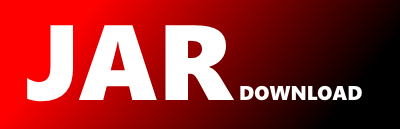
com.hellosign.openapi.model.OAuthTokenGenerateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hellosign-java-sdk Show documentation
Show all versions of hellosign-java-sdk Show documentation
Use the HelloSign Java SDK to connect your Java app to HelloSign's service in microseconds!
The newest version!
/*
* HelloSign API
* HelloSign v3 API
*
* The version of the OpenAPI document: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.hellosign.openapi.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.hellosign.openapi.JSON;
import com.hellosign.openapi.ApiException;
/**
* OAuthTokenGenerateRequest
*/
@JsonPropertyOrder({
OAuthTokenGenerateRequest.JSON_PROPERTY_CLIENT_ID,
OAuthTokenGenerateRequest.JSON_PROPERTY_CLIENT_SECRET,
OAuthTokenGenerateRequest.JSON_PROPERTY_CODE,
OAuthTokenGenerateRequest.JSON_PROPERTY_GRANT_TYPE,
OAuthTokenGenerateRequest.JSON_PROPERTY_STATE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class OAuthTokenGenerateRequest {
public static final String JSON_PROPERTY_CLIENT_ID = "client_id";
private String clientId;
public static final String JSON_PROPERTY_CLIENT_SECRET = "client_secret";
private String clientSecret;
public static final String JSON_PROPERTY_CODE = "code";
private String code;
public static final String JSON_PROPERTY_GRANT_TYPE = "grant_type";
private String grantType = "authorization_code";
public static final String JSON_PROPERTY_STATE = "state";
private String state;
public OAuthTokenGenerateRequest() {
}
public OAuthTokenGenerateRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* The client id of the app requesting authorization.
* @return clientId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The client id of the app requesting authorization.")
@JsonProperty(JSON_PROPERTY_CLIENT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getClientId() {
return clientId;
}
@JsonProperty(JSON_PROPERTY_CLIENT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setClientId(String clientId) {
this.clientId = clientId;
}
public OAuthTokenGenerateRequest clientSecret(String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
/**
* The secret token of your app.
* @return clientSecret
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The secret token of your app.")
@JsonProperty(JSON_PROPERTY_CLIENT_SECRET)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getClientSecret() {
return clientSecret;
}
@JsonProperty(JSON_PROPERTY_CLIENT_SECRET)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setClientSecret(String clientSecret) {
this.clientSecret = clientSecret;
}
public OAuthTokenGenerateRequest code(String code) {
this.code = code;
return this;
}
/**
* The code passed to your callback when the user granted access.
* @return code
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The code passed to your callback when the user granted access.")
@JsonProperty(JSON_PROPERTY_CODE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getCode() {
return code;
}
@JsonProperty(JSON_PROPERTY_CODE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCode(String code) {
this.code = code;
}
public OAuthTokenGenerateRequest grantType(String grantType) {
this.grantType = grantType;
return this;
}
/**
* When generating a new token use `authorization_code`.
* @return grantType
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "When generating a new token use `authorization_code`.")
@JsonProperty(JSON_PROPERTY_GRANT_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getGrantType() {
return grantType;
}
@JsonProperty(JSON_PROPERTY_GRANT_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setGrantType(String grantType) {
this.grantType = grantType;
}
public OAuthTokenGenerateRequest state(String state) {
this.state = state;
return this;
}
/**
* Same as the state you specified earlier.
* @return state
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Same as the state you specified earlier.")
@JsonProperty(JSON_PROPERTY_STATE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getState() {
return state;
}
@JsonProperty(JSON_PROPERTY_STATE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setState(String state) {
this.state = state;
}
/**
* Return true if this OAuthTokenGenerateRequest object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OAuthTokenGenerateRequest oauthTokenGenerateRequest = (OAuthTokenGenerateRequest) o;
return Objects.equals(this.clientId, oauthTokenGenerateRequest.clientId) &&
Objects.equals(this.clientSecret, oauthTokenGenerateRequest.clientSecret) &&
Objects.equals(this.code, oauthTokenGenerateRequest.code) &&
Objects.equals(this.grantType, oauthTokenGenerateRequest.grantType) &&
Objects.equals(this.state, oauthTokenGenerateRequest.state);
}
@Override
public int hashCode() {
return Objects.hash(clientId, clientSecret, code, grantType, state);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OAuthTokenGenerateRequest {\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" clientSecret: ").append(toIndentedString(clientSecret)).append("\n");
sb.append(" code: ").append(toIndentedString(code)).append("\n");
sb.append(" grantType: ").append(toIndentedString(grantType)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append("}");
return sb.toString();
}
public Map createFormData() throws ApiException {
Map map = new HashMap<>();
boolean fileTypeFound = false;
try {
if (clientId != null) {
if (isFileTypeOrListOfFiles(clientId)) {
fileTypeFound = true;
}
if (clientId.getClass().equals(java.io.File.class) ||
clientId.getClass().equals(Integer.class) ||
clientId.getClass().equals(String.class) ) {
map.put("client_id", clientId);
} else if (isListOfFile(clientId)) {
for(int i = 0; i< getListSize(clientId); i++) {
map.put("client_id[" + i + "]", getFromList(clientId, i));
}
}
else {
map.put("client_id", JSON.getDefault().getMapper().writeValueAsString(clientId));
}
}
if (clientSecret != null) {
if (isFileTypeOrListOfFiles(clientSecret)) {
fileTypeFound = true;
}
if (clientSecret.getClass().equals(java.io.File.class) ||
clientSecret.getClass().equals(Integer.class) ||
clientSecret.getClass().equals(String.class) ) {
map.put("client_secret", clientSecret);
} else if (isListOfFile(clientSecret)) {
for(int i = 0; i< getListSize(clientSecret); i++) {
map.put("client_secret[" + i + "]", getFromList(clientSecret, i));
}
}
else {
map.put("client_secret", JSON.getDefault().getMapper().writeValueAsString(clientSecret));
}
}
if (code != null) {
if (isFileTypeOrListOfFiles(code)) {
fileTypeFound = true;
}
if (code.getClass().equals(java.io.File.class) ||
code.getClass().equals(Integer.class) ||
code.getClass().equals(String.class) ) {
map.put("code", code);
} else if (isListOfFile(code)) {
for(int i = 0; i< getListSize(code); i++) {
map.put("code[" + i + "]", getFromList(code, i));
}
}
else {
map.put("code", JSON.getDefault().getMapper().writeValueAsString(code));
}
}
if (grantType != null) {
if (isFileTypeOrListOfFiles(grantType)) {
fileTypeFound = true;
}
if (grantType.getClass().equals(java.io.File.class) ||
grantType.getClass().equals(Integer.class) ||
grantType.getClass().equals(String.class) ) {
map.put("grant_type", grantType);
} else if (isListOfFile(grantType)) {
for(int i = 0; i< getListSize(grantType); i++) {
map.put("grant_type[" + i + "]", getFromList(grantType, i));
}
}
else {
map.put("grant_type", JSON.getDefault().getMapper().writeValueAsString(grantType));
}
}
if (state != null) {
if (isFileTypeOrListOfFiles(state)) {
fileTypeFound = true;
}
if (state.getClass().equals(java.io.File.class) ||
state.getClass().equals(Integer.class) ||
state.getClass().equals(String.class) ) {
map.put("state", state);
} else if (isListOfFile(state)) {
for(int i = 0; i< getListSize(state); i++) {
map.put("state[" + i + "]", getFromList(state, i));
}
}
else {
map.put("state", JSON.getDefault().getMapper().writeValueAsString(state));
}
}
} catch (Exception e) {
throw new ApiException(e);
}
return fileTypeFound ? map : new HashMap<>();
}
private boolean isFileTypeOrListOfFiles(Object obj) throws Exception {
return obj.getClass().equals(java.io.File.class) || isListOfFile(obj);
}
private boolean isListOfFile(Object obj) throws Exception {
return obj instanceof java.util.List && !isListEmpty(obj) && getFromList(obj, 0) instanceof java.io.File;
}
private boolean isListEmpty(Object obj) throws Exception {
return (boolean) Class.forName(java.util.List.class.getName()).getMethod("isEmpty").invoke(obj);
}
private Object getFromList(Object obj, int index) throws Exception {
return Class.forName(java.util.List.class.getName()).getMethod("get", int.class).invoke(obj, index);
}
private int getListSize(Object obj) throws Exception {
return (int) Class.forName(java.util.List.class.getName()).getMethod("size").invoke(obj);
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy