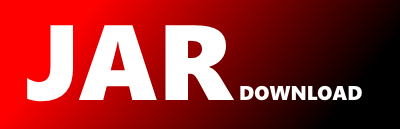
com.htyleo.extsort.ExternalSortConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of external-sort Show documentation
Show all versions of external-sort Show documentation
An implementation of external sorting using parallelism
The newest version!
package com.htyleo.extsort;
import java.util.Comparator;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import com.htyleo.extsort.common.LineFilter;
/**
* External sorting config
*
* @author htyleo
*/
public class ExternalSortConfig {
/** File encoding, used for reading and writing files */
private String encoding = "UTF-8";
/** Buffer size, default is 8 KB */
private int bufferSize = 8 * 1024;
/** Slice size, default is 2 MB */
private int sliceSize = 2 * 1024 * 1024;
/** The first headerLines lines are regarded as the header, which will be extracted and written to a separate file */
private int headerLines = 0;
/** Whether we ignore (i.e. do not count them in headerLines) the leading blank lines in the file */
private boolean ignoreHeaderBlankLines = false;
/** The last tailLines lines are regarded as the tail, which will be extracted and written to a separate file */
private int tailLines = 0;
/** Whether we ignore (i.e. do not count them in tailLines) the trailing blank lines in the file */
private boolean ignoreTailBlankLines = false;
/** Line filter. By default we do not filter out any line */
private LineFilter lineFilter = new LineFilter() {
@Override
public boolean isConcerned(String line) {
return true;
}
};
/** Che comparator used for sorting. By default, lines are sorted in alphabetical order. */
private Comparator lineComparator = new Comparator() {
@Override
public int compare(String s1, String s2) {
return s1.compareTo(s2);
}
};
/**
* ThreadPoolExecutor used to run external sorting in parallel
* Default parameters:
*
* - coreSize = 5
* - maxSize = 8
* - keepAliveTime = 300s
*
*/
private ThreadPoolExecutor executor = new ThreadPoolExecutor(5, 8, 300,
TimeUnit.SECONDS,
new LinkedBlockingQueue());
/**
* Getter method for property encoding.
*
* @return property value of encoding
*/
public String getEncoding() {
return encoding;
}
/**
* Setter method for property encoding.
*
* @param encoding value to be assigned to property encoding
*/
public void setEncoding(String encoding) {
this.encoding = encoding;
}
/**
* Getter method for property bufferSize.
*
* @return property value of bufferSize
*/
public int getBufferSize() {
return bufferSize;
}
/**
* Setter method for property bufferSize.
*
* @param bufferSize value to be assigned to property bufferSize
*/
public void setBufferSize(int bufferSize) {
this.bufferSize = bufferSize;
}
/**
* Getter method for property sliceSize.
*
* @return property value of sliceSize
*/
public int getSliceSize() {
return sliceSize;
}
/**
* Setter method for property sliceSize.
*
* @param sliceSize value to be assigned to property sliceSize
*/
public void setSliceSize(int sliceSize) {
this.sliceSize = sliceSize;
}
/**
* Getter method for property headerLines.
*
* @return property value of headerLines
*/
public int getHeaderLines() {
return headerLines;
}
/**
* Setter method for property headerLines.
*
* @param headerLines value to be assigned to property headerLines
*/
public void setHeaderLines(int headerLines) {
this.headerLines = headerLines;
}
/**
* Getter method for property ignoreHeaderBlankLines.
*
* @return property value of ignoreHeaderBlankLines
*/
public boolean getIgnoreHeaderBlankLines() {
return ignoreHeaderBlankLines;
}
/**
* Setter method for property ignoreHeaderBlankLines.
*
* @param ignoreHeaderBlankLines value to be assigned to property ignoreHeaderBlankLines
*/
public void setIgnoreHeaderBlankLines(boolean ignoreHeaderBlankLines) {
this.ignoreHeaderBlankLines = ignoreHeaderBlankLines;
}
/**
* Getter method for property tailLines.
*
* @return property value of tailLines
*/
public int getTailLines() {
return tailLines;
}
/**
* Setter method for property tailLines.
*
* @param tailLines value to be assigned to property tailLines
*/
public void setTailLines(int tailLines) {
this.tailLines = tailLines;
}
/**
* Getter method for property ignoreTailBlankLines.
*
* @return property value of ignoreTailBlankLines
*/
public boolean getIgnoreTailBlankLines() {
return ignoreTailBlankLines;
}
/**
* Setter method for property ignoreTailBlankLines.
*
* @param ignoreTailBlankLines value to be assigned to property ignoreTailBlankLines
*/
public void setIgnoreTailBlankLines(boolean ignoreTailBlankLines) {
this.ignoreTailBlankLines = ignoreTailBlankLines;
}
/**
* Getter method for property lineFilter.
*
* @return property value of lineFilter
*/
public LineFilter getLineFilter() {
return lineFilter;
}
/**
* Setter method for property lineFilter.
*
* @param lineFilter value to be assigned to property lineFilter
*/
public void setLineFilter(LineFilter lineFilter) {
this.lineFilter = lineFilter;
}
/**
* Getter method for property lineComparator.
*
* @return property value of lineComparator
*/
public Comparator getLineComparator() {
return lineComparator;
}
/**
* Setter method for property lineComparator.
*
* @param lineComparator value to be assigned to property lineComparator
*/
public void setLineComparator(Comparator lineComparator) {
this.lineComparator = lineComparator;
}
/**
* Getter method for property executor.
*
* @return property value of executor
*/
public ThreadPoolExecutor getExecutor() {
return executor;
}
/**
* Setter method for property executor.
*
* @param executor value to be assigned to property executor
*/
public void setExecutor(ThreadPoolExecutor executor) {
this.executor = executor;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy