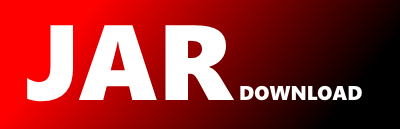
com.hpe.nv.analysis.dtos.reports.metrics.Resources Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hpe-nv-java-api Show documentation
Show all versions of hpe-nv-java-api Show documentation
This Java library lets you call the NV API in your automatic tests (for example, your Selenium tests).
You can use this API instead of using the NV Test Manager user interface.
The newest version!
/*************************************************************************
(c) Copyright [2016] Hewlett Packard Enterprise Development LP
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*************************************************************************/
package com.hpe.nv.analysis.dtos.reports.metrics;
import java.util.HashMap;
public class Resources {
static final public String pluginName = "metrics";
static final public String plguinType = "Metrics report";
static final public String pluginSubtype = "Summary Metrics report";
static final public String pluginVersion = "0.80";
static final public String tcpMetrics = "tcp";
static final public String udpMetrics = "udp";
static final public String httpMetrics = "http";
static final public String httpsMetrics = "https";
static final public String hlsMetrics = "hls";
static final public String errorTimeouts = "Timeouts";
static final public String errorFastRetransmissions = "FastRetransmissions";
static final public String errorRefusedConnections = "RefusedConnections";
static final public String errorBadChecksum = "BadChecksum";
static final public String error400 = "HTTP400";
static final public String error401 = "HTTP401";
static final public String error403 = "HTTP403";
static final public String error404 = "HTTP404";
static final public String error405 = "HTTP405";
static final public String error406 = "HTTP406";
static final public String error407 = "HTTP407";
static final public String error408 = "HTTP408";
static final public String error409 = "HTTP409";
static final public String error410 = "HTTP410";
static final public String error411 = "HTTP411";
static final public String error412 = "HTTP412";
static final public String error413 = "HTTP413";
static final public String error414 = "HTTP414";
static final public String error415 = "HTTP415";
static final public String error416 = "HTTP416";
static final public String error417 = "HTTP417";
static final public String error500 = "HTTP500";
static final public String error501 = "HTTP501";
static final public String error502 = "HTTP502";
static final public String error503 = "HTTP503";
static final public String error504 = "HTTP504";
static final public String error505 = "HTTP505";
static final public String errorHlsBuffering = "Buffering";
static final public String errorHlsSkipped = "Skipped";
static final public String errorHlsPlaylistChange = "PlaylistChange";
// key = type, value = description
static HashMap errorsDescriptions;
static {
errorsDescriptions = new HashMap();
errorsDescriptions.put(errorTimeouts, "Timeout Retransmissions");
errorsDescriptions.put(errorFastRetransmissions, "Fast Retransmissions");
errorsDescriptions.put(errorRefusedConnections, "Refused Connections from server");
errorsDescriptions.put(errorBadChecksum, "Bad checksum");
errorsDescriptions.put(error400, "Error code 400: Bad request.");
errorsDescriptions.put(error401, "Error code 401: Unauthorized.");
errorsDescriptions.put(error403, "Error code 403: Forbidden.");
errorsDescriptions.put(error404, "Error code 404: Not Found.");
errorsDescriptions.put(error405, "Error code 405: Method Not Allowed.");
errorsDescriptions.put(error406, "Error code 406: Not Acceptable.");
errorsDescriptions.put(error407, "Error code 407: Proxy Authentication Required.");
errorsDescriptions.put(error408, "Error code 408: Request Timeout.");
errorsDescriptions.put(error409, "Error code 409: Conflict.");
errorsDescriptions.put(error410, "Error code 410: Gone.");
errorsDescriptions.put(error411, "Error code 411: Length Required.");
errorsDescriptions.put(error412, "Error code 412: Precondition Failed.");
errorsDescriptions.put(error413, "Error code 413: Request Entity Too Large.");
errorsDescriptions.put(error414, "Error code 414: Request-URI Too Long.");
errorsDescriptions.put(error415, "Error code 415: Unsupported Media Type.");
errorsDescriptions.put(error416, "Error code 416: Requested Range Not Satisfiable.");
errorsDescriptions.put(error417, "Error code 417: Expectation Failed.");
errorsDescriptions.put(error500, "Error code 500: Internal Server Error.");
errorsDescriptions.put(error501, "Error code 501: Not Implemented.");
errorsDescriptions.put(error502, "Error code 502: Bad Gateway.");
errorsDescriptions.put(error503, "Error code 503: Service Unavailable.");
errorsDescriptions.put(error504, "Error code 504: Gateway Timeout.");
errorsDescriptions.put(error505, "Error code 505: HTTP Version Not Supported.");
errorsDescriptions.put(errorHlsBuffering, "Video Buffering");
errorsDescriptions.put(errorHlsSkipped, "Skipped Files");
errorsDescriptions.put(errorHlsPlaylistChange, "Playlist Changed");
}
// key = type, value = description
static HashMap errorsNames;
static {
errorsNames = new HashMap();
errorsNames.put(errorTimeouts, "Timeouts");
errorsNames.put(errorFastRetransmissions, "Fast Retransmissions");
errorsNames.put(errorRefusedConnections, "Refused Connections");
errorsNames.put(errorBadChecksum, "Bad checksum");
errorsNames.put(error400, "HTTP 400");
errorsNames.put(error401, "HTTP 401");
errorsNames.put(error403, "HTTP 403");
errorsNames.put(error404, "HTTP 404");
errorsNames.put(error405, "HTTP 405");
errorsNames.put(error406, "HTTP 406");
errorsNames.put(error407, "HTTP 407");
errorsNames.put(error408, "HTTP 408");
errorsNames.put(error409, "HTTP 409");
errorsNames.put(error410, "HTTP 410");
errorsNames.put(error411, "HTTP 411");
errorsNames.put(error412, "HTTP 412");
errorsNames.put(error413, "HTTP 413");
errorsNames.put(error414, "HTTP 414");
errorsNames.put(error415, "HTTP 415");
errorsNames.put(error416, "HTTP 416");
errorsNames.put(error417, "HTTP 417");
errorsNames.put(error500, "HTTP 500");
errorsNames.put(error501, "HTTP 501");
errorsNames.put(error502, "HTTP 502");
errorsNames.put(error503, "HTTP 503");
errorsNames.put(error504, "HTTP 504");
errorsNames.put(error505, "HTTP 505");
errorsNames.put(errorHlsBuffering, "Video Buffering");
errorsNames.put(errorHlsSkipped, "Skipped Files");
errorsNames.put(errorHlsPlaylistChange, "Playlist Changes");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy