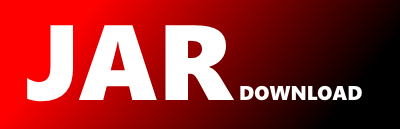
org.semanticweb.HermiT.model.DLOntology Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.semanticweb.hermit Show documentation
Show all versions of org.semanticweb.hermit Show documentation
HermiT is reasoner for ontologies written using the Web
Ontology Language (OWL). Given an OWL file, HermiT can determine whether or
not the ontology is consistent, identify subsumption relationships between
classes, and much more.
This is the maven build of HermiT and is designed for people who wish to use
HermiT from within the OWL API. It is now versioned in the main HermiT
version repository, although not officially supported by the HermiT
developers.
The version number of this package is a composite of the HermiT version and
an value representing releases of this packaged version. So, 1.3.7.1 is the
first release of the mavenized version of HermiT based on the 1.3.7 release
of HermiT.
This package includes the Jautomata library
(http://jautomata.sourceforge.net/), and builds with it directly. This
library appears to be no longer under active development, and so a "fork"
seems appropriate. No development is intended or anticipated on this code
base.
/* Copyright 2008, 2009, 2010 by the Oxford University Computing Laboratory
This file is part of HermiT.
HermiT is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
HermiT is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with HermiT. If not, see .
*/
package org.semanticweb.HermiT.model;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
import java.io.Serializable;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import org.semanticweb.HermiT.Prefixes;
/**
* Represents a DL ontology as a set of rules.
*/
public class DLOntology implements Serializable {
private static final long serialVersionUID=3189937959595369812L;
protected static final String CRLF=System.getProperty("line.separator");
protected final String m_ontologyIRI;
protected final Set m_dlClauses;
protected final Set m_positiveFacts;
protected final Set m_negativeFacts;
protected final boolean m_hasInverseRoles;
protected final boolean m_hasAtMostRestrictions;
protected final boolean m_hasNominals;
protected final boolean m_hasDatatypes;
protected final boolean m_isHorn;
protected final Set m_allAtomicConcepts;
protected final int m_numberOfExternalConcepts;
protected final Set m_allAtomicObjectRoles;
protected final Set m_allComplexObjectRoles;
protected final Set m_allAtomicDataRoles;
protected final Set m_allUnknownDatatypeRestrictions;
protected final Set m_definedDatatypeIRIs;
protected final Set m_allIndividuals;
protected final Set m_allDescriptionGraphs;
protected final Map>> m_dataPropertyAssertions;
public DLOntology(String ontologyIRI,Set dlClauses,Set positiveFacts,Set negativeFacts, Set atomicConcepts,
Set atomicObjectRoles,Set allComplexObjectRoles,Set atomicDataRoles,
Set allUnknownDatatypeRestrictions,Set definedDatatypeIRIs,Set individuals,
boolean hasInverseRoles,boolean hasAtMostRestrictions,boolean hasNominals,boolean hasDatatypes) {
m_ontologyIRI=ontologyIRI;
m_dlClauses=dlClauses;
m_positiveFacts=positiveFacts;
m_negativeFacts=negativeFacts;
m_hasInverseRoles=hasInverseRoles;
m_hasAtMostRestrictions=hasAtMostRestrictions;
m_hasNominals=hasNominals;
m_hasDatatypes=hasDatatypes;
if (atomicConcepts==null)
m_allAtomicConcepts=new TreeSet(AtomicConceptComparator.INSTANCE);
else
m_allAtomicConcepts=atomicConcepts;
int numberOfExternalConcepts=0;
for (AtomicConcept c : m_allAtomicConcepts)
if (!Prefixes.isInternalIRI(c.getIRI()))
numberOfExternalConcepts++;
m_numberOfExternalConcepts=numberOfExternalConcepts;
if (atomicObjectRoles==null)
m_allAtomicObjectRoles=new TreeSet(AtomicRoleComparator.INSTANCE);
else
m_allAtomicObjectRoles=atomicObjectRoles;
if (allComplexObjectRoles==null)
m_allComplexObjectRoles=new HashSet();
else
m_allComplexObjectRoles=allComplexObjectRoles;
if (atomicDataRoles==null)
m_allAtomicDataRoles=new TreeSet(AtomicRoleComparator.INSTANCE);
else
m_allAtomicDataRoles=atomicDataRoles;
if (allUnknownDatatypeRestrictions==null)
m_allUnknownDatatypeRestrictions=new HashSet();
else
m_allUnknownDatatypeRestrictions=allUnknownDatatypeRestrictions;
if (definedDatatypeIRIs==null)
m_definedDatatypeIRIs=new HashSet();
else
m_definedDatatypeIRIs=definedDatatypeIRIs;
if (individuals==null)
m_allIndividuals=new TreeSet(IndividualComparator.INSTANCE);
else
m_allIndividuals=individuals;
m_allDescriptionGraphs=new HashSet();
boolean isHorn=true;
for (DLClause dlClause : m_dlClauses) {
if (dlClause.getHeadLength()>1)
isHorn=false;
for (int bodyIndex=dlClause.getBodyLength()-1;bodyIndex>=0;--bodyIndex) {
DLPredicate dlPredicate=dlClause.getBodyAtom(bodyIndex).getDLPredicate();
addDLPredicate(dlPredicate);
}
for (int headIndex=dlClause.getHeadLength()-1;headIndex>=0;--headIndex) {
DLPredicate dlPredicate=dlClause.getHeadAtom(headIndex).getDLPredicate();
addDLPredicate(dlPredicate);
}
}
m_isHorn=isHorn;
m_dataPropertyAssertions=new HashMap>>();
for (Atom atom : m_positiveFacts) {
addDLPredicate(atom.getDLPredicate());
for (int i=0;i> individualsToConstants;
if (m_dataPropertyAssertions.containsKey(atomicRole))
individualsToConstants=m_dataPropertyAssertions.get(atomicRole);
else {
individualsToConstants=new HashMap>();
m_dataPropertyAssertions.put(atomicRole,individualsToConstants);
}
Set constants;
if (individualsToConstants.containsKey(sourceIndividual))
constants=individualsToConstants.get(sourceIndividual);
else {
constants=new HashSet();
individualsToConstants.put(sourceIndividual,constants);
}
constants.add((Constant)possibleConstant);
}
}
}
for (Atom atom : m_negativeFacts) {
addDLPredicate(atom.getDLPredicate());
for (int i=0;i getAllAtomicConcepts() {
return m_allAtomicConcepts;
}
public boolean containsAtomicConcept(AtomicConcept concept) {
return m_allAtomicConcepts.contains(concept);
}
public int getNumberOfExternalConcepts() {
return m_numberOfExternalConcepts;
}
public Set getAllAtomicObjectRoles() {
return m_allAtomicObjectRoles;
}
public boolean containsObjectRole(AtomicRole role) {
return m_allAtomicObjectRoles.contains(role);
}
public Set getAllComplexObjectRoles() {
return m_allComplexObjectRoles;
}
public boolean isComplexObjectRole(Role role) {
return m_allComplexObjectRoles.contains(role);
}
public Set getAllAtomicDataRoles() {
return m_allAtomicDataRoles;
}
public boolean containsDataRole(AtomicRole role) {
return m_allAtomicDataRoles.contains(role);
}
public Set getAllUnknownDatatypeRestrictions() {
return m_allUnknownDatatypeRestrictions;
}
public Set getAllIndividuals() {
return m_allIndividuals;
}
public boolean containsIndividual(Individual individual) {
return m_allIndividuals.contains(individual);
}
public Set getAllDescriptionGraphs() {
return m_allDescriptionGraphs;
}
public Set getDLClauses() {
return m_dlClauses;
}
public Set getPositiveFacts() {
return m_positiveFacts;
}
public Map>> getDataPropertyAssertions() {
return m_dataPropertyAssertions;
}
public Set getNegativeFacts() {
return m_negativeFacts;
}
public boolean hasInverseRoles() {
return m_hasInverseRoles;
}
public boolean hasAtMostRestrictions() {
return m_hasAtMostRestrictions;
}
public boolean hasNominals() {
return m_hasNominals;
}
public boolean hasDatatypes() {
return m_hasDatatypes;
}
public boolean hasUnknownDatatypeRestrictions() {
return !m_allUnknownDatatypeRestrictions.isEmpty();
}
public boolean isHorn() {
return m_isHorn;
}
public Set getDefinedDatatypeIRIs() {
return m_definedDatatypeIRIs;
}
protected Set getBodyOnlyAtomicConcepts() {
Set bodyOnlyAtomicConcepts=new HashSet(m_allAtomicConcepts);
for (DLClause dlClause : m_dlClauses)
for (int headIndex=0;headIndex computeGraphAtomicRoles() {
Set graphAtomicRoles=new HashSet();
for (DescriptionGraph descriptionGraph : m_allDescriptionGraphs)
for (int edgeIndex=0;edgeIndex roles) {
for (int atomIndex=0;atomIndex roles) {
boolean change=false;
for (int atomIndex=0;atomIndex entry : prefixes.getPrefixIRIsByPrefixName().entrySet()) {
stringBuffer.append(" ");
stringBuffer.append(entry.getKey());
stringBuffer.append(" = <");
stringBuffer.append(entry.getValue());
stringBuffer.append('>');
stringBuffer.append(CRLF);
}
stringBuffer.append("]");
stringBuffer.append(CRLF);
stringBuffer.append("Deterministic DL-clauses: [");
stringBuffer.append(CRLF);
int numDeterministicClauses=0;
for (DLClause dlClause : m_dlClauses)
if (dlClause.getHeadLength()<=1) {
numDeterministicClauses++;
stringBuffer.append(" ");
stringBuffer.append(dlClause.toString(prefixes));
stringBuffer.append(CRLF);
}
stringBuffer.append("]");
stringBuffer.append(CRLF);
stringBuffer.append("Disjunctive DL-clauses: [");
stringBuffer.append(CRLF);
int numNondeterministicClauses=0;
int numDisjunctions=0;
for (DLClause dlClause : m_dlClauses)
if (dlClause.getHeadLength()>1) {
numNondeterministicClauses++;
numDisjunctions+=dlClause.getHeadLength();
stringBuffer.append(" ");
stringBuffer.append(dlClause.toString(prefixes));
stringBuffer.append(CRLF);
}
stringBuffer.append("]");
stringBuffer.append(CRLF);
stringBuffer.append("ABox: [");
stringBuffer.append(CRLF);
for (Atom atom : m_positiveFacts) {
stringBuffer.append(" ");
stringBuffer.append(atom.toString(prefixes));
stringBuffer.append(CRLF);
}
for (Atom atom : m_negativeFacts) {
stringBuffer.append(" !");
stringBuffer.append(atom.toString(prefixes));
stringBuffer.append(CRLF);
}
stringBuffer.append("]");
stringBuffer.append(CRLF);
stringBuffer.append("Statistics: [");
stringBuffer.append(CRLF);
stringBuffer.append(" Number of deterministic clauses: " + numDeterministicClauses);
stringBuffer.append(CRLF);
stringBuffer.append(" Number of nondeterministic clauses: " + numNondeterministicClauses);
stringBuffer.append(CRLF);
stringBuffer.append(" Number of disjunctions: " + numDisjunctions);
stringBuffer.append(CRLF);
stringBuffer.append(" Number of positive facts: " + m_positiveFacts.size());
stringBuffer.append(CRLF);
stringBuffer.append(" Number of negative facts: " + m_negativeFacts.size());
stringBuffer.append(CRLF);
stringBuffer.append("]");
return stringBuffer.toString();
}
public String getStatistics() {
return getStatistics(null,null,null);
}
protected String getStatistics(Integer numDeterministicClauses, Integer numNondeterministicClauses, Integer numDisjunctions) {
if (numDeterministicClauses==null || numNondeterministicClauses==null || numDisjunctions==null) {
numDeterministicClauses=0;
numNondeterministicClauses=0;
numDisjunctions=0;
for (DLClause dlClause : m_dlClauses) {
if (dlClause.getHeadLength()<=1)
numDeterministicClauses++;
else {
numNondeterministicClauses++;
numDisjunctions+=dlClause.getHeadLength();
}
}
}
StringBuffer stringBuffer=new StringBuffer();
stringBuffer.append("DL clauses statistics: [");
stringBuffer.append(CRLF);
stringBuffer.append(" Number of deterministic clauses: " + numDeterministicClauses);
stringBuffer.append(CRLF);
stringBuffer.append(" Number of nondeterministic clauses: " + numNondeterministicClauses);
stringBuffer.append(CRLF);
stringBuffer.append(" Overall number of disjunctions: " + numDisjunctions);
stringBuffer.append(CRLF);
stringBuffer.append(" Number of positive facts: " + m_positiveFacts.size());
stringBuffer.append(CRLF);
stringBuffer.append(" Number of negative facts: " + m_negativeFacts.size());
stringBuffer.append(CRLF);
stringBuffer.append(" Inverses: " + this.hasInverseRoles());
stringBuffer.append(CRLF);
stringBuffer.append(" At-Mosts: " + this.hasAtMostRestrictions());
stringBuffer.append(CRLF);
stringBuffer.append(" Datatypes: " + this.hasDatatypes());
stringBuffer.append(CRLF);
stringBuffer.append(" Nominals: " + this.hasNominals());
stringBuffer.append(CRLF);
stringBuffer.append(" Number of atomic concepts: " + m_allAtomicConcepts.size());
stringBuffer.append(CRLF);
stringBuffer.append(" Number of object properties: " + m_allAtomicObjectRoles.size());
stringBuffer.append(CRLF);
stringBuffer.append(" Number of data properties: " + m_allAtomicDataRoles.size());
stringBuffer.append(CRLF);
stringBuffer.append(" Number of individuals: " + m_allIndividuals.size());
stringBuffer.append(CRLF);
stringBuffer.append("]");
return stringBuffer.toString();
}
public String toString() {
return toString(Prefixes.STANDARD_PREFIXES);
}
public void save(File file) throws IOException {
OutputStream outputStream=new BufferedOutputStream(new FileOutputStream(file));
try {
save(outputStream);
}
finally {
outputStream.close();
}
}
public void save(OutputStream outputStream) throws IOException {
ObjectOutputStream objectOutputStream=new ObjectOutputStream(outputStream);
objectOutputStream.writeObject(this);
objectOutputStream.flush();
}
public static DLOntology load(InputStream inputStream) throws IOException {
try {
ObjectInputStream objectInputStream=new ObjectInputStream(inputStream);
return (DLOntology)objectInputStream.readObject();
}
catch (ClassNotFoundException e) {
IOException error=new IOException();
error.initCause(e);
throw error;
}
}
public static DLOntology load(File file) throws IOException {
InputStream inputStream=new BufferedInputStream(new FileInputStream(file));
try {
return load(inputStream);
}
finally {
inputStream.close();
}
}
public static class AtomicConceptComparator implements Serializable,Comparator {
private static final long serialVersionUID=2386841732225838685L;
public static final Comparator INSTANCE=new AtomicConceptComparator();
public int compare(AtomicConcept o1,AtomicConcept o2) {
return o1.getIRI().compareTo(o2.getIRI());
}
protected Object readResolve() {
return INSTANCE;
}
}
public static class AtomicRoleComparator implements Serializable,Comparator {
private static final long serialVersionUID=3483541702854959793L;
public static final Comparator INSTANCE=new AtomicRoleComparator();
public int compare(AtomicRole o1,AtomicRole o2) {
return o1.getIRI().compareTo(o2.getIRI());
}
protected Object readResolve() {
return INSTANCE;
}
}
public static class IndividualComparator implements Serializable,Comparator {
private static final long serialVersionUID=2386841732225838685L;
public static final Comparator INSTANCE=new IndividualComparator();
public int compare(Individual o1,Individual o2) {
return o1.getIRI().compareTo(o2.getIRI());
}
protected Object readResolve() {
return INSTANCE;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy