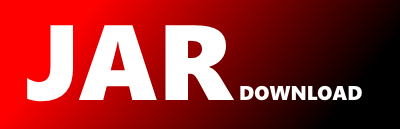
com.hibegin.http.server.web.Router Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simplewebserver Show documentation
Show all versions of simplewebserver Show documentation
Simple, flexible, less dependent, more extended. Less memory footprint, can quickly build Web project.
Can quickly run embedded, Android devices
package com.hibegin.http.server.web;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
public class Router {
private final Map routerMap = new HashMap();
public void addMapper(String urlPath, Class extends Controller> clazz) {
if ("/".equals(urlPath)) {
urlPath = "";
}
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
if (method.getModifiers() == Modifier.PUBLIC) {
getRouterMap().put(urlPath + "/" + method.getName(), method);
}
if (Objects.equals(method.getName(), "index")) {
getRouterMap().put(urlPath, method);
getRouterMap().put(urlPath + "/", method);
}
}
}
public void addMapper(String urlPath, Class extends Controller> clazz, String methodName) {
if ("/".equals(urlPath)) {
urlPath = "";
}
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
if (Objects.equals(method.getName(), methodName)) {
getRouterMap().put(urlPath, method);
}
}
}
public Method getMethod(String url) {
Method method = getRouterMap().get(url);
if (method == null && url.contains("-")) {
method = getRouterMap().get(url.substring(0, url.indexOf("-")));
}
return method;
}
public Map getRouterMap() {
return routerMap;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
if (!routerMap.isEmpty()) {
sb.append("\r\n=========== Router Info ===========");
}
for (Map.Entry entry : routerMap.entrySet()) {
sb.append("\r\n").append(entry.getKey()).append(" -> ").append(entry.getValue());
}
if (!routerMap.isEmpty()) {
sb.append("\r\n===================================");
}
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy