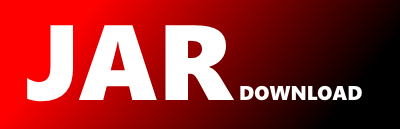
com.holonplatform.vaadin7.components.builders.BaseSelectInputBuilder Maven / Gradle / Ivy
/*
* Copyright 2016-2017 Axioma srl.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.holonplatform.vaadin7.components.builders;
import java.util.Set;
import com.holonplatform.core.i18n.Localizable;
import com.holonplatform.vaadin7.components.Input;
import com.holonplatform.vaadin7.components.MultiSelect;
import com.holonplatform.vaadin7.components.SingleSelect;
import com.holonplatform.vaadin7.components.ItemSet.ItemCaptionGenerator;
import com.holonplatform.vaadin7.components.ItemSet.ItemIconGenerator;
import com.vaadin.server.Resource;
import com.vaadin.shared.ui.combobox.FilteringMode;
/**
* Base builder to create selection {@link Input}s.
*
* @param Value type
* @param Input type
* @param Selection type
* @param - Selection items type
* @param Concrete builder type
*
* @since 5.0.0
*/
public interface BaseSelectInputBuilder
, S, ITEM, B extends BaseSelectInputBuilder>
extends InputBuilder, BaseItemDataSourceComponentBuilder {
/**
* Select UI rendering mode
*/
public enum RenderingMode {
/**
* Renders as native select, i.e. using client browser native rendering.
*
* This rendering mode is not suitable for multi-select mode. In this case, {@link #SELECT} will be used as
* fallback.
*
*/
NATIVE_SELECT,
/**
* Renders as a select field (A ComboBox for single selection mode or a ListSelect for multiple selection
* mode)
*/
SELECT,
/**
* Renders as an options group (A checkbox group for single selection mode or a radio buttons group for
* multiple selection mode)
*/
OPTIONS
}
/**
* Set the item caption generator to use to display item captions.
* @param itemCaptionGenerator The generator to set
* @return this
*/
B itemCaptionGenerator(ItemCaptionGenerator- itemCaptionGenerator);
/**
* Set the item icon generator to use to display item icons.
* @param itemIconGenerator The generator to set
* @return this
*/
B itemIconGenerator(ItemIconGenerator
- itemIconGenerator);
/**
* Set an explicit caption for given item.
* @param item Item to set the caption for (not null)
* @param caption Item caption (not null)
* @return this
*/
B itemCaption(ITEM item, Localizable caption);
/**
* Set an explicit caption for given item.
* @param item Item to set the caption for (not null)
* @param caption Item caption
* @return this
*/
default B itemCaption(ITEM item, String caption) {
return itemCaption(item, Localizable.builder().message(caption).build());
}
/**
* Set an explicit caption for given item.
* @param item Item to set the caption for (not null)
* @param caption Item caption
* @param messageCode Item caption translation code
* @return this
*/
default B itemCaption(ITEM item, String caption, String messageCode) {
return itemCaption(item, Localizable.builder().message(caption).messageCode(messageCode).build());
}
/**
* Sets the icon for an item.
* @param item the item to set the icon for
* @param icon Item icon
* @return this
*/
B itemIcon(ITEM item, Resource icon);
/**
* Single select component configurator.
* @param
Field type
* @param - Item type
* @param Concrete builder type
*/
public interface SingleSelectConfigurator
>
extends BaseSelectInputBuilder, T, ITEM, B> {
/**
* Disables the possibility to input text into the field, so the field area of the component is just used to
* show what is selected. If the concrete select component does not support user input, this method has no
* effect.
* @return this
*/
B disableTextInput();
/**
* Sets whether to scroll the selected item visible (directly open the page on which it is) when opening the
* combo box popup or not. Only applies to select components with a suggestions popup. This requires finding the
* index of the item, which can be expensive in many large lazy loading containers.
* @param scrollToSelectedItem true to find the page with the selected item when opening the selection popup
* @return this
*/
B scrollToSelectedItem(boolean scrollToSelectedItem);
/**
* Sets the suggestion pop-up's width as a CSS string. By using relative units (e.g. "50%") it's possible to set
* the popup's width relative to the selection component itself.
*
* Only applies to select field with backing components supporting a suggestion popup.
*
* @param width the suggestion pop-up width
* @return this
*/
B suggestionPopupWidth(String width);
/**
* Sets the option filtering mode.
*
* Only applies to select field with backing components supporting a suggestion popup.
*
* @param filteringMode the filtering mode to use
* @return this
*/
B filteringMode(FilteringMode filteringMode);
}
/**
* Multi select component configurator.
* @param Field type
* @param - Item type
* @param Concrete builder type
*/
public interface MultiSelectConfigurator
>
extends BaseSelectInputBuilder, MultiSelect, T, ITEM, B> {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy