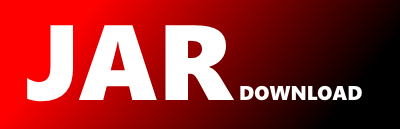
commons.box.util.Beans Maven / Gradle / Ivy
Show all versions of commons-box-bean Show documentation
package commons.box.util;
import com.google.common.collect.Ordering;
import commons.box.app.AppError;
import commons.box.bean.AppBean;
import commons.box.bean.AppClass;
import commons.box.bean.BeanOrder;
import commons.box.bean.ClassAccess;
import java.util.Map;
/**
* 创建作者:xingxiuyi
* 版权所属:xingxiuyi
*/
public final class Beans {
private final static AppBean BEAN_OP = new AppBean(true);
private final static AppBean BEAN_SAFE_OP = new AppBean(false);
private Beans() {
}
/**
* 返回Bean操作器
*
* @return
*/
public static AppBean bean() {
return AppBean.inst();
}
@SuppressWarnings("unchecked")
public static ClassAccess access(Class type) {
return AppClass.from(type).access();
}
@SuppressWarnings("unchecked")
public static ClassAccess access(T object) {
Class cls = (object != null) ? (Class) object.getClass() : null;
return AppClass.from(cls).access();
}
/**
* 返回Bean操作器
*
* throwError表示在操作时发生异常是否抛出 如果不抛出则仅记录error日志
*
* @param throwError
* @return
*/
public static AppBean bean(boolean throwError) {
return AppBean.inst(throwError);
}
/**
* Bean排序 本排序器空值认为是最小值 支持内联属性
*
* @param props
* @param
* @return
*/
public static Ordering orderByProps(String... props) {
return new BeanOrder(props);
}
/**
* Bean排序 本排序器空值认为是最小值 支持内联属性
*
* 注意:本方法在不包含内联属性时,与byProps(String... props)相比速度会略快
*
* @param type
* @param props
* @param
* @return
*/
public static Ordering orderByProps(Class type, String... props) {
return new BeanOrder(type, props);
}
/**
* 按map排序的Builder
*
* @return
*/
public static BeanOrder.Builder