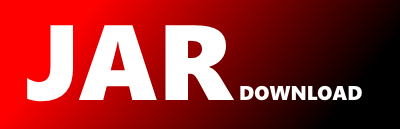
com.holonplatform.vaadin.internal.data.IdentifiablePropertyBox Maven / Gradle / Ivy
/*
* Copyright 2000-2017 Holon TDCN.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.holonplatform.vaadin.internal.data;
import java.util.Iterator;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Stream;
import com.holonplatform.core.Validator.ValidationException;
import com.holonplatform.core.internal.utils.ObjectUtils;
import com.holonplatform.core.property.Property;
import com.holonplatform.core.property.PropertyBox;
import com.holonplatform.vaadin.data.ItemIdentifierProvider;
/**
* A {@link PropertyBox} wrapper which uses an {@link ItemIdentifierProvider} to extract the identifiers to be used for
* equals
and hashCode
methods.
*
* @since 5.0.0
*/
public class IdentifiablePropertyBox implements PropertyBox {
private final PropertyBox propertyBox;
private final Object identifier;
public IdentifiablePropertyBox(PropertyBox propertyBox, ItemIdentifierProvider identifier) {
this(propertyBox, (Function) pb -> identifier.getItemId(pb));
}
public IdentifiablePropertyBox(PropertyBox propertyBox, Function identifier) {
super();
ObjectUtils.argumentNotNull(propertyBox, "PropertyBox must be not null");
ObjectUtils.argumentNotNull(identifier, "Identifier function must be not null");
this.propertyBox = propertyBox;
this.identifier = identifier.apply(propertyBox);
if (this.identifier == null) {
throw new IllegalStateException(
"The identifier function returned a null identifier for ProprtyBox [" + propertyBox + "]");
}
}
/**
* Get the {@link PropertyBox} identifier.
* @return the identifier (not null)
*/
public Object getIdentifier() {
return identifier;
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertySet#size()
*/
@Override
public int size() {
return propertyBox.size();
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertySet#contains(com.holonplatform.core.property.Property)
*/
@SuppressWarnings("rawtypes")
@Override
public boolean contains(Property property) {
return propertyBox.contains(property);
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertySet#stream()
*/
@SuppressWarnings("rawtypes")
@Override
public Stream stream() {
return propertyBox.stream();
}
/*
* (non-Javadoc)
* @see java.lang.Iterable#iterator()
*/
@SuppressWarnings("rawtypes")
@Override
public Iterator iterator() {
return propertyBox.iterator();
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertyBox#containsValue(com.holonplatform.core.property.Property)
*/
@Override
public boolean containsValue(Property property) {
return propertyBox.containsValue(property);
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertyBox#getValue(com.holonplatform.core.property.Property)
*/
@Override
public T getValue(Property property) {
return propertyBox.getValue(property);
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertyBox#getValueIfPresent(com.holonplatform.core.property.Property)
*/
@Override
public Optional getValueIfPresent(Property property) {
return propertyBox.getValueIfPresent(property);
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertyBox#propertyValues()
*/
@Override
public Stream> propertyValues() {
return propertyBox.propertyValues();
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertyBox#setValue(com.holonplatform.core.property.Property,
* java.lang.Object)
*/
@Override
public void setValue(Property property, T value) {
propertyBox.setValue(property, value);
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertyBox#isInvalidAllowed()
*/
@Override
public boolean isInvalidAllowed() {
return propertyBox.isInvalidAllowed();
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertyBox#setInvalidAllowed(boolean)
*/
@Override
public void setInvalidAllowed(boolean invalidAllowed) {
propertyBox.setInvalidAllowed(invalidAllowed);
}
/*
* (non-Javadoc)
* @see com.holonplatform.core.property.PropertyBox#validate()
*/
@Override
public void validate() throws ValidationException {
propertyBox.validate();
}
/*
* (non-Javadoc)
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((identifier == null) ? 0 : identifier.hashCode());
return result;
}
/*
* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
IdentifiablePropertyBox other = (IdentifiablePropertyBox) obj;
if (identifier == null) {
if (other.identifier != null)
return false;
} else if (!identifier.equals(other.identifier))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy