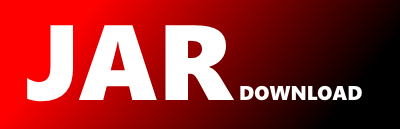
com.hopper.cloud.airlines.model.CfarContract Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-airlines-java Show documentation
Show all versions of cloud-airlines-java Show documentation
Use the Hopper cloud airlines Web Services in Java!
The newest version!
/*
* Airline API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: v1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.hopper.cloud.airlines.model;
import java.util.Objects;
import com.google.gson.*;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.google.gson.reflect.TypeToken;
import com.hopper.cloud.airlines.JSON;
/**
* A CFAR contract
*/
@ApiModel(description = "A CFAR contract")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-06-28T12:18:49.517876+02:00[Europe/Paris]")
public class CfarContract {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_REFERENCE = "reference";
@SerializedName(SERIALIZED_NAME_REFERENCE)
private String reference;
public static final String SERIALIZED_NAME_OFFERS = "offers";
@SerializedName(SERIALIZED_NAME_OFFERS)
private List offers = new ArrayList<>();
public static final String SERIALIZED_NAME_ITINERARY = "itinerary";
@SerializedName(SERIALIZED_NAME_ITINERARY)
private CfarItinerary itinerary;
public static final String SERIALIZED_NAME_COVERAGE_PERCENTAGE = "coverage_percentage";
@SerializedName(SERIALIZED_NAME_COVERAGE_PERCENTAGE)
private String coveragePercentage;
public static final String SERIALIZED_NAME_COVERAGE = "coverage";
@SerializedName(SERIALIZED_NAME_COVERAGE)
private String coverage;
public static final String SERIALIZED_NAME_PREMIUM = "premium";
@SerializedName(SERIALIZED_NAME_PREMIUM)
private String premium;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_TAXES_TOTAL = "taxes_total";
@SerializedName(SERIALIZED_NAME_TAXES_TOTAL)
private String taxesTotal;
public static final String SERIALIZED_NAME_TAXES = "taxes";
@SerializedName(SERIALIZED_NAME_TAXES)
private List taxes = new ArrayList<>();
public static final String SERIALIZED_NAME_CREATED_DATE_TIME = "created_date_time";
@SerializedName(SERIALIZED_NAME_CREATED_DATE_TIME)
private OffsetDateTime createdDateTime;
public static final String SERIALIZED_NAME_EXPIRY_DATE_TIME = "expiry_date_time";
@SerializedName(SERIALIZED_NAME_EXPIRY_DATE_TIME)
private OffsetDateTime expiryDateTime;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private CfarContractStatus status;
public static final String SERIALIZED_NAME_CONFIRMED_DATE_TIME = "confirmed_date_time";
@SerializedName(SERIALIZED_NAME_CONFIRMED_DATE_TIME)
private OffsetDateTime confirmedDateTime;
public static final String SERIALIZED_NAME_PNR_REFERENCE = "pnr_reference";
@SerializedName(SERIALIZED_NAME_PNR_REFERENCE)
private String pnrReference;
public static final String SERIALIZED_NAME_EXT_ATTRIBUTES = "ext_attributes";
@SerializedName(SERIALIZED_NAME_EXT_ATTRIBUTES)
private Map extAttributes = new HashMap<>();
public CfarContract() {
}
public CfarContract id(String id) {
this.id = id;
return this;
}
/**
* Unique identifier for a contract
*
* @return id
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "9f4f2f2b-adfd-4f02-83ad-da336ed57ce0", required = true, value = "Unique identifier for a contract")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public CfarContract reference(String reference) {
this.reference = reference;
return this;
}
/**
* Unique reference for a contract
*
* @return reference
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "MCNZZABRTYFEUAAL", required = true, value = "Unique reference for a contract")
public String getReference() {
return reference;
}
public void setReference(String reference) {
this.reference = reference;
}
public CfarContract offers(List offers) {
this.offers = offers;
return this;
}
public CfarContract addOffersItem(CfarOffer offersItem) {
this.offers.add(offersItem);
return this;
}
/**
* An offer associated with this contract
*
* @return offers
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "60a128e4-a12d-42bf-b977-b6329392282d", required = true, value = "An offer associated with this contract")
public List getOffers() {
return offers;
}
public void setOffers(List offers) {
this.offers = offers;
}
public CfarContract itinerary(CfarItinerary itinerary) {
this.itinerary = itinerary;
return this;
}
/**
* Get itinerary
*
* @return itinerary
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public CfarItinerary getItinerary() {
return itinerary;
}
public void setItinerary(CfarItinerary itinerary) {
this.itinerary = itinerary;
}
public CfarContract coveragePercentage(String coveragePercentage) {
this.coveragePercentage = coveragePercentage;
return this;
}
/**
* Percentage of the amount to be refunded to customer compared to flight tickets price
* @return coveragePercentage
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "80.0", required = true, value = "Percentage of the amount to be refunded to customer compared to flight tickets price")
public String getCoveragePercentage() {
return coveragePercentage;
}
public void setCoveragePercentage(String coveragePercentage) {
this.coveragePercentage = coveragePercentage;
}
public CfarContract coverage(String coverage) {
this.coverage = coverage;
return this;
}
/**
* Total amount to be refunded to user upon CFAR exercise
*
* @return coverage
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "361.20", required = true, value = "Total amount to be refunded to user upon CFAR exercise")
public String getCoverage() {
return coverage;
}
public void setCoverage(String coverage) {
this.coverage = coverage;
}
public CfarContract premium(String premium) {
this.premium = premium;
return this;
}
/**
* Get premium
*
* @return premium
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public String getPremium() {
return premium;
}
public void setPremium(String premium) {
this.premium = premium;
}
public String getTaxesTotal() {
return taxesTotal;
}
public void setTaxesTotal(String taxesTotal) {
this.taxesTotal = taxesTotal;
}
public CfarContract taxes(List taxes) {
this.taxes = taxes;
return this;
}
public CfarContract addCfarTaxItem(CfarTax cfarTaxItem) {
if (this.taxes != null) {
this.taxes.add(cfarTaxItem);
}
return this;
}
public List getTaxes() {
return taxes;
}
public void setTaxes(List taxes) {
this.taxes = taxes;
}
public CfarContract currency(String currency) {
this.currency = currency;
return this;
}
/**
* Currency of the contract
*
* @return currency
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "CAD", required = true, value = "Currency of the contract")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CfarContract createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
return this;
}
/**
* A UTC [RFC3339](https://xml2rfc.tools.ietf.org/public/rfc/html/rfc3339.html#anchor14) datetime; the date and time at which the contract was created
*
* @return createdDateTime
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "2020-11-02T18:34:30Z", required = true, value = "A UTC [RFC3339](https://xml2rfc.tools.ietf.org/public/rfc/html/rfc3339.html#anchor14) datetime; the date and time at which the contract was created")
public OffsetDateTime getCreatedDateTime() {
return createdDateTime;
}
public void setCreatedDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
}
public CfarContract expiryDateTime(OffsetDateTime expiryDateTime) {
this.expiryDateTime = expiryDateTime;
return this;
}
/**
* Get expiryDateTime
*
* @return expiryDateTime
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public OffsetDateTime getExpiryDateTime() {
return expiryDateTime;
}
public void setExpiryDateTime(OffsetDateTime expiryDateTime) {
this.expiryDateTime = expiryDateTime;
}
public CfarContract status(CfarContractStatus status) {
this.status = status;
return this;
}
/**
* Get status
*
* @return status
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public CfarContractStatus getStatus() {
return status;
}
public void setStatus(CfarContractStatus status) {
this.status = status;
}
public CfarContract confirmedDateTime(OffsetDateTime confirmedDateTime) {
this.confirmedDateTime = confirmedDateTime;
return this;
}
/**
* Get confirmedDateTime
*
* @return confirmedDateTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public OffsetDateTime getConfirmedDateTime() {
return confirmedDateTime;
}
public void setConfirmedDateTime(OffsetDateTime confirmedDateTime) {
this.confirmedDateTime = confirmedDateTime;
}
/**
* Get pnrReference
*
* @return pnrReference
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getPnrReference() {
return pnrReference;
}
public void setPnrReference(String pnrReference) {
this.pnrReference = pnrReference;
}
public CfarContract extAttributes(Map extAttributes) {
this.extAttributes = extAttributes;
return this;
}
public CfarContract putExtAttributesItem(String key, String extAttributesItem) {
this.extAttributes.put(key, extAttributesItem);
return this;
}
/**
* Get extAttributes
*
* @return extAttributes
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public Map getExtAttributes() {
return extAttributes;
}
public void setExtAttributes(Map extAttributes) {
this.extAttributes = extAttributes;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CfarContract cfarContract = (CfarContract) o;
return Objects.equals(this.id, cfarContract.id) &&
Objects.equals(this.reference, cfarContract.reference) &&
Objects.equals(this.offers, cfarContract.offers) &&
Objects.equals(this.itinerary, cfarContract.itinerary) &&
Objects.equals(this.coverage, cfarContract.coverage) &&
Objects.equals(this.coveragePercentage, cfarContract.coveragePercentage) &&
Objects.equals(this.premium, cfarContract.premium) &&
Objects.equals(this.taxesTotal, cfarContract.taxesTotal) &&
Objects.equals(this.taxes, cfarContract.taxes) &&
Objects.equals(this.currency, cfarContract.currency) &&
Objects.equals(this.createdDateTime, cfarContract.createdDateTime) &&
Objects.equals(this.expiryDateTime, cfarContract.expiryDateTime) &&
Objects.equals(this.status, cfarContract.status) &&
Objects.equals(this.confirmedDateTime, cfarContract.confirmedDateTime) &&
Objects.equals(this.pnrReference, cfarContract.pnrReference) &&
Objects.equals(this.extAttributes, cfarContract.extAttributes);
}
@Override
public int hashCode() {
return Objects.hash(id, offers, reference, itinerary, coverage, coveragePercentage, premium, taxesTotal, taxes, currency, createdDateTime, expiryDateTime, status, confirmedDateTime, pnrReference, extAttributes);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CfarContract {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" reference: ").append(toIndentedString(reference)).append("\n");
sb.append(" offers: ").append(toIndentedString(offers)).append("\n");
sb.append(" itinerary: ").append(toIndentedString(itinerary)).append("\n");
sb.append(" coverage: ").append(toIndentedString(coverage)).append("\n");
sb.append(" coveragePercentage: ").append(toIndentedString(coveragePercentage)).append("\n");
sb.append(" premium: ").append(toIndentedString(premium)).append("\n");
sb.append(" taxes_total: ").append(toIndentedString(taxesTotal)).append("\n");
sb.append(" taxes: ").append(toIndentedString(taxes)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" createdDateTime: ").append(toIndentedString(createdDateTime)).append("\n");
sb.append(" expiryDateTime: ").append(toIndentedString(expiryDateTime)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" confirmedDateTime: ").append(toIndentedString(confirmedDateTime)).append("\n");
sb.append(" pnrReference: ").append(toIndentedString(pnrReference)).append("\n");
sb.append(" extAttributes: ").append(toIndentedString(extAttributes)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CfarContract.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CfarContract' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CfarContract.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CfarContract value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public CfarContract read(JsonReader in) throws IOException {
JsonObject jsonObj = elementAdapter.read(in).getAsJsonObject();
return thisAdapter.fromJsonTree(jsonObj);
}
}.nullSafe();
}
}
/**
* Create an instance of CfarContract given an JSON string
*
* @param jsonString JSON string
* @return An instance of CfarContract
* @throws IOException if the JSON string is invalid with respect to CfarContract
*/
public static CfarContract fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CfarContract.class);
}
/**
* Convert an instance of CfarContract to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy