getClass().equals(java.io.File.class) ||
title.getClass().equals(Integer.class) ||
title.getClass().equals(String.class) ) {
map.put("title", title);
} else if (isListOfFile(title)) {
for(int i = 0; i< getListSize(title); i++) {
map.put("title[" + i + "]", getFromList(title, i));
}
}
else {
map.put("title", JSON.getDefault().getMapper().writeValueAsString(title));
}
}
if (originalTitle != null) {
if (isFileTypeOrListOfFiles(originalTitle)) {
fileTypeFound = true;
}
if (originalTitle.getClass().equals(java.io.File.class) ||
originalTitle.getClass().equals(Integer.class) ||
originalTitle.getClass().equals(String.class) ) {
map.put("original_title", originalTitle);
} else if (isListOfFile(originalTitle)) {
for(int i = 0; i< getListSize(originalTitle); i++) {
map.put("original_title[" + i + "]", getFromList(originalTitle, i));
}
}
else {
map.put("original_title", JSON.getDefault().getMapper().writeValueAsString(originalTitle));
}
}
if (subject != null) {
if (isFileTypeOrListOfFiles(subject)) {
fileTypeFound = true;
}
if (subject.getClass().equals(java.io.File.class) ||
subject.getClass().equals(Integer.class) ||
subject.getClass().equals(String.class) ) {
map.put("subject", subject);
} else if (isListOfFile(subject)) {
for(int i = 0; i< getListSize(subject); i++) {
map.put("subject[" + i + "]", getFromList(subject, i));
}
}
else {
map.put("subject", JSON.getDefault().getMapper().writeValueAsString(subject));
}
}
if (message != null) {
if (isFileTypeOrListOfFiles(message)) {
fileTypeFound = true;
}
if (message.getClass().equals(java.io.File.class) ||
message.getClass().equals(Integer.class) ||
message.getClass().equals(String.class) ) {
map.put("message", message);
} else if (isListOfFile(message)) {
for(int i = 0; i< getListSize(message); i++) {
map.put("message[" + i + "]", getFromList(message, i));
}
}
else {
map.put("message", JSON.getDefault().getMapper().writeValueAsString(message));
}
}
if (metadata != null) {
if (isFileTypeOrListOfFiles(metadata)) {
fileTypeFound = true;
}
if (metadata.getClass().equals(java.io.File.class) ||
metadata.getClass().equals(Integer.class) ||
metadata.getClass().equals(String.class) ) {
map.put("metadata", metadata);
} else if (isListOfFile(metadata)) {
for(int i = 0; i< getListSize(metadata); i++) {
map.put("metadata[" + i + "]", getFromList(metadata, i));
}
}
else {
map.put("metadata", JSON.getDefault().getMapper().writeValueAsString(metadata));
}
}
if (createdAt != null) {
if (isFileTypeOrListOfFiles(createdAt)) {
fileTypeFound = true;
}
if (createdAt.getClass().equals(java.io.File.class) ||
createdAt.getClass().equals(Integer.class) ||
createdAt.getClass().equals(String.class) ) {
map.put("created_at", createdAt);
} else if (isListOfFile(createdAt)) {
for(int i = 0; i< getListSize(createdAt); i++) {
map.put("created_at[" + i + "]", getFromList(createdAt, i));
}
}
else {
map.put("created_at", JSON.getDefault().getMapper().writeValueAsString(createdAt));
}
}
if (isComplete != null) {
if (isFileTypeOrListOfFiles(isComplete)) {
fileTypeFound = true;
}
if (isComplete.getClass().equals(java.io.File.class) ||
isComplete.getClass().equals(Integer.class) ||
isComplete.getClass().equals(String.class) ) {
map.put("is_complete", isComplete);
} else if (isListOfFile(isComplete)) {
for(int i = 0; i< getListSize(isComplete); i++) {
map.put("is_complete[" + i + "]", getFromList(isComplete, i));
}
}
else {
map.put("is_complete", JSON.getDefault().getMapper().writeValueAsString(isComplete));
}
}
if (isDeclined != null) {
if (isFileTypeOrListOfFiles(isDeclined)) {
fileTypeFound = true;
}
if (isDeclined.getClass().equals(java.io.File.class) ||
isDeclined.getClass().equals(Integer.class) ||
isDeclined.getClass().equals(String.class) ) {
map.put("is_declined", isDeclined);
} else if (isListOfFile(isDeclined)) {
for(int i = 0; i< getListSize(isDeclined); i++) {
map.put("is_declined[" + i + "]", getFromList(isDeclined, i));
}
}
else {
map.put("is_declined", JSON.getDefault().getMapper().writeValueAsString(isDeclined));
}
}
if (hasError != null) {
if (isFileTypeOrListOfFiles(hasError)) {
fileTypeFound = true;
}
if (hasError.getClass().equals(java.io.File.class) ||
hasError.getClass().equals(Integer.class) ||
hasError.getClass().equals(String.class) ) {
map.put("has_error", hasError);
} else if (isListOfFile(hasError)) {
for(int i = 0; i< getListSize(hasError); i++) {
map.put("has_error[" + i + "]", getFromList(hasError, i));
}
}
else {
map.put("has_error", JSON.getDefault().getMapper().writeValueAsString(hasError));
}
}
if (filesUrl != null) {
if (isFileTypeOrListOfFiles(filesUrl)) {
fileTypeFound = true;
}
if (filesUrl.getClass().equals(java.io.File.class) ||
filesUrl.getClass().equals(Integer.class) ||
filesUrl.getClass().equals(String.class) ) {
map.put("files_url", filesUrl);
} else if (isListOfFile(filesUrl)) {
for(int i = 0; i< getListSize(filesUrl); i++) {
map.put("files_url[" + i + "]", getFromList(filesUrl, i));
}
}
else {
map.put("files_url", JSON.getDefault().getMapper().writeValueAsString(filesUrl));
}
}
if (signingUrl != null) {
if (isFileTypeOrListOfFiles(signingUrl)) {
fileTypeFound = true;
}
if (signingUrl.getClass().equals(java.io.File.class) ||
signingUrl.getClass().equals(Integer.class) ||
signingUrl.getClass().equals(String.class) ) {
map.put("signing_url", signingUrl);
} else if (isListOfFile(signingUrl)) {
for(int i = 0; i< getListSize(signingUrl); i++) {
map.put("signing_url[" + i + "]", getFromList(signingUrl, i));
}
}
else {
map.put("signing_url", JSON.getDefault().getMapper().writeValueAsString(signingUrl));
}
}
if (detailsUrl != null) {
if (isFileTypeOrListOfFiles(detailsUrl)) {
fileTypeFound = true;
}
if (detailsUrl.getClass().equals(java.io.File.class) ||
detailsUrl.getClass().equals(Integer.class) ||
detailsUrl.getClass().equals(String.class) ) {
map.put("details_url", detailsUrl);
} else if (isListOfFile(detailsUrl)) {
for(int i = 0; i< getListSize(detailsUrl); i++) {
map.put("details_url[" + i + "]", getFromList(detailsUrl, i));
}
}
else {
map.put("details_url", JSON.getDefault().getMapper().writeValueAsString(detailsUrl));
}
}
if (ccEmailAddresses != null) {
if (isFileTypeOrListOfFiles(ccEmailAddresses)) {
fileTypeFound = true;
}
if (ccEmailAddresses.getClass().equals(java.io.File.class) ||
ccEmailAddresses.getClass().equals(Integer.class) ||
ccEmailAddresses.getClass().equals(String.class) ) {
map.put("cc_email_addresses", ccEmailAddresses);
} else if (isListOfFile(ccEmailAddresses)) {
for(int i = 0; i< getListSize(ccEmailAddresses); i++) {
map.put("cc_email_addresses[" + i + "]", getFromList(ccEmailAddresses, i));
}
}
else {
map.put("cc_email_addresses", JSON.getDefault().getMapper().writeValueAsString(ccEmailAddresses));
}
}
if (signingRedirectUrl != null) {
if (isFileTypeOrListOfFiles(signingRedirectUrl)) {
fileTypeFound = true;
}
if (signingRedirectUrl.getClass().equals(java.io.File.class) ||
signingRedirectUrl.getClass().equals(Integer.class) ||
signingRedirectUrl.getClass().equals(String.class) ) {
map.put("signing_redirect_url", signingRedirectUrl);
} else if (isListOfFile(signingRedirectUrl)) {
for(int i = 0; i< getListSize(signingRedirectUrl); i++) {
map.put("signing_redirect_url[" + i + "]", getFromList(signingRedirectUrl, i));
}
}
else {
map.put("signing_redirect_url", JSON.getDefault().getMapper().writeValueAsString(signingRedirectUrl));
}
}
if (templateIds != null) {
if (isFileTypeOrListOfFiles(templateIds)) {
fileTypeFound = true;
}
if (templateIds.getClass().equals(java.io.File.class) ||
templateIds.getClass().equals(Integer.class) ||
templateIds.getClass().equals(String.class) ) {
map.put("template_ids", templateIds);
} else if (isListOfFile(templateIds)) {
for(int i = 0; i< getListSize(templateIds); i++) {
map.put("template_ids[" + i + "]", getFromList(templateIds, i));
}
}
else {
map.put("template_ids", JSON.getDefault().getMapper().writeValueAsString(templateIds));
}
}
if (customFields != null) {
if (isFileTypeOrListOfFiles(customFields)) {
fileTypeFound = true;
}
if (customFields.getClass().equals(java.io.File.class) ||
customFields.getClass().equals(Integer.class) ||
customFields.getClass().equals(String.class) ) {
map.put("custom_fields", customFields);
} else if (isListOfFile(customFields)) {
for(int i = 0; i< getListSize(customFields); i++) {
map.put("custom_fields[" + i + "]", getFromList(customFields, i));
}
}
else {
map.put("custom_fields", JSON.getDefault().getMapper().writeValueAsString(customFields));
}
}
if (attachments != null) {
if (isFileTypeOrListOfFiles(attachments)) {
fileTypeFound = true;
}
if (attachments.getClass().equals(java.io.File.class) ||
attachments.getClass().equals(Integer.class) ||
attachments.getClass().equals(String.class) ) {
map.put("attachments", attachments);
} else if (isListOfFile(attachments)) {
for(int i = 0; i< getListSize(attachments); i++) {
map.put("attachments[" + i + "]", getFromList(attachments, i));
}
}
else {
map.put("attachments", JSON.getDefault().getMapper().writeValueAsString(attachments));
}
}
if (responseData != null) {
if (isFileTypeOrListOfFiles(responseData)) {
fileTypeFound = true;
}
if (responseData.getClass().equals(java.io.File.class) ||
responseData.getClass().equals(Integer.class) ||
responseData.getClass().equals(String.class) ) {
map.put("response_data", responseData);
} else if (isListOfFile(responseData)) {
for(int i = 0; i< getListSize(responseData); i++) {
map.put("response_data[" + i + "]", getFromList(responseData, i));
}
}
else {
map.put("response_data", JSON.getDefault().getMapper().writeValueAsString(responseData));
}
}
if (signatures != null) {
if (isFileTypeOrListOfFiles(signatures)) {
fileTypeFound = true;
}
if (signatures.getClass().equals(java.io.File.class) ||
signatures.getClass().equals(Integer.class) ||
signatures.getClass().equals(String.class) ) {
map.put("signatures", signatures);
} else if (isListOfFile(signatures)) {
for(int i = 0; i< getListSize(signatures); i++) {
map.put("signatures[" + i + "]", getFromList(signatures, i));
}
}
else {
map.put("signatures", JSON.getDefault().getMapper().writeValueAsString(signatures));
}
}
if (bulkSendJobId != null) {
if (isFileTypeOrListOfFiles(bulkSendJobId)) {
fileTypeFound = true;
}
if (bulkSendJobId.getClass().equals(java.io.File.class) ||
bulkSendJobId.getClass().equals(Integer.class) ||
bulkSendJobId.getClass().equals(String.class) ) {
map.put("bulk_send_job_id", bulkSendJobId);
} else if (isListOfFile(bulkSendJobId)) {
for(int i = 0; i< getListSize(bulkSendJobId); i++) {
map.put("bulk_send_job_id[" + i + "]", getFromList(bulkSendJobId, i));
}
}
else {
map.put("bulk_send_job_id", JSON.getDefault().getMapper().writeValueAsString(bulkSendJobId));
}
}
} catch (Exception e) {
throw new ApiException(e);
}
return fileTypeFound ? map : new HashMap<>();
}
private boolean isFileTypeOrListOfFiles(Object obj) throws Exception {
return obj.getClass().equals(java.io.File.class) || isListOfFile(obj);
}
private boolean isListOfFile(Object obj) throws Exception {
return obj instanceof java.util.List && !isListEmpty(obj) && getFromList(obj, 0) instanceof java.io.File;
}
private boolean isListEmpty(Object obj) throws Exception {
return (boolean) Class.forName(java.util.List.class.getName()).getMethod("isEmpty").invoke(obj);
}
private Object getFromList(Object obj, int index) throws Exception {
return Class.forName(java.util.List.class.getName()).getMethod("get", int.class).invoke(obj, index);
}
private int getListSize(Object obj) throws Exception {
return (int) Class.forName(java.util.List.class.getName()).getMethod("size").invoke(obj);
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy