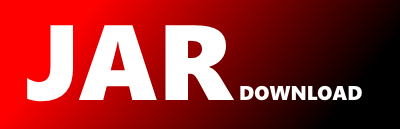
com.hotels.bdp.circustrain.api.conf.TableReplication Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) ${license.git.copyrightYears} Expedia, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hotels.bdp.circustrain.api.conf;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import javax.validation.Valid;
import javax.validation.constraints.NotNull;
import com.google.common.collect.ImmutableMap;
import com.hotels.bdp.circustrain.api.validation.constraints.TableReplicationFullReplicationModeConstraint;
@TableReplicationFullReplicationModeConstraint
public class TableReplication {
private @Valid @NotNull SourceTable sourceTable;
private @Valid @NotNull ReplicaTable replicaTable;
private Map copierOptions;
private Map transformOptions = new HashMap<>();
private short partitionIteratorBatchSize = (short) 1000;
private short partitionFetcherBufferSize = (short) 1000;
private @NotNull ReplicationMode replicationMode = ReplicationMode.FULL;
private @NotNull ReplicationStrategy replicationStrategy = ReplicationStrategy.UPSERT;
private @NotNull OrphanedDataStrategy orphanedDataStrategy = OrphanedDataStrategy.HOUSEKEEPING;
// Only relevant to view replications
private Map tableMappings;
public SourceTable getSourceTable() {
return sourceTable;
}
public void setSourceTable(SourceTable sourceTable) {
this.sourceTable = sourceTable;
}
public ReplicaTable getReplicaTable() {
return replicaTable;
}
public void setReplicaTable(ReplicaTable replicaTable) {
this.replicaTable = replicaTable;
}
public Map getCopierOptions() {
return copierOptions;
}
public Map getMergedCopierOptions(Map baseCopierOptions) {
return getMergedCopierOptions(baseCopierOptions, getCopierOptions());
}
public static Map getMergedCopierOptions(
Map baseCopierOptions,
Map overrideCopierOptions) {
Map mergedCopierOptions = new HashMap<>();
if (baseCopierOptions != null) {
mergedCopierOptions.putAll(baseCopierOptions);
}
if (overrideCopierOptions != null) {
mergedCopierOptions.putAll(overrideCopierOptions);
}
return ImmutableMap.copyOf(mergedCopierOptions);
}
public void setCopierOptions(Map copierOptions) {
this.copierOptions = copierOptions;
}
public Map getTransformOptions() {
return transformOptions;
}
public void setTransformOptions(Map transformOptions) {
this.transformOptions = transformOptions;
}
public String getReplicaDatabaseName() {
SourceTable sourceTable = getSourceTable();
ReplicaTable replicaTable = getReplicaTable();
String databaseName = replicaTable.getDatabaseName() != null ? replicaTable.getDatabaseName()
: sourceTable.getDatabaseName();
return databaseName.toLowerCase(Locale.ROOT);
}
public String getReplicaTableName() {
SourceTable sourceTable = getSourceTable();
ReplicaTable replicaTable = getReplicaTable();
String tableNameName = replicaTable.getTableName() != null ? replicaTable.getTableName()
: sourceTable.getTableName();
return tableNameName.toLowerCase(Locale.ROOT);
}
public String getQualifiedReplicaName() {
return getReplicaDatabaseName() + "." + getReplicaTableName();
}
public short getPartitionIteratorBatchSize() {
return partitionIteratorBatchSize;
}
public void setPartitionIteratorBatchSize(short partitionIteratorBatchSize) {
this.partitionIteratorBatchSize = partitionIteratorBatchSize;
}
public short getPartitionFetcherBufferSize() {
return partitionFetcherBufferSize;
}
public void setPartitionFetcherBufferSize(short partitionFetcherBufferSize) {
this.partitionFetcherBufferSize = partitionFetcherBufferSize;
}
public ReplicationMode getReplicationMode() {
return replicationMode;
}
public void setReplicationMode(ReplicationMode replicationMode) {
this.replicationMode = replicationMode;
}
public Map getTableMappings() {
return tableMappings;
}
public void setTableMappings(Map tableMappings) {
this.tableMappings = tableMappings;
}
public ReplicationStrategy getReplicationStrategy() {
return replicationStrategy;
}
public void setReplicationStrategy(ReplicationStrategy replicationStrategy) {
this.replicationStrategy = replicationStrategy;
}
public OrphanedDataStrategy getOrphanedDataStrategy() {
return orphanedDataStrategy;
}
public void setOrphanedDataStrategy(OrphanedDataStrategy orphanedDataStrategy) {
this.orphanedDataStrategy = orphanedDataStrategy;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy