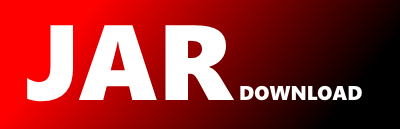
com.hopper.cloud.airlines.model.CfarItinerarySliceSegment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-airlines-java Show documentation
Show all versions of cloud-airlines-java Show documentation
Use the Hopper cloud airlines Web Services in Java!
The newest version!
/*
* Airline API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: v1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.hopper.cloud.airlines.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.hopper.cloud.airlines.model.FareClass;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import java.util.HashSet;
import java.util.Map.Entry;
import java.util.Set;
import com.hopper.cloud.airlines.JSON;
/**
* An object detailing a segment of a fare slice
*/
@ApiModel(description = "An object detailing a segment of a fare slice")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-06-28T12:18:49.517876+02:00[Europe/Paris]")
@JsonInclude(JsonInclude.Include. NON_NULL)
public class CfarItinerarySliceSegment {
public static final String SERIALIZED_NAME_ORIGIN_AIRPORT = "origin_airport";
@SerializedName(SERIALIZED_NAME_ORIGIN_AIRPORT)
private String originAirport;
public static final String SERIALIZED_NAME_DESTINATION_AIRPORT = "destination_airport";
@SerializedName(SERIALIZED_NAME_DESTINATION_AIRPORT)
private String destinationAirport;
public static final String SERIALIZED_NAME_DEPARTURE_DATE_TIME = "departure_date_time";
@SerializedName(SERIALIZED_NAME_DEPARTURE_DATE_TIME)
private String departureDateTime;
public static final String SERIALIZED_NAME_ARRIVAL_DATE_TIME = "arrival_date_time";
@SerializedName(SERIALIZED_NAME_ARRIVAL_DATE_TIME)
private String arrivalDateTime;
public static final String SERIALIZED_NAME_FLIGHT_NUMBER = "flight_number";
@SerializedName(SERIALIZED_NAME_FLIGHT_NUMBER)
private String flightNumber;
public static final String SERIALIZED_NAME_VALIDATING_CARRIER_CODE = "validating_carrier_code";
@SerializedName(SERIALIZED_NAME_VALIDATING_CARRIER_CODE)
private String validatingCarrierCode;
public static final String SERIALIZED_NAME_FARE_CLASS = "fare_class";
@SerializedName(SERIALIZED_NAME_FARE_CLASS)
private FareClass fareClass;
public static final String SERIALIZED_NAME_FARE_BRAND = "fare_brand";
@SerializedName(SERIALIZED_NAME_FARE_BRAND)
private String fareBrand;
public CfarItinerarySliceSegment() {
}
public CfarItinerarySliceSegment originAirport(String originAirport) {
this.originAirport = originAirport;
return this;
}
/**
* IATA airport code of origin
* @return originAirport
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "LGA", required = true, value = "IATA airport code of origin")
public String getOriginAirport() {
return originAirport;
}
public void setOriginAirport(String originAirport) {
this.originAirport = originAirport;
}
public CfarItinerarySliceSegment destinationAirport(String destinationAirport) {
this.destinationAirport = destinationAirport;
return this;
}
/**
* IATA airport code of destination
* @return destinationAirport
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "BOS", required = true, value = "IATA airport code of destination")
public String getDestinationAirport() {
return destinationAirport;
}
public void setDestinationAirport(String destinationAirport) {
this.destinationAirport = destinationAirport;
}
public CfarItinerarySliceSegment departureDateTime(String departureDateTime) {
this.departureDateTime = departureDateTime;
return this;
}
/**
* The local date and time of departure in ISO Local Date Time format
* @return departureDateTime
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "2020-11-02T11:34:30", required = true, value = "The local date and time of departure in ISO Local Date Time format")
public String getDepartureDateTime() {
return departureDateTime;
}
public void setDepartureDateTime(String departureDateTime) {
this.departureDateTime = departureDateTime;
}
public CfarItinerarySliceSegment arrivalDateTime(String arrivalDateTime) {
this.arrivalDateTime = arrivalDateTime;
return this;
}
/**
* The local date and time of arrival in ISO Local Date Time format
* @return arrivalDateTime
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "2020-11-02T12:12:30", required = true, value = "The local date and time of arrival in ISO Local Date Time format")
public String getArrivalDateTime() {
return arrivalDateTime;
}
public void setArrivalDateTime(String arrivalDateTime) {
this.arrivalDateTime = arrivalDateTime;
}
public CfarItinerarySliceSegment flightNumber(String flightNumber) {
this.flightNumber = flightNumber;
return this;
}
/**
* The number of the flight
* @return flightNumber
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "JB776", required = true, value = "The number of the flight")
public String getFlightNumber() {
return flightNumber;
}
public void setFlightNumber(String flightNumber) {
this.flightNumber = flightNumber;
}
public CfarItinerarySliceSegment validatingCarrierCode(String validatingCarrierCode) {
this.validatingCarrierCode = validatingCarrierCode;
return this;
}
/**
* The IATA airline code of the validating carrier for this segment
* @return validatingCarrierCode
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "B6", required = true, value = "The IATA airline code of the validating carrier for this segment")
public String getValidatingCarrierCode() {
return validatingCarrierCode;
}
public void setValidatingCarrierCode(String validatingCarrierCode) {
this.validatingCarrierCode = validatingCarrierCode;
}
public CfarItinerarySliceSegment fareClass(FareClass fareClass) {
this.fareClass = fareClass;
return this;
}
/**
* Get fareClass
* @return fareClass
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public FareClass getFareClass() {
return fareClass;
}
public void setFareClass(FareClass fareClass) {
this.fareClass = fareClass;
}
public CfarItinerarySliceSegment fareBrand(String fareBrand) {
this.fareBrand = fareBrand;
return this;
}
/**
* Get fareBrand
* @return fareBrand
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = false, value = "")
public String getFareBrand() {
return fareBrand;
}
public void setFareBrand(String fareBrand) {
this.fareBrand = fareBrand;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CfarItinerarySliceSegment cfarItinerarySliceSegment = (CfarItinerarySliceSegment) o;
return Objects.equals(this.originAirport, cfarItinerarySliceSegment.originAirport) &&
Objects.equals(this.destinationAirport, cfarItinerarySliceSegment.destinationAirport) &&
Objects.equals(this.departureDateTime, cfarItinerarySliceSegment.departureDateTime) &&
Objects.equals(this.arrivalDateTime, cfarItinerarySliceSegment.arrivalDateTime) &&
Objects.equals(this.flightNumber, cfarItinerarySliceSegment.flightNumber) &&
Objects.equals(this.validatingCarrierCode, cfarItinerarySliceSegment.validatingCarrierCode) &&
Objects.equals(this.fareClass, cfarItinerarySliceSegment.fareClass) &&
Objects.equals(this.fareBrand, cfarItinerarySliceSegment.fareBrand);
}
@Override
public int hashCode() {
return Objects.hash(originAirport, destinationAirport, departureDateTime, arrivalDateTime, flightNumber, validatingCarrierCode, fareClass, fareBrand);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CfarItinerarySliceSegment {\n");
sb.append(" originAirport: ").append(toIndentedString(originAirport)).append("\n");
sb.append(" destinationAirport: ").append(toIndentedString(destinationAirport)).append("\n");
sb.append(" departureDateTime: ").append(toIndentedString(departureDateTime)).append("\n");
sb.append(" arrivalDateTime: ").append(toIndentedString(arrivalDateTime)).append("\n");
sb.append(" flightNumber: ").append(toIndentedString(flightNumber)).append("\n");
sb.append(" validatingCarrierCode: ").append(toIndentedString(validatingCarrierCode)).append("\n");
sb.append(" fareClass: ").append(toIndentedString(fareClass)).append("\n");
sb.append(" fareBrand: ").append(toIndentedString(fareBrand)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!CfarItinerarySliceSegment.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CfarItinerarySliceSegment' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(CfarItinerarySliceSegment.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, CfarItinerarySliceSegment value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public CfarItinerarySliceSegment read(JsonReader in) throws IOException {
JsonObject jsonObj = elementAdapter.read(in).getAsJsonObject();
return thisAdapter.fromJsonTree(jsonObj);
}
}.nullSafe();
}
}
/**
* Create an instance of CfarItinerarySliceSegment given an JSON string
*
* @param jsonString JSON string
* @return An instance of CfarItinerarySliceSegment
* @throws IOException if the JSON string is invalid with respect to CfarItinerarySliceSegment
*/
public static CfarItinerarySliceSegment fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, CfarItinerarySliceSegment.class);
}
/**
* Convert an instance of CfarItinerarySliceSegment to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy