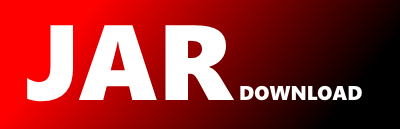
com.highmobility.autoapi.Charging Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright (c) 2014- High-Mobility GmbH (https://high-mobility.com)
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.highmobility.autoapi;
import com.highmobility.autoapi.property.ByteEnum;
import com.highmobility.autoapi.property.Property;
import com.highmobility.autoapi.value.ActiveState;
import com.highmobility.autoapi.value.DepartureTime;
import com.highmobility.autoapi.value.EnabledState;
import com.highmobility.autoapi.value.Position;
import com.highmobility.autoapi.value.ReductionTime;
import com.highmobility.autoapi.value.Timer;
import com.highmobility.autoapi.value.measurement.Duration;
import com.highmobility.autoapi.value.measurement.ElectricCurrent;
import com.highmobility.autoapi.value.measurement.ElectricPotentialDifference;
import com.highmobility.autoapi.value.measurement.Length;
import com.highmobility.autoapi.value.measurement.Power;
import com.highmobility.autoapi.value.measurement.Temperature;
import com.highmobility.value.Bytes;
import java.util.ArrayList;
import java.util.List;
import static com.highmobility.utils.ByteUtils.hexFromByte;
/**
* The Charging capability
*/
public class Charging {
public static final int IDENTIFIER = Identifier.CHARGING;
public static final byte PROPERTY_ESTIMATED_RANGE = 0x02;
public static final byte PROPERTY_BATTERY_LEVEL = 0x03;
public static final byte PROPERTY_BATTERY_CURRENT_AC = 0x04;
public static final byte PROPERTY_BATTERY_CURRENT_DC = 0x05;
public static final byte PROPERTY_CHARGER_VOLTAGE_AC = 0x06;
public static final byte PROPERTY_CHARGER_VOLTAGE_DC = 0x07;
public static final byte PROPERTY_CHARGE_LIMIT = 0x08;
public static final byte PROPERTY_TIME_TO_COMPLETE_CHARGE = 0x09;
public static final byte PROPERTY_CHARGING_RATE_KW = 0x0a;
public static final byte PROPERTY_CHARGE_PORT_STATE = 0x0b;
public static final byte PROPERTY_CHARGE_MODE = 0x0c;
public static final byte PROPERTY_MAX_CHARGING_CURRENT = 0x0e;
public static final byte PROPERTY_PLUG_TYPE = 0x0f;
public static final byte PROPERTY_CHARGING_WINDOW_CHOSEN = 0x10;
public static final byte PROPERTY_DEPARTURE_TIMES = 0x11;
public static final byte PROPERTY_REDUCTION_TIMES = 0x13;
public static final byte PROPERTY_BATTERY_TEMPERATURE = 0x14;
public static final byte PROPERTY_TIMERS = 0x15;
public static final byte PROPERTY_PLUGGED_IN = 0x16;
public static final byte PROPERTY_STATUS = 0x17;
public static final byte PROPERTY_CHARGING_RATE = 0x18;
public static final byte PROPERTY_BATTERY_CURRENT = 0x19;
public static final byte PROPERTY_CHARGER_VOLTAGE = 0x1a;
public static final byte PROPERTY_CURRENT_TYPE = 0x1b;
public static final byte PROPERTY_MAX_RANGE = 0x1c;
public static final byte PROPERTY_STARTER_BATTERY_STATE = 0x1d;
public static final byte PROPERTY_SMART_CHARGING_STATUS = 0x1e;
public static final byte PROPERTY_BATTERY_LEVEL_AT_DEPARTURE = 0x1f;
public static final byte PROPERTY_PRECONDITIONING_DEPARTURE_STATUS = 0x20;
public static final byte PROPERTY_PRECONDITIONING_IMMEDIATE_STATUS = 0x21;
public static final byte PROPERTY_PRECONDITIONING_DEPARTURE_ENABLED = 0x22;
public static final byte PROPERTY_PRECONDITIONING_ERROR = 0x23;
/**
* Get Charging property availability information.
*/
public static class GetStateAvailability extends GetAvailabilityCommand {
/**
* Get every Charging property availability
*/
public GetStateAvailability() {
super(IDENTIFIER);
}
/**
* Get specific Charging property availabilities
*
* @param propertyIdentifiers The property identifierBytes
*/
public GetStateAvailability(Bytes propertyIdentifiers) {
super(IDENTIFIER, propertyIdentifiers);
}
/**
* Get specific Charging property availabilities
*
* @param propertyIdentifiers The property identifierBytes
*/
public GetStateAvailability(byte... propertyIdentifiers) {
super(IDENTIFIER, new Bytes(propertyIdentifiers));
}
GetStateAvailability(byte[] bytes, @SuppressWarnings("unused") boolean fromRaw) throws CommandParseException {
super(bytes);
}
}
/**
* Get Charging properties
*/
public static class GetState extends GetCommand {
/**
* Get all Charging properties
*/
public GetState() {
super(State.class, IDENTIFIER);
}
/**
* Get specific Charging properties
*
* @param propertyIdentifiers The property identifiers
*/
public GetState(Bytes propertyIdentifiers) {
super(State.class, IDENTIFIER, propertyIdentifiers);
}
/**
* Get specific Charging properties
*
* @param propertyIdentifiers The property identifiers
*/
public GetState(byte... propertyIdentifiers) {
super(State.class, IDENTIFIER, new Bytes(propertyIdentifiers));
}
GetState(byte[] bytes, @SuppressWarnings("unused") boolean fromRaw) throws CommandParseException {
super(State.class, bytes);
}
}
/**
* Get specific Charging properties
*
* @deprecated use {@link GetState#GetState(byte...)} instead
*/
@Deprecated
public static class GetProperties extends GetCommand {
/**
* @param propertyIdentifiers The property identifiers
*/
public GetProperties(Bytes propertyIdentifiers) {
super(State.class, IDENTIFIER, propertyIdentifiers);
}
/**
* @param propertyIdentifiers The property identifiers
*/
public GetProperties(byte... propertyIdentifiers) {
super(State.class, IDENTIFIER, new Bytes(propertyIdentifiers));
}
GetProperties(byte[] bytes, @SuppressWarnings("unused") boolean fromRaw) throws CommandParseException {
super(State.class, bytes);
}
}
/**
* Start stop charging
*/
public static class StartStopCharging extends SetCommand {
Property status = new Property<>(Status.class, PROPERTY_STATUS);
/**
* @return The status
*/
public Property getStatus() {
return status;
}
/**
* Start stop charging
*
* @param status The status
*/
public StartStopCharging(Status status) {
super(IDENTIFIER);
if (status == Status.CHARGING_COMPLETE ||
status == Status.INITIALISING ||
status == Status.CHARGING_PAUSED ||
status == Status.CHARGING_ERROR ||
status == Status.CABLE_UNPLUGGED ||
status == Status.SLOW_CHARGING ||
status == Status.FAST_CHARGING ||
status == Status.DISCHARGING ||
status == Status.FOREIGN_OBJECT_DETECTED) throw new IllegalArgumentException();
addProperty(this.status.update(status));
createBytes();
}
StartStopCharging(byte[] bytes) throws CommandParseException, NoPropertiesException {
super(bytes);
while (propertyIterator.hasNext()) {
propertyIterator.parseNext(p -> {
if (p.getPropertyIdentifier() == PROPERTY_STATUS) return status.update(p);
return null;
});
}
if (this.status.getValue() == null)
throw new NoPropertiesException();
}
}
/**
* Set charge limit
*/
public static class SetChargeLimit extends SetCommand {
Property chargeLimit = new Property<>(Double.class, PROPERTY_CHARGE_LIMIT);
/**
* @return The charge limit
*/
public Property getChargeLimit() {
return chargeLimit;
}
/**
* Set charge limit
*
* @param chargeLimit Charge limit percentage between 0.0-1.0
*/
public SetChargeLimit(Double chargeLimit) {
super(IDENTIFIER);
addProperty(this.chargeLimit.update(chargeLimit));
createBytes();
}
SetChargeLimit(byte[] bytes) throws CommandParseException, NoPropertiesException {
super(bytes);
while (propertyIterator.hasNext()) {
propertyIterator.parseNext(p -> {
if (p.getPropertyIdentifier() == PROPERTY_CHARGE_LIMIT) return chargeLimit.update(p);
return null;
});
}
if (this.chargeLimit.getValue() == null)
throw new NoPropertiesException();
}
}
/**
* Open close charging port
*/
public static class OpenCloseChargingPort extends SetCommand {
Property chargePortState = new Property<>(Position.class, PROPERTY_CHARGE_PORT_STATE);
/**
* @return The charge port state
*/
public Property getChargePortState() {
return chargePortState;
}
/**
* Open close charging port
*
* @param chargePortState The charge port state
*/
public OpenCloseChargingPort(Position chargePortState) {
super(IDENTIFIER);
addProperty(this.chargePortState.update(chargePortState));
createBytes();
}
OpenCloseChargingPort(byte[] bytes) throws CommandParseException, NoPropertiesException {
super(bytes);
while (propertyIterator.hasNext()) {
propertyIterator.parseNext(p -> {
if (p.getPropertyIdentifier() == PROPERTY_CHARGE_PORT_STATE) return chargePortState.update(p);
return null;
});
}
if (this.chargePortState.getValue() == null)
throw new NoPropertiesException();
}
}
/**
* Set charge mode
*/
public static class SetChargeMode extends SetCommand {
Property chargeMode = new Property<>(ChargeMode.class, PROPERTY_CHARGE_MODE);
/**
* @return The charge mode
*/
public Property getChargeMode() {
return chargeMode;
}
/**
* Set charge mode
*
* @param chargeMode The charge mode
*/
public SetChargeMode(ChargeMode chargeMode) {
super(IDENTIFIER);
if (chargeMode == ChargeMode.INDUCTIVE) throw new IllegalArgumentException();
addProperty(this.chargeMode.update(chargeMode));
createBytes();
}
SetChargeMode(byte[] bytes) throws CommandParseException, NoPropertiesException {
super(bytes);
while (propertyIterator.hasNext()) {
propertyIterator.parseNext(p -> {
if (p.getPropertyIdentifier() == PROPERTY_CHARGE_MODE) return chargeMode.update(p);
return null;
});
}
if (this.chargeMode.getValue() == null)
throw new NoPropertiesException();
}
}
/**
* Set charging timers
*/
public static class SetChargingTimers extends SetCommand {
List> timers;
/**
* @return The timers
*/
public List> getTimers() {
return timers;
}
/**
* Set charging timers
*
* @param timers The timers
*/
public SetChargingTimers(List timers) {
super(IDENTIFIER);
final ArrayList> timersBuilder = new ArrayList<>();
if (timers != null) {
for (Timer timer : timers) {
Property prop = new Property<>(0x15, timer);
timersBuilder.add(prop);
addProperty(prop);
}
}
this.timers = timersBuilder;
createBytes();
}
SetChargingTimers(byte[] bytes) throws CommandParseException, NoPropertiesException {
super(bytes);
final ArrayList> timersBuilder = new ArrayList<>();
while (propertyIterator.hasNext()) {
propertyIterator.parseNext(p -> {
if (p.getPropertyIdentifier() == PROPERTY_TIMERS) {
Property timer = new Property<>(Timer.class, p);
timersBuilder.add(timer);
return timer;
}
return null;
});
}
timers = timersBuilder;
if (this.timers.size() == 0)
throw new NoPropertiesException();
}
}
/**
* Set reduction of charging current times
*/
public static class SetReductionOfChargingCurrentTimes extends SetCommand {
List> reductionTimes;
/**
* @return The reduction times
*/
public List> getReductionTimes() {
return reductionTimes;
}
/**
* Set reduction of charging current times
*
* @param reductionTimes The reduction times
*/
public SetReductionOfChargingCurrentTimes(List reductionTimes) {
super(IDENTIFIER);
final ArrayList> reductionTimesBuilder = new ArrayList<>();
if (reductionTimes != null) {
for (ReductionTime reductionTime : reductionTimes) {
Property prop = new Property<>(0x13, reductionTime);
reductionTimesBuilder.add(prop);
addProperty(prop);
}
}
this.reductionTimes = reductionTimesBuilder;
createBytes();
}
SetReductionOfChargingCurrentTimes(byte[] bytes) throws CommandParseException, NoPropertiesException {
super(bytes);
final ArrayList> reductionTimesBuilder = new ArrayList<>();
while (propertyIterator.hasNext()) {
propertyIterator.parseNext(p -> {
if (p.getPropertyIdentifier() == PROPERTY_REDUCTION_TIMES) {
Property reductionTime = new Property<>(ReductionTime.class, p);
reductionTimesBuilder.add(reductionTime);
return reductionTime;
}
return null;
});
}
reductionTimes = reductionTimesBuilder;
if (this.reductionTimes.size() == 0)
throw new NoPropertiesException();
}
}
/**
* The charging state
*/
public static class State extends SetCommand {
Property estimatedRange = new Property<>(Length.class, PROPERTY_ESTIMATED_RANGE);
Property batteryLevel = new Property<>(Double.class, PROPERTY_BATTERY_LEVEL);
Property batteryCurrentAC = new Property<>(ElectricCurrent.class, PROPERTY_BATTERY_CURRENT_AC);
Property batteryCurrentDC = new Property<>(ElectricCurrent.class, PROPERTY_BATTERY_CURRENT_DC);
Property chargerVoltageAC = new Property<>(ElectricPotentialDifference.class, PROPERTY_CHARGER_VOLTAGE_AC);
Property chargerVoltageDC = new Property<>(ElectricPotentialDifference.class, PROPERTY_CHARGER_VOLTAGE_DC);
Property chargeLimit = new Property<>(Double.class, PROPERTY_CHARGE_LIMIT);
Property timeToCompleteCharge = new Property<>(Duration.class, PROPERTY_TIME_TO_COMPLETE_CHARGE);
Property chargingRateKW = new Property<>(Power.class, PROPERTY_CHARGING_RATE_KW);
Property chargePortState = new Property<>(Position.class, PROPERTY_CHARGE_PORT_STATE);
Property chargeMode = new Property<>(ChargeMode.class, PROPERTY_CHARGE_MODE);
Property maxChargingCurrent = new Property<>(ElectricCurrent.class, PROPERTY_MAX_CHARGING_CURRENT);
Property plugType = new Property<>(PlugType.class, PROPERTY_PLUG_TYPE);
Property chargingWindowChosen = new Property<>(ChargingWindowChosen.class, PROPERTY_CHARGING_WINDOW_CHOSEN);
List> departureTimes;
List> reductionTimes;
Property batteryTemperature = new Property<>(Temperature.class, PROPERTY_BATTERY_TEMPERATURE);
List> timers;
Property pluggedIn = new Property<>(PluggedIn.class, PROPERTY_PLUGGED_IN);
Property status = new Property<>(Status.class, PROPERTY_STATUS);
Property chargingRate = new Property<>(Power.class, PROPERTY_CHARGING_RATE);
Property batteryCurrent = new Property<>(ElectricCurrent.class, PROPERTY_BATTERY_CURRENT);
Property chargerVoltage = new Property<>(ElectricPotentialDifference.class, PROPERTY_CHARGER_VOLTAGE);
Property currentType = new Property<>(CurrentType.class, PROPERTY_CURRENT_TYPE);
Property maxRange = new Property<>(Length.class, PROPERTY_MAX_RANGE);
Property starterBatteryState = new Property<>(StarterBatteryState.class, PROPERTY_STARTER_BATTERY_STATE);
Property smartChargingStatus = new Property<>(SmartChargingStatus.class, PROPERTY_SMART_CHARGING_STATUS);
Property batteryLevelAtDeparture = new Property<>(Double.class, PROPERTY_BATTERY_LEVEL_AT_DEPARTURE);
Property preconditioningDepartureStatus = new Property<>(ActiveState.class, PROPERTY_PRECONDITIONING_DEPARTURE_STATUS);
Property preconditioningImmediateStatus = new Property<>(ActiveState.class, PROPERTY_PRECONDITIONING_IMMEDIATE_STATUS);
Property preconditioningDepartureEnabled = new Property<>(EnabledState.class, PROPERTY_PRECONDITIONING_DEPARTURE_ENABLED);
Property preconditioningError = new Property<>(PreconditioningError.class, PROPERTY_PRECONDITIONING_ERROR);
/**
* @return Estimated range
*/
public Property getEstimatedRange() {
return estimatedRange;
}
/**
* @return Battery level percentage between 0.0-1.0
*/
public Property getBatteryLevel() {
return batteryLevel;
}
/**
* @return Battery alternating current
* @deprecated moved AC/DC distinction into a separate property. Replaced by {@link #getBatteryCurrent()}
*/
@Deprecated
public Property getBatteryCurrentAC() {
return batteryCurrentAC;
}
/**
* @return Battery direct current
* @deprecated moved AC/DC distinction into a separate property. Replaced by {@link #getBatteryCurrent()}
*/
@Deprecated
public Property getBatteryCurrentDC() {
return batteryCurrentDC;
}
/**
* @return Charger voltage for alternating current
* @deprecated moved AC/DC distinction into a separate property. Replaced by {@link #getChargerVoltage()}
*/
@Deprecated
public Property getChargerVoltageAC() {
return chargerVoltageAC;
}
/**
* @return Charger voltage for direct current
* @deprecated moved AC/DC distinction into a separate property. Replaced by {@link #getChargerVoltage()}
*/
@Deprecated
public Property getChargerVoltageDC() {
return chargerVoltageDC;
}
/**
* @return Charge limit percentage between 0.0-1.0
*/
public Property getChargeLimit() {
return chargeLimit;
}
/**
* @return Time until charging completed
*/
public Property getTimeToCompleteCharge() {
return timeToCompleteCharge;
}
/**
* @return Charging rate
* @deprecated removed the unit from the name. Replaced by {@link #getChargingRate()}
*/
@Deprecated
public Property getChargingRateKW() {
return chargingRateKW;
}
/**
* @return The charge port state
*/
public Property getChargePortState() {
return chargePortState;
}
/**
* @return The charge mode
*/
public Property getChargeMode() {
return chargeMode;
}
/**
* @return Maximum charging current
*/
public Property getMaxChargingCurrent() {
return maxChargingCurrent;
}
/**
* @return The plug type
*/
public Property getPlugType() {
return plugType;
}
/**
* @return The charging window chosen
*/
public Property getChargingWindowChosen() {
return chargingWindowChosen;
}
/**
* @return The departure times
*/
public List> getDepartureTimes() {
return departureTimes;
}
/**
* @return The reduction times
*/
public List> getReductionTimes() {
return reductionTimes;
}
/**
* @return Battery temperature
*/
public Property getBatteryTemperature() {
return batteryTemperature;
}
/**
* @return The timers
*/
public List> getTimers() {
return timers;
}
/**
* @return The plugged in
*/
public Property getPluggedIn() {
return pluggedIn;
}
/**
* @return The status
*/
public Property getStatus() {
return status;
}
/**
* @return Charge rate when charging
*/
public Property getChargingRate() {
return chargingRate;
}
/**
* @return Battery current
*/
public Property getBatteryCurrent() {
return batteryCurrent;
}
/**
* @return Charger voltage
*/
public Property getChargerVoltage() {
return chargerVoltage;
}
/**
* @return Type of current in use
*/
public Property getCurrentType() {
return currentType;
}
/**
* @return Maximum electric range with 100% of battery
*/
public Property getMaxRange() {
return maxRange;
}
/**
* @return State of the starter battery
*/
public Property getStarterBatteryState() {
return starterBatteryState;
}
/**
* @return Status of optimized/intelligent charging
*/
public Property getSmartChargingStatus() {
return smartChargingStatus;
}
/**
* @return Battery charge level expected at time of departure
*/
public Property getBatteryLevelAtDeparture() {
return batteryLevelAtDeparture;
}
/**
* @return Status of preconditioning at departure time
*/
public Property getPreconditioningDepartureStatus() {
return preconditioningDepartureStatus;
}
/**
* @return Status of immediate preconditioning
*/
public Property getPreconditioningImmediateStatus() {
return preconditioningImmediateStatus;
}
/**
* @return Preconditioning activation status at departure
*/
public Property getPreconditioningDepartureEnabled() {
return preconditioningDepartureEnabled;
}
/**
* @return Preconditioning error if one is encountered
*/
public Property getPreconditioningError() {
return preconditioningError;
}
State(byte[] bytes) throws CommandParseException {
super(bytes);
final ArrayList> departureTimesBuilder = new ArrayList<>();
final ArrayList> reductionTimesBuilder = new ArrayList<>();
final ArrayList> timersBuilder = new ArrayList<>();
while (propertyIterator.hasNext()) {
propertyIterator.parseNext(p -> {
switch (p.getPropertyIdentifier()) {
case PROPERTY_ESTIMATED_RANGE: return estimatedRange.update(p);
case PROPERTY_BATTERY_LEVEL: return batteryLevel.update(p);
case PROPERTY_BATTERY_CURRENT_AC: return batteryCurrentAC.update(p);
case PROPERTY_BATTERY_CURRENT_DC: return batteryCurrentDC.update(p);
case PROPERTY_CHARGER_VOLTAGE_AC: return chargerVoltageAC.update(p);
case PROPERTY_CHARGER_VOLTAGE_DC: return chargerVoltageDC.update(p);
case PROPERTY_CHARGE_LIMIT: return chargeLimit.update(p);
case PROPERTY_TIME_TO_COMPLETE_CHARGE: return timeToCompleteCharge.update(p);
case PROPERTY_CHARGING_RATE_KW: return chargingRateKW.update(p);
case PROPERTY_CHARGE_PORT_STATE: return chargePortState.update(p);
case PROPERTY_CHARGE_MODE: return chargeMode.update(p);
case PROPERTY_MAX_CHARGING_CURRENT: return maxChargingCurrent.update(p);
case PROPERTY_PLUG_TYPE: return plugType.update(p);
case PROPERTY_CHARGING_WINDOW_CHOSEN: return chargingWindowChosen.update(p);
case PROPERTY_DEPARTURE_TIMES:
Property departureTime = new Property<>(DepartureTime.class, p);
departureTimesBuilder.add(departureTime);
return departureTime;
case PROPERTY_REDUCTION_TIMES:
Property reductionTime = new Property<>(ReductionTime.class, p);
reductionTimesBuilder.add(reductionTime);
return reductionTime;
case PROPERTY_BATTERY_TEMPERATURE: return batteryTemperature.update(p);
case PROPERTY_TIMERS:
Property timer = new Property<>(Timer.class, p);
timersBuilder.add(timer);
return timer;
case PROPERTY_PLUGGED_IN: return pluggedIn.update(p);
case PROPERTY_STATUS: return status.update(p);
case PROPERTY_CHARGING_RATE: return chargingRate.update(p);
case PROPERTY_BATTERY_CURRENT: return batteryCurrent.update(p);
case PROPERTY_CHARGER_VOLTAGE: return chargerVoltage.update(p);
case PROPERTY_CURRENT_TYPE: return currentType.update(p);
case PROPERTY_MAX_RANGE: return maxRange.update(p);
case PROPERTY_STARTER_BATTERY_STATE: return starterBatteryState.update(p);
case PROPERTY_SMART_CHARGING_STATUS: return smartChargingStatus.update(p);
case PROPERTY_BATTERY_LEVEL_AT_DEPARTURE: return batteryLevelAtDeparture.update(p);
case PROPERTY_PRECONDITIONING_DEPARTURE_STATUS: return preconditioningDepartureStatus.update(p);
case PROPERTY_PRECONDITIONING_IMMEDIATE_STATUS: return preconditioningImmediateStatus.update(p);
case PROPERTY_PRECONDITIONING_DEPARTURE_ENABLED: return preconditioningDepartureEnabled.update(p);
case PROPERTY_PRECONDITIONING_ERROR: return preconditioningError.update(p);
}
return null;
});
}
departureTimes = departureTimesBuilder;
reductionTimes = reductionTimesBuilder;
timers = timersBuilder;
}
private State(Builder builder) {
super(builder);
estimatedRange = builder.estimatedRange;
batteryLevel = builder.batteryLevel;
batteryCurrentAC = builder.batteryCurrentAC;
batteryCurrentDC = builder.batteryCurrentDC;
chargerVoltageAC = builder.chargerVoltageAC;
chargerVoltageDC = builder.chargerVoltageDC;
chargeLimit = builder.chargeLimit;
timeToCompleteCharge = builder.timeToCompleteCharge;
chargingRateKW = builder.chargingRateKW;
chargePortState = builder.chargePortState;
chargeMode = builder.chargeMode;
maxChargingCurrent = builder.maxChargingCurrent;
plugType = builder.plugType;
chargingWindowChosen = builder.chargingWindowChosen;
departureTimes = builder.departureTimes;
reductionTimes = builder.reductionTimes;
batteryTemperature = builder.batteryTemperature;
timers = builder.timers;
pluggedIn = builder.pluggedIn;
status = builder.status;
chargingRate = builder.chargingRate;
batteryCurrent = builder.batteryCurrent;
chargerVoltage = builder.chargerVoltage;
currentType = builder.currentType;
maxRange = builder.maxRange;
starterBatteryState = builder.starterBatteryState;
smartChargingStatus = builder.smartChargingStatus;
batteryLevelAtDeparture = builder.batteryLevelAtDeparture;
preconditioningDepartureStatus = builder.preconditioningDepartureStatus;
preconditioningImmediateStatus = builder.preconditioningImmediateStatus;
preconditioningDepartureEnabled = builder.preconditioningDepartureEnabled;
preconditioningError = builder.preconditioningError;
}
public static final class Builder extends SetCommand.Builder {
private Property estimatedRange;
private Property batteryLevel;
private Property batteryCurrentAC;
private Property batteryCurrentDC;
private Property chargerVoltageAC;
private Property chargerVoltageDC;
private Property chargeLimit;
private Property timeToCompleteCharge;
private Property chargingRateKW;
private Property chargePortState;
private Property chargeMode;
private Property maxChargingCurrent;
private Property plugType;
private Property chargingWindowChosen;
private final List> departureTimes = new ArrayList<>();
private final List> reductionTimes = new ArrayList<>();
private Property batteryTemperature;
private final List> timers = new ArrayList<>();
private Property pluggedIn;
private Property status;
private Property chargingRate;
private Property batteryCurrent;
private Property chargerVoltage;
private Property currentType;
private Property maxRange;
private Property starterBatteryState;
private Property smartChargingStatus;
private Property batteryLevelAtDeparture;
private Property preconditioningDepartureStatus;
private Property preconditioningImmediateStatus;
private Property preconditioningDepartureEnabled;
private Property preconditioningError;
public Builder() {
super(IDENTIFIER);
}
public State build() {
return new State(this);
}
/**
* @param estimatedRange Estimated range
* @return The builder
*/
public Builder setEstimatedRange(Property estimatedRange) {
this.estimatedRange = estimatedRange.setIdentifier(PROPERTY_ESTIMATED_RANGE);
addProperty(this.estimatedRange);
return this;
}
/**
* @param batteryLevel Battery level percentage between 0.0-1.0
* @return The builder
*/
public Builder setBatteryLevel(Property batteryLevel) {
this.batteryLevel = batteryLevel.setIdentifier(PROPERTY_BATTERY_LEVEL);
addProperty(this.batteryLevel);
return this;
}
/**
* @param batteryCurrentAC Battery alternating current
* @return The builder
* @deprecated moved AC/DC distinction into a separate property. Replaced by {@link #getBatteryCurrent()}
*/
@Deprecated
public Builder setBatteryCurrentAC(Property batteryCurrentAC) {
this.batteryCurrentAC = batteryCurrentAC.setIdentifier(PROPERTY_BATTERY_CURRENT_AC);
addProperty(this.batteryCurrentAC);
return this;
}
/**
* @param batteryCurrentDC Battery direct current
* @return The builder
* @deprecated moved AC/DC distinction into a separate property. Replaced by {@link #getBatteryCurrent()}
*/
@Deprecated
public Builder setBatteryCurrentDC(Property batteryCurrentDC) {
this.batteryCurrentDC = batteryCurrentDC.setIdentifier(PROPERTY_BATTERY_CURRENT_DC);
addProperty(this.batteryCurrentDC);
return this;
}
/**
* @param chargerVoltageAC Charger voltage for alternating current
* @return The builder
* @deprecated moved AC/DC distinction into a separate property. Replaced by {@link #getChargerVoltage()}
*/
@Deprecated
public Builder setChargerVoltageAC(Property chargerVoltageAC) {
this.chargerVoltageAC = chargerVoltageAC.setIdentifier(PROPERTY_CHARGER_VOLTAGE_AC);
addProperty(this.chargerVoltageAC);
return this;
}
/**
* @param chargerVoltageDC Charger voltage for direct current
* @return The builder
* @deprecated moved AC/DC distinction into a separate property. Replaced by {@link #getChargerVoltage()}
*/
@Deprecated
public Builder setChargerVoltageDC(Property chargerVoltageDC) {
this.chargerVoltageDC = chargerVoltageDC.setIdentifier(PROPERTY_CHARGER_VOLTAGE_DC);
addProperty(this.chargerVoltageDC);
return this;
}
/**
* @param chargeLimit Charge limit percentage between 0.0-1.0
* @return The builder
*/
public Builder setChargeLimit(Property chargeLimit) {
this.chargeLimit = chargeLimit.setIdentifier(PROPERTY_CHARGE_LIMIT);
addProperty(this.chargeLimit);
return this;
}
/**
* @param timeToCompleteCharge Time until charging completed
* @return The builder
*/
public Builder setTimeToCompleteCharge(Property timeToCompleteCharge) {
this.timeToCompleteCharge = timeToCompleteCharge.setIdentifier(PROPERTY_TIME_TO_COMPLETE_CHARGE);
addProperty(this.timeToCompleteCharge);
return this;
}
/**
* @param chargingRateKW Charging rate
* @return The builder
* @deprecated removed the unit from the name. Replaced by {@link #getChargingRate()}
*/
@Deprecated
public Builder setChargingRateKW(Property chargingRateKW) {
this.chargingRateKW = chargingRateKW.setIdentifier(PROPERTY_CHARGING_RATE_KW);
addProperty(this.chargingRateKW);
return this;
}
/**
* @param chargePortState The charge port state
* @return The builder
*/
public Builder setChargePortState(Property chargePortState) {
this.chargePortState = chargePortState.setIdentifier(PROPERTY_CHARGE_PORT_STATE);
addProperty(this.chargePortState);
return this;
}
/**
* @param chargeMode The charge mode
* @return The builder
*/
public Builder setChargeMode(Property chargeMode) {
this.chargeMode = chargeMode.setIdentifier(PROPERTY_CHARGE_MODE);
addProperty(this.chargeMode);
return this;
}
/**
* @param maxChargingCurrent Maximum charging current
* @return The builder
*/
public Builder setMaxChargingCurrent(Property maxChargingCurrent) {
this.maxChargingCurrent = maxChargingCurrent.setIdentifier(PROPERTY_MAX_CHARGING_CURRENT);
addProperty(this.maxChargingCurrent);
return this;
}
/**
* @param plugType The plug type
* @return The builder
*/
public Builder setPlugType(Property plugType) {
this.plugType = plugType.setIdentifier(PROPERTY_PLUG_TYPE);
addProperty(this.plugType);
return this;
}
/**
* @param chargingWindowChosen The charging window chosen
* @return The builder
*/
public Builder setChargingWindowChosen(Property chargingWindowChosen) {
this.chargingWindowChosen = chargingWindowChosen.setIdentifier(PROPERTY_CHARGING_WINDOW_CHOSEN);
addProperty(this.chargingWindowChosen);
return this;
}
/**
* Add an array of departure times.
*
* @param departureTimes The departure times
* @return The builder
*/
public Builder setDepartureTimes(Property[] departureTimes) {
this.departureTimes.clear();
for (int i = 0; i < departureTimes.length; i++) {
addDepartureTime(departureTimes[i]);
}
return this;
}
/**
* Add a single departure time.
*
* @param departureTime The departure time
* @return The builder
*/
public Builder addDepartureTime(Property departureTime) {
departureTime.setIdentifier(PROPERTY_DEPARTURE_TIMES);
addProperty(departureTime);
departureTimes.add(departureTime);
return this;
}
/**
* Add an array of reduction times.
*
* @param reductionTimes The reduction times
* @return The builder
*/
public Builder setReductionTimes(Property[] reductionTimes) {
this.reductionTimes.clear();
for (int i = 0; i < reductionTimes.length; i++) {
addReductionTime(reductionTimes[i]);
}
return this;
}
/**
* Add a single reduction time.
*
* @param reductionTime The reduction time
* @return The builder
*/
public Builder addReductionTime(Property reductionTime) {
reductionTime.setIdentifier(PROPERTY_REDUCTION_TIMES);
addProperty(reductionTime);
reductionTimes.add(reductionTime);
return this;
}
/**
* @param batteryTemperature Battery temperature
* @return The builder
*/
public Builder setBatteryTemperature(Property batteryTemperature) {
this.batteryTemperature = batteryTemperature.setIdentifier(PROPERTY_BATTERY_TEMPERATURE);
addProperty(this.batteryTemperature);
return this;
}
/**
* Add an array of timers.
*
* @param timers The timers
* @return The builder
*/
public Builder setTimers(Property[] timers) {
this.timers.clear();
for (int i = 0; i < timers.length; i++) {
addTimer(timers[i]);
}
return this;
}
/**
* Add a single timer.
*
* @param timer The timer
* @return The builder
*/
public Builder addTimer(Property timer) {
timer.setIdentifier(PROPERTY_TIMERS);
addProperty(timer);
timers.add(timer);
return this;
}
/**
* @param pluggedIn The plugged in
* @return The builder
*/
public Builder setPluggedIn(Property pluggedIn) {
this.pluggedIn = pluggedIn.setIdentifier(PROPERTY_PLUGGED_IN);
addProperty(this.pluggedIn);
return this;
}
/**
* @param status The status
* @return The builder
*/
public Builder setStatus(Property status) {
this.status = status.setIdentifier(PROPERTY_STATUS);
addProperty(this.status);
return this;
}
/**
* @param chargingRate Charge rate when charging
* @return The builder
*/
public Builder setChargingRate(Property chargingRate) {
this.chargingRate = chargingRate.setIdentifier(PROPERTY_CHARGING_RATE);
addProperty(this.chargingRate);
return this;
}
/**
* @param batteryCurrent Battery current
* @return The builder
*/
public Builder setBatteryCurrent(Property batteryCurrent) {
this.batteryCurrent = batteryCurrent.setIdentifier(PROPERTY_BATTERY_CURRENT);
addProperty(this.batteryCurrent);
return this;
}
/**
* @param chargerVoltage Charger voltage
* @return The builder
*/
public Builder setChargerVoltage(Property chargerVoltage) {
this.chargerVoltage = chargerVoltage.setIdentifier(PROPERTY_CHARGER_VOLTAGE);
addProperty(this.chargerVoltage);
return this;
}
/**
* @param currentType Type of current in use
* @return The builder
*/
public Builder setCurrentType(Property currentType) {
this.currentType = currentType.setIdentifier(PROPERTY_CURRENT_TYPE);
addProperty(this.currentType);
return this;
}
/**
* @param maxRange Maximum electric range with 100% of battery
* @return The builder
*/
public Builder setMaxRange(Property maxRange) {
this.maxRange = maxRange.setIdentifier(PROPERTY_MAX_RANGE);
addProperty(this.maxRange);
return this;
}
/**
* @param starterBatteryState State of the starter battery
* @return The builder
*/
public Builder setStarterBatteryState(Property starterBatteryState) {
this.starterBatteryState = starterBatteryState.setIdentifier(PROPERTY_STARTER_BATTERY_STATE);
addProperty(this.starterBatteryState);
return this;
}
/**
* @param smartChargingStatus Status of optimized/intelligent charging
* @return The builder
*/
public Builder setSmartChargingStatus(Property smartChargingStatus) {
this.smartChargingStatus = smartChargingStatus.setIdentifier(PROPERTY_SMART_CHARGING_STATUS);
addProperty(this.smartChargingStatus);
return this;
}
/**
* @param batteryLevelAtDeparture Battery charge level expected at time of departure
* @return The builder
*/
public Builder setBatteryLevelAtDeparture(Property batteryLevelAtDeparture) {
this.batteryLevelAtDeparture = batteryLevelAtDeparture.setIdentifier(PROPERTY_BATTERY_LEVEL_AT_DEPARTURE);
addProperty(this.batteryLevelAtDeparture);
return this;
}
/**
* @param preconditioningDepartureStatus Status of preconditioning at departure time
* @return The builder
*/
public Builder setPreconditioningDepartureStatus(Property preconditioningDepartureStatus) {
this.preconditioningDepartureStatus = preconditioningDepartureStatus.setIdentifier(PROPERTY_PRECONDITIONING_DEPARTURE_STATUS);
addProperty(this.preconditioningDepartureStatus);
return this;
}
/**
* @param preconditioningImmediateStatus Status of immediate preconditioning
* @return The builder
*/
public Builder setPreconditioningImmediateStatus(Property preconditioningImmediateStatus) {
this.preconditioningImmediateStatus = preconditioningImmediateStatus.setIdentifier(PROPERTY_PRECONDITIONING_IMMEDIATE_STATUS);
addProperty(this.preconditioningImmediateStatus);
return this;
}
/**
* @param preconditioningDepartureEnabled Preconditioning activation status at departure
* @return The builder
*/
public Builder setPreconditioningDepartureEnabled(Property preconditioningDepartureEnabled) {
this.preconditioningDepartureEnabled = preconditioningDepartureEnabled.setIdentifier(PROPERTY_PRECONDITIONING_DEPARTURE_ENABLED);
addProperty(this.preconditioningDepartureEnabled);
return this;
}
/**
* @param preconditioningError Preconditioning error if one is encountered
* @return The builder
*/
public Builder setPreconditioningError(Property preconditioningError) {
this.preconditioningError = preconditioningError.setIdentifier(PROPERTY_PRECONDITIONING_ERROR);
addProperty(this.preconditioningError);
return this;
}
}
}
public enum ChargeMode implements ByteEnum {
IMMEDIATE((byte) 0x00),
TIMER_BASED((byte) 0x01),
INDUCTIVE((byte) 0x02);
public static ChargeMode fromByte(byte byteValue) throws CommandParseException {
ChargeMode[] values = ChargeMode.values();
for (int i = 0; i < values.length; i++) {
ChargeMode state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.ChargeMode does not contain: " + hexFromByte(byteValue));
}
private final byte value;
ChargeMode(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
public enum PlugType implements ByteEnum {
TYPE_1((byte) 0x00),
TYPE_2((byte) 0x01),
CCS((byte) 0x02),
CHADEMO((byte) 0x03);
public static PlugType fromByte(byte byteValue) throws CommandParseException {
PlugType[] values = PlugType.values();
for (int i = 0; i < values.length; i++) {
PlugType state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.PlugType does not contain: " + hexFromByte(byteValue));
}
private final byte value;
PlugType(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
public enum ChargingWindowChosen implements ByteEnum {
NOT_CHOSEN((byte) 0x00),
CHOSEN((byte) 0x01);
public static ChargingWindowChosen fromByte(byte byteValue) throws CommandParseException {
ChargingWindowChosen[] values = ChargingWindowChosen.values();
for (int i = 0; i < values.length; i++) {
ChargingWindowChosen state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.ChargingWindowChosen does not contain: " + hexFromByte(byteValue));
}
private final byte value;
ChargingWindowChosen(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
public enum PluggedIn implements ByteEnum {
DISCONNECTED((byte) 0x00),
PLUGGED_IN((byte) 0x01);
public static PluggedIn fromByte(byte byteValue) throws CommandParseException {
PluggedIn[] values = PluggedIn.values();
for (int i = 0; i < values.length; i++) {
PluggedIn state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.PluggedIn does not contain: " + hexFromByte(byteValue));
}
private final byte value;
PluggedIn(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
public enum Status implements ByteEnum {
NOT_CHARGING((byte) 0x00),
CHARGING((byte) 0x01),
CHARGING_COMPLETE((byte) 0x02),
INITIALISING((byte) 0x03),
CHARGING_PAUSED((byte) 0x04),
CHARGING_ERROR((byte) 0x05),
CABLE_UNPLUGGED((byte) 0x06),
SLOW_CHARGING((byte) 0x07),
FAST_CHARGING((byte) 0x08),
DISCHARGING((byte) 0x09),
FOREIGN_OBJECT_DETECTED((byte) 0x0a);
public static Status fromByte(byte byteValue) throws CommandParseException {
Status[] values = Status.values();
for (int i = 0; i < values.length; i++) {
Status state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.Status does not contain: " + hexFromByte(byteValue));
}
private final byte value;
Status(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
public enum CurrentType implements ByteEnum {
ALTERNATING_CURRENT((byte) 0x00),
DIRECT_CURRENT((byte) 0x01);
public static CurrentType fromByte(byte byteValue) throws CommandParseException {
CurrentType[] values = CurrentType.values();
for (int i = 0; i < values.length; i++) {
CurrentType state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.CurrentType does not contain: " + hexFromByte(byteValue));
}
private final byte value;
CurrentType(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
public enum StarterBatteryState implements ByteEnum {
/**
* Battery charge is greater than 0%
*/
RED((byte) 0x00),
/**
* Battery charge is greater than 40%
*/
YELLOW((byte) 0x01),
/**
* Battery charge is greater than 70%
*/
GREEN((byte) 0x02);
public static StarterBatteryState fromByte(byte byteValue) throws CommandParseException {
StarterBatteryState[] values = StarterBatteryState.values();
for (int i = 0; i < values.length; i++) {
StarterBatteryState state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.StarterBatteryState does not contain: " + hexFromByte(byteValue));
}
private final byte value;
StarterBatteryState(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
public enum SmartChargingStatus implements ByteEnum {
WALLBOX_IS_ACTIVE((byte) 0x00),
/**
* Smart Charge Communication is active
*/
SCC_IS_ACTIVE((byte) 0x01),
/**
* On/Off-peak setting is active (charges when electricity is cheaper)
*/
PEAK_SETTING_ACTIVE((byte) 0x02);
public static SmartChargingStatus fromByte(byte byteValue) throws CommandParseException {
SmartChargingStatus[] values = SmartChargingStatus.values();
for (int i = 0; i < values.length; i++) {
SmartChargingStatus state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.SmartChargingStatus does not contain: " + hexFromByte(byteValue));
}
private final byte value;
SmartChargingStatus(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
public enum PreconditioningError implements ByteEnum {
NO_CHANGE((byte) 0x00),
/**
* Preconditioning not possible because battery or fuel is low
*/
NOT_POSSIBLE_LOW((byte) 0x01),
/**
* Preconditioning not possible because charging is not finished
*/
NOT_POSSIBLE_FINISHED((byte) 0x02),
AVAILABLE_AFTER_ENGINE_RESTART((byte) 0x03),
GENERAL_ERROR((byte) 0x04);
public static PreconditioningError fromByte(byte byteValue) throws CommandParseException {
PreconditioningError[] values = PreconditioningError.values();
for (int i = 0; i < values.length; i++) {
PreconditioningError state = values[i];
if (state.getByte() == byteValue) {
return state;
}
}
throw new CommandParseException("Charging.PreconditioningError does not contain: " + hexFromByte(byteValue));
}
private final byte value;
PreconditioningError(byte value) {
this.value = value;
}
@Override public byte getByte() {
return value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy