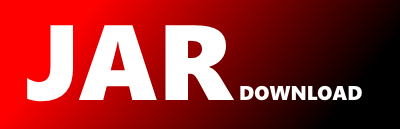
com.hmsonline.storm.cassandra.bolt.mapper.ValuelessColumnsMapper Maven / Gradle / Ivy
package com.hmsonline.storm.cassandra.bolt.mapper;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import backtype.storm.topology.OutputFieldsDeclarer;
import backtype.storm.tuple.Fields;
import backtype.storm.tuple.Tuple;
import backtype.storm.tuple.Values;
/**
* A mapper implementation that emits tuples based on a combination of cassandra
* rowkey, collumnkey, and delimiter.
*
* When this bolt receives a tuple, it will attempt the following:
*
* - Look up a value in the tuple using
rowKeyField
* - Fetch the corresponding row from cassandra
* - Fetch the column
columnKeyField
value from the row.
* - Split the column value into an array based on
delimiter
* - For each value, emit a tuple with
{emitIdFieldName}={value}
*
* For example, given the following cassandra row:
*
*
* RowKey: mike
* => (column=followers, value=john:bob, timestamp=1328848653055000)
*
*
* and the following bolt setup:
*
*
* rowKeyField = "rowKey"
* columnKeyField = "followers"
* delimiter = ":"
* emitIdFieldName = "rowKey"
* emitValueFieldName = "follower"
*
*
* if the following tuple were received by the bolt:
*
*
* {rowKey:mike}
*
*
* The following tuples would be emitted:
*
*
* {rowKey:mike, follower:john}
* {rowKey:mike, follower:bob}
*
*
* @author tgoetz
* @author boneill42
*/
@SuppressWarnings("serial")
public class ValuelessColumnsMapper implements ColumnMapper, Serializable {
private String emitFieldForRowKey;
private String emitFieldForColumnName;
private boolean isDrpc;
/**
* Constructs a ValuelessColumnsMapper
*
* @param emitFieldForRowKey
* This is the field name for the rowkey in the outbound tuple(s)
* @param emitFieldForColumnName
* This is the field name for column names in the outbound
* tuple(s)
* @param isDrpc
*/
public ValuelessColumnsMapper(String emitFieldForRowKey, String emitFieldForColumnName, boolean isDrpc) {
this.isDrpc = isDrpc;
this.emitFieldForRowKey = emitFieldForRowKey;
this.emitFieldForColumnName = emitFieldForColumnName;
}
/**
* Declares the fields produced by this bolt.
*
*
* @param declarer
*/
@Override
public void declareOutputFields(OutputFieldsDeclarer declarer) {
if (this.isDrpc) {
declarer.declare(new Fields("id", this.emitFieldForRowKey, this.emitFieldForColumnName));
} else {
declarer.declare(new Fields(this.emitFieldForRowKey, this.emitFieldForColumnName));
}
}
/**
* Given a set of columns, maps to values to emit.
*
* @param columns
* @return
*/
@Override
public List mapToValues(String rowKey, Map columns, Tuple input) {
List values = new ArrayList();
Set vals = columns.keySet();
for(String columnName : vals){
if(this.isDrpc){
values.add(new Values(input.getValue(0), rowKey, columnName));
} else {
values.add(new Values(rowKey, columnName));
}
}
return values;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy