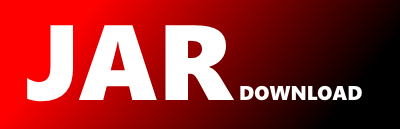
org.semanticweb.HermiT.hierarchy.Hierarchy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.semanticweb.hermit Show documentation
Show all versions of org.semanticweb.hermit Show documentation
HermiT is reasoner for ontologies written using the Web
Ontology Language (OWL). Given an OWL file, HermiT can determine whether or
not the ontology is consistent, identify subsumption relationships between
classes, and much more.
This is the maven build of HermiT and is designed for people who wish to use
HermiT from within the OWL API. It is now versioned in the main HermiT
version repository, although not officially supported by the HermiT
developers.
The version number of this package is a composite of the HermiT version and
an value representing releases of this packaged version. So, 1.3.7.1 is the
first release of the mavenized version of HermiT based on the 1.3.7 release
of HermiT.
This package includes the Jautomata library
(http://jautomata.sourceforge.net/), and builds with it directly. This
library appears to be no longer under active development, and so a "fork"
seems appropriate. No development is intended or anticipated on this code
base.
/* Copyright 2009 by the Oxford University Computing Laboratory
This file is part of HermiT.
HermiT is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
HermiT is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with HermiT. If not, see .
*/
package org.semanticweb.HermiT.hierarchy;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public class Hierarchy {
protected final HierarchyNode m_topNode;
protected final HierarchyNode m_bottomNode;
protected final Map> m_nodesByElements;
public Hierarchy(HierarchyNode topNode,HierarchyNode bottomNode) {
m_topNode=topNode;
m_bottomNode=bottomNode;
m_nodesByElements=new HashMap>();
for (E element : m_topNode.m_equivalentElements)
m_nodesByElements.put(element,m_topNode);
for (E element : m_bottomNode.m_equivalentElements)
m_nodesByElements.put(element,m_bottomNode);
}
public HierarchyNode getTopNode() {
return m_topNode;
}
public HierarchyNode getBottomNode() {
return m_bottomNode;
}
public boolean isEmpty() {
return m_nodesByElements.size()==2 && m_topNode.m_equivalentElements.size()==1 && m_bottomNode.m_equivalentElements.size()==1;
}
public HierarchyNode getNodeForElement(E element) {
return m_nodesByElements.get(element);
}
public Collection> getAllNodes() {
return Collections.unmodifiableCollection(m_nodesByElements.values());
}
public Set> getAllNodesSet() {
return Collections.unmodifiableSet(new HashSet>(m_nodesByElements.values()));
}
public Set getAllElements() {
return Collections.unmodifiableSet(m_nodesByElements.keySet());
}
public int getDepth() {
HierarchyDepthFinder depthFinder=new HierarchyDepthFinder(m_bottomNode);
traverseDepthFirst(depthFinder);
return depthFinder.depth;
}
protected final class HierarchyDepthFinder implements Hierarchy.HierarchyNodeVisitor {
protected final HierarchyNode m_bottomNode;
protected int depth=0;
public HierarchyDepthFinder(HierarchyNode bottomNode) {
m_bottomNode=bottomNode;
}
public boolean redirect(HierarchyNode[] nodes) {
return true;
}
public void visit(int level,HierarchyNode node,HierarchyNode parentNode,boolean firstVisit) {
if (node.equals(m_bottomNode)&&level>depth)
depth=level;
}
}
public Hierarchy transform(Transformer super E,T> transformer,Comparator comparator) {
HierarchyNodeComparator newNodeComparator=new HierarchyNodeComparator(comparator);
Map,HierarchyNode> oldToNew=new HashMap,HierarchyNode>();
for (HierarchyNode oldNode : m_nodesByElements.values()) {
Set newEquivalentElements;
Set> newParentNodes;
Set> newChildNodes;
if (comparator==null) {
newEquivalentElements=new HashSet();
newParentNodes=new HashSet>();
newChildNodes=new HashSet>();
}
else {
newEquivalentElements=new TreeSet(comparator);
newParentNodes=new TreeSet>(newNodeComparator);
newChildNodes=new TreeSet>(newNodeComparator);
}
for (E oldElement : oldNode.m_equivalentElements) {
T newElement=transformer.transform(oldElement);
newEquivalentElements.add(newElement);
}
T newRepresentative=transformer.determineRepresentative(oldNode.m_representative,newEquivalentElements);
HierarchyNode newNode=new HierarchyNode(newRepresentative,newEquivalentElements,newParentNodes,newChildNodes);
oldToNew.put(oldNode,newNode);
}
for (HierarchyNode oldParentNode : m_nodesByElements.values()) {
HierarchyNode newParentNode=oldToNew.get(oldParentNode);
for (HierarchyNode oldChildNode : oldParentNode.m_childNodes) {
HierarchyNode newChildNode=oldToNew.get(oldChildNode);
newParentNode.m_childNodes.add(newChildNode);
newChildNode.m_parentNodes.add(newParentNode);
}
}
HierarchyNode newTopNode=oldToNew.get(m_topNode);
HierarchyNode newBottomNode=oldToNew.get(m_bottomNode);
Hierarchy newHierarchy=new Hierarchy(newTopNode,newBottomNode);
for (HierarchyNode newNode : oldToNew.values())
for (T newElement : newNode.m_equivalentElements)
newHierarchy.m_nodesByElements.put(newElement,newNode);
return newHierarchy;
}
@SuppressWarnings("unchecked")
public void traverseDepthFirst(HierarchyNodeVisitor visitor) {
HierarchyNode[] redirectBuffer=new HierarchyNode[2];
Set> visited=new HashSet>();
traverseDepthFirst(visitor,0,m_topNode,null,visited,redirectBuffer);
}
protected void traverseDepthFirst(HierarchyNodeVisitor visitor,int level,HierarchyNode node,HierarchyNode parentNode,Set> visited,HierarchyNode[] redirectBuffer) {
redirectBuffer[0]=node;
redirectBuffer[1]=parentNode;
if (visitor.redirect(redirectBuffer)) {
node=redirectBuffer[0];
parentNode=redirectBuffer[1];
boolean firstVisit=visited.add(node);
visitor.visit(level,node,parentNode,firstVisit);
if (firstVisit)
for (HierarchyNode childNode : node.m_childNodes)
traverseDepthFirst(visitor,level+1,childNode,node,visited,redirectBuffer);
}
}
public String toString() {
StringWriter buffer=new StringWriter();
final PrintWriter output=new PrintWriter(buffer);
traverseDepthFirst(new HierarchyNodeVisitor() {
public boolean redirect(HierarchyNode[] nodes) {
return true;
}
public void visit(int level,HierarchyNode node,HierarchyNode parentNode,boolean firstVisit) {
if (!node.equals(m_bottomNode))
printNode(level,node,parentNode,firstVisit);
}
public void printNode(int level,HierarchyNode node,HierarchyNode parentNode,boolean firstVisit) {
Set equivalences=node.getEquivalentElements();
boolean printSubClasOf=(parentNode!=null);
boolean printEquivalences=firstVisit && equivalences.size()>1;
if (printSubClasOf || printEquivalences) {
for (int i=4*level;i>0;--i)
output.print(' ');
output.print(node.getRepresentative().toString());
if (printEquivalences) {
output.print('[');
boolean first=true;
for (E element : equivalences) {
if (!node.getRepresentative().equals(element)) {
if (first)
first=false;
else
output.print(' ');
output.print(element);
}
}
output.print(']');
}
if (printSubClasOf) {
output.print(" -> ");
output.print(parentNode.getRepresentative().toString());
}
output.println();
}
}
});
output.flush();
return buffer.toString();
}
public static Hierarchy emptyHierarchy(Collection elements,T topElement,T bottomElement) {
HierarchyNode topBottomNode=new HierarchyNode(topElement);
topBottomNode.m_equivalentElements.add(topElement);
topBottomNode.m_equivalentElements.add(bottomElement);
topBottomNode.m_equivalentElements.addAll(elements);
return new Hierarchy(topBottomNode,topBottomNode);
}
public static Hierarchy trivialHierarchy(T topElement,T bottomElement) {
HierarchyNode topNode=new HierarchyNode(topElement);
topNode.m_equivalentElements.add(topElement);
HierarchyNode bottomNode=new HierarchyNode(bottomElement);
bottomNode.m_equivalentElements.add(bottomElement);
topNode.m_childNodes.add(bottomNode);
bottomNode.m_parentNodes.add(topNode);
return new Hierarchy(topNode,bottomNode);
}
protected static interface HierarchyNodeVisitor {
boolean redirect(HierarchyNode[] nodes);
void visit(int level,HierarchyNode node,HierarchyNode parentNode,boolean firstVisit);
}
public static interface Transformer {
T transform(E element);
T determineRepresentative(E oldRepresentative,Set newEquivalentElements);
}
protected static class HierarchyNodeComparator implements Comparator> {
protected final Comparator m_elementComparator;
public HierarchyNodeComparator(Comparator elementComparator) {
m_elementComparator=elementComparator;
}
public int compare(HierarchyNode n1,HierarchyNode n2) {
return m_elementComparator.compare(n1.m_representative,n2.m_representative);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy