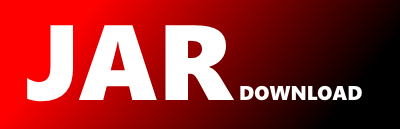
com.hp.autonomy.frontend.configuration.redis.RedisConfig Maven / Gradle / Ivy
/*
* Copyright 2013-2015 Hewlett-Packard Development Company, L.P.
* Licensed under the MIT License (the "License"); you may not use this file except in compliance with the License.
*/
package com.hp.autonomy.frontend.configuration.redis;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.hp.autonomy.frontend.configuration.ConfigException;
import com.hp.autonomy.frontend.configuration.SimpleComponent;
import com.hp.autonomy.frontend.configuration.server.HostAndPort;
import lombok.Builder;
import lombok.Getter;
import lombok.Singular;
import lombok.ToString;
import org.apache.commons.lang.StringUtils;
import java.util.Collection;
/**
* Configuration for a Redis server. This allows both a single Redis or a Redis Sentinel configuration
*/
@SuppressWarnings({"WeakerAccess", "InstanceVariableOfConcreteClass", "MismatchedQueryAndUpdateOfCollection"})
@Getter
@Builder
@ToString
@JsonDeserialize(builder = RedisConfig.RedisConfigBuilder.class)
public class RedisConfig extends SimpleComponent {
private static final String CONFIG_SECTION = "redis";
private final String masterName;
private final String password;
private final HostAndPort address;
@Singular
private final Collection sentinels;
private final Integer database;
/**
* If true, indicates that application should configure redis. Otherwise, the application can assume that redis has
* been configured correctly. This is useful because some secure redis instances (eg Azure) don't allow clients to
* run the CONFIG command.
*/
private final Boolean autoConfigure;
/**
* Validates the configuration
*
* @throws ConfigException If either:
*
* - address is non null and invalid
* - address is null and sentinels is null or empty
* - sentinels is non null and non empty and masterName is null or blank
* - any sentinels are invalid
*
*/
@Override
public void basicValidate(final String section) throws ConfigException {
super.basicValidate(CONFIG_SECTION);
if (address == null && (sentinels == null || sentinels.isEmpty())) {
throw new ConfigException(CONFIG_SECTION, "Redis configuration requires either an address or at least one sentinel to connect to");
}
if (sentinels != null && !sentinels.isEmpty()) {
if (StringUtils.isBlank(masterName)) {
throw new ConfigException(CONFIG_SECTION, "Redis configuration requires a masterName when connecting to sentinel");
}
for (final HostAndPort sentinel : sentinels) {
sentinel.basicValidate(CONFIG_SECTION);
}
}
}
@JsonPOJOBuilder(withPrefix = "")
public static class RedisConfigBuilder {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy