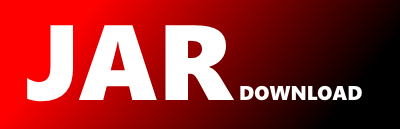
com.hpe.nv.api.Flow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hpe-nv-java-api Show documentation
Show all versions of hpe-nv-java-api Show documentation
This Java library lets you call the NV API in your automatic tests (for example, your Selenium tests).
You can use this API instead of using the NV Test Manager user interface.
The newest version!
/*************************************************************************
(c) Copyright [2016] Hewlett Packard Enterprise Development LP
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*************************************************************************/
package com.hpe.nv.api;
import org.apache.log4j.Logger;
/**
* This class represents an Flow instance.
*
* @author Eleanor Ainy
* @version 1.0.0
*/
public class Flow extends IPConfiguration {
final static Logger logger = Logger.getLogger(Flow.class);
private String flowId;//flow id
private double latency = 100;
private double packetloss = 0.1;
private double bandwidthIn = 0;
private double bandwidthOut = 0;
private boolean isCaptureClientPL = true;
private boolean isDefaultFlow;//can specify isDefaultFlow=true to set the flow to default flow
private boolean shareBandwidth = true;
/**
* Class constructor
*
* @param flowId flow ID
* @param latency Default: 100
* @param packetloss Default: 0.1
* @param bandwidthIn Default: 0
* @param bandwidthOut Default: 0
* @exception NVExceptions.MissingPropertyException if the flowId parameter is null.
*/
public Flow(String flowId, double latency, double packetloss, double bandwidthIn, double bandwidthOut) throws NVExceptions.MissingPropertyException {
super();
String errorMessage;
if (flowId == null) {
errorMessage = "Mandatory property flowId is missing. Flow must have a unique flowId";
logger.error(errorMessage);
throw new NVExceptions.MissingPropertyException(errorMessage);
}
this.flowId = flowId;
this.latency = latency;
this.packetloss = packetloss;
this.bandwidthIn = bandwidthIn;
this.bandwidthOut = bandwidthOut;
}
/**
* Call this constructor method to use the default values of the other parameters
*
* @param flowId flow ID
*/
public Flow(String flowId) {
this.flowId = flowId;
this.latency = 100;
this.packetloss = 0.1;
this.bandwidthIn = 0;
this.bandwidthOut = 0;
this.isCaptureClientPL = true;
this.isDefaultFlow = false;
}
/**
* Returns true if the specified flow is equal to this flow
*
* @param otherFlow a {@link Flow} object
* @return true if the specified flow is equal to this flow
*/
public boolean equals(Flow otherFlow) {
boolean isIPConfigEqual = super.equals(otherFlow);
if (!(otherFlow instanceof Flow)) {
return false;
}
if (otherFlow == null) {
return false;
}
return (this.flowId.equals(otherFlow.flowId) &&
this.latency == otherFlow.latency &&
this.packetloss == otherFlow.packetloss &&
this.bandwidthIn == otherFlow.bandwidthIn &&
this.bandwidthOut == otherFlow.bandwidthOut &&
this.isCaptureClientPL == otherFlow.isCaptureClientPL &&
(this.isDefaultFlow == otherFlow.isDefaultFlow) &&
isIPConfigEqual);
}
/**
* Removes the specified source IP range from the srcIpRange Range object's "exclude" array
*
* @param ipRange an {@link IPRange} object
* @exception NVExceptions.IllegalArgumentException if the specified argument is not an instance of {@link IPRange} class.
* @exception NVExceptions.NotSupportedException if the flow is defined as "Default Flow" and the exclude range protocol and port settings are set.
*/
@Override
public void excludeSourceIPRange(IPRange ipRange) throws NVExceptions.IllegalArgumentException, NVExceptions.NotSupportedException {
String errorMessage;
if (this.isDefaultFlow) {
errorMessage = "The exclude range protocol and port settings cannot be set for a default flow.";
logger.error(errorMessage);
throw new NVExceptions.NotSupportedException(errorMessage);
}
super.excludeSourceIPRange(ipRange);
};
/**
* Removes the specified destination IP range from the destIpRange {@link Range} object's "exclude" array
*
* @param ipRange an {@link IPRange} object
* @exception NVExceptions.IllegalArgumentException if the specified argument is not an instance of {@link IPRange} class.
* @exception NVExceptions.NotSupportedException if the flow is defined as "Default Flow" and the exclude range protocol and port settings are set.
*/
@Override
public void excludeDestIPRange(IPRange ipRange) throws NVExceptions.IllegalArgumentException, NVExceptions.NotSupportedException {
String errorMessage;
if (this.isDefaultFlow) {
errorMessage = "The exclude range protocol and port settings cannot be set for a default flow.";
logger.error(errorMessage);
throw new NVExceptions.NotSupportedException(errorMessage);
}
super.excludeDestIPRange(ipRange);
};
/**
* Returns the flow ID
*
* @return the flow ID
*/
public String getFlowId() {
return flowId;
}
/**
* Sets the flow ID
*
* @param flowId an ID for the flow
*/
public void setFlowId(String flowId) {
this.flowId = flowId;
}
/**
* Returns the flow's latency
*
* @return the flow's latency
*/
public double getLatency() {
return latency;
}
/**
* Sets the flow's latency
*
* @param latency a latency for the flow
*/
public void setLatency(double latency) {
this.latency = latency;
}
/**
* Returns the flow's packet loss value
*
* @return the flow's packet loss value
*/
public double getPacketloss() {
return packetloss;
}
/**
* Sets the flow's packet loss value
*
* @param packetloss a packet loss value for the flow
*/
public void setPacketloss(double packetloss) {
this.packetloss = packetloss;
}
/**
* Returns the flow's bandwidthIn value
*
* @return the flow's bandwidthIn value
*/
public double getBandwidthIn() {
return bandwidthIn;
}
/**
* Sets the flow's bandwidthIn value
*
* @param bandwidthIn a bandwidthIn value for the flow
*/
public void setBandwidthIn(double bandwidthIn) {
this.bandwidthIn = bandwidthIn;
}
/**
* Returns the flow's bandwidthOut value
*
* @return the flow's bandwidthOut value
*/
public double getBandwidthOut() {
return bandwidthOut;
}
/**
* Sets the flow's bandwidthOut value
*
* @param bandwidthOut a bandwidthOut value for the flow
*/
public void setBandwidthOut(double bandwidthOut) {
this.bandwidthOut = bandwidthOut;
}
/**
* Returns true if packets lists are captured for the flow
*
* @return true if packets lists are captured for the flow
*/
public boolean isCaptureClientPL() {
return isCaptureClientPL;
}
/**
* Sets the isCaptureClientPL Boolean value
*
* @param captureClientPL if set to false no packets lists are captured for the flow
*/
public void setCaptureClientPL(boolean captureClientPL) {
isCaptureClientPL = captureClientPL;
}
/**
* Returns true if the flow is defined as "Default Flow"
*
* @return true if the flow is defined as "Default Flow"
*/
public boolean isDefaultFlow() {
return isDefaultFlow;
}
/**
* Sets the defaultFlow Boolean value
*
* @param defaultFlow set to true to use "Default Flow"
*/
public void setDefaultFlow(boolean defaultFlow) {
isDefaultFlow = defaultFlow;
}
/**
* Returns true if bandwidth is shared
*
* @return true if bandwidth is shared
*/
public boolean shareBandwidth() {
return shareBandwidth;
}
/**
* Sets the shareBandwidth Boolean value.
* Set to false to let every Source-Destination IP pair that fits this flow definition use the defined bandwidth.
* This enables packets to be handled without delay. (When set to true, a queue manages the packets, which may result in delayed packet handling.)
* Default: true
*
* @param shareBandwidth a Boolean value
*/
public void setShareBandwidth(boolean shareBandwidth) {
this.shareBandwidth = shareBandwidth;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy