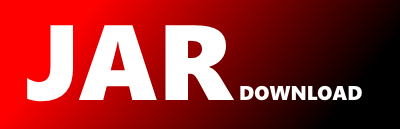
com.holonplatform.vaadin.internal.components.SingleSelectField Maven / Gradle / Ivy
/*
* Copyright 2016-2017 Axioma srl.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.holonplatform.vaadin.internal.components;
import java.util.Collection;
import java.util.Optional;
import com.holonplatform.core.i18n.Localizable;
import com.holonplatform.core.i18n.LocalizationContext;
import com.holonplatform.core.internal.utils.ObjectUtils;
import com.holonplatform.core.property.Property;
import com.holonplatform.core.property.PropertyBox;
import com.holonplatform.core.query.QueryFilter;
import com.holonplatform.vaadin.components.Field;
import com.holonplatform.vaadin.components.SingleSelect;
import com.holonplatform.vaadin.components.builders.BaseSelectInputBuilder.RenderingMode;
import com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.NativeModeSinglePropertySelectInputBuilder;
import com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.OptionsModeSinglePropertySelectInputBuilder;
import com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.SelectModeSinglePropertySelectInputBuilder;
import com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder.NativeModeSingleSelectInputBuilder;
import com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder.OptionsModeSingleSelectInputBuilder;
import com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder.SelectModeSingleSelectInputBuilder;
import com.holonplatform.vaadin.components.builders.SelectInputBuilder;
import com.holonplatform.vaadin.components.builders.SinglePropertySelectInputBuilder;
import com.holonplatform.vaadin.components.builders.SinglePropertySelectInputBuilder.GenericSinglePropertySelectInputBuilder;
import com.holonplatform.vaadin.components.builders.SingleSelectInputBuilder;
import com.holonplatform.vaadin.components.builders.SingleSelectInputBuilder.GenericSingleSelectInputBuilder;
import com.holonplatform.vaadin.data.ItemDataProvider;
import com.holonplatform.vaadin.internal.components.builders.AbstractSelectFieldBuilder;
import com.holonplatform.vaadin.internal.data.ItemDataProviderAdapter;
import com.holonplatform.vaadin.internal.data.PropertyItemIdentifier;
import com.vaadin.data.Converter;
import com.vaadin.data.HasDataProvider;
import com.vaadin.data.HasFilterableDataProvider;
import com.vaadin.data.provider.DataProvider;
import com.vaadin.event.selection.SingleSelectionEvent;
import com.vaadin.server.SerializableFunction;
import com.vaadin.server.SerializablePredicate;
import com.vaadin.ui.AbstractSingleSelect;
import com.vaadin.ui.ComboBox;
import com.vaadin.ui.ComboBox.CaptionFilter;
import com.vaadin.ui.NativeSelect;
import com.vaadin.ui.RadioButtonGroup;
/**
* Default single select {@link Field} implementation.
*
* @param Field type
*
* @since 5.0.0
*/
public class SingleSelectField extends AbstractSelectField>
implements SingleSelect {
private static final long serialVersionUID = -52116276228997170L;
/**
* Constructor
* @param type Selection value type
* @param renderingMode Rendering mode
*/
public SingleSelectField(Class extends T> type, RenderingMode renderingMode) {
super(type, renderingMode);
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.AbstractCustomField#buildInternalField(java.lang.Class)
*/
@Override
protected AbstractSingleSelect- buildInternalField(Class extends T> type) {
RenderingMode mode = getRenderingMode();
if (mode == null) {
mode = RenderingMode.SELECT;
}
if (mode == RenderingMode.NATIVE_SELECT) {
final NativeSelect
- field = new NativeSelect<>();
field.setItemCaptionGenerator(i -> generateItemCaption(i));
field.addSelectionListener(e -> fireSelectionListeners(buildSelectionEvent(e)));
return field;
}
if (mode == RenderingMode.OPTIONS) {
final RadioButtonGroup
- field = new RadioButtonGroup<>();
field.setItemCaptionGenerator(i -> generateItemCaption(i));
field.setItemIconGenerator(i -> generateItemIcon(i));
field.addSelectionListener(e -> fireSelectionListeners(buildSelectionEvent(e)));
return field;
}
final ComboBox
- field = new ComboBox<>();
field.setItemCaptionGenerator(i -> generateItemCaption(i));
field.setItemIconGenerator(i -> generateItemIcon(i));
field.addSelectionListener(e -> fireSelectionListeners(buildSelectionEvent(e)));
return field;
}
protected SelectionEvent
buildSelectionEvent(SingleSelectionEvent- event) {
return new DefaultSelectionEvent<>(event.getFirstSelectedItem().map(item -> fromInternalValue(item)).orElse(null),
event.isUserOriginated());
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.AbstractCustomField#fromInternalValue(java.lang.Object)
*/
@Override
protected T fromInternalValue(ITEM value) {
return toSelection(value);
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.AbstractCustomField#toInternalValue(java.lang.Object)
*/
@Override
protected ITEM toInternalValue(T value) {
return toItem(value);
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.AbstractSelectField#setItems(java.util.Collection)
*/
@Override
public void setItems(Collection
- items) {
getInternalField().setItems(items);
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.AbstractSelectField#setDataProvider(com.vaadin.data.provider.
* DataProvider)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@Override
public void setDataProvider(DataProvider
- dataProvider) {
if (getInternalField() instanceof HasDataProvider) {
((HasDataProvider) getInternalField()).setDataProvider(dataProvider);
} else if (getInternalField() instanceof HasFilterableDataProvider) {
((HasFilterableDataProvider) getInternalField()).setDataProvider(dataProvider, t -> null);
}
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.AbstractSelectField#setDataProvider(com.vaadin.data.provider.
* DataProvider, com.vaadin.server.SerializableFunction)
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@Override
public void setDataProvider(DataProvider
- dataProvider, SerializableFunction
filterConverter) {
if (filterConverter != null && getInternalField() instanceof ComboBox) {
((ComboBox) getInternalField()).setDataProvider(dataProvider, filterConverter);
} else {
setDataProvider(dataProvider);
}
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.AbstractSelectField#setItems(java.util.Collection,
* com.vaadin.ui.ComboBox.CaptionFilter)
*/
@Override
public void setItems(Collection- items, CaptionFilter filter) {
if (filter != null && getInternalField() instanceof ComboBox) {
((ComboBox
- ) getInternalField()).setItems(filter, items);
} else {
setItems(items);
}
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.AbstractSelectField#getDataProvider()
*/
@Override
public Optional
> getDataProvider() {
return Optional.ofNullable(getInternalField().getDataProvider());
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.Selectable#select(java.lang.Object)
*/
@Override
public void select(T item) {
getInternalField().setSelectedItem(toInternalValue(item));
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.Selectable#deselect(java.lang.Object)
*/
@Override
public void deselect(T item) {
if (item != null) {
getSelectedItem().filter(i -> i.equals(item)).ifPresent(i -> getInternalField().setSelectedItem(null));
}
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.SingleSelect#getSelectedItem()
*/
@Override
public Optional getSelectedItem() {
return Optional.of(getValue());
}
/**
* Set whether is possible to input text into the field. If the concrete select component does not support user
* input, this method has no effect.
* @param textInputAllowed true to allow entering text, false to just show the current selection
*/
public void setTextInputAllowed(boolean textInputAllowed) {
if (getInternalField() instanceof ComboBox) {
((ComboBox>) getInternalField()).setTextInputAllowed(textInputAllowed);
}
}
/**
* Sets the placeholder string - a textual prompt that is displayed when the select would otherwise be empty, to
* prompt the user for input.
* @param inputPrompt the desired placeholder, or null to disable
*/
public void setInputPrompt(String inputPrompt) {
if (getInternalField() instanceof ComboBox) {
((ComboBox>) getInternalField()).setPlaceholder(inputPrompt);
}
}
/**
* Sets whether to scroll the selected item visible (directly open the page on which it is) when opening the
* suggestions popup or not. This requires finding the index of the item, which can be expensive in many large lazy
* loading containers.
*
* Only applies to select field with backing components supporting a suggestion popup.
*
* @param scrollToSelectedItem true to find the page with the selected item when opening the selection popup
*/
public void setScrollToSelectedItem(boolean scrollToSelectedItem) {
if (getInternalField() instanceof ComboBox) {
((ComboBox>) getInternalField()).setScrollToSelectedItem(scrollToSelectedItem);
}
}
/**
* Sets the suggestion pop-up's width as a CSS string. By using relative units (e.g. "50%") it's possible to set the
* popup's width relative to the selection component itself.
*
* Only applies to select field with backing components supporting a suggestion popup.
*
* @param width the suggestion pop-up width
*/
public void setSuggestionPopupWidth(String width) {
if (getInternalField() instanceof ComboBox) {
((ComboBox>) getInternalField()).setPopupWidth(width);
}
}
public void setEmptySelectionAllowed(boolean emptySelectionAllowed) {
if (getInternalField() instanceof ComboBox) {
((ComboBox>) getInternalField()).setEmptySelectionAllowed(emptySelectionAllowed);
}
if (getInternalField() instanceof NativeSelect) {
((NativeSelect>) getInternalField()).setEmptySelectionAllowed(emptySelectionAllowed);
}
}
public void setEmptySelectionCaption(String caption) {
if (getInternalField() instanceof ComboBox) {
((ComboBox>) getInternalField()).setEmptySelectionCaption(caption);
}
if (getInternalField() instanceof NativeSelect) {
((NativeSelect>) getInternalField()).setEmptySelectionCaption(caption);
}
}
public void setHtmlContentAllowed(boolean htmlContentAllowed) {
if (getInternalField() instanceof RadioButtonGroup) {
((RadioButtonGroup>) getInternalField()).setHtmlContentAllowed(htmlContentAllowed);
}
}
@SuppressWarnings("unchecked")
public void setItemEnabledProvider(SerializablePredicate itemEnabledProvider) {
if (getInternalField() instanceof RadioButtonGroup) {
((RadioButtonGroup) getInternalField()).setItemEnabledProvider(itemEnabledProvider);
}
}
// Builders
/**
* Base {@link SingleSelect} builder.
* @param Selection type
*/
static abstract class AbstractSingleSelectFieldBuilder>
extends AbstractSelectFieldBuilder, T, ITEM, SingleSelectField, B>
implements SelectInputBuilder.SingleSelectConfigurator {
protected CaptionFilter captionFilter;
protected SerializableFunction filterProvider;
/**
* Constructor
* @param type Selection value type
* @param renderingMode Rendering mode
*/
public AbstractSingleSelectFieldBuilder(Class extends T> type, RenderingMode renderingMode) {
super(new SingleSelectField<>(type, renderingMode));
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.internal.components.builders.AbstractSelectFieldBuilder#configureDataSource(com.
* holonplatform.vaadin.internal.components.AbstractSelectField)
*/
@Override
protected void configureDataSource(SingleSelectField instance) {
if (!items.isEmpty()) {
instance.setItems(items, captionFilter);
} else if (itemDataProvider != null) {
instance.setDataProvider(new ItemDataProviderAdapter<>(itemDataProvider, itemIdentifier, null),
filterProvider);
} else {
instance.setDataProvider(dataProvider, filterProvider);
}
}
}
/**
* Default {@link SingleSelectInputBuilder} implementation.
* @param Selection type
*/
public static abstract class Builder>
extends AbstractSingleSelectFieldBuilder implements SingleSelectInputBuilder {
/**
* Constructor
* @param type Selection value type
* @param renderingMode Rendering mode
*/
public Builder(Class extends T> type, RenderingMode renderingMode) {
super(type, renderingMode);
getInstance().setItemConverter(Converter.identity());
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.SelectItemDataSourceBuilder#dataSource(com.holonplatform.vaadin.
* data.ItemDataProvider)
*/
@Override
public B dataSource(ItemDataProvider dataProvider) {
this.itemDataProvider = dataProvider;
return builder();
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.SelectItemDataSourceBuilder#dataSource(com.vaadin.data.provider.
* DataProvider)
*/
@Override
public B dataSource(DataProvider dataProvider) {
this.dataProvider = dataProvider;
return builder();
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.SingleSelectInputBuilder#dataSource(com.holonplatform.vaadin.
* data.ItemDataProvider, com.vaadin.server.SerializableFunction)
*/
@Override
public B dataSource(ItemDataProvider dataProvider,
SerializableFunction filterProvider) {
this.itemDataProvider = dataProvider;
this.filterProvider = filterProvider;
return builder();
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.SingleSelectInputBuilder#dataSource(com.vaadin.data.provider.
* DataProvider, com.vaadin.server.SerializableFunction)
*/
@Override
public B dataSource(DataProvider dataProvider, SerializableFunction filterProvider) {
this.dataProvider = dataProvider;
this.filterProvider = filterProvider;
return builder();
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.builders.AbstractSelectFieldBuilder#buildSelect(com.
* holonplatform.vaadin.internal.components.AbstractSelectField)
*/
@Override
protected SingleSelect buildSelect(SingleSelectField instance) {
return instance;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.builders.AbstractSelectFieldBuilder#buildSelectAsField(com.
* holonplatform.vaadin.internal.components.AbstractSelectField)
*/
@Override
protected Field buildSelectAsField(SingleSelectField instance) {
return instance;
}
}
public static class GenericBuilder extends Builder>
implements GenericSingleSelectInputBuilder {
public GenericBuilder(Class extends T> type, RenderingMode renderingMode) {
super(type, renderingMode);
}
@Override
protected GenericSingleSelectInputBuilder builder() {
return this;
}
}
public static class NativeModeBuilder extends Builder>
implements NativeModeSingleSelectInputBuilder {
protected Localizable emptySelectionCaption;
public NativeModeBuilder(Class extends T> type) {
super(type, RenderingMode.NATIVE_SELECT);
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.builders.AbstractComponentConfigurator#builder()
*/
@Override
protected NativeModeSingleSelectInputBuilder builder() {
return this;
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.internal.components.SingleSelectField.AbstractSingleSelectFieldBuilder#localize(com.
* holonplatform.vaadin.internal.components.SingleSelectField)
*/
@Override
protected void localize(SingleSelectField instance) {
super.localize(instance);
if (emptySelectionCaption != null) {
getInstance().setEmptySelectionCaption(LocalizationContext.translate(emptySelectionCaption, true));
}
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder#emptySelectionAllowed(
* boolean)
*/
@Override
public NativeModeSingleSelectInputBuilder emptySelectionAllowed(boolean emptySelectionAllowed) {
getInstance().setEmptySelectionAllowed(emptySelectionAllowed);
return this;
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder#emptySelectionCaption(com
* .holonplatform.core.i18n.Localizable)
*/
@Override
public NativeModeSingleSelectInputBuilder emptySelectionCaption(Localizable caption) {
this.emptySelectionCaption = caption;
return this;
}
}
public static class OptionsModeBuilder extends Builder>
implements OptionsModeSingleSelectInputBuilder {
public OptionsModeBuilder(Class extends T> type) {
super(type, RenderingMode.OPTIONS);
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder.
* OptionsModeSingleSelectInputBuilder#htmlContentAllowed(boolean)
*/
@Override
public OptionsModeSingleSelectInputBuilder htmlContentAllowed(boolean htmlContentAllowed) {
getInstance().setHtmlContentAllowed(htmlContentAllowed);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder.
* OptionsModeSingleSelectInputBuilder#itemEnabledProvider(com.vaadin.server.SerializablePredicate)
*/
@Override
public OptionsModeSingleSelectInputBuilder itemEnabledProvider(
SerializablePredicate itemEnabledProvider) {
ObjectUtils.argumentNotNull(itemEnabledProvider, "ItemEnabledProvider must be not null");
getInstance().setItemEnabledProvider(itemEnabledProvider);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.builders.AbstractComponentConfigurator#builder()
*/
@Override
protected OptionsModeSingleSelectInputBuilder builder() {
return this;
}
}
public static class SelectModeBuilder extends Builder>
implements SelectModeSingleSelectInputBuilder {
protected Localizable inputPrompt;
protected Localizable emptySelectionCaption;
public SelectModeBuilder(Class extends T> type) {
super(type, RenderingMode.SELECT);
}
@Override
protected SelectModeSingleSelectInputBuilder builder() {
return this;
}
@Override
protected void localize(SingleSelectField instance) {
super.localize(instance);
if (inputPrompt != null) {
getInstance().setInputPrompt(LocalizationContext.translate(inputPrompt, true));
}
if (emptySelectionCaption != null) {
getInstance().setEmptySelectionCaption(LocalizationContext.translate(emptySelectionCaption, true));
}
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.SingleSelectFieldBuilder#disableTextInput()
*/
@Override
public SelectModeSingleSelectInputBuilder disableTextInput() {
getInstance().setTextInputAllowed(false);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.SingleSelectFieldBuilder#scrollToSelectedItem(boolean)
*/
@Override
public SelectModeSingleSelectInputBuilder scrollToSelectedItem(boolean scrollToSelectedItem) {
getInstance().setScrollToSelectedItem(scrollToSelectedItem);
return this;
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.SingleSelectFieldBuilder#suggestionPopupWidth(java.lang.String)
*/
@Override
public SelectModeSingleSelectInputBuilder suggestionPopupWidth(String width) {
getInstance().setSuggestionPopupWidth(width);
return this;
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.BaseSelectInputBuilder.SingleSelectConfigurator#filteringMode(
* com.vaadin.ui.ComboBox.CaptionFilter)
*/
@Override
public SelectModeSingleSelectInputBuilder filteringMode(CaptionFilter captionFilter) {
this.captionFilter = captionFilter;
return this;
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.BaseSelectInputBuilder.SingleSelectConfigurator#inputPrompt(com.
* holonplatform.core.i18n.Localizable)
*/
@Override
public SelectModeSingleSelectInputBuilder inputPrompt(Localizable inputPrompt) {
this.inputPrompt = inputPrompt;
return this;
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder#emptySelectionAllowed(
* boolean)
*/
@Override
public SelectModeSingleSelectInputBuilder emptySelectionAllowed(boolean emptySelectionAllowed) {
getInstance().setEmptySelectionAllowed(emptySelectionAllowed);
return this;
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.BaseSelectModeSingleSelectInputBuilder#emptySelectionCaption(com
* .holonplatform.core.i18n.Localizable)
*/
@Override
public SelectModeSingleSelectInputBuilder emptySelectionCaption(Localizable caption) {
this.emptySelectionCaption = caption;
return this;
}
}
/**
* Default {@link SinglePropertySelectInputBuilder} implementation.
* @param Selection type
*/
public static abstract class PropertyBuilder> extends
AbstractSingleSelectFieldBuilder implements SinglePropertySelectInputBuilder {
/**
* Constructor
* @param selectProperty Selection (and identifier) property
* @param renderingMode Rendering mode
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public PropertyBuilder(Property selectProperty, RenderingMode renderingMode) {
super(selectProperty.getType(), renderingMode);
itemIdentifier = new PropertyItemIdentifier(selectProperty);
getInstance().setItemConverter(new DefaultPropertyBoxConverter<>(selectProperty));
}
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.builders.PropertySelectInputBuilder#dataSource(com.holonplatform.vaadin.
* data.ItemDataProvider)
*/
@Override
public B dataSource(ItemDataProvider dataProvider) {
this.itemDataProvider = dataProvider;
return builder();
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.builders.AbstractSelectFieldBuilder#buildSelect(com.
* holonframework.vaadin.internal.components.AbstractSelectField)
*/
@Override
protected SingleSelect buildSelect(SingleSelectField instance) {
return instance;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.builders.AbstractSelectFieldBuilder#buildSelectAsField(com.
* holonplatform.vaadin.internal.components.AbstractSelectField)
*/
@Override
protected Field buildSelectAsField(SingleSelectField instance) {
return instance;
}
}
public static class GenericPropertyBuilder extends PropertyBuilder>
implements GenericSinglePropertySelectInputBuilder {
public GenericPropertyBuilder(Property selectProperty, RenderingMode renderingMode) {
super(selectProperty, renderingMode);
}
@Override
protected GenericSinglePropertySelectInputBuilder builder() {
return this;
}
}
public static class NativeModePropertyBuilder
extends PropertyBuilder>
implements NativeModeSinglePropertySelectInputBuilder {
protected Localizable emptySelectionCaption;
public NativeModePropertyBuilder(Property selectProperty) {
super(selectProperty, RenderingMode.NATIVE_SELECT);
}
@Override
protected NativeModeSinglePropertySelectInputBuilder builder() {
return this;
}
@Override
protected void localize(SingleSelectField instance) {
super.localize(instance);
if (emptySelectionCaption != null) {
getInstance().setEmptySelectionCaption(LocalizationContext.translate(emptySelectionCaption, true));
}
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder#
* emptySelectionAllowed(boolean)
*/
@Override
public NativeModeSinglePropertySelectInputBuilder emptySelectionAllowed(boolean emptySelectionAllowed) {
getInstance().setEmptySelectionAllowed(emptySelectionAllowed);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder#
* emptySelectionCaption(com.holonplatform.core.i18n.Localizable)
*/
@Override
public NativeModeSinglePropertySelectInputBuilder emptySelectionCaption(Localizable caption) {
this.emptySelectionCaption = caption;
return this;
}
}
public static class OptionsModePropertyBuilder
extends PropertyBuilder>
implements OptionsModeSinglePropertySelectInputBuilder {
public OptionsModePropertyBuilder(Property selectProperty) {
super(selectProperty, RenderingMode.OPTIONS);
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.
* OptionsModeSinglePropertySelectInputBuilder#htmlContentAllowed(boolean)
*/
@Override
public OptionsModeSinglePropertySelectInputBuilder htmlContentAllowed(boolean htmlContentAllowed) {
getInstance().setHtmlContentAllowed(htmlContentAllowed);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.
* OptionsModeSinglePropertySelectInputBuilder#itemEnabledProvider(com.vaadin.server.SerializablePredicate)
*/
@Override
public OptionsModeSinglePropertySelectInputBuilder itemEnabledProvider(
SerializablePredicate itemEnabledProvider) {
ObjectUtils.argumentNotNull(itemEnabledProvider, "ItemEnabledProvider must be not null");
getInstance().setItemEnabledProvider(itemEnabledProvider);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.internal.components.builders.AbstractComponentConfigurator#builder()
*/
@Override
protected OptionsModeSinglePropertySelectInputBuilder builder() {
return this;
}
}
public static class SelectModePropertyBuilder
extends PropertyBuilder>
implements SelectModeSinglePropertySelectInputBuilder {
protected Localizable inputPrompt;
protected Localizable emptySelectionCaption;
public SelectModePropertyBuilder(Property selectProperty) {
super(selectProperty, RenderingMode.SELECT);
}
@Override
protected SelectModeSinglePropertySelectInputBuilder builder() {
return this;
}
@Override
public SelectModeSinglePropertySelectInputBuilder captionQueryFilter(
SerializableFunction filterProvider) {
this.filterProvider = filterProvider;
return this;
}
@Override
protected void localize(SingleSelectField instance) {
super.localize(instance);
if (inputPrompt != null) {
getInstance().setInputPrompt(LocalizationContext.translate(inputPrompt, true));
}
if (emptySelectionCaption != null) {
getInstance().setEmptySelectionCaption(LocalizationContext.translate(emptySelectionCaption, true));
}
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder#
* emptySelectionAllowed(boolean)
*/
@Override
public SelectModeSinglePropertySelectInputBuilder emptySelectionAllowed(boolean emptySelectionAllowed) {
getInstance().setEmptySelectionAllowed(emptySelectionAllowed);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder#
* emptySelectionCaption(com.holonplatform.core.i18n.Localizable)
*/
@Override
public SelectModeSinglePropertySelectInputBuilder emptySelectionCaption(Localizable caption) {
this.emptySelectionCaption = caption;
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.
* SelectModeSinglePropertySelectInputBuilder#inputPrompt(com.holonplatform.core.i18n.Localizable)
*/
@Override
public SelectModeSinglePropertySelectInputBuilder inputPrompt(Localizable inputPrompt) {
this.inputPrompt = inputPrompt;
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.
* SelectModeSinglePropertySelectInputBuilder#disableTextInput()
*/
@Override
public SelectModeSinglePropertySelectInputBuilder disableTextInput() {
getInstance().setTextInputAllowed(false);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.
* SelectModeSinglePropertySelectInputBuilder#scrollToSelectedItem(boolean)
*/
@Override
public SelectModeSinglePropertySelectInputBuilder scrollToSelectedItem(boolean scrollToSelectedItem) {
getInstance().setScrollToSelectedItem(scrollToSelectedItem);
return this;
}
/*
* (non-Javadoc)
* @see com.holonplatform.vaadin.components.builders.BaseSelectModeSinglePropertySelectInputBuilder.
* SelectModeSinglePropertySelectInputBuilder#suggestionPopupWidth(java.lang.String)
*/
@Override
public SelectModeSinglePropertySelectInputBuilder suggestionPopupWidth(String width) {
getInstance().setSuggestionPopupWidth(width);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy