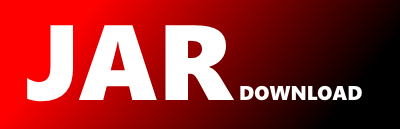
com.hellosign.openapi.model.SignatureRequestBulkSendWithTemplateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hellosign-java-sdk Show documentation
Show all versions of hellosign-java-sdk Show documentation
Use the HelloSign Java SDK to connect your Java app to HelloSign's service in microseconds!
The newest version!
/*
* HelloSign API
* HelloSign v3 API
*
* The version of the OpenAPI document: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.hellosign.openapi.model;
import java.util.Objects;
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.hellosign.openapi.model.SubBulkSignerList;
import com.hellosign.openapi.model.SubCC;
import com.hellosign.openapi.model.SubCustomField;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.hellosign.openapi.JSON;
import com.hellosign.openapi.ApiException;
/**
* SignatureRequestBulkSendWithTemplateRequest
*/
@JsonPropertyOrder({
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_TEMPLATE_IDS,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_SIGNER_FILE,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_SIGNER_LIST,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_ALLOW_DECLINE,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_CCS,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_CLIENT_ID,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_CUSTOM_FIELDS,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_MESSAGE,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_METADATA,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_SIGNING_REDIRECT_URL,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_SUBJECT,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_TEST_MODE,
SignatureRequestBulkSendWithTemplateRequest.JSON_PROPERTY_TITLE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SignatureRequestBulkSendWithTemplateRequest {
public static final String JSON_PROPERTY_TEMPLATE_IDS = "template_ids";
private List templateIds = new ArrayList<>();
public static final String JSON_PROPERTY_SIGNER_FILE = "signer_file";
private File signerFile;
public static final String JSON_PROPERTY_SIGNER_LIST = "signer_list";
private List signerList = null;
public static final String JSON_PROPERTY_ALLOW_DECLINE = "allow_decline";
private Boolean allowDecline = false;
public static final String JSON_PROPERTY_CCS = "ccs";
private List ccs = null;
public static final String JSON_PROPERTY_CLIENT_ID = "client_id";
private String clientId;
public static final String JSON_PROPERTY_CUSTOM_FIELDS = "custom_fields";
private List customFields = null;
public static final String JSON_PROPERTY_MESSAGE = "message";
private String message;
public static final String JSON_PROPERTY_METADATA = "metadata";
private Map metadata = null;
public static final String JSON_PROPERTY_SIGNING_REDIRECT_URL = "signing_redirect_url";
private String signingRedirectUrl;
public static final String JSON_PROPERTY_SUBJECT = "subject";
private String subject;
public static final String JSON_PROPERTY_TEST_MODE = "test_mode";
private Boolean testMode = false;
public static final String JSON_PROPERTY_TITLE = "title";
private String title;
public SignatureRequestBulkSendWithTemplateRequest() {
}
public SignatureRequestBulkSendWithTemplateRequest templateIds(List templateIds) {
this.templateIds = templateIds;
return this;
}
public SignatureRequestBulkSendWithTemplateRequest addTemplateIdsItem(String templateIdsItem) {
this.templateIds.add(templateIdsItem);
return this;
}
/**
* Use `template_ids` to create a SignatureRequest from one or more templates, in the order in which the template will be used.
* @return templateIds
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Use `template_ids` to create a SignatureRequest from one or more templates, in the order in which the template will be used.")
@JsonProperty(JSON_PROPERTY_TEMPLATE_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getTemplateIds() {
return templateIds;
}
@JsonProperty(JSON_PROPERTY_TEMPLATE_IDS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTemplateIds(List templateIds) {
this.templateIds = templateIds;
}
public SignatureRequestBulkSendWithTemplateRequest signerFile(File signerFile) {
this.signerFile = signerFile;
return this;
}
/**
* `signer_file` is a CSV file defining values and options for signer fields. Required unless a `signer_list` is used, you may not use both. The CSV can have the following columns: - `name`: the name of the signer filling the role of RoleName - `email_address`: email address of the signer filling the role of RoleName - `pin`: the 4- to 12-character access code that will secure this signer's signature page (optional) - `sms_phone_number`: An E.164 formatted phone number that will receive a code via SMS to access this signer's signature page. (optional) **Note**: Not available in test mode and requires a Standard plan or higher. - `*_field`: any column with a _field\" suffix will be treated as a custom field (optional) You may only specify field values here, any other options should be set in the custom_fields request parameter. Example CSV: ``` name, email_address, pin, company_field George, [email protected], d79a3td, ABC Corp Mary, [email protected], gd9as5b, 123 LLC ```
* @return signerFile
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "`signer_file` is a CSV file defining values and options for signer fields. Required unless a `signer_list` is used, you may not use both. The CSV can have the following columns: - `name`: the name of the signer filling the role of RoleName - `email_address`: email address of the signer filling the role of RoleName - `pin`: the 4- to 12-character access code that will secure this signer's signature page (optional) - `sms_phone_number`: An E.164 formatted phone number that will receive a code via SMS to access this signer's signature page. (optional) **Note**: Not available in test mode and requires a Standard plan or higher. - `*_field`: any column with a _field\" suffix will be treated as a custom field (optional) You may only specify field values here, any other options should be set in the custom_fields request parameter. Example CSV: ``` name, email_address, pin, company_field George, [email protected], d79a3td, ABC Corp Mary, [email protected], gd9as5b, 123 LLC ```")
@JsonProperty(JSON_PROPERTY_SIGNER_FILE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public File getSignerFile() {
return signerFile;
}
@JsonProperty(JSON_PROPERTY_SIGNER_FILE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSignerFile(File signerFile) {
this.signerFile = signerFile;
}
public SignatureRequestBulkSendWithTemplateRequest signerList(List signerList) {
this.signerList = signerList;
return this;
}
public SignatureRequestBulkSendWithTemplateRequest addSignerListItem(SubBulkSignerList signerListItem) {
if (this.signerList == null) {
this.signerList = new ArrayList<>();
}
this.signerList.add(signerListItem);
return this;
}
/**
* `signer_list` is an array defining values and options for signer fields. Required unless a `signer_file` is used, you may not use both.
* @return signerList
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "`signer_list` is an array defining values and options for signer fields. Required unless a `signer_file` is used, you may not use both.")
@JsonProperty(JSON_PROPERTY_SIGNER_LIST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSignerList() {
return signerList;
}
@JsonProperty(JSON_PROPERTY_SIGNER_LIST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSignerList(List signerList) {
this.signerList = signerList;
}
public SignatureRequestBulkSendWithTemplateRequest allowDecline(Boolean allowDecline) {
this.allowDecline = allowDecline;
return this;
}
/**
* Allows signers to decline to sign a document if `true`. Defaults to `false`.
* @return allowDecline
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Allows signers to decline to sign a document if `true`. Defaults to `false`.")
@JsonProperty(JSON_PROPERTY_ALLOW_DECLINE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getAllowDecline() {
return allowDecline;
}
@JsonProperty(JSON_PROPERTY_ALLOW_DECLINE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAllowDecline(Boolean allowDecline) {
this.allowDecline = allowDecline;
}
public SignatureRequestBulkSendWithTemplateRequest ccs(List ccs) {
this.ccs = ccs;
return this;
}
public SignatureRequestBulkSendWithTemplateRequest addCcsItem(SubCC ccsItem) {
if (this.ccs == null) {
this.ccs = new ArrayList<>();
}
this.ccs.add(ccsItem);
return this;
}
/**
* Add CC email recipients. Required when a CC role exists for the Template.
* @return ccs
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Add CC email recipients. Required when a CC role exists for the Template.")
@JsonProperty(JSON_PROPERTY_CCS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCcs() {
return ccs;
}
@JsonProperty(JSON_PROPERTY_CCS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCcs(List ccs) {
this.ccs = ccs;
}
public SignatureRequestBulkSendWithTemplateRequest clientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* The client id of the API App you want to associate with this request. Used to apply the branding and callback url defined for the app.
* @return clientId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The client id of the API App you want to associate with this request. Used to apply the branding and callback url defined for the app.")
@JsonProperty(JSON_PROPERTY_CLIENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getClientId() {
return clientId;
}
@JsonProperty(JSON_PROPERTY_CLIENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setClientId(String clientId) {
this.clientId = clientId;
}
public SignatureRequestBulkSendWithTemplateRequest customFields(List customFields) {
this.customFields = customFields;
return this;
}
public SignatureRequestBulkSendWithTemplateRequest addCustomFieldsItem(SubCustomField customFieldsItem) {
if (this.customFields == null) {
this.customFields = new ArrayList<>();
}
this.customFields.add(customFieldsItem);
return this;
}
/**
* When used together with merge fields, `custom_fields` allows users to add pre-filled data to their signature requests. Pre-filled data can be used with \"send-once\" signature requests by adding merge fields with `form_fields_per_document` or [Text Tags](https://app.hellosign.com/api/textTagsWalkthrough#TextTagIntro) while passing values back with `custom_fields` together in one API call. For using pre-filled on repeatable signature requests, merge fields are added to templates in the HelloSign UI or by calling [/template/create_embedded_draft](/api/reference/operation/templateCreateEmbeddedDraft) and then passing `custom_fields` on subsequent signature requests referencing that template.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "When used together with merge fields, `custom_fields` allows users to add pre-filled data to their signature requests. Pre-filled data can be used with \"send-once\" signature requests by adding merge fields with `form_fields_per_document` or [Text Tags](https://app.hellosign.com/api/textTagsWalkthrough#TextTagIntro) while passing values back with `custom_fields` together in one API call. For using pre-filled on repeatable signature requests, merge fields are added to templates in the HelloSign UI or by calling [/template/create_embedded_draft](/api/reference/operation/templateCreateEmbeddedDraft) and then passing `custom_fields` on subsequent signature requests referencing that template.")
@JsonProperty(JSON_PROPERTY_CUSTOM_FIELDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCustomFields() {
return customFields;
}
@JsonProperty(JSON_PROPERTY_CUSTOM_FIELDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCustomFields(List customFields) {
this.customFields = customFields;
}
public SignatureRequestBulkSendWithTemplateRequest message(String message) {
this.message = message;
return this;
}
/**
* The custom message in the email that will be sent to the signers.
* @return message
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom message in the email that will be sent to the signers.")
@JsonProperty(JSON_PROPERTY_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMessage() {
return message;
}
@JsonProperty(JSON_PROPERTY_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMessage(String message) {
this.message = message;
}
public SignatureRequestBulkSendWithTemplateRequest metadata(Map metadata) {
this.metadata = metadata;
return this;
}
public SignatureRequestBulkSendWithTemplateRequest putMetadataItem(String key, Object metadataItem) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, metadataItem);
return this;
}
/**
* Key-value data that should be attached to the signature request. This metadata is included in all API responses and events involving the signature request. For example, use the metadata field to store a signer's order number for look up when receiving events for the signature request. Each request can include up to 10 metadata keys (or 50 nested metadata keys), with key names up to 40 characters long and values up to 1000 characters long.
* @return metadata
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Key-value data that should be attached to the signature request. This metadata is included in all API responses and events involving the signature request. For example, use the metadata field to store a signer's order number for look up when receiving events for the signature request. Each request can include up to 10 metadata keys (or 50 nested metadata keys), with key names up to 40 characters long and values up to 1000 characters long.")
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public Map getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(content = JsonInclude.Include.ALWAYS, value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(Map metadata) {
this.metadata = metadata;
}
public SignatureRequestBulkSendWithTemplateRequest signingRedirectUrl(String signingRedirectUrl) {
this.signingRedirectUrl = signingRedirectUrl;
return this;
}
/**
* The URL you want signers redirected to after they successfully sign.
* @return signingRedirectUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The URL you want signers redirected to after they successfully sign.")
@JsonProperty(JSON_PROPERTY_SIGNING_REDIRECT_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSigningRedirectUrl() {
return signingRedirectUrl;
}
@JsonProperty(JSON_PROPERTY_SIGNING_REDIRECT_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSigningRedirectUrl(String signingRedirectUrl) {
this.signingRedirectUrl = signingRedirectUrl;
}
public SignatureRequestBulkSendWithTemplateRequest subject(String subject) {
this.subject = subject;
return this;
}
/**
* The subject in the email that will be sent to the signers.
* @return subject
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The subject in the email that will be sent to the signers.")
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getSubject() {
return subject;
}
@JsonProperty(JSON_PROPERTY_SUBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSubject(String subject) {
this.subject = subject;
}
public SignatureRequestBulkSendWithTemplateRequest testMode(Boolean testMode) {
this.testMode = testMode;
return this;
}
/**
* Whether this is a test, the signature request will not be legally binding if set to `true`. Defaults to `false`.
* @return testMode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether this is a test, the signature request will not be legally binding if set to `true`. Defaults to `false`.")
@JsonProperty(JSON_PROPERTY_TEST_MODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getTestMode() {
return testMode;
}
@JsonProperty(JSON_PROPERTY_TEST_MODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTestMode(Boolean testMode) {
this.testMode = testMode;
}
public SignatureRequestBulkSendWithTemplateRequest title(String title) {
this.title = title;
return this;
}
/**
* The title you want to assign to the SignatureRequest.
* @return title
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The title you want to assign to the SignatureRequest.")
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTitle() {
return title;
}
@JsonProperty(JSON_PROPERTY_TITLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTitle(String title) {
this.title = title;
}
/**
* Return true if this SignatureRequestBulkSendWithTemplateRequest object is equal to o.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SignatureRequestBulkSendWithTemplateRequest signatureRequestBulkSendWithTemplateRequest = (SignatureRequestBulkSendWithTemplateRequest) o;
return Objects.equals(this.templateIds, signatureRequestBulkSendWithTemplateRequest.templateIds) &&
Objects.equals(this.signerFile, signatureRequestBulkSendWithTemplateRequest.signerFile) &&
Objects.equals(this.signerList, signatureRequestBulkSendWithTemplateRequest.signerList) &&
Objects.equals(this.allowDecline, signatureRequestBulkSendWithTemplateRequest.allowDecline) &&
Objects.equals(this.ccs, signatureRequestBulkSendWithTemplateRequest.ccs) &&
Objects.equals(this.clientId, signatureRequestBulkSendWithTemplateRequest.clientId) &&
Objects.equals(this.customFields, signatureRequestBulkSendWithTemplateRequest.customFields) &&
Objects.equals(this.message, signatureRequestBulkSendWithTemplateRequest.message) &&
Objects.equals(this.metadata, signatureRequestBulkSendWithTemplateRequest.metadata) &&
Objects.equals(this.signingRedirectUrl, signatureRequestBulkSendWithTemplateRequest.signingRedirectUrl) &&
Objects.equals(this.subject, signatureRequestBulkSendWithTemplateRequest.subject) &&
Objects.equals(this.testMode, signatureRequestBulkSendWithTemplateRequest.testMode) &&
Objects.equals(this.title, signatureRequestBulkSendWithTemplateRequest.title);
}
@Override
public int hashCode() {
return Objects.hash(templateIds, signerFile, signerList, allowDecline, ccs, clientId, customFields, message, metadata, signingRedirectUrl, subject, testMode, title);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SignatureRequestBulkSendWithTemplateRequest {\n");
sb.append(" templateIds: ").append(toIndentedString(templateIds)).append("\n");
sb.append(" signerFile: ").append(toIndentedString(signerFile)).append("\n");
sb.append(" signerList: ").append(toIndentedString(signerList)).append("\n");
sb.append(" allowDecline: ").append(toIndentedString(allowDecline)).append("\n");
sb.append(" ccs: ").append(toIndentedString(ccs)).append("\n");
sb.append(" clientId: ").append(toIndentedString(clientId)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" signingRedirectUrl: ").append(toIndentedString(signingRedirectUrl)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" testMode: ").append(toIndentedString(testMode)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append("}");
return sb.toString();
}
public Map createFormData() throws ApiException {
Map map = new HashMap<>();
boolean fileTypeFound = false;
try {
if (templateIds != null) {
if (isFileTypeOrListOfFiles(templateIds)) {
fileTypeFound = true;
}
if (templateIds.getClass().equals(java.io.File.class) ||
templateIds.getClass().equals(Integer.class) ||
templateIds.getClass().equals(String.class) ) {
map.put("template_ids", templateIds);
} else if (isListOfFile(templateIds)) {
for(int i = 0; i< getListSize(templateIds); i++) {
map.put("template_ids[" + i + "]", getFromList(templateIds, i));
}
}
else {
map.put("template_ids", JSON.getDefault().getMapper().writeValueAsString(templateIds));
}
}
if (signerFile != null) {
if (isFileTypeOrListOfFiles(signerFile)) {
fileTypeFound = true;
}
if (signerFile.getClass().equals(java.io.File.class) ||
signerFile.getClass().equals(Integer.class) ||
signerFile.getClass().equals(String.class) ) {
map.put("signer_file", signerFile);
} else if (isListOfFile(signerFile)) {
for(int i = 0; i< getListSize(signerFile); i++) {
map.put("signer_file[" + i + "]", getFromList(signerFile, i));
}
}
else {
map.put("signer_file", JSON.getDefault().getMapper().writeValueAsString(signerFile));
}
}
if (signerList != null) {
if (isFileTypeOrListOfFiles(signerList)) {
fileTypeFound = true;
}
if (signerList.getClass().equals(java.io.File.class) ||
signerList.getClass().equals(Integer.class) ||
signerList.getClass().equals(String.class) ) {
map.put("signer_list", signerList);
} else if (isListOfFile(signerList)) {
for(int i = 0; i< getListSize(signerList); i++) {
map.put("signer_list[" + i + "]", getFromList(signerList, i));
}
}
else {
map.put("signer_list", JSON.getDefault().getMapper().writeValueAsString(signerList));
}
}
if (allowDecline != null) {
if (isFileTypeOrListOfFiles(allowDecline)) {
fileTypeFound = true;
}
if (allowDecline.getClass().equals(java.io.File.class) ||
allowDecline.getClass().equals(Integer.class) ||
allowDecline.getClass().equals(String.class) ) {
map.put("allow_decline", allowDecline);
} else if (isListOfFile(allowDecline)) {
for(int i = 0; i< getListSize(allowDecline); i++) {
map.put("allow_decline[" + i + "]", getFromList(allowDecline, i));
}
}
else {
map.put("allow_decline", JSON.getDefault().getMapper().writeValueAsString(allowDecline));
}
}
if (ccs != null) {
if (isFileTypeOrListOfFiles(ccs)) {
fileTypeFound = true;
}
if (ccs.getClass().equals(java.io.File.class) ||
ccs.getClass().equals(Integer.class) ||
ccs.getClass().equals(String.class) ) {
map.put("ccs", ccs);
} else if (isListOfFile(ccs)) {
for(int i = 0; i< getListSize(ccs); i++) {
map.put("ccs[" + i + "]", getFromList(ccs, i));
}
}
else {
map.put("ccs", JSON.getDefault().getMapper().writeValueAsString(ccs));
}
}
if (clientId != null) {
if (isFileTypeOrListOfFiles(clientId)) {
fileTypeFound = true;
}
if (clientId.getClass().equals(java.io.File.class) ||
clientId.getClass().equals(Integer.class) ||
clientId.getClass().equals(String.class) ) {
map.put("client_id", clientId);
} else if (isListOfFile(clientId)) {
for(int i = 0; i< getListSize(clientId); i++) {
map.put("client_id[" + i + "]", getFromList(clientId, i));
}
}
else {
map.put("client_id", JSON.getDefault().getMapper().writeValueAsString(clientId));
}
}
if (customFields != null) {
if (isFileTypeOrListOfFiles(customFields)) {
fileTypeFound = true;
}
if (customFields.getClass().equals(java.io.File.class) ||
customFields.getClass().equals(Integer.class) ||
customFields.getClass().equals(String.class) ) {
map.put("custom_fields", customFields);
} else if (isListOfFile(customFields)) {
for(int i = 0; i< getListSize(customFields); i++) {
map.put("custom_fields[" + i + "]", getFromList(customFields, i));
}
}
else {
map.put("custom_fields", JSON.getDefault().getMapper().writeValueAsString(customFields));
}
}
if (message != null) {
if (isFileTypeOrListOfFiles(message)) {
fileTypeFound = true;
}
if (message.getClass().equals(java.io.File.class) ||
message.getClass().equals(Integer.class) ||
message.getClass().equals(String.class) ) {
map.put("message", message);
} else if (isListOfFile(message)) {
for(int i = 0; i< getListSize(message); i++) {
map.put("message[" + i + "]", getFromList(message, i));
}
}
else {
map.put("message", JSON.getDefault().getMapper().writeValueAsString(message));
}
}
if (metadata != null) {
if (isFileTypeOrListOfFiles(metadata)) {
fileTypeFound = true;
}
if (metadata.getClass().equals(java.io.File.class) ||
metadata.getClass().equals(Integer.class) ||
metadata.getClass().equals(String.class) ) {
map.put("metadata", metadata);
} else if (isListOfFile(metadata)) {
for(int i = 0; i< getListSize(metadata); i++) {
map.put("metadata[" + i + "]", getFromList(metadata, i));
}
}
else {
map.put("metadata", JSON.getDefault().getMapper().writeValueAsString(metadata));
}
}
if (signingRedirectUrl != null) {
if (isFileTypeOrListOfFiles(signingRedirectUrl)) {
fileTypeFound = true;
}
if (signingRedirectUrl.getClass().equals(java.io.File.class) ||
signingRedirectUrl.getClass().equals(Integer.class) ||
signingRedirectUrl.getClass().equals(String.class) ) {
map.put("signing_redirect_url", signingRedirectUrl);
} else if (isListOfFile(signingRedirectUrl)) {
for(int i = 0; i< getListSize(signingRedirectUrl); i++) {
map.put("signing_redirect_url[" + i + "]", getFromList(signingRedirectUrl, i));
}
}
else {
map.put("signing_redirect_url", JSON.getDefault().getMapper().writeValueAsString(signingRedirectUrl));
}
}
if (subject != null) {
if (isFileTypeOrListOfFiles(subject)) {
fileTypeFound = true;
}
if (subject.getClass().equals(java.io.File.class) ||
subject.getClass().equals(Integer.class) ||
subject.getClass().equals(String.class) ) {
map.put("subject", subject);
} else if (isListOfFile(subject)) {
for(int i = 0; i< getListSize(subject); i++) {
map.put("subject[" + i + "]", getFromList(subject, i));
}
}
else {
map.put("subject", JSON.getDefault().getMapper().writeValueAsString(subject));
}
}
if (testMode != null) {
if (isFileTypeOrListOfFiles(testMode)) {
fileTypeFound = true;
}
if (testMode.getClass().equals(java.io.File.class) ||
testMode.getClass().equals(Integer.class) ||
testMode.getClass().equals(String.class) ) {
map.put("test_mode", testMode);
} else if (isListOfFile(testMode)) {
for(int i = 0; i< getListSize(testMode); i++) {
map.put("test_mode[" + i + "]", getFromList(testMode, i));
}
}
else {
map.put("test_mode", JSON.getDefault().getMapper().writeValueAsString(testMode));
}
}
if (title != null) {
if (isFileTypeOrListOfFiles(title)) {
fileTypeFound = true;
}
if (title.getClass().equals(java.io.File.class) ||
title.getClass().equals(Integer.class) ||
title.getClass().equals(String.class) ) {
map.put("title", title);
} else if (isListOfFile(title)) {
for(int i = 0; i< getListSize(title); i++) {
map.put("title[" + i + "]", getFromList(title, i));
}
}
else {
map.put("title", JSON.getDefault().getMapper().writeValueAsString(title));
}
}
} catch (Exception e) {
throw new ApiException(e);
}
return fileTypeFound ? map : new HashMap<>();
}
private boolean isFileTypeOrListOfFiles(Object obj) throws Exception {
return obj.getClass().equals(java.io.File.class) || isListOfFile(obj);
}
private boolean isListOfFile(Object obj) throws Exception {
return obj instanceof java.util.List && !isListEmpty(obj) && getFromList(obj, 0) instanceof java.io.File;
}
private boolean isListEmpty(Object obj) throws Exception {
return (boolean) Class.forName(java.util.List.class.getName()).getMethod("isEmpty").invoke(obj);
}
private Object getFromList(Object obj, int index) throws Exception {
return Class.forName(java.util.List.class.getName()).getMethod("get", int.class).invoke(obj, index);
}
private int getListSize(Object obj) throws Exception {
return (int) Class.forName(java.util.List.class.getName()).getMethod("size").invoke(obj);
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy