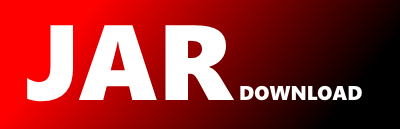
com.hi3project.broccoli.bsdl.impl.Ontology Maven / Gradle / Ivy
Show all versions of broccoli-api Show documentation
/*******************************************************************************
*
* Copyright (C) 2015 Mytech Ingenieria Aplicada
* Copyright (C) 2015 Alejandro Paz
*
* This file is part of Broccoli.
*
* Broccoli is free software: you can redistribute it and/or modify it under the
* terms of the GNU Affero General Public License as published by the Free
* Software Foundation, either version 3 of the License, or (at your option) any
* later version.
*
* Broccoli is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU Affero General Public License for more
* details.
*
* You should have received a copy of the GNU Affero General Public License
* along with Broccoli. If not, see .
*
******************************************************************************/
package com.hi3project.broccoli.bsdl.impl;
import com.hi3project.broccoli.bsdl.api.IAxiom;
import com.hi3project.broccoli.bsdl.api.IPredicate;
import com.hi3project.broccoli.bsdl.api.meta.INaturalLanguage;
import com.hi3project.broccoli.bsdl.api.IOntology;
import com.hi3project.broccoli.bsdl.api.ISemanticIdentifier;
import com.hi3project.broccoli.bsdl.api.meta.IOntologyLanguage;
import com.hi3project.broccoli.bsdl.api.meta.IVersionNumber;
import com.hi3project.broccoli.bsdl.api.parsing.IImport;
import com.hi3project.broccoli.bsdl.impl.exceptions.SemanticModelException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
/**
*
* Implementation of IOntology that contains: Concepts, Instances and Imports
*
*
*
*/
public class Ontology extends SemanticAxiom implements IOntology
{
private Collection imports = new ArrayList();
private List concepts = new ArrayList();
private List instances = new ArrayList();
public Ontology() throws SemanticModelException
{
super();
}
public Ontology(ISemanticIdentifier semanticIdentifier) throws SemanticModelException
{
super();
this.setSemanticIdentifier(semanticIdentifier);
}
@Override
public ISemanticIdentifier identifier()
{
return this.getSemanticIdentifier();
}
public Collection getConcepts(SemanticIdentifier sa)
{
Collection conceptsCol = new ArrayList();
for (Concept concept : concepts)
{
if (concept.getSemanticIdentifier().equals(sa))
{
conceptsCol.add(concept);
}
// TODO: if concept subsumes sa...
}
return conceptsCol;
}
public Collection concepts()
{
return concepts;
}
public Collection getDistinctConcepts()
{
Collection distinctConcepts = new ArrayList();
for (Concept concept : this.concepts())
{
if (!isContained(concept, distinctConcepts))
{
distinctConcepts.add(concept);
}
}
return distinctConcepts;
}
public void addAxiom(SemanticAxiom axiom)
{
if (axiom instanceof Concept)
{
addConcept((Concept) axiom);
}
if (axiom instanceof Instance)
{
addInstance((Instance) axiom);
}
}
public void addConcept(Concept concept)
{
if (!concepts.contains(concept))
{
concepts.add(concept);
}
}
public Collection getInstancesByConceptURI(SemanticIdentifier sa) throws SemanticModelException
{
Collection conceptsToSearch = getConcepts(sa);
Collection instancesCol = new ArrayList();
for (Instance instance : instances)
{
for (Concept conceptToSearch : conceptsToSearch)
{
if (instance.getConcept().semanticAxiom().equals(conceptToSearch))
{
instancesCol.add(instance);
}
}
}
return instancesCol;
}
public Collection instances()
{
return instances;
}
public Collection getDistinctInstances()
{
Collection distinctInstances = new ArrayList();
for (Instance instance : this.instances())
{
if (!isContained(instance, distinctInstances))
{
distinctInstances.add(instance);
}
}
return distinctInstances;
}
public void addInstance(Instance instance)
{
if (!instances.contains(instance))
{
instances.add(instance);
}
}
public Collection imports()
{
return imports;
}
public void addImport(IImport imp)
{
if (!imports.contains(imp))
{
imports.add(imp);
}
}
@Override
public SemanticAxiom joinWith(SemanticAxiom other) throws SemanticModelException
{
if (!(other instanceof Ontology))
{
throw new SemanticModelException("Not an ontology: " + other.toString(), null);
}
if (other.getSemanticIdentifier().equals(getSemanticIdentifier()))
{
Ontology otherOntology = (Ontology) other;
for (IImport imp : otherOntology.imports())
{
addImport(imp);
}
for (Concept concept : otherOntology.concepts())
{
addConcept(concept);
}
for (Instance instance : otherOntology.instances())
{
addInstance(instance);
}
}
return this;
}
@Override
public Collection axioms()
{
Collection axioms = new ArrayList();
axioms.addAll(getDistinctConcepts());
axioms.addAll(getDistinctInstances());
return axioms;
}
@Override
public IVersionNumber version() throws SemanticModelException
{
return getVersionNumber();
}
@Override
public IOntologyLanguage ontologyLanguage() throws SemanticModelException
{
return getOntologyLanguage();
}
@Override
public INaturalLanguage naturalLanguage() throws SemanticModelException
{
return getNaturalLanguage();
}
@Override
public Collection properties() throws SemanticModelException
{
Collection properties = new ArrayList();
for (Concept concept : concepts())
{
properties.add(new Property().setSubject(this).setObject(concept));
}
for (Instance instance : instances())
{
properties.add(new PropertyValue().setSubject(this).setObject(instance));
}
return properties;
}
private static boolean isContained(SemanticAxiom axiomContained, Collection axioms)
{
if (null == axiomContained) return false;
for (Object axiom : axioms)
{
if ( null != axiom
&& null != ((SemanticAxiom)axiom).getSemanticIdentifier()
&& null != axiomContained.getSemanticIdentifier()
&& ((SemanticAxiom)axiom).getSemanticIdentifier().equals(axiomContained.getSemanticIdentifier()) )
{
return true;
}
}
return false;
}
}