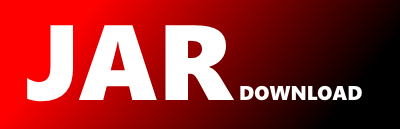
com.holonplatform.vaadin7.components.Dialog Maven / Gradle / Ivy
/*
* Copyright 2016-2017 Axioma srl.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.holonplatform.vaadin7.components;
import com.holonplatform.core.Context;
import com.holonplatform.core.i18n.Localizable;
import com.holonplatform.core.i18n.LocalizationContext;
import com.holonplatform.vaadin7.Registration;
import com.holonplatform.vaadin7.components.builders.ComponentBuilder;
import com.holonplatform.vaadin7.components.builders.ButtonConfigurator.BaseButtonConfigurator;
import com.holonplatform.vaadin7.internal.components.DefaultDialog;
import com.holonplatform.vaadin7.internal.components.QuestionDialog;
import com.vaadin.ui.Component;
import com.vaadin.ui.UI;
/**
* Dialog window representation.
*
* @since 5.0.0
*/
public interface Dialog extends Component {
/**
* Returns whether the dialog is modal.
* @return true
if the dialog is modal, false
otherwise
*/
boolean isModal();
/**
* Sets whether the dialog is modal. When a modal dialog is open, components outside that dialog window cannot be
* accessed.
* @param modal true
to set the dialog as modal, false
to unset
*/
void setModal(boolean modal);
/**
* If there are currently several window dialogs visible, calling this method makes this dialog window topmost.
*
* This method can only be called if this dialog is connected to a UI. Else an illegal state exception is thrown.
* Also if there are modal windows and this dialog window is not modal, and illegal state exception is thrown.
*
*/
void bringToFront();
/**
* Open the dialog window using current {@link UI}.
*/
default void open() {
open(UI.getCurrent());
}
/**
* Open the dialog window using given {@link UI}.
* @param ui UI to which to attach the dialog window
*/
void open(UI ui);
/**
* Closes the dialog window
*/
void close();
/**
* Adds a {@link CloseListener} for dialog closing events.
* @param listener The listener to add (not null)
* @return the listener {@link Registration}
*/
Registration addCloseListener(CloseListener listener);
/**
* Listener for dialog closing event
*/
@FunctionalInterface
public interface CloseListener {
/**
* Invoked when the {@link Dialog} is closed
* @param dialog the dialog which was closed
* @param actionId Optional id of the action which caused the dialog closure
*/
void dialogClosed(Dialog dialog, Object actionId);
}
/**
* {@link CloseListener} extension which acts as callback for a question dialog to react to the user's response.
*/
@FunctionalInterface
public interface QuestionCallback extends CloseListener {
/*
* (non-Javadoc)
* @see
* com.holonplatform.vaadin.components.Dialog.CloseListener#dialogClosed(com.holonplatform.vaadin.components.
* Dialog, java.lang.Object)
*/
@Override
default void dialogClosed(Dialog dialog, Object actionId) {
questionResponse(actionId != null && (boolean) actionId);
}
/**
* Invoked when the question dialog is closed as a result of a user action.
* @param answeredYes true
if the user selected the yes action, false
* otherwise
*/
void questionResponse(boolean answeredYes);
}
// Builders
/**
* Gets a builder to create and open a {@link Dialog} window.
*
* The dialog will present by default a single ok button.
*
* @return {@link Dialog} builder
*/
public static DialogBuilder builder() {
return new DefaultDialog.DefaultBuilder();
}
/**
* Gets a builder to create and open a question {@link Dialog} window.
*
* The dialog will present by default a yes and a no button. Use
* {@link QuestionDialogBuilder#callback(com.holonplatform.vaadin7.components.Dialog.QuestionCallback)} to handle
* the user selected answer.
*
* @return Question {@link Dialog} builder
*/
public static QuestionDialogBuilder question() {
return new QuestionDialog.DefaultBuilder();
}
/**
* Interface to configure {@link Dialog} buttons at build time.
*/
@FunctionalInterface
public interface DialogButtonConfigurator {
/**
* Configure the dialog button
* @param configurator Configurator to configure the dialog button
*/
void configureDialogButton(BaseButtonConfigurator configurator);
}
/**
* Question {@link Dialog} builder.
*/
public interface QuestionDialogBuilder extends Builder {
/**
* Set a {@link DialogButtonConfigurator} to configure the yes answer dialog button.
* @param configurator Dialog button configurator
* @return this
*/
QuestionDialogBuilder yesButtonConfigurator(DialogButtonConfigurator configurator);
/**
* Set a {@link DialogButtonConfigurator} to configure the no answer dialog button.
* @param configurator Dialog button configurator
* @return this
*/
QuestionDialogBuilder noButtonConfigurator(DialogButtonConfigurator configurator);
/**
* Set the callback to use when user select a yes or no answer from the question dialog.
* @param callback Question dialog callback
* @return this
*/
QuestionDialogBuilder callback(QuestionCallback callback);
}
/**
* Default {@link Dialog} builder.
*/
public interface DialogBuilder extends Builder {
/**
* Set a {@link DialogButtonConfigurator} to configure the ok dialog button.
* @param configurator Dialog button configurator
* @return this
*/
DialogBuilder okButtonConfigurator(DialogButtonConfigurator configurator);
}
/**
* Base dialog builder
* @param Concrete builder type
*/
public interface Builder> extends ComponentBuilder
© 2015 - 2024 Weber Informatics LLC | Privacy Policy