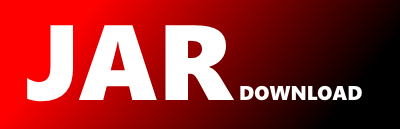
com.hopper.cloud.airlines.model.CfarContractExercise Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-airlines-java Show documentation
Show all versions of cloud-airlines-java Show documentation
Use the Hopper cloud airlines Web Services in Java!
The newest version!
/*
* Airline API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: v1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.hopper.cloud.airlines.model;
import java.time.OffsetDateTime;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import java.util.HashSet;
import java.util.Map.Entry;
import java.util.Set;
import com.hopper.cloud.airlines.JSON;
/**
* An object containing exercise information for a contract
*/
@ApiModel(description = "An object containing exercise information for a contract")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-06-28T12:18:49.517876+02:00[Europe/Paris]")
public class CfarContractExercise {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_CONTRACT_ID = "contract_id";
@SerializedName(SERIALIZED_NAME_CONTRACT_ID)
private String contractId;
public static final String SERIALIZED_NAME_EXERCISE_INITIATED_DATE_TIME = "exercise_initiated_date_time";
@SerializedName(SERIALIZED_NAME_EXERCISE_INITIATED_DATE_TIME)
private OffsetDateTime exerciseInitiatedDateTime;
public static final String SERIALIZED_NAME_CASH_REFUND_ALLOWANCE = "cash_refund_allowance";
@SerializedName(SERIALIZED_NAME_CASH_REFUND_ALLOWANCE)
private String cashRefundAllowance;
public static final String SERIALIZED_NAME_EXT_ATTRIBUTES = "ext_attributes";
@SerializedName(SERIALIZED_NAME_EXT_ATTRIBUTES)
private Map extAttributes = new HashMap<>();
public static final String SERIALIZED_NAME_FTC_REFUND_ALLOWANCE = "ftc_refund_allowance";
@SerializedName(SERIALIZED_NAME_FTC_REFUND_ALLOWANCE)
private String ftcRefundAllowance;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_REDIRECTION_TOKEN = "redirection_token";
@SerializedName(SERIALIZED_REDIRECTION_TOKEN)
private String redirectionToken;
public static final String SERIALIZED_NAME_REDIRECTION_URL = "redirection_url";
@SerializedName(SERIALIZED_NAME_REDIRECTION_URL)
private String redirectionUrl;
public CfarContractExercise() {
}
public CfarContractExercise id(String id) {
this.id = id;
return this;
}
/**
* Unique identifier for a CFAR exercise
* @return id
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "8ef454c6-74a5-48cf-972e-fac72d05e59c", required = true, value = "Unique identifier for a CFAR exercise")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public CfarContractExercise contractId(String contractId) {
this.contractId = contractId;
return this;
}
/**
* Unique identifier for a contract
* @return contractId
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "9f4f2f2b-adfd-4f02-83ad-da336ed57ce0", required = true, value = "Unique identifier for a contract")
public String getContractId() {
return contractId;
}
public void setContractId(String contractId) {
this.contractId = contractId;
}
public CfarContractExercise exerciseInitiatedDateTime(OffsetDateTime exerciseInitiatedDateTime) {
this.exerciseInitiatedDateTime = exerciseInitiatedDateTime;
return this;
}
/**
* A UTC [RFC3339](https://xml2rfc.tools.ietf.org/public/rfc/html/rfc3339.html#anchor14) datetime; the date and time at which a contract exercise was initiated
* @return exerciseInitiatedDateTime
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "2020-11-02T18:34:30Z", required = true, value = "A UTC [RFC3339](https://xml2rfc.tools.ietf.org/public/rfc/html/rfc3339.html#anchor14) datetime; the date and time at which a contract exercise was initiated")
public OffsetDateTime getExerciseInitiatedDateTime() {
return exerciseInitiatedDateTime;
}
public void setExerciseInitiatedDateTime(OffsetDateTime exerciseInitiatedDateTime) {
this.exerciseInitiatedDateTime = exerciseInitiatedDateTime;
}
public CfarContractExercise cashRefundAllowance(String cashRefundAllowance) {
this.cashRefundAllowance = cashRefundAllowance;
return this;
}
/**
* Refundable amount allowed in cash
* @return cashRefundAllowance
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Refundable amount allowed in cash")
public String getCashRefundAllowance() {
return cashRefundAllowance;
}
public void setCashRefundAllowance(String cashRefundAllowance) {
this.cashRefundAllowance = cashRefundAllowance;
}
public CfarContractExercise extAttributes(Map extAttributes) {
this.extAttributes = extAttributes;
return this;
}
public CfarContractExercise putExtAttributesItem(String key, String extAttributesItem) {
this.extAttributes.put(key, extAttributesItem);
return this;
}
/**
* Get extAttributes
* @return extAttributes
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public Map getExtAttributes() {
return extAttributes;
}
public void setExtAttributes(Map extAttributes) {
this.extAttributes = extAttributes;
}
public CfarContractExercise ftcRefundAllowance(String ftcRefundAllowance) {
this.ftcRefundAllowance = ftcRefundAllowance;
return this;
}
/**
* Get the refundable amount allowed in future travel credit
* @return ftcRefundAllowance
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Refundable amount allowed in future travel credit")
public String getFtcRefundAllowance() {
return ftcRefundAllowance;
}
public void setFtcRefundAllowance(String ftcRefundAllowance) {
this.ftcRefundAllowance = ftcRefundAllowance;
}
public CfarContractExercise currency(String currency) {
this.currency = currency;
return this;
}
/**
* Get currency
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "CAD", value = "")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public CfarContractExercise redirectionToken(String redirectionToken) {
this.redirectionToken = redirectionToken;
return this;
}
public String getRedirectionToken() {
return redirectionToken;
}
public void setRedirectionToken(String redirectionToken) {
this.redirectionToken = redirectionToken;
}
public CfarContractExercise redirectionUrl(String redirectionUrl) {
this.redirectionUrl = redirectionUrl;
return this;
}
/**
* URL on which the customer should be redirected to exercise (if applicable)
* @return redirectionUrl
**/
@ApiModelProperty(value = "URL on which the customer should be redirected to exercise (if applicable)")
public String getRedirectionUrl() {
return redirectionUrl;
}
public void setRedirectionUrl(String redirectionUrl) {
this.redirectionUrl = redirectionUrl;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CfarContractExercise cfarContractExercise = (CfarContractExercise) o;
return Objects.equals(this.id, cfarContractExercise.id) &&
Objects.equals(this.contractId, cfarContractExercise.contractId) &&
Objects.equals(this.exerciseInitiatedDateTime, cfarContractExercise.exerciseInitiatedDateTime) &&
Objects.equals(this.cashRefundAllowance, cfarContractExercise.cashRefundAllowance) &&
Objects.equals(this.extAttributes, cfarContractExercise.extAttributes) &&
Objects.equals(this.ftcRefundAllowance, cfarContractExercise.ftcRefundAllowance) &&
Objects.equals(this.redirectionToken, cfarContractExercise.redirectionToken)
&& Objects.equals(this.redirectionUrl, cfarContractExercise.redirectionUrl) &&
Objects.equals(this.currency, cfarContractExercise.currency);
}
@Override
public int hashCode() {
return Objects.hash(id, contractId, exerciseInitiatedDateTime, cashRefundAllowance, extAttributes, ftcRefundAllowance, redirectionToken, redirectionUrl, currency);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CfarContractExercise {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" contractId: ").append(toIndentedString(contractId)).append("\n");
sb.append(" exerciseInitiatedDateTime: ").append(toIndentedString(exerciseInitiatedDateTime)).append("\n");
sb.append(" cashRefundAllowance: ").append(toIndentedString(cashRefundAllowance)).append("\n");
sb.append(" extAttributes: ").append(toIndentedString(extAttributes)).append("\n");
sb.append(" ftcRefundAllowance: ").append(toIndentedString(ftcRefundAllowance)).append("\n");
sb.append(" redirectionToken: ").append(toIndentedString(redirectionToken)).append("\n");
sb.append(" redirectionUrl: ").append(toIndentedString(redirectionUrl)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CfarContractExercise.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CfarContractExercise' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CfarContractExercise.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CfarContractExercise value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public CfarContractExercise read(JsonReader in) throws IOException {
JsonObject jsonObj = elementAdapter.read(in).getAsJsonObject();
return thisAdapter.fromJsonTree(jsonObj);
}
}.nullSafe();
}
}
/**
* Create an instance of CfarContractExercise given an JSON string
*
* @param jsonString JSON string
* @return An instance of CfarContractExercise
* @throws IOException if the JSON string is invalid with respect to CfarContractExercise
*/
public static CfarContractExercise fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CfarContractExercise.class);
}
/**
* Convert an instance of CfarContractExercise to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy