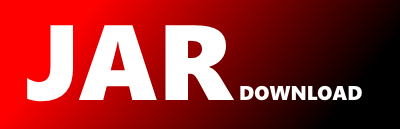
com.hmsonline.storm.cassandra.bolt.mapper.TridentTupleMapper Maven / Gradle / Ivy
package com.hmsonline.storm.cassandra.bolt.mapper;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import storm.trident.tuple.TridentTuple;
import com.hmsonline.storm.cassandra.exceptions.TupleMappingException;
/**
* Interface for mapping Trident storm.trident.tuple.TridentTuple
* objects to Cassandra rows.
*
* @author tgoetz
*
* @param - the Cassandra row key type
* @param - the Cassandra column key/name type
* @param - the Cassandra column value type
*/
/* TODO tgoetz: This interface is suffering from serious growing pains,
* which is a good indication that the API needs to be rethought.
*/
public interface TridentTupleMapper extends Serializable {
/**
* Given a storm.trident.tuple.TridentTuple
object,
* return the name of the column family that data should be
* written to.
*
* @param tuple
* @return the column family name
*/
String mapToColumnFamily(TridentTuple tuple) throws TupleMappingException;
/**
* Given a backtype.storm.tuple.Tuple
object, map the keyspace to write to.
*
* @param tuple
* @return
*/
String mapToKeyspace(TridentTuple tuple);
/**
* Given a storm.trident.tuple.TridentTuple
object,
* return an object representing the Cassandra row key.
*
* @param tuple
* @return
* @throws TupleMappingException
*/
K mapToRowKey(TridentTuple tuple) throws TupleMappingException;
/**
* Given a storm.trident.tuple.TridentTuple
object,
* return a java.util.Map
object representing the
* column names/values to persist to Cassandra.
*
* @param tuple
* @return
* @throws TupleMappingException
*/
Map mapToColumns(TridentTuple tuple) throws TupleMappingException;
/**
* Given a storm.trident.tuple.TridentTuple
object,
* return a list of column name (column key) objects in order to execute a
* slice query against a particular row.
*
* The row key will be determined by the return value of mapToRowKey()
.
*
*
* @param tuple
* @return
* @throws TupleMappingException
*/
List mapToColumnsForLookup(TridentTuple tuple) throws TupleMappingException;
/**
* Map a storm.trident.tuple.TridentTuple
object
* to a an end key in order to perform a Cassandra column range query.
*
* The row key will be determined by the return value of mapToRowKey()
.
*
* The start key/column will be determined by the value of mapToStartKey()
.
*
* @param tuple
* @return
* @throws TupleMappingException
*/
C mapToEndKey(TridentTuple tuple) throws TupleMappingException;
/**
*
* @param tuple
* @return
* @throws TupleMappingException
*/
C mapToStartKey(TridentTuple tuple) throws TupleMappingException;
boolean shouldDelete(TridentTuple tuple);
/**
* Returns the row key class.
*
* @return
*/
Class getKeyClass();
/**
* Returns the coulumn name(key) class.
*
* @return
*/
Class getColumnNameClass();
/**
* Returns the column value class.
*
* @return
*/
Class getColumnValueClass();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy