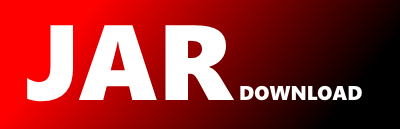
com.highmobility.autoapi.Diagnostics Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright (c) 2014- High-Mobility GmbH (https://high-mobility.com)
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.highmobility.autoapi;
import com.highmobility.autoapi.property.Property;
import com.highmobility.autoapi.value.ActiveState;
import com.highmobility.autoapi.value.CheckControlMessage;
import com.highmobility.autoapi.value.ConfirmedTroubleCode;
import com.highmobility.autoapi.value.DieselExhaustFilterStatus;
import com.highmobility.autoapi.value.FluidLevel;
import com.highmobility.autoapi.value.OemTroubleCodeValue;
import com.highmobility.autoapi.value.TirePressure;
import com.highmobility.autoapi.value.TirePressureStatus;
import com.highmobility.autoapi.value.TireTemperature;
import com.highmobility.autoapi.value.TroubleCode;
import com.highmobility.autoapi.value.WheelRpm;
import com.highmobility.autoapi.value.measurement.AngularVelocity;
import com.highmobility.autoapi.value.measurement.Duration;
import com.highmobility.autoapi.value.measurement.ElectricPotentialDifference;
import com.highmobility.autoapi.value.measurement.Length;
import com.highmobility.autoapi.value.measurement.Speed;
import com.highmobility.autoapi.value.measurement.Temperature;
import com.highmobility.autoapi.value.measurement.Volume;
import com.highmobility.value.Bytes;
import java.util.ArrayList;
import java.util.List;
/**
* The Diagnostics capability
*/
public class Diagnostics {
public static final int IDENTIFIER = Identifier.DIAGNOSTICS;
public static final byte PROPERTY_MILEAGE = 0x01;
public static final byte PROPERTY_ENGINE_OIL_TEMPERATURE = 0x02;
public static final byte PROPERTY_SPEED = 0x03;
public static final byte PROPERTY_ENGINE_RPM = 0x04;
public static final byte PROPERTY_FUEL_LEVEL = 0x05;
public static final byte PROPERTY_ESTIMATED_RANGE = 0x06;
public static final byte PROPERTY_WASHER_FLUID_LEVEL = 0x09;
public static final byte PROPERTY_BATTERY_VOLTAGE = 0x0b;
public static final byte PROPERTY_ADBLUE_LEVEL = 0x0c;
public static final byte PROPERTY_DISTANCE_SINCE_RESET = 0x0d;
public static final byte PROPERTY_DISTANCE_SINCE_START = 0x0e;
public static final byte PROPERTY_FUEL_VOLUME = 0x0f;
public static final byte PROPERTY_ANTI_LOCK_BRAKING = 0x10;
public static final byte PROPERTY_ENGINE_COOLANT_TEMPERATURE = 0x11;
public static final byte PROPERTY_ENGINE_TOTAL_OPERATING_HOURS = 0x12;
public static final byte PROPERTY_ENGINE_TOTAL_FUEL_CONSUMPTION = 0x13;
public static final byte PROPERTY_BRAKE_FLUID_LEVEL = 0x14;
public static final byte PROPERTY_ENGINE_TORQUE = 0x15;
public static final byte PROPERTY_ENGINE_LOAD = 0x16;
public static final byte PROPERTY_WHEEL_BASED_SPEED = 0x17;
public static final byte PROPERTY_BATTERY_LEVEL = 0x18;
public static final byte PROPERTY_CHECK_CONTROL_MESSAGES = 0x19;
public static final byte PROPERTY_TIRE_PRESSURES = 0x1a;
public static final byte PROPERTY_TIRE_TEMPERATURES = 0x1b;
public static final byte PROPERTY_WHEEL_RPMS = 0x1c;
public static final byte PROPERTY_TROUBLE_CODES = 0x1d;
public static final byte PROPERTY_MILEAGE_METERS = 0x1e;
public static final byte PROPERTY_ODOMETER = 0x1f;
public static final byte PROPERTY_ENGINE_TOTAL_OPERATING_TIME = 0x20;
public static final byte PROPERTY_TIRE_PRESSURE_STATUSES = 0x21;
public static final byte PROPERTY_BRAKE_LINING_WEAR_PRE_WARNING = 0x22;
public static final byte PROPERTY_ENGINE_OIL_LIFE_REMAINING = 0x23;
public static final byte PROPERTY_OEM_TROUBLE_CODE_VALUES = 0x24;
public static final byte PROPERTY_DIESEL_EXHAUST_FLUID_RANGE = 0x25;
public static final byte PROPERTY_DIESEL_PARTICULATE_FILTER_SOOT_LEVEL = 0x26;
public static final byte PROPERTY_CONFIRMED_TROUBLE_CODES = 0x27;
public static final byte PROPERTY_DIESEL_EXHAUST_FILTER_STATUS = 0x28;
/**
* Get Diagnostics property availability information.
*/
public static class GetStateAvailability extends GetAvailabilityCommand {
/**
* Get every Diagnostics property availability
*/
public GetStateAvailability() {
super(IDENTIFIER);
}
/**
* Get specific Diagnostics property availabilities
*
* @param propertyIdentifiers The property identifierBytes
*/
public GetStateAvailability(Bytes propertyIdentifiers) {
super(IDENTIFIER, propertyIdentifiers);
}
/**
* Get specific Diagnostics property availabilities
*
* @param propertyIdentifiers The property identifierBytes
*/
public GetStateAvailability(byte... propertyIdentifiers) {
super(IDENTIFIER, new Bytes(propertyIdentifiers));
}
GetStateAvailability(byte[] bytes, @SuppressWarnings("unused") boolean fromRaw) throws CommandParseException {
super(bytes);
}
}
/**
* Get Diagnostics properties
*/
public static class GetState extends GetCommand {
/**
* Get all Diagnostics properties
*/
public GetState() {
super(State.class, IDENTIFIER);
}
/**
* Get specific Diagnostics properties
*
* @param propertyIdentifiers The property identifiers
*/
public GetState(Bytes propertyIdentifiers) {
super(State.class, IDENTIFIER, propertyIdentifiers);
}
/**
* Get specific Diagnostics properties
*
* @param propertyIdentifiers The property identifiers
*/
public GetState(byte... propertyIdentifiers) {
super(State.class, IDENTIFIER, new Bytes(propertyIdentifiers));
}
GetState(byte[] bytes, @SuppressWarnings("unused") boolean fromRaw) throws CommandParseException {
super(State.class, bytes);
}
}
/**
* Get specific Diagnostics properties
*
* @deprecated use {@link GetState#GetState(byte...)} instead
*/
@Deprecated
public static class GetProperties extends GetCommand {
/**
* @param propertyIdentifiers The property identifiers
*/
public GetProperties(Bytes propertyIdentifiers) {
super(State.class, IDENTIFIER, propertyIdentifiers);
}
/**
* @param propertyIdentifiers The property identifiers
*/
public GetProperties(byte... propertyIdentifiers) {
super(State.class, IDENTIFIER, new Bytes(propertyIdentifiers));
}
GetProperties(byte[] bytes, @SuppressWarnings("unused") boolean fromRaw) throws CommandParseException {
super(State.class, bytes);
}
}
/**
* The diagnostics state
*/
public static class State extends SetCommand {
Property mileage = new Property<>(Length.class, PROPERTY_MILEAGE);
Property engineOilTemperature = new Property<>(Temperature.class, PROPERTY_ENGINE_OIL_TEMPERATURE);
Property speed = new Property<>(Speed.class, PROPERTY_SPEED);
Property engineRPM = new Property<>(AngularVelocity.class, PROPERTY_ENGINE_RPM);
Property fuelLevel = new Property<>(Double.class, PROPERTY_FUEL_LEVEL);
Property estimatedRange = new Property<>(Length.class, PROPERTY_ESTIMATED_RANGE);
Property washerFluidLevel = new Property<>(FluidLevel.class, PROPERTY_WASHER_FLUID_LEVEL);
Property batteryVoltage = new Property<>(ElectricPotentialDifference.class, PROPERTY_BATTERY_VOLTAGE);
Property adBlueLevel = new Property<>(Double.class, PROPERTY_ADBLUE_LEVEL);
Property distanceSinceReset = new Property<>(Length.class, PROPERTY_DISTANCE_SINCE_RESET);
Property distanceSinceStart = new Property<>(Length.class, PROPERTY_DISTANCE_SINCE_START);
Property fuelVolume = new Property<>(Volume.class, PROPERTY_FUEL_VOLUME);
Property antiLockBraking = new Property<>(ActiveState.class, PROPERTY_ANTI_LOCK_BRAKING);
Property engineCoolantTemperature = new Property<>(Temperature.class, PROPERTY_ENGINE_COOLANT_TEMPERATURE);
Property engineTotalOperatingHours = new Property<>(Duration.class, PROPERTY_ENGINE_TOTAL_OPERATING_HOURS);
Property engineTotalFuelConsumption = new Property<>(Volume.class, PROPERTY_ENGINE_TOTAL_FUEL_CONSUMPTION);
Property brakeFluidLevel = new Property<>(FluidLevel.class, PROPERTY_BRAKE_FLUID_LEVEL);
Property engineTorque = new Property<>(Double.class, PROPERTY_ENGINE_TORQUE);
Property engineLoad = new Property<>(Double.class, PROPERTY_ENGINE_LOAD);
Property wheelBasedSpeed = new Property<>(Speed.class, PROPERTY_WHEEL_BASED_SPEED);
Property batteryLevel = new Property<>(Double.class, PROPERTY_BATTERY_LEVEL);
List> checkControlMessages;
List> tirePressures;
List> tireTemperatures;
List> wheelRPMs;
List> troubleCodes;
Property mileageMeters = new Property<>(Length.class, PROPERTY_MILEAGE_METERS);
Property odometer = new Property<>(Length.class, PROPERTY_ODOMETER);
Property engineTotalOperatingTime = new Property<>(Duration.class, PROPERTY_ENGINE_TOTAL_OPERATING_TIME);
List> tirePressureStatuses;
Property brakeLiningWearPreWarning = new Property<>(ActiveState.class, PROPERTY_BRAKE_LINING_WEAR_PRE_WARNING);
Property engineOilLifeRemaining = new Property<>(Double.class, PROPERTY_ENGINE_OIL_LIFE_REMAINING);
List> oemTroubleCodeValues;
Property dieselExhaustFluidRange = new Property<>(Length.class, PROPERTY_DIESEL_EXHAUST_FLUID_RANGE);
Property dieselParticulateFilterSootLevel = new Property<>(Double.class, PROPERTY_DIESEL_PARTICULATE_FILTER_SOOT_LEVEL);
List> confirmedTroubleCodes;
Property dieselExhaustFilterStatus = new Property<>(DieselExhaustFilterStatus.class, PROPERTY_DIESEL_EXHAUST_FILTER_STATUS);
/**
* @return The vehicle mileage (odometer)
* @deprecated 'mileage' is an incorrect term for this. Replaced by {@link #getOdometer()}
*/
@Deprecated
public Property getMileage() {
return mileage;
}
/**
* @return Engine oil temperature
*/
public Property getEngineOilTemperature() {
return engineOilTemperature;
}
/**
* @return The vehicle speed
*/
public Property getSpeed() {
return speed;
}
/**
* @return Engine RPM (revolutions per minute)
*/
public Property getEngineRPM() {
return engineRPM;
}
/**
* @return Fuel level percentage between 0.0-1.0
*/
public Property getFuelLevel() {
return fuelLevel;
}
/**
* @return Estimated range (with combustion engine)
*/
public Property getEstimatedRange() {
return estimatedRange;
}
/**
* @return The washer fluid level
*/
public Property getWasherFluidLevel() {
return washerFluidLevel;
}
/**
* @return Battery voltage
*/
public Property getBatteryVoltage() {
return batteryVoltage;
}
/**
* @return AdBlue level percentage between 0.0-1.0
*/
public Property getAdBlueLevel() {
return adBlueLevel;
}
/**
* @return The distance driven since reset
*/
public Property getDistanceSinceReset() {
return distanceSinceReset;
}
/**
* @return The distance driven since trip start
*/
public Property getDistanceSinceStart() {
return distanceSinceStart;
}
/**
* @return The fuel volume measured in liters
*/
public Property getFuelVolume() {
return fuelVolume;
}
/**
* @return The anti lock braking
*/
public Property getAntiLockBraking() {
return antiLockBraking;
}
/**
* @return Engine coolant temperature
*/
public Property getEngineCoolantTemperature() {
return engineCoolantTemperature;
}
/**
* @return The accumulated time of engine operation
* @deprecated removed the unit from the name. Replaced by {@link #getEngineTotalOperatingTime()}
*/
@Deprecated
public Property getEngineTotalOperatingHours() {
return engineTotalOperatingHours;
}
/**
* @return The accumulated lifespan fuel consumption
*/
public Property getEngineTotalFuelConsumption() {
return engineTotalFuelConsumption;
}
/**
* @return The brake fluid level
*/
public Property getBrakeFluidLevel() {
return brakeFluidLevel;
}
/**
* @return Current engine torque percentage between 0.0-1.0
*/
public Property getEngineTorque() {
return engineTorque;
}
/**
* @return Current engine load percentage between 0.0-1.0
*/
public Property getEngineLoad() {
return engineLoad;
}
/**
* @return The vehicle speed measured at the wheel base
*/
public Property getWheelBasedSpeed() {
return wheelBasedSpeed;
}
/**
* @return Battery level in %, value between 0.0 and 1.0
*/
public Property getBatteryLevel() {
return batteryLevel;
}
/**
* @return The check control messages
*/
public List> getCheckControlMessages() {
return checkControlMessages;
}
/**
* @return The tire pressures
*/
public List> getTirePressures() {
return tirePressures;
}
/**
* @return The tire temperatures
*/
public List> getTireTemperatures() {
return tireTemperatures;
}
/**
* @return The wheel rpms
*/
public List> getWheelRPMs() {
return wheelRPMs;
}
/**
* @return The trouble codes
*/
public List> getTroubleCodes() {
return troubleCodes;
}
/**
* @return The vehicle mileage (odometer) in meters
* @deprecated 'mileage' is an incorrect term for this. Replaced by {@link #getOdometer()}
*/
@Deprecated
public Property getMileageMeters() {
return mileageMeters;
}
/**
* @return The vehicle odometer value in a given units.
*/
public Property getOdometer() {
return odometer;
}
/**
* @return The accumulated time of engine operation
*/
public Property getEngineTotalOperatingTime() {
return engineTotalOperatingTime;
}
/**
* @return The tire pressure statuses
*/
public List> getTirePressureStatuses() {
return tirePressureStatuses;
}
/**
* @return Status of brake lining wear pre-warning
*/
public Property getBrakeLiningWearPreWarning() {
return brakeLiningWearPreWarning;
}
/**
* @return Remaining life of engine oil which decreases over time
*/
public Property getEngineOilLifeRemaining() {
return engineOilLifeRemaining;
}
/**
* @return Additional OEM trouble codes
*/
public List> getOemTroubleCodeValues() {
return oemTroubleCodeValues;
}
/**
* @return Distance remaining until diesel exhaust fluid is empty
*/
public Property getDieselExhaustFluidRange() {
return dieselExhaustFluidRange;
}
/**
* @return Level of soot in diesel exhaust particulate filter
*/
public Property getDieselParticulateFilterSootLevel() {
return dieselParticulateFilterSootLevel;
}
/**
* @return The confirmed trouble codes
*/
public List> getConfirmedTroubleCodes() {
return confirmedTroubleCodes;
}
/**
* @return The diesel exhaust filter status
*/
public Property getDieselExhaustFilterStatus() {
return dieselExhaustFilterStatus;
}
State(byte[] bytes) throws CommandParseException {
super(bytes);
final ArrayList> checkControlMessagesBuilder = new ArrayList<>();
final ArrayList> tirePressuresBuilder = new ArrayList<>();
final ArrayList> tireTemperaturesBuilder = new ArrayList<>();
final ArrayList> wheelRPMsBuilder = new ArrayList<>();
final ArrayList> troubleCodesBuilder = new ArrayList<>();
final ArrayList> tirePressureStatusesBuilder = new ArrayList<>();
final ArrayList> oemTroubleCodeValuesBuilder = new ArrayList<>();
final ArrayList> confirmedTroubleCodesBuilder = new ArrayList<>();
while (propertyIterator.hasNext()) {
propertyIterator.parseNext(p -> {
switch (p.getPropertyIdentifier()) {
case PROPERTY_MILEAGE: return mileage.update(p);
case PROPERTY_ENGINE_OIL_TEMPERATURE: return engineOilTemperature.update(p);
case PROPERTY_SPEED: return speed.update(p);
case PROPERTY_ENGINE_RPM: return engineRPM.update(p);
case PROPERTY_FUEL_LEVEL: return fuelLevel.update(p);
case PROPERTY_ESTIMATED_RANGE: return estimatedRange.update(p);
case PROPERTY_WASHER_FLUID_LEVEL: return washerFluidLevel.update(p);
case PROPERTY_BATTERY_VOLTAGE: return batteryVoltage.update(p);
case PROPERTY_ADBLUE_LEVEL: return adBlueLevel.update(p);
case PROPERTY_DISTANCE_SINCE_RESET: return distanceSinceReset.update(p);
case PROPERTY_DISTANCE_SINCE_START: return distanceSinceStart.update(p);
case PROPERTY_FUEL_VOLUME: return fuelVolume.update(p);
case PROPERTY_ANTI_LOCK_BRAKING: return antiLockBraking.update(p);
case PROPERTY_ENGINE_COOLANT_TEMPERATURE: return engineCoolantTemperature.update(p);
case PROPERTY_ENGINE_TOTAL_OPERATING_HOURS: return engineTotalOperatingHours.update(p);
case PROPERTY_ENGINE_TOTAL_FUEL_CONSUMPTION: return engineTotalFuelConsumption.update(p);
case PROPERTY_BRAKE_FLUID_LEVEL: return brakeFluidLevel.update(p);
case PROPERTY_ENGINE_TORQUE: return engineTorque.update(p);
case PROPERTY_ENGINE_LOAD: return engineLoad.update(p);
case PROPERTY_WHEEL_BASED_SPEED: return wheelBasedSpeed.update(p);
case PROPERTY_BATTERY_LEVEL: return batteryLevel.update(p);
case PROPERTY_CHECK_CONTROL_MESSAGES:
Property checkControlMessage = new Property<>(CheckControlMessage.class, p);
checkControlMessagesBuilder.add(checkControlMessage);
return checkControlMessage;
case PROPERTY_TIRE_PRESSURES:
Property tirePressure = new Property<>(TirePressure.class, p);
tirePressuresBuilder.add(tirePressure);
return tirePressure;
case PROPERTY_TIRE_TEMPERATURES:
Property tireTemperature = new Property<>(TireTemperature.class, p);
tireTemperaturesBuilder.add(tireTemperature);
return tireTemperature;
case PROPERTY_WHEEL_RPMS:
Property wheelRpm = new Property<>(WheelRpm.class, p);
wheelRPMsBuilder.add(wheelRpm);
return wheelRpm;
case PROPERTY_TROUBLE_CODES:
Property troubleCode = new Property<>(TroubleCode.class, p);
troubleCodesBuilder.add(troubleCode);
return troubleCode;
case PROPERTY_MILEAGE_METERS: return mileageMeters.update(p);
case PROPERTY_ODOMETER: return odometer.update(p);
case PROPERTY_ENGINE_TOTAL_OPERATING_TIME: return engineTotalOperatingTime.update(p);
case PROPERTY_TIRE_PRESSURE_STATUSES:
Property tirePressureStatus = new Property<>(TirePressureStatus.class, p);
tirePressureStatusesBuilder.add(tirePressureStatus);
return tirePressureStatus;
case PROPERTY_BRAKE_LINING_WEAR_PRE_WARNING: return brakeLiningWearPreWarning.update(p);
case PROPERTY_ENGINE_OIL_LIFE_REMAINING: return engineOilLifeRemaining.update(p);
case PROPERTY_OEM_TROUBLE_CODE_VALUES:
Property oemTroubleCodeValue = new Property<>(OemTroubleCodeValue.class, p);
oemTroubleCodeValuesBuilder.add(oemTroubleCodeValue);
return oemTroubleCodeValue;
case PROPERTY_DIESEL_EXHAUST_FLUID_RANGE: return dieselExhaustFluidRange.update(p);
case PROPERTY_DIESEL_PARTICULATE_FILTER_SOOT_LEVEL: return dieselParticulateFilterSootLevel.update(p);
case PROPERTY_CONFIRMED_TROUBLE_CODES:
Property confirmedTroubleCode = new Property<>(ConfirmedTroubleCode.class, p);
confirmedTroubleCodesBuilder.add(confirmedTroubleCode);
return confirmedTroubleCode;
case PROPERTY_DIESEL_EXHAUST_FILTER_STATUS: return dieselExhaustFilterStatus.update(p);
}
return null;
});
}
checkControlMessages = checkControlMessagesBuilder;
tirePressures = tirePressuresBuilder;
tireTemperatures = tireTemperaturesBuilder;
wheelRPMs = wheelRPMsBuilder;
troubleCodes = troubleCodesBuilder;
tirePressureStatuses = tirePressureStatusesBuilder;
oemTroubleCodeValues = oemTroubleCodeValuesBuilder;
confirmedTroubleCodes = confirmedTroubleCodesBuilder;
}
private State(Builder builder) {
super(builder);
mileage = builder.mileage;
engineOilTemperature = builder.engineOilTemperature;
speed = builder.speed;
engineRPM = builder.engineRPM;
fuelLevel = builder.fuelLevel;
estimatedRange = builder.estimatedRange;
washerFluidLevel = builder.washerFluidLevel;
batteryVoltage = builder.batteryVoltage;
adBlueLevel = builder.adBlueLevel;
distanceSinceReset = builder.distanceSinceReset;
distanceSinceStart = builder.distanceSinceStart;
fuelVolume = builder.fuelVolume;
antiLockBraking = builder.antiLockBraking;
engineCoolantTemperature = builder.engineCoolantTemperature;
engineTotalOperatingHours = builder.engineTotalOperatingHours;
engineTotalFuelConsumption = builder.engineTotalFuelConsumption;
brakeFluidLevel = builder.brakeFluidLevel;
engineTorque = builder.engineTorque;
engineLoad = builder.engineLoad;
wheelBasedSpeed = builder.wheelBasedSpeed;
batteryLevel = builder.batteryLevel;
checkControlMessages = builder.checkControlMessages;
tirePressures = builder.tirePressures;
tireTemperatures = builder.tireTemperatures;
wheelRPMs = builder.wheelRPMs;
troubleCodes = builder.troubleCodes;
mileageMeters = builder.mileageMeters;
odometer = builder.odometer;
engineTotalOperatingTime = builder.engineTotalOperatingTime;
tirePressureStatuses = builder.tirePressureStatuses;
brakeLiningWearPreWarning = builder.brakeLiningWearPreWarning;
engineOilLifeRemaining = builder.engineOilLifeRemaining;
oemTroubleCodeValues = builder.oemTroubleCodeValues;
dieselExhaustFluidRange = builder.dieselExhaustFluidRange;
dieselParticulateFilterSootLevel = builder.dieselParticulateFilterSootLevel;
confirmedTroubleCodes = builder.confirmedTroubleCodes;
dieselExhaustFilterStatus = builder.dieselExhaustFilterStatus;
}
public static final class Builder extends SetCommand.Builder {
private Property mileage;
private Property engineOilTemperature;
private Property speed;
private Property engineRPM;
private Property fuelLevel;
private Property estimatedRange;
private Property washerFluidLevel;
private Property batteryVoltage;
private Property adBlueLevel;
private Property distanceSinceReset;
private Property distanceSinceStart;
private Property fuelVolume;
private Property antiLockBraking;
private Property engineCoolantTemperature;
private Property engineTotalOperatingHours;
private Property engineTotalFuelConsumption;
private Property brakeFluidLevel;
private Property engineTorque;
private Property engineLoad;
private Property wheelBasedSpeed;
private Property batteryLevel;
private final List> checkControlMessages = new ArrayList<>();
private final List> tirePressures = new ArrayList<>();
private final List> tireTemperatures = new ArrayList<>();
private final List> wheelRPMs = new ArrayList<>();
private final List> troubleCodes = new ArrayList<>();
private Property mileageMeters;
private Property odometer;
private Property engineTotalOperatingTime;
private final List> tirePressureStatuses = new ArrayList<>();
private Property brakeLiningWearPreWarning;
private Property engineOilLifeRemaining;
private final List> oemTroubleCodeValues = new ArrayList<>();
private Property dieselExhaustFluidRange;
private Property dieselParticulateFilterSootLevel;
private final List> confirmedTroubleCodes = new ArrayList<>();
private Property dieselExhaustFilterStatus;
public Builder() {
super(IDENTIFIER);
}
public State build() {
return new State(this);
}
/**
* @param mileage The vehicle mileage (odometer)
* @return The builder
* @deprecated 'mileage' is an incorrect term for this. Replaced by {@link #getOdometer()}
*/
@Deprecated
public Builder setMileage(Property mileage) {
this.mileage = mileage.setIdentifier(PROPERTY_MILEAGE);
addProperty(this.mileage);
return this;
}
/**
* @param engineOilTemperature Engine oil temperature
* @return The builder
*/
public Builder setEngineOilTemperature(Property engineOilTemperature) {
this.engineOilTemperature = engineOilTemperature.setIdentifier(PROPERTY_ENGINE_OIL_TEMPERATURE);
addProperty(this.engineOilTemperature);
return this;
}
/**
* @param speed The vehicle speed
* @return The builder
*/
public Builder setSpeed(Property speed) {
this.speed = speed.setIdentifier(PROPERTY_SPEED);
addProperty(this.speed);
return this;
}
/**
* @param engineRPM Engine RPM (revolutions per minute)
* @return The builder
*/
public Builder setEngineRPM(Property engineRPM) {
this.engineRPM = engineRPM.setIdentifier(PROPERTY_ENGINE_RPM);
addProperty(this.engineRPM);
return this;
}
/**
* @param fuelLevel Fuel level percentage between 0.0-1.0
* @return The builder
*/
public Builder setFuelLevel(Property fuelLevel) {
this.fuelLevel = fuelLevel.setIdentifier(PROPERTY_FUEL_LEVEL);
addProperty(this.fuelLevel);
return this;
}
/**
* @param estimatedRange Estimated range (with combustion engine)
* @return The builder
*/
public Builder setEstimatedRange(Property estimatedRange) {
this.estimatedRange = estimatedRange.setIdentifier(PROPERTY_ESTIMATED_RANGE);
addProperty(this.estimatedRange);
return this;
}
/**
* @param washerFluidLevel The washer fluid level
* @return The builder
*/
public Builder setWasherFluidLevel(Property washerFluidLevel) {
this.washerFluidLevel = washerFluidLevel.setIdentifier(PROPERTY_WASHER_FLUID_LEVEL);
addProperty(this.washerFluidLevel);
return this;
}
/**
* @param batteryVoltage Battery voltage
* @return The builder
*/
public Builder setBatteryVoltage(Property batteryVoltage) {
this.batteryVoltage = batteryVoltage.setIdentifier(PROPERTY_BATTERY_VOLTAGE);
addProperty(this.batteryVoltage);
return this;
}
/**
* @param adBlueLevel AdBlue level percentage between 0.0-1.0
* @return The builder
*/
public Builder setAdBlueLevel(Property adBlueLevel) {
this.adBlueLevel = adBlueLevel.setIdentifier(PROPERTY_ADBLUE_LEVEL);
addProperty(this.adBlueLevel);
return this;
}
/**
* @param distanceSinceReset The distance driven since reset
* @return The builder
*/
public Builder setDistanceSinceReset(Property distanceSinceReset) {
this.distanceSinceReset = distanceSinceReset.setIdentifier(PROPERTY_DISTANCE_SINCE_RESET);
addProperty(this.distanceSinceReset);
return this;
}
/**
* @param distanceSinceStart The distance driven since trip start
* @return The builder
*/
public Builder setDistanceSinceStart(Property distanceSinceStart) {
this.distanceSinceStart = distanceSinceStart.setIdentifier(PROPERTY_DISTANCE_SINCE_START);
addProperty(this.distanceSinceStart);
return this;
}
/**
* @param fuelVolume The fuel volume measured in liters
* @return The builder
*/
public Builder setFuelVolume(Property fuelVolume) {
this.fuelVolume = fuelVolume.setIdentifier(PROPERTY_FUEL_VOLUME);
addProperty(this.fuelVolume);
return this;
}
/**
* @param antiLockBraking The anti lock braking
* @return The builder
*/
public Builder setAntiLockBraking(Property antiLockBraking) {
this.antiLockBraking = antiLockBraking.setIdentifier(PROPERTY_ANTI_LOCK_BRAKING);
addProperty(this.antiLockBraking);
return this;
}
/**
* @param engineCoolantTemperature Engine coolant temperature
* @return The builder
*/
public Builder setEngineCoolantTemperature(Property engineCoolantTemperature) {
this.engineCoolantTemperature = engineCoolantTemperature.setIdentifier(PROPERTY_ENGINE_COOLANT_TEMPERATURE);
addProperty(this.engineCoolantTemperature);
return this;
}
/**
* @param engineTotalOperatingHours The accumulated time of engine operation
* @return The builder
* @deprecated removed the unit from the name. Replaced by {@link #getEngineTotalOperatingTime()}
*/
@Deprecated
public Builder setEngineTotalOperatingHours(Property engineTotalOperatingHours) {
this.engineTotalOperatingHours = engineTotalOperatingHours.setIdentifier(PROPERTY_ENGINE_TOTAL_OPERATING_HOURS);
addProperty(this.engineTotalOperatingHours);
return this;
}
/**
* @param engineTotalFuelConsumption The accumulated lifespan fuel consumption
* @return The builder
*/
public Builder setEngineTotalFuelConsumption(Property engineTotalFuelConsumption) {
this.engineTotalFuelConsumption = engineTotalFuelConsumption.setIdentifier(PROPERTY_ENGINE_TOTAL_FUEL_CONSUMPTION);
addProperty(this.engineTotalFuelConsumption);
return this;
}
/**
* @param brakeFluidLevel The brake fluid level
* @return The builder
*/
public Builder setBrakeFluidLevel(Property brakeFluidLevel) {
this.brakeFluidLevel = brakeFluidLevel.setIdentifier(PROPERTY_BRAKE_FLUID_LEVEL);
addProperty(this.brakeFluidLevel);
return this;
}
/**
* @param engineTorque Current engine torque percentage between 0.0-1.0
* @return The builder
*/
public Builder setEngineTorque(Property engineTorque) {
this.engineTorque = engineTorque.setIdentifier(PROPERTY_ENGINE_TORQUE);
addProperty(this.engineTorque);
return this;
}
/**
* @param engineLoad Current engine load percentage between 0.0-1.0
* @return The builder
*/
public Builder setEngineLoad(Property engineLoad) {
this.engineLoad = engineLoad.setIdentifier(PROPERTY_ENGINE_LOAD);
addProperty(this.engineLoad);
return this;
}
/**
* @param wheelBasedSpeed The vehicle speed measured at the wheel base
* @return The builder
*/
public Builder setWheelBasedSpeed(Property wheelBasedSpeed) {
this.wheelBasedSpeed = wheelBasedSpeed.setIdentifier(PROPERTY_WHEEL_BASED_SPEED);
addProperty(this.wheelBasedSpeed);
return this;
}
/**
* @param batteryLevel Battery level in %, value between 0.0 and 1.0
* @return The builder
*/
public Builder setBatteryLevel(Property batteryLevel) {
this.batteryLevel = batteryLevel.setIdentifier(PROPERTY_BATTERY_LEVEL);
addProperty(this.batteryLevel);
return this;
}
/**
* Add an array of check control messages.
*
* @param checkControlMessages The check control messages
* @return The builder
*/
public Builder setCheckControlMessages(Property[] checkControlMessages) {
this.checkControlMessages.clear();
for (int i = 0; i < checkControlMessages.length; i++) {
addCheckControlMessage(checkControlMessages[i]);
}
return this;
}
/**
* Add a single check control message.
*
* @param checkControlMessage The check control message
* @return The builder
*/
public Builder addCheckControlMessage(Property checkControlMessage) {
checkControlMessage.setIdentifier(PROPERTY_CHECK_CONTROL_MESSAGES);
addProperty(checkControlMessage);
checkControlMessages.add(checkControlMessage);
return this;
}
/**
* Add an array of tire pressures.
*
* @param tirePressures The tire pressures
* @return The builder
*/
public Builder setTirePressures(Property[] tirePressures) {
this.tirePressures.clear();
for (int i = 0; i < tirePressures.length; i++) {
addTirePressure(tirePressures[i]);
}
return this;
}
/**
* Add a single tire pressure.
*
* @param tirePressure The tire pressure
* @return The builder
*/
public Builder addTirePressure(Property tirePressure) {
tirePressure.setIdentifier(PROPERTY_TIRE_PRESSURES);
addProperty(tirePressure);
tirePressures.add(tirePressure);
return this;
}
/**
* Add an array of tire temperatures.
*
* @param tireTemperatures The tire temperatures
* @return The builder
*/
public Builder setTireTemperatures(Property[] tireTemperatures) {
this.tireTemperatures.clear();
for (int i = 0; i < tireTemperatures.length; i++) {
addTireTemperature(tireTemperatures[i]);
}
return this;
}
/**
* Add a single tire temperature.
*
* @param tireTemperature The tire temperature
* @return The builder
*/
public Builder addTireTemperature(Property tireTemperature) {
tireTemperature.setIdentifier(PROPERTY_TIRE_TEMPERATURES);
addProperty(tireTemperature);
tireTemperatures.add(tireTemperature);
return this;
}
/**
* Add an array of wheel rpms.
*
* @param wheelRPMs The wheel rpms
* @return The builder
*/
public Builder setWheelRPMs(Property[] wheelRPMs) {
this.wheelRPMs.clear();
for (int i = 0; i < wheelRPMs.length; i++) {
addWheelRpm(wheelRPMs[i]);
}
return this;
}
/**
* Add a single wheel rpm.
*
* @param wheelRpm The wheel rpm
* @return The builder
*/
public Builder addWheelRpm(Property wheelRpm) {
wheelRpm.setIdentifier(PROPERTY_WHEEL_RPMS);
addProperty(wheelRpm);
wheelRPMs.add(wheelRpm);
return this;
}
/**
* Add an array of trouble codes.
*
* @param troubleCodes The trouble codes
* @return The builder
*/
public Builder setTroubleCodes(Property[] troubleCodes) {
this.troubleCodes.clear();
for (int i = 0; i < troubleCodes.length; i++) {
addTroubleCode(troubleCodes[i]);
}
return this;
}
/**
* Add a single trouble code.
*
* @param troubleCode The trouble code
* @return The builder
*/
public Builder addTroubleCode(Property troubleCode) {
troubleCode.setIdentifier(PROPERTY_TROUBLE_CODES);
addProperty(troubleCode);
troubleCodes.add(troubleCode);
return this;
}
/**
* @param mileageMeters The vehicle mileage (odometer) in meters
* @return The builder
* @deprecated 'mileage' is an incorrect term for this. Replaced by {@link #getOdometer()}
*/
@Deprecated
public Builder setMileageMeters(Property mileageMeters) {
this.mileageMeters = mileageMeters.setIdentifier(PROPERTY_MILEAGE_METERS);
addProperty(this.mileageMeters);
return this;
}
/**
* @param odometer The vehicle odometer value in a given units.
* @return The builder
*/
public Builder setOdometer(Property odometer) {
this.odometer = odometer.setIdentifier(PROPERTY_ODOMETER);
addProperty(this.odometer);
return this;
}
/**
* @param engineTotalOperatingTime The accumulated time of engine operation
* @return The builder
*/
public Builder setEngineTotalOperatingTime(Property engineTotalOperatingTime) {
this.engineTotalOperatingTime = engineTotalOperatingTime.setIdentifier(PROPERTY_ENGINE_TOTAL_OPERATING_TIME);
addProperty(this.engineTotalOperatingTime);
return this;
}
/**
* Add an array of tire pressure statuses.
*
* @param tirePressureStatuses The tire pressure statuses
* @return The builder
*/
public Builder setTirePressureStatuses(Property[] tirePressureStatuses) {
this.tirePressureStatuses.clear();
for (int i = 0; i < tirePressureStatuses.length; i++) {
addTirePressureStatus(tirePressureStatuses[i]);
}
return this;
}
/**
* Add a single tire pressure status.
*
* @param tirePressureStatus The tire pressure status
* @return The builder
*/
public Builder addTirePressureStatus(Property tirePressureStatus) {
tirePressureStatus.setIdentifier(PROPERTY_TIRE_PRESSURE_STATUSES);
addProperty(tirePressureStatus);
tirePressureStatuses.add(tirePressureStatus);
return this;
}
/**
* @param brakeLiningWearPreWarning Status of brake lining wear pre-warning
* @return The builder
*/
public Builder setBrakeLiningWearPreWarning(Property brakeLiningWearPreWarning) {
this.brakeLiningWearPreWarning = brakeLiningWearPreWarning.setIdentifier(PROPERTY_BRAKE_LINING_WEAR_PRE_WARNING);
addProperty(this.brakeLiningWearPreWarning);
return this;
}
/**
* @param engineOilLifeRemaining Remaining life of engine oil which decreases over time
* @return The builder
*/
public Builder setEngineOilLifeRemaining(Property engineOilLifeRemaining) {
this.engineOilLifeRemaining = engineOilLifeRemaining.setIdentifier(PROPERTY_ENGINE_OIL_LIFE_REMAINING);
addProperty(this.engineOilLifeRemaining);
return this;
}
/**
* Add an array of oem trouble code values.
*
* @param oemTroubleCodeValues The oem trouble code values. Additional OEM trouble codes
* @return The builder
*/
public Builder setOemTroubleCodeValues(Property[] oemTroubleCodeValues) {
this.oemTroubleCodeValues.clear();
for (int i = 0; i < oemTroubleCodeValues.length; i++) {
addOemTroubleCodeValue(oemTroubleCodeValues[i]);
}
return this;
}
/**
* Add a single oem trouble code value.
*
* @param oemTroubleCodeValue The oem trouble code value. Additional OEM trouble codes
* @return The builder
*/
public Builder addOemTroubleCodeValue(Property oemTroubleCodeValue) {
oemTroubleCodeValue.setIdentifier(PROPERTY_OEM_TROUBLE_CODE_VALUES);
addProperty(oemTroubleCodeValue);
oemTroubleCodeValues.add(oemTroubleCodeValue);
return this;
}
/**
* @param dieselExhaustFluidRange Distance remaining until diesel exhaust fluid is empty
* @return The builder
*/
public Builder setDieselExhaustFluidRange(Property dieselExhaustFluidRange) {
this.dieselExhaustFluidRange = dieselExhaustFluidRange.setIdentifier(PROPERTY_DIESEL_EXHAUST_FLUID_RANGE);
addProperty(this.dieselExhaustFluidRange);
return this;
}
/**
* @param dieselParticulateFilterSootLevel Level of soot in diesel exhaust particulate filter
* @return The builder
*/
public Builder setDieselParticulateFilterSootLevel(Property dieselParticulateFilterSootLevel) {
this.dieselParticulateFilterSootLevel = dieselParticulateFilterSootLevel.setIdentifier(PROPERTY_DIESEL_PARTICULATE_FILTER_SOOT_LEVEL);
addProperty(this.dieselParticulateFilterSootLevel);
return this;
}
/**
* Add an array of confirmed trouble codes.
*
* @param confirmedTroubleCodes The confirmed trouble codes
* @return The builder
*/
public Builder setConfirmedTroubleCodes(Property[] confirmedTroubleCodes) {
this.confirmedTroubleCodes.clear();
for (int i = 0; i < confirmedTroubleCodes.length; i++) {
addConfirmedTroubleCode(confirmedTroubleCodes[i]);
}
return this;
}
/**
* Add a single confirmed trouble code.
*
* @param confirmedTroubleCode The confirmed trouble code
* @return The builder
*/
public Builder addConfirmedTroubleCode(Property confirmedTroubleCode) {
confirmedTroubleCode.setIdentifier(PROPERTY_CONFIRMED_TROUBLE_CODES);
addProperty(confirmedTroubleCode);
confirmedTroubleCodes.add(confirmedTroubleCode);
return this;
}
/**
* @param dieselExhaustFilterStatus The diesel exhaust filter status
* @return The builder
*/
public Builder setDieselExhaustFilterStatus(Property dieselExhaustFilterStatus) {
this.dieselExhaustFilterStatus = dieselExhaustFilterStatus.setIdentifier(PROPERTY_DIESEL_EXHAUST_FILTER_STATUS);
addProperty(this.dieselExhaustFilterStatus);
return this;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy