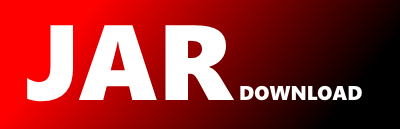
com.hp.autonomy.aci.content.fieldtext.FieldText Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of content-parameter-api Show documentation
Show all versions of content-parameter-api Show documentation
API for representing aspects of IDOL's Content server, including FieldText and document References
The newest version!
/*
* Copyright 2009-2015 Open Text.
*
* Licensed under the MIT License (the "License"); you may not use this file
* except in compliance with the License.
*
* The only warranties for products and services of Open Text and its affiliates
* and licensors ("Open Text") are as may be set forth in the express warranty
* statements accompanying such products and services. Nothing herein should be
* construed as constituting an additional warranty. Open Text shall not be
* liable for technical or editorial errors or omissions contained herein. The
* information contained herein is subject to change without notice.
*/
package com.hp.autonomy.aci.content.fieldtext;
/**
* Interface for representing IDOL FieldText expressions. These expressions are made up of specifiers combined with AND,
* OR, XOR, NOT and brackets. e.g.
*
*
* MATCH{xls,doc}:EXT+AND+EQUAL{4}:TAG
*
*
* The {@link FieldTextBuilder} class can be used to combine FieldText specifiers into more complex expressions.
* However, it is often unnecessary to explicitly instantiate a {@link FieldTextBuilder} as the {@code FieldText}
* interface forces all specifiers to provide support for combining expressions.
*
* The {@link Specifier} class provides both a generic object for representing a specifier as well as being a suitable
* base class for other specifiers.
*
* Example:
*
*
* FieldText ft = new MATCH("EXT", "xls", "doc").AND(new EQUAL("TAG", 4));
* String fieldText = ft.toString();
*
*
*/
public interface FieldText {
/**
* Combines two FieldText expressions ({@code this} and {@code fieldText}) using the AND operator. This should
* return a {@code FieldText} object equivalent to:
*
* (this) AND (fieldText)
*
* Calling this method might alter the {@code FieldText} object on which it is called.
*
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
*/
FieldText AND(FieldText fieldText);
/**
* Combines two FieldText expressions ({@code this} and {@code fieldText}) using the OR operator. This should return
* a {@code FieldText} object equivalent to:
*
* (this) OR (fieldText)
*
* Calling this method might alter the {@code FieldText} object on which it is called.
*
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
*/
FieldText OR(FieldText fieldText);
/**
* Applies a NOT operator to this FieldText expression. This should return a {@code FieldText} object equivalent to:
*
* NOT (this)
*
* Calling this method might alter the {@code FieldText} object on which it is called.
*
* @return The negated expression.
*/
FieldText NOT();
/**
* Combines two FieldText expressions ({@code this} and {@code fieldText}) using the XOR operator. This should
* return a {@code FieldText} object equivalent to:
*
* (this) XOR (fieldText)
*
* Calling this method might alter the {@code FieldText} object on which it is called.
*
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
*/
FieldText XOR(FieldText fieldText);
// Currently IDOL doesn't support chaining BEFOREs/AFTERs, which makes them tricky to implement well. Until someone
// requests them we'll leave them out for now
//
// FieldText BEFORE(FieldText fieldText);
// FieldText AFTER(FieldText fieldText);
/*
* Combines two FieldText specifiers ({@code this} and {@code fieldText}) using the WHEN operator. Both specifiers
* must have a size of 1 or an exception will be thrown. The returned {@code FieldText} object will be equivalent
* to:
*
* this WHEN fieldText
*
* Calling this method might alter the {@code FieldText} object on which it is called.
*
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
* @throws IllegalStateException If {@code this} does not have a size of 1
* @throws IllegalArgumentException If {@code fieldText} does not have a size of 1
*/
FieldText WHEN(FieldText fieldText);
/*
* Combines two FieldText expressions (this and fieldText)
* using the WHENn operator. This should return a FieldText object
* equivalent to:
*
* (this) WHENn (fieldText)
*
* Calling this method might alter the FieldText object on which it
* is called.
*
* @param depth The n in WHENn
* @param fieldText A fieldtext expression or specifier.
* @return The combined expression.
* @throws IllegalStateException If {@code this} does not have a size of 1
* @throws IllegalArgumentException If {@code fieldText} does not have a size of 1
*/
FieldText WHEN(int depth, FieldText fieldText);
/**
* The size is defined as the number of specifiers in the FieldText expression. An individual specifier would have a
* size of 1.
*
* @return The size of the expression.
*/
int size();
/**
* A {@code FieldText} object is empty if its size is zero.
*
* @return {@code true} if and only if size is 0.
*/
boolean isEmpty();
/**
* Should return the {@code String} representation of the FieldText expression, exactly as it should be sent to
* IDOL.
*
* @return The {@code String} representation.
*/
@Override
String toString();
/**
* {@code FieldText} objects are considered equal if their {@code String} representations are equal.
*
* @param obj An object to test for equality.
* @return {@code true} if and only if {@code obj} is a {@code FieldText} object with the same {@code toString()}
* value as this object.
*/
@Override
boolean equals(Object obj);
/**
* The hashcode should be that of the {@code String} representation.
*
* @return The hashcode of the {@code String} representation.
*/
@Override
int hashCode();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy