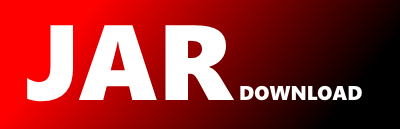
com.hivemq.persistence.clientsession.ClientSessionPersistence Maven / Gradle / Ivy
Show all versions of hivemq-community-edition-embedded Show documentation
/*
* Copyright 2019-present HiveMQ GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hivemq.persistence.clientsession;
import com.google.common.util.concurrent.ListenableFuture;
import com.hivemq.codec.encoder.mqtt5.UnsignedDataTypes;
import com.hivemq.extension.sdk.api.annotations.NotNull;
import com.hivemq.extension.sdk.api.annotations.Nullable;
import com.hivemq.extensions.iteration.ChunkCursor;
import com.hivemq.extensions.iteration.MultipleChunkResult;
import com.hivemq.mqtt.message.connect.MqttWillPublish;
import com.hivemq.mqtt.message.reason.Mqtt5DisconnectReasonCode;
import java.util.Map;
import java.util.Set;
import static com.hivemq.persistence.clientsession.ClientSessionPersistenceImpl.DisconnectSource;
/**
* @author Lukas Brandl
*/
public interface ClientSessionPersistence {
boolean isExistent(@NotNull String client);
@NotNull ListenableFuture clientDisconnected(@NotNull String client, boolean sendWill, long sessionExpiry);
/**
* Mark the client session as connected
*
* @param client The client ID
* @param cleanStart clean start or use previous session
* @param sessionExpiryInterval The session expiry interval for this client
* @param queueLimit for this session specifically
* @return The state of incomplete outgoing message transmissions for this client
*/
@NotNull ListenableFuture clientConnected(
@NotNull String client,
boolean cleanStart,
long sessionExpiryInterval,
@Nullable MqttWillPublish willPublish,
@Nullable Long queueLimit);
/**
* Close the persistence.
*
* @return a future which completes as soon as the persistence is closed.
*/
@NotNull ListenableFuture closeDB();
/**
* Get a client session for a specific identifier.
*
* @param clientId the client id.
* @param includeWill if the Will message should be included, the will is set to {@code null} if this parameter
* is {@code false}.
* @return the client session for this identifier or {@code null}.
*/
@Nullable ClientSession getSession(@NotNull String clientId, boolean includeWill);
/**
* Trigger a cleanup for a specific bucket
*
* @param bucketIndex the index of the bucket
* @return a future which completes as soon as the clean up is done.
*/
@NotNull ListenableFuture cleanUp(int bucketIndex);
/**
* @return a future of all client ids in the persistence.
*/
@NotNull ListenableFuture> getAllClients();
/**
* Enforce a client disconnect from a specific source, preventing or delivering the will message.
*
* @param clientId The client id of the client to disconnect.
* @param preventLwtMessage The flag if the will message should be prevented or delivered.
* @param source The source of the enforce call.
* @return a future of a boolean which gives the information that a client was disconnected (true) or wasn't
* connected (false).
*/
@NotNull ListenableFuture forceDisconnectClient(
@NotNull String clientId, boolean preventLwtMessage, @NotNull DisconnectSource source);
/**
* Enforce a client disconnect from a specific source, preventing or delivering the will message.
*
* @param clientId The client id of the client to disconnect.
* @param preventLwtMessage The flag if the will message should be prevented or delivered.
* @param source The source of the enforce call.
* @param reasonCode The reason code for the enforced disconnect.
* @param reasonString The reason string for the enforced disconnect.
* @return a future of a boolean which gives the information that a client was disconnected (true) or wasn't
* connected (false).
*/
@NotNull ListenableFuture forceDisconnectClient(
@NotNull String clientId,
boolean preventLwtMessage,
@NotNull DisconnectSource source,
@Nullable Mqtt5DisconnectReasonCode reasonCode,
@Nullable String reasonString);
/**
* Returns the session expiry interval for a client in seconds.
*
* {@link UnsignedDataTypes#UNSIGNED_INT_MAX_VALUE} = max value
*
* 0 = Session expires on disconnect
*
* @param clientId the client identifier of the client
* @return the {@link Integer} Session expiry interval in seconds.
*/
@Nullable Long getSessionExpiryInterval(@NotNull String clientId);
/**
* Sets the session expiry interval for a client in seconds.
*
* @param clientId the client identifier of the client
* @param sessionExpiryInterval session expiry interval for the client in seconds
* @return a {@link com.google.common.util.concurrent.ListenableFuture} which is returned when the Session Expiry
* Interval is set
* successfully or a Exception was caught.
*/
@NotNull ListenableFuture setSessionExpiryInterval(@NotNull String clientId, long sessionExpiryInterval);
/**
* Checks if there is a session for a given amount of clients.
*
* @param clients to check
* @return The client id mapped to a boolean that is true if the session exists
*/
@NotNull Map isExistent(@NotNull Set clients);
/**
* Invalidates the client session for a client with the given client identifier. If the client is currently
* connected, it will be disconnected as well.
*
* Sets session expiry interval to 0. Attention: The given Session expiry interval of 0 may be overwritten at client
* reconnection.
*
* @param clientId The client identifier of the client which session should be invalidated
* @param disconnectSource The source of the invalidate session call.
* @return a {@link com.google.common.util.concurrent.ListenableFuture} succeeding with a {@link Boolean} which is
* true when the client has been actively disconnected by the broker otherwise false,
* @since 3.4
*/
@NotNull ListenableFuture invalidateSession(
@NotNull String clientId, @NotNull DisconnectSource disconnectSource);
/**
* @return The delay of all will messages that have not been sent yet, mapped to the client id.
*/
@NotNull ListenableFuture