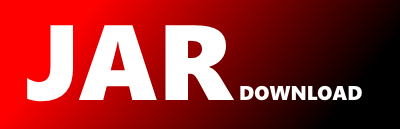
com.hp.autonomy.aci.content.identifier.IndexingIdentifiers Maven / Gradle / Ivy
Show all versions of content-parameter-api Show documentation
/*
* Copyright 2009-2015 Hewlett-Packard Development Company, L.P.
* Licensed under the MIT License (the "License"); you may not use this file except in compliance with the License.
*/
package com.hp.autonomy.aci.content.identifier;
/**
* {@code IndexingIdentifiers} are document identifiers that can be used in the data of a DREREPLACE. e.g.:
*
*
* IndexingIdentifiers identifiers = ...;
*
* // Build up the first line of the replace body
* StringBuilder dreReplace = new StringBuilder()
* .append('#')
* .append(identifiers.getIndexingIdentifierName())
* .append(' ')
* .append(identifiers.toIndexingString())
* .append('\n');
*
*/
public interface IndexingIdentifiers {
String DREALL = "DREALL";
String DREDOCID = "DREDOCID";
String DREDBNAME = "DREDBNAME";
String DREDOCREF = "DREDOCREF";
String DRESTATEID = "DRESTATEID";
/**
* {@code IndexingIdentifier} to represent DREALL, which can be reused to replace fields on all the
* documents in an engine. This might be used when purging a particular field-value pair from an entire engine
* without the need to explicitly find which documents have that value.
*
* This object is immutable.
*/
IndexingIdentifiers ALL = new IndexingIdentifiers() {
@Override
public String toIndexingString() {
return "";
}
@Override
public String getIndexingIdentifierName() {
return DREALL;
}
@Override
public boolean isEmpty() {
return false;
}
};
/**
* Whether or not the identifier is empty.
*
*
An empty identifier is one that cannot correspond to any documents, e.g. an empty reference list. Under some
* circumstances it can be unsafe to use an empty identifier as it could match all documents, rather than no
* documents. Though the classes in this API attempt to cope with this contingency as smoothly as possible (using
* dummy values and throwing exceptions as appropriate), it is usually preferable to do an empty check prior to
* using an identifier and handling that case separately.
*
* @return {@code true} if the identifier is empty
*/
boolean isEmpty();
/**
* The string representation of this identifier, suitable for use as part of a DREREPLACE.
*
*
Though the {@code IndexingIdentifiers} interface does not prescribe an implementation of the
* {@link Object#toString()} method, in practice it usually gives an ACI-ready version of the identifier. In most
* cases this will be the same as the indexing version but care should be taken to use the correct method as
* differences between the strings can cause subtly incorrect behaviour.
*
* @return A DREREPLACE identifier
*/
String toIndexingString();
/**
* The identifier name, as used in DREREPLACE. It will not include the leading #. e.g. DREDOCREF.
*
* @return A DREREPLACE identifier name
*/
String getIndexingIdentifierName();
}