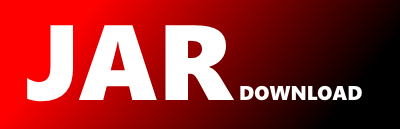
com.hopper.cloud.airlines.model.CfarItinerarySlice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-airlines-java Show documentation
Show all versions of cloud-airlines-java Show documentation
Use the Hopper cloud airlines Web Services in Java!
The newest version!
/*
* Airline API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: v1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.hopper.cloud.airlines.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.hopper.cloud.airlines.model.CfarItinerarySliceSegment;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import java.util.HashSet;
import java.util.Map.Entry;
import java.util.Set;
import com.hopper.cloud.airlines.JSON;
/**
* An object containing the list of flight segments for a fare slice
*/
@ApiModel(description = "An object containing the list of flight segments for a fare slice")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-06-28T12:18:49.517876+02:00[Europe/Paris]")
@JsonInclude(JsonInclude.Include. NON_NULL)
public class CfarItinerarySlice {
public static final String SERIALIZED_NAME_SEGMENTS = "segments";
@SerializedName(SERIALIZED_NAME_SEGMENTS)
private List segments = new ArrayList<>();
public static final String SERIALIZED_NAME_PASSENGER_PRICING = "passenger_pricing";
@SerializedName(SERIALIZED_NAME_PASSENGER_PRICING)
private List passengerPricing = null;
public static final String SERIALIZED_NAME_TOTAL_PRICE = "total_price";
@SerializedName(SERIALIZED_NAME_TOTAL_PRICE)
private String totalPrice;
public static final String SERIALIZED_NAME_FARE_BRAND = "fare_brand";
@SerializedName(SERIALIZED_NAME_FARE_BRAND)
private String fareBrand;
public static final String SERIALIZED_NAME_FARE_BASIS = "fare_basis";
@SerializedName(SERIALIZED_NAME_FARE_BASIS)
private String fareBasis;
public static final String SERIALIZED_NAME_FARE_RULES = "fare_rules";
@SerializedName(SERIALIZED_NAME_FARE_RULES)
private List fareRules = null;
public static final String SERIALIZED_NAME_OTHER_FARES = "other_fares";
@SerializedName(SERIALIZED_NAME_OTHER_FARES)
private List otherFares = null;
public CfarItinerarySlice() {
}
public CfarItinerarySlice segments(List segments) {
this.segments = segments;
return this;
}
public CfarItinerarySlice addSegmentsItem(CfarItinerarySliceSegment segmentsItem) {
this.segments.add(segmentsItem);
return this;
}
/**
* A list of segments which make up the slice of the fare
* @return segments
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "A list of segments which make up the slice of the fare")
public List getSegments() {
return segments;
}
public void setSegments(List segments) {
this.segments = segments;
}
public CfarItinerarySlice passengerPricing(List passengerPricing) {
this.passengerPricing = passengerPricing;
return this;
}
public CfarItinerarySlice addPassengerPricingItem(PassengerPricing passengerPricingItem) {
this.passengerPricing.add(passengerPricingItem);
return this;
}
/**
* List of passengers type, count and pricing for the slice
* @return passengerPricing
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "List of passengers type, count and pricing for the slice")
public List getPassengerPricing() {
return passengerPricing;
}
public void setPassengerPricing(List passengerPricing) {
this.passengerPricing = passengerPricing;
}
public CfarItinerarySlice totalPrice(String totalPrice) {
this.totalPrice = totalPrice;
return this;
}
/**
* The price of the slice for all the passengers
* @return totalPrice
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "401.10", required = true, value = "The price of the slice for all the passengers")
public String getTotalPrice() {
return totalPrice;
}
public void setTotalPrice(String totalPrice) {
this.totalPrice = totalPrice;
}
public CfarItinerarySlice fareBrand(String fareBrand) {
this.fareBrand = fareBrand;
return this;
}
/**
* Get fareBrand
* @return fareBrand
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = false, value = "")
public String getFareBrand() {
return fareBrand;
}
public void setFareBrand(String fareBrand) {
this.fareBrand = fareBrand;
}
public CfarItinerarySlice fareBasis(String fareBasis) {
this.fareBasis = fareBasis;
return this;
}
/**
* Code of the fare basis applied to the slice
* @return fareBasis
**/
@ApiModelProperty(required = false, value = "Code of the fare basis applied to the slice")
public String getFareBasis() {
return fareBasis;
}
public void setFareBasis(String fareBasis) {
this.fareBasis = fareBasis;
}
public CfarItinerarySlice fareRules(List fareRules) {
this.fareRules = fareRules;
return this;
}
public CfarItinerarySlice addFareRulesItem(FareRule fareRuleItem) {
if (this.fareRules == null) {
this.fareRules = new ArrayList<>();
}
this.fareRules.add(fareRuleItem);
return this;
}
/**
* The fare rules associated to the slice
* @return fareRules
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The fare rules associated to the slice")
public List getFareRules() {
return fareRules;
}
public void setFareRules(List fareRules) {
this.fareRules = fareRules;
}
public CfarItinerarySlice otherFares(List otherFares) {
this.otherFares = otherFares;
return this;
}
public CfarItinerarySlice addOtherFaresItem(Fare otherFareItem) {
if (this.otherFares == null) {
this.otherFares = new ArrayList<>();
}
this.otherFares.add(otherFareItem);
return this;
}
/**
* Other available fares in the same cabin
* @return fareRules
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Other available fares in the same cabin")
public List getOtherFares() {
return otherFares;
}
public void setOtherFares(List otherFares) {
this.otherFares = otherFares;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CfarItinerarySlice cfarItinerarySlice = (CfarItinerarySlice) o;
return Objects.equals(this.segments, cfarItinerarySlice.segments) &&
Objects.equals(this.passengerPricing, cfarItinerarySlice.passengerPricing) &&
Objects.equals(this.totalPrice, cfarItinerarySlice.totalPrice) &&
Objects.equals(this.fareBrand, cfarItinerarySlice.fareBrand) &&
Objects.equals(this.fareBasis, cfarItinerarySlice.fareBasis) &&
Objects.equals(this.fareRules, cfarItinerarySlice.fareRules) &&
Objects.equals(this.otherFares, cfarItinerarySlice.otherFares);
}
@Override
public int hashCode() {
return Objects.hash(segments, passengerPricing, totalPrice, fareBrand, fareBasis, fareRules, otherFares);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CfarItinerarySlice {\n");
sb.append(" segments: ").append(toIndentedString(segments)).append("\n");
sb.append(" passengerPricing: ").append(toIndentedString(passengerPricing)).append("\n");
sb.append(" totalPrice: ").append(toIndentedString(totalPrice)).append("\n");
sb.append(" fareBrand: ").append(toIndentedString(fareBrand)).append("\n");
sb.append(" fareBasis: ").append(toIndentedString(fareBasis)).append("\n");
sb.append(" fareRules: ").append(toIndentedString(fareRules)).append("\n");
sb.append(" otherFares: ").append(toIndentedString(otherFares)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CfarItinerarySlice.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CfarItinerarySlice' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CfarItinerarySlice.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CfarItinerarySlice value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public CfarItinerarySlice read(JsonReader in) throws IOException {
JsonObject jsonObj = elementAdapter.read(in).getAsJsonObject();
return thisAdapter.fromJsonTree(jsonObj);
}
}.nullSafe();
}
}
/**
* Create an instance of CfarItinerarySlice given an JSON string
*
* @param jsonString JSON string
* @return An instance of CfarItinerarySlice
* @throws IOException if the JSON string is invalid with respect to CfarItinerarySlice
*/
public static CfarItinerarySlice fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CfarItinerarySlice.class);
}
/**
* Convert an instance of CfarItinerarySlice to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy