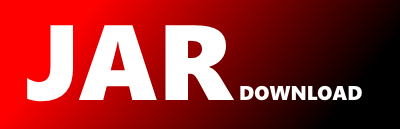
com.holonplatform.vaadin7.components.builders.PropertySelectInputBuilder Maven / Gradle / Ivy
/*
* Copyright 2016-2017 Axioma srl.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.holonplatform.vaadin7.components.builders;
import com.holonplatform.core.datastore.DataTarget;
import com.holonplatform.core.datastore.Datastore;
import com.holonplatform.core.internal.utils.ObjectUtils;
import com.holonplatform.core.property.Property;
import com.holonplatform.core.property.PropertyBox;
import com.holonplatform.core.property.PropertySet;
import com.holonplatform.vaadin7.components.Input;
import com.holonplatform.vaadin7.data.ItemDataProvider;
import com.holonplatform.vaadin7.data.container.ItemAdapter;
/**
* Builder to create selection {@link Input}s with {@link Property} data source support.
*
* @param Value type
* @param Component type
* @param Selection type
* @param Concrete builder type
*
* @since 5.0.0
*/
public interface PropertySelectInputBuilder, S, B extends PropertySelectInputBuilder>
extends BaseSelectInputBuilder {
/**
* Set the selection items data provider to obtain items.
* @param Property type
* @param dataProvider Items data provider (not null)
* @param properties Item property set (not null)
* @return this
*/
> B dataSource(ItemDataProvider dataProvider, Iterable properties);
/**
* Set the selection items data provider to obtain items.
* @param
Property type
* @param dataProvider Items data provider (not null)
* @param properties Item property set (not null)
* @return this
*/
@SuppressWarnings("unchecked")
default
> B dataSource(ItemDataProvider dataProvider, P... properties) {
ObjectUtils.argumentNotNull(properties, "Properties must be not null");
return dataSource(dataProvider, PropertySet.of(properties));
}
/**
* Use given {@link Datastore} with given dataTarget
as items data source.
* @param Property type
* @param datastore Datastore to use (not null)
* @param dataTarget Data target to use to load items (not null)
* @param properties Item property set (not null)
* @return this
*/
@SuppressWarnings("rawtypes")
default
B dataSource(Datastore datastore, DataTarget> dataTarget, Iterable
properties) {
ObjectUtils.argumentNotNull(properties, "Properties must be not null");
return dataSource(
ItemDataProvider.create(datastore, dataTarget,
(properties instanceof PropertySet) ? (PropertySet>) properties : PropertySet.of(properties)),
properties);
}
/**
* Use given {@link Datastore} with given dataTarget
as items data source.
* @param
Property type
* @param datastore Datastore to use (not null)
* @param dataTarget Data target to use to load items (not null)
* @param properties Item property set (not null)
* @return this
*/
@SuppressWarnings("unchecked")
default
> B dataSource(Datastore datastore, DataTarget> dataTarget, P... properties) {
ObjectUtils.argumentNotNull(properties, "Properties must be not null");
final PropertySet
set = PropertySet.of(properties);
return dataSource(ItemDataProvider.create(datastore, dataTarget, set), set);
}
/**
* Set the item adapter to use to convert data source items into container items and back.
* @param itemAdapter the item adapter (not null)
* @return this
*/
B itemAdapter(ItemAdapter itemAdapter);
}