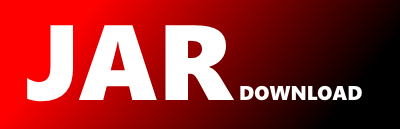
com.holonplatform.http.HttpHeaders Maven / Gradle / Ivy
/*
* Copyright 2016-2017 Axioma srl.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.holonplatform.http;
import java.net.URI;
import java.util.Date;
import java.util.List;
import java.util.Locale;
import java.util.Optional;
import com.holonplatform.core.internal.utils.ObjectUtils;
import com.holonplatform.core.messaging.MessageHeaders;
import com.holonplatform.http.internal.HttpUtils;
/**
* HTTP headers representation.
*
* @since 5.0.0
*/
public interface HttpHeaders extends MessageHeaders> {
/**
* The HTTP Accept
header field name.
* @see Section 5.3.2 of RFC 7231
*/
String ACCEPT = "Accept";
/**
* The HTTP Accept-Charset
header field name.
* @see Section 5.3.3 of RFC 7231
*/
String ACCEPT_CHARSET = "Accept-Charset";
/**
* The HTTP Accept-Encoding
header field name.
* @see Section 5.3.4 of RFC 7231
*/
String ACCEPT_ENCODING = "Accept-Encoding";
/**
* The HTTP Accept-Language
header field name.
* @see Section 5.3.5 of RFC 7231
*/
String ACCEPT_LANGUAGE = "Accept-Language";
/**
* The HTTP Accept-Ranges
header field name.
* @see Section 5.3.5 of RFC 7233
*/
String ACCEPT_RANGES = "Accept-Ranges";
/**
* The HTTP Age
header field name.
* @see Section 5.1 of RFC 7234
*/
String AGE = "Age";
/**
* The HTTP Allow
header field name.
* @see Section 7.4.1 of RFC 7231
*/
String ALLOW = "Allow";
/**
* The HTTP Authorization
header field name.
* @see Section 4.2 of RFC 7235
*/
String AUTHORIZATION = "Authorization";
/**
* The HTTP Cache-Control
header field name.
* @see Section 5.2 of RFC 7234
*/
String CACHE_CONTROL = "Cache-Control";
/**
* The HTTP Connection
header field name.
* @see Section 6.1 of RFC 7230
*/
String CONNECTION = "Connection";
/**
* The HTTP Content-Encoding
header field name.
* @see Section 3.1.2.2 of RFC 7231
*/
String CONTENT_ENCODING = "Content-Encoding";
/**
* The HTTP Content-Disposition
header field name
* @see RFC 6266
*/
String CONTENT_DISPOSITION = "Content-Disposition";
/**
* The HTTP Content-Language
header field name.
* @see Section 3.1.3.2 of RFC 7231
*/
String CONTENT_LANGUAGE = "Content-Language";
/**
* The HTTP Content-Length
header field name.
* @see Section 3.3.2 of RFC 7230
*/
String CONTENT_LENGTH = "Content-Length";
/**
* The HTTP Content-Location
header field name.
* @see Section 3.1.4.2 of RFC 7231
*/
String CONTENT_LOCATION = "Content-Location";
/**
* The HTTP Content-Range
header field name.
* @see Section 4.2 of RFC 7233
*/
String CONTENT_RANGE = "Content-Range";
/**
* The HTTP Content-Type
header field name.
* @see Section 3.1.1.5 of RFC 7231
*/
String CONTENT_TYPE = "Content-Type";
/**
* The HTTP Cookie
header field name.
* @see Section 4.3.4 of RFC 2109
*/
String COOKIE = "Cookie";
/**
* The HTTP Date
header field name.
* @see Section 7.1.1.2 of RFC 7231
*/
String DATE = "Date";
/**
* The HTTP ETag
header field name.
* @see Section 2.3 of RFC 7232
*/
String ETAG = "ETag";
/**
* The HTTP Expect
header field name.
* @see Section 5.1.1 of RFC 7231
*/
String EXPECT = "Expect";
/**
* The HTTP Expires
header field name.
* @see Section 5.3 of RFC 7234
*/
String EXPIRES = "Expires";
/**
* The HTTP From
header field name.
* @see Section 5.5.1 of RFC 7231
*/
String FROM = "From";
/**
* The HTTP Host
header field name.
* @see Section 5.4 of RFC 7230
*/
String HOST = "Host";
/**
* The HTTP If-Match
header field name.
* @see Section 3.1 of RFC 7232
*/
String IF_MATCH = "If-Match";
/**
* The HTTP If-Modified-Since
header field name.
* @see Section 3.3 of RFC 7232
*/
String IF_MODIFIED_SINCE = "If-Modified-Since";
/**
* The HTTP If-None-Match
header field name.
* @see Section 3.2 of RFC 7232
*/
String IF_NONE_MATCH = "If-None-Match";
/**
* The HTTP If-Range
header field name.
* @see Section 3.2 of RFC 7233
*/
String IF_RANGE = "If-Range";
/**
* The HTTP If-Unmodified-Since
header field name.
* @see Section 3.4 of RFC 7232
*/
String IF_UNMODIFIED_SINCE = "If-Unmodified-Since";
/**
* The HTTP Last-Modified
header field name.
* @see Section 2.2 of RFC 7232
*/
String LAST_MODIFIED = "Last-Modified";
/**
* The HTTP Link
header field name.
* @see RFC 5988
*/
String LINK = "Link";
/**
* The HTTP Location
header field name.
* @see Section 7.1.2 of RFC 7231
*/
String LOCATION = "Location";
/**
* The HTTP Max-Forwards
header field name.
* @see Section 5.1.2 of RFC 7231
*/
String MAX_FORWARDS = "Max-Forwards";
/**
* The HTTP Origin
header field name.
* @see RFC 6454
*/
String ORIGIN = "Origin";
/**
* The HTTP Pragma
header field name.
* @see Section 5.4 of RFC 7234
*/
String PRAGMA = "Pragma";
/**
* The HTTP Proxy-Authenticate
header field name.
* @see Section 4.3 of RFC 7235
*/
String PROXY_AUTHENTICATE = "Proxy-Authenticate";
/**
* The HTTP Proxy-Authorization
header field name.
* @see Section 4.4 of RFC 7235
*/
String PROXY_AUTHORIZATION = "Proxy-Authorization";
/**
* The HTTP Range
header field name.
* @see Section 3.1 of RFC 7233
*/
String RANGE = "Range";
/**
* The HTTP Referer
header field name.
* @see Section 5.5.2 of RFC 7231
*/
String REFERER = "Referer";
/**
* The HTTP Retry-After
header field name.
* @see Section 7.1.3 of RFC 7231
*/
String RETRY_AFTER = "Retry-After";
/**
* The HTTP Server
header field name.
* @see Section 7.4.2 of RFC 7231
*/
String SERVER = "Server";
/**
* The HTTP Set-Cookie
header field name.
* @see Section 4.2.2 of RFC 2109
*/
String SET_COOKIE = "Set-Cookie";
/**
* The HTTP Trailer
header field name.
* @see Section 4.4 of RFC 7230
*/
String TRAILER = "Trailer";
/**
* The HTTP Transfer-Encoding
header field name.
* @see Section 3.3.1 of RFC 7230
*/
String TRANSFER_ENCODING = "Transfer-Encoding";
/**
* The HTTP Upgrade
header field name.
* @see Section 6.7 of RFC 7230
*/
String UPGRADE = "Upgrade";
/**
* The HTTP User-Agent
header field name.
* @see Section 5.5.3 of RFC 7231
*/
String USER_AGENT = "User-Agent";
/**
* The HTTP Vary
header field name.
* @see Section 7.1.4 of RFC 7231
*/
String VARY = "Vary";
/**
* The HTTP Via
header field name.
* @see Section 5.7.1 of RFC 7230
*/
String VIA = "Via";
/**
* The HTTP Warning
header field name.
* @see Section 5.5 of RFC 7234
*/
String WARNING = "Warning";
/**
* The HTTP WWW-Authenticate
header field name.
* @see Section 4.1 of RFC 7235
*/
String WWW_AUTHENTICATE = "WWW-Authenticate";
// Authentication schemes
/**
* Basic authentication scheme
*/
String SCHEME_BASIC = "Basic";
/**
* Bearer authentication scheme
*/
String SCHEME_BEARER = "Bearer";
/**
* Digest authentication scheme
*/
String SCHEME_DIGEST = "Digest";
/**
* Get a HTTP header as a single string value.
* @param name The header name (not null)
* @return The HTTP header value. If the HTTP header is present more than once then the values are joined together
* and separated by a ',' character.
*/
default Optional getHeaderValue(String name) {
ObjectUtils.argumentNotNull(name, "Header name must be not null");
return getHeader(name).filter(l -> l != null && !l.isEmpty()).map(l -> l.get(0));
}
/**
* Return the date and time at which the message was created, as specified by the {@link #DATE} header.
* @return Message date/time, if available
*/
default Optional getDate() {
return getHeaderValue(DATE).map(d -> HttpUtils.parseHeaderDate(d));
}
/**
* Return the length of the request body in bytes, as specified by the {@link #CONTENT_LENGTH} header.
* @return Content length in bytes, if available
*/
default Optional getContentLength() {
return getHeaderValue(CONTENT_LENGTH).map(v -> Long.parseLong(v));
}
/**
* Return the resource location as specified by the {@link #LOCATION} header.
* @return location URI, if available
*/
default Optional getLocation() {
return getHeaderValue(LOCATION).map(v -> URI.create(v));
}
/**
* Try to get a list of {@link Locale} using the {@link #ACCEPT_LANGUAGE} header, if present. If more than one
* language is specified in the Accept-Language
header, returned Locales will be ordered relying on
* quality parameter, if specified.
* @return List of Locale for the languages of the Accept-Language
header, if any. If header is not
* present, an empty list is returned.
*/
default List getLocales() {
return HttpUtils.getAcceptLanguageLocales(getHeaderValue(ACCEPT_LANGUAGE).orElse(null));
}
/**
* Get the first (most qualified) {@link Locale} using the {@link #ACCEPT_LANGUAGE} header, if present.
* @return The first (most qualified) {@link Locale} using the {@link #ACCEPT_LANGUAGE} header, if available
*/
default Optional getLocale() {
final List locales = getLocales();
return (!locales.isEmpty()) ? Optional.ofNullable(locales.get(0)) : Optional.empty();
}
/**
* Return the authorization bearer token from the {@link #AUTHORIZATION} header, if present and of scheme
* type {@link #SCHEME_BEARER}.
* @return Authorization bearer token, if available
*/
default Optional getAuthorizationBearer() {
return getHeaderValue(AUTHORIZATION).map(v -> HttpUtils.extractAuthorizationBearer(v));
}
/**
* Return the basic authorization credential values from the {@link #AUTHORIZATION} header, if present and
* of scheme type {@link #SCHEME_BASIC}.
*
* The authorization credentials are decoded from Base64 before returning them to caller.
*
* @return Basic authorization credentials as an array which contains the username at index 0 and the
* password at index 1, if available
*/
default Optional getAuthorizationBasicCredentials() {
return getHeaderValue(AUTHORIZATION).map(v -> HttpUtils.extractAuthorizationBasicCredentials(v));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy