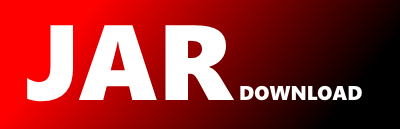
com.hopper.cloud.airlines.model.PaymentCardDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-airlines-java Show documentation
Show all versions of cloud-airlines-java Show documentation
Use the Hopper cloud airlines Web Services in Java!
The newest version!
/*
* Airline API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: v1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.hopper.cloud.airlines.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.google.gson.*;
import com.google.gson.annotations.SerializedName;
import com.google.gson.reflect.TypeToken;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.hopper.cloud.airlines.JSON;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.Objects;
/**
* An object giving details of a payment card"
*/
@ApiModel(description = "An object giving details of a payment card")
@JsonInclude(JsonInclude.Include.NON_NULL)
public class PaymentCardDetails {
public static final String SERIALIZED_NAME_NUMBER = "number";
@SerializedName(SERIALIZED_NAME_NUMBER)
private String number = null;
public static final String SERIALIZED_NAME_EXPIRATION_MONTH = "expiration_month";
@SerializedName(SERIALIZED_NAME_EXPIRATION_MONTH)
private String expirationMonth = null;
public static final String SERIALIZED_NAME_EXPIRATION_YEAR = "expiration_year";
@SerializedName(SERIALIZED_NAME_EXPIRATION_YEAR)
private String expirationYear = null;
public static final String SERIALIZED_NAME_FIRST_NAME = "first_name";
@SerializedName(SERIALIZED_NAME_FIRST_NAME)
private String firstName = null;
public static final String SERIALIZED_NAME_LAST_NAME = "last_name";
@SerializedName(SERIALIZED_NAME_LAST_NAME)
private String lastName = null;
public PaymentCardDetails() {
}
public PaymentCardDetails number(String number) {
this.number = number;
return this;
}
/**
* The full card number
* @return number
**/
@ApiModelProperty(value = "The full card number")
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public PaymentCardDetails expirationMonth(String expirationMonth) {
this.expirationMonth = expirationMonth;
return this;
}
/**
* The expiration month of the card
* @return expirationMonth
**/
@ApiModelProperty(value = "The expiration month of the card")
public String getExpirationMonth() {
return expirationMonth;
}
public void setExpirationMonth(String expirationMonth) {
this.expirationMonth = expirationMonth;
}
public PaymentCardDetails expirationYear(String expirationYear) {
this.expirationYear = expirationYear;
return this;
}
/**
* The expiration year of the card
* @return expirationYear
**/
@ApiModelProperty(value = "The expiration year of the card")
public String getExpirationYear() {
return expirationYear;
}
public void setExpirationYear(String expirationYear) {
this.expirationYear = expirationYear;
}
public PaymentCardDetails firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* The first name of the cardholder
* @return firstName
**/
@ApiModelProperty(value = "The first name of the cardholder")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public PaymentCardDetails lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* The last name of the cardholder
* @return lastName
**/
@ApiModelProperty(value = "The last name of the cardholder")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentCardDetails paymentCardDetails = (PaymentCardDetails) o;
return Objects.equals(this.number, paymentCardDetails.number) &&
Objects.equals(this.expirationMonth, paymentCardDetails.expirationMonth) &&
Objects.equals(this.expirationYear, paymentCardDetails.expirationYear) &&
Objects.equals(this.firstName, paymentCardDetails.firstName) &&
Objects.equals(this.lastName, paymentCardDetails.lastName);
}
@Override
public int hashCode() {
return Objects.hash(number, expirationMonth, expirationYear, firstName, lastName);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreditCardDetail {\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" expirationMonth: ").append(toIndentedString(expirationMonth)).append("\n");
sb.append(" expirationYear: ").append(toIndentedString(expirationYear)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!PaymentCardDetails.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'CreditCardDetail' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(PaymentCardDetails.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, PaymentCardDetails value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public PaymentCardDetails read(JsonReader in) throws IOException {
JsonObject jsonObj = elementAdapter.read(in).getAsJsonObject();
return thisAdapter.fromJsonTree(jsonObj);
}
}.nullSafe();
}
}
/**
* Create an instance of CreditCardDetail given an JSON string
*
* @param jsonString JSON string
* @return An instance of CreditCardDetail
* @throws IOException if the JSON string is invalid with respect to CreditCardDetail
*/
public static PaymentCardDetails fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, PaymentCardDetails.class);
}
/**
* Convert an instance of CreditCardDetail to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy