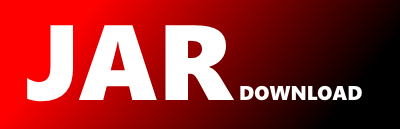
com.hp.autonomy.aci.content.fieldtext.AbstractFieldText Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of content-parameter-api Show documentation
Show all versions of content-parameter-api Show documentation
API for representing aspects of IDOL's Content server, including FieldText and document References
The newest version!
/*
* Copyright 2009-2017 Open Text.
*
* Licensed under the MIT License (the "License"); you may not use this file
* except in compliance with the License.
*
* The only warranties for products and services of Open Text and its affiliates
* and licensors ("Open Text") are as may be set forth in the express warranty
* statements accompanying such products and services. Nothing herein should be
* construed as constituting an additional warranty. Open Text shall not be
* liable for technical or editorial errors or omissions contained herein. The
* information contained herein is subject to change without notice.
*/
package com.hp.autonomy.aci.content.fieldtext;
/**
* An abstract base-class that partially implements the {@link FieldText} interface using sensible defaults. Under
* specific circumstances, alternatives exist to subclassing {@code AbstractFieldText} directly:
*
*
* - Subclassing {@link Specifier} for an individual fieldtext specifier.
*
- Making an immutable singleton, wrapped by {@link FieldTextWrapper}, if the expression is a constant.
*
*
*/
public abstract class AbstractFieldText implements FieldText {
/**
* Appends another fieldtext expression onto the end of this expression using the AND operator. The returned
* {@link FieldText} object is equivalent to:
*
* (this) AND (fieldText)
*
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
*/
@Override
public FieldText AND(final FieldText fieldText) {
return new FieldTextBuilder(this).AND(fieldText);
}
/**
* Appends another fieldtext expression onto the end of this expression using the OR operator. The returned
* {@link FieldText} object is equivalent to:
*
* (this) OR (fieldText)
*
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
*/
@Override
public FieldText OR(final FieldText fieldText) {
return new FieldTextBuilder(this).OR(fieldText);
}
/**
* Applies a NOT operator to this fieldtext. The returned {@link FieldText} object is equivalent to:
*
* NOT (this)
*
* @return The negated fieldtext.
*/
@Override
public FieldText NOT() {
return new FieldTextBuilder(this).NOT();
}
/**
* Appends another fieldtext expression onto the end of this expression using the XOR operator. The returned
* {@link FieldText} object is equivalent to:
*
* (this) XOR (fieldText)
*
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
*/
@Override
public FieldText XOR(final FieldText fieldText) {
return new FieldTextBuilder(this).XOR(fieldText);
}
/**
* Appends another fieldtext expression onto the end of this expression using the WHEN operator. The returned
* {@link FieldText} object is equivalent to:
*
* (this) WHEN (fieldText)
*
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
*/
@Override
public FieldText WHEN(final FieldText fieldText) {
return new FieldTextBuilder(this).WHEN(fieldText);
}
/**
* Appends another fieldtext expression onto the end of this expression using the WHENn operator. The
* returned {@link FieldText} object is equivalent to:
*
* (this) WHENn (fieldText)
*
* @param depth The n in WHENn
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
*/
@Override
public FieldText WHEN(final int depth, final FieldText fieldText) {
return new FieldTextBuilder(this).WHEN(depth, fieldText);
}
/**
* Simple implementation that tests for emptiness using {@code size() == 0}. In most cases this should be
* overridden with a better implementation.
*
* @return {@code true} if and only if size is {@code 0}.
*/
@Override
public boolean isEmpty() {
return size() == 0;
}
/**
* Tests for equality of this object with another {@link FieldText} object.
*
* @param obj An object to test for equality.
* @return {@code true} if and only if obj is a {@link FieldText} object with the same toString()
* value as this object.
*/
@Override
public boolean equals(final Object obj) {
return this == obj || obj instanceof FieldText && toString().equals(obj.toString());
}
/**
* A suitable hash code based on the toString() method.
*
* @return A hash code.
*/
@Override
public int hashCode() {
return toString().hashCode();
}
/**
* Should return the {@code String} representation of the FieldText expression, exactly as it should be sent to
* IDOL.
*
* @return The {@code String} representation.
*/
@Override
public abstract String toString();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy