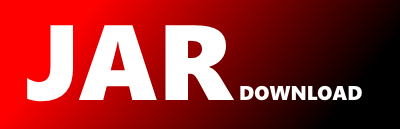
com.holonplatform.vaadin7.data.ItemDataSource Maven / Gradle / Ivy
/*
* Copyright 2016-2017 Axioma srl.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.holonplatform.vaadin7.data;
import java.io.Serializable;
import java.util.Collection;
import java.util.Optional;
import com.holonplatform.core.exceptions.DataAccessException;
import com.holonplatform.core.internal.utils.ObjectUtils;
import com.holonplatform.core.query.QueryConfigurationProvider;
import com.holonplatform.core.query.QuerySort;
import com.holonplatform.vaadin7.internal.data.DefaultItemSort;
/**
* Represents an item set data source, providing operations to retrieve items by id and change the item set composition
* adding a removing items.
*
* Items values are accessed using a defined set of properties.
*
*
* Item set modifications can be persisted in a backend data store using a {@link CommitHandler}, triggered through the
* {@link #commit()} method.
*
*
* @param - Item type
* @param
Item property type
*
* @since 5.0.0
*
* @see ItemDataProvider
*/
public interface ItemDataSource- extends Serializable {
/**
* Get the data source configuration.
* @return Data source configuration (never null)
*/
Configuration
getConfiguration();
/**
* Refresh data source items.
* @throws DataAccessException Error performing concrete items loading operations
*/
void refresh();
/**
* Clear data source contents (current item set)
*/
void clear();
/**
* Returns current data source size (considering any applied filter), i.e. the total number of available items.
* @return the total number of available items in data source
*/
int size();
/**
* Get all item ids available from this data source
* @return Item ids, empty if none
*/
Collection> getItemIds();
/**
* Get the identifier of given item
* @param item Item to get the identifier for
* @return Item identifier
*/
Object getId(ITEM item);
/**
* Get the item identified by given itemId
.
* @param itemId Item id (not null)
* @return Optional item identified by given itemId
, empty if not found
*/
Optional- get(Object itemId);
/**
* Adds an item to the data source.
* @param item The item to add (not null)
* @return Id of the added item
*/
Object add(ITEM item);
/**
* Update given item in data source.
* @param item Item to update (not null)
*/
void update(ITEM item);
/**
* Removes given item from the data source.
* @param item Item to remove (not null)
* @return
true
if the item was successfully removed from data source
*/
boolean remove(ITEM item);
/**
* Refresh given item in data source
* @param item Item to refresh (not null)
* @throws UnsupportedOperationException If the refresh operation is not supported by concrete data store
*/
void refresh(ITEM item);
/**
* Updates all changes since the previous commit.
*/
void commit();
/**
* Discards all changes since last commit.
*/
void discard();
/**
* Get whether the data source is in buffered mode.
* @return whether the data source is in buffered mode
*/
boolean isBuffered();
/**
* Sort the data source items using given {@link ItemSort} directives.
* @param sorts Item sorts to apply
*/
@SuppressWarnings("unchecked")
void sort(ItemSort... sorts);
/**
* Item actions enumeration.
*/
public enum ItemAction {
/**
* An item was loaded
*/
LOADED,
/**
* An item was added
*/
ADDED,
/**
* An item was modified
*/
MODIFIED,
/**
* An item was modified
*/
REFRESHED,
/**
* An item was removed
*/
REMOVED,
/**
* The item set changed
*/
SET_CHANGED;
}
// Data source configuration
public interface Configuration extends QueryConfigurationProvider, Serializable {
/**
* Get available item properties.
* @return Available item properties iterable
*/
Iterable getProperties();
/**
* Gets the data type of the given data source property.
* @param property Property (not null)
* @return Property data type
*/
Class> getPropertyType(PROPERTY property);
/**
* Get whether the given property is read only.
* @param property Property (not null)
* @return true
if property is read only, false
otherwise
*/
boolean isPropertyReadOnly(PROPERTY property);
/**
* Get whether the given property is sortable.
* @param property Property (not null)
* @return true
if property is sortable, false
otherwise
*/
boolean isPropertySortable(PROPERTY property);
/**
* Get the default value for the given property.
* @param property Property (not null)
* @return Property default value, or null
if not available
*/
Object getPropertyDefaultValue(PROPERTY property);
}
/**
* An item sort directive.
* @param Item property type
*/
public interface ItemSort extends Serializable {
/**
* Item property to sort
* @return Item property to sort
*/
PROPERTY getProperty();
/**
* Sort direction
* @return true
if ascending, false
if descending
*/
boolean isAscending();
/**
* Create an {@link ItemSort} using given property and sort direction.
* @param Property type
* @param property Property to sort (not null)
* @param ascending true
to sort ascending, false
for descending
* @return Item sort
*/
static ItemSort of(PROPERTY property, boolean ascending) {
ObjectUtils.argumentNotNull(property, "Sort property must be not null");
return new DefaultItemSort<>(property, ascending);
}
/**
* Create an ascending {@link ItemSort} using given property.
* @param Property type
* @param property Property to sort (not null)
* @return Item sort
*/
static ItemSort asc(PROPERTY property) {
return of(property, true);
}
/**
* Create an descending {@link ItemSort} using given property.
* @param Property type
* @param property Property to sort (not null)
* @return Item sort
*/
static ItemSort desc(PROPERTY property) {
return of(property, false);
}
}
/**
* {@link QuerySort} generator to provide query sorts for an item property.
*/
@FunctionalInterface
public interface PropertySortGenerator extends Serializable {
/**
* Get the {@link QuerySort} to use for given property
with specified sort direction.
* @param property Property to sort
* @param ascending Sort direction
* @return QuerySort
*/
QuerySort getQuerySort(PROPERTY property, boolean ascending);
}
/**
* Data source commit handler to perform concrete persistence operations when data is committed invoking
* {@link ItemDataSource#commit()}.
* @param - Item type
*/
@FunctionalInterface
public interface CommitHandler
- extends Serializable {
/**
* Commit item modifications.
* @param addedItems Added items: an empty collection if none
* @param modifiedItems Modified items: an empty collection if none
* @param removedItems Removed items: an empty collection if none
*/
void commit(Collection
- addedItems, Collection
- modifiedItems, Collection
- removedItems);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy