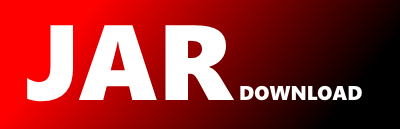
com.hmsonline.storm.cassandra.bolt.CassandraBolt Maven / Gradle / Ivy
package com.hmsonline.storm.cassandra.bolt;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import backtype.storm.task.TopologyContext;
import backtype.storm.tuple.Tuple;
import com.hmsonline.storm.cassandra.bolt.mapper.TupleCounterMapper;
import com.hmsonline.storm.cassandra.bolt.mapper.TupleMapper;
import com.hmsonline.storm.cassandra.client.AstyanaxClient;
@SuppressWarnings("serial")
public abstract class CassandraBolt implements Serializable {
private static final Logger LOG = LoggerFactory.getLogger(CassandraBolt.class);
// private Class columnNameClass;
// private Class columnValueClass;
private String clientConfigKey;
protected AstyanaxClient client;
protected TupleMapper tupleMapper;
protected Map stormConfig;
public CassandraBolt(String clientConfigKey, TupleMapper tupleMapper) {
this.tupleMapper = tupleMapper;
// this.columnNameClass = columnNameClass;
// this.columnValueClass = columnValueClass;
this.clientConfigKey = clientConfigKey;
LOG.debug("Creating Cassandra Bolt (" + this + ")");
}
@SuppressWarnings("unchecked")
public void prepare(Map stormConf, TopologyContext context) {
Map config = (Map) stormConf.get(this.clientConfigKey);
this.client = new AstyanaxClient();
this.client.start(config);
}
public void cleanup() {
this.client.stop();
}
public void writeTuple(Tuple input, TupleMapper tupleMapper) throws Exception {
this.client.writeTuple(input, tupleMapper);
}
public void writeTuples(List inputs, TupleMapper tupleMapper) throws Exception {
this.client.writeTuples(inputs, tupleMapper);
}
public Map getComponentConfiguration() {
return null;
}
public void incrementCounter(Tuple input, TupleCounterMapper tupleMapper) throws Exception {
this.client.incrementCountColumn(input, tupleMapper);
}
public void incrementCounters(List inputs, TupleCounterMapper tupleMapper) throws Exception {
this.client.incrementCountColumns(inputs, tupleMapper);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy