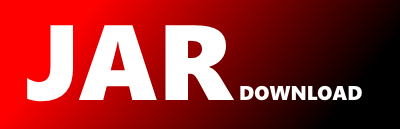
commonMain.com.huawei.hilink.c2c.integration.helper.callbackAdapters.DeviceConversionAdapters.kt Maven / Gradle / Ivy
package com.huawei.hilink.c2c.integration.helper.callbackAdapters
import com.huawei.hilink.c2c.integration.helper.IllegalAccessException
import com.huawei.hilink.c2c.integration.helper.PartnerImplCrashException
import com.huawei.hilink.c2c.integration.helper.PartnerImplErrorException
import com.huawei.hilink.c2c.integration.helper.UnauthorizedException
import com.huawei.hilink.c2c.integration.helper.api.*
import com.huawei.hilink.c2c.integration.helper.model.firstParamViolationOrNull
import kotlin.coroutines.resume
import kotlin.coroutines.resumeWithException
import kotlin.coroutines.suspendCoroutine
/**
* Called when HiLink cloud wants to modify device state for a given device.
* This happens when end-user uses AI Life to control the device.
*
* @param userInfo object containing an access token and a HuaweiId of the user.
* If you're implementing [CentralAccessTokenCheck], it will also contain the id of the authorized user.
* @param deviceId the id, in the partner's system, of the device to execute the command on
* @param serviceId the id of a deviceService, which is to be altered.
* For example it could be "brightness" for a lamp device
* @param dataJson raw json with a "type" property which holds deviceService ID, for polymorphic deserialization.
*/
internal suspend fun DeviceConversion.executeCommandSuspending(
userInfo: UserInfo,
deviceId: String,
serviceId: String,
dataJson: String
): CommandResult =
suspendCoroutine {
try {
executeCommand(userInfo, deviceId, serviceId, dataJson, object : CommandResultConsumer {
override fun onResult(result: CommandResult) {
it.resume(result)
}
override fun onUnauthorized() {
it.resumeWithException(UnauthorizedException())
}
override fun onIllegalAccess() {
it.resumeWithException(IllegalAccessException())
}
})
} catch (t: Throwable) {
it.resumeWithException(PartnerImplCrashException(SITE_EXECUTE_COMMAND))
}
}
/**
* Called when HiLink cloud needs to learn about the device, what it's called,
* what deviceServices it provides (what features it has, that can be controlled by user)
*
* @param userInfo object containing an access token and a HuaweiId of the user.
* If you're implementing [CentralAccessTokenCheck], it will also contain the id of the authorized user.
* @param deviceId id of the device to gather information about.
*/
internal suspend fun DeviceConversion.discoverSuspending(userInfo: UserInfo, deviceId: String): DeviceInformation =
suspendCoroutine {
try {
discover(userInfo, deviceId, object : ResultConsumer {
override fun onSuccess(result: DeviceInformation) {
result.firstParamViolationOrNull(SITE_DISCOVER)
?.let { exception -> return it.resumeWithException(exception) }
it.resume(result)
}
override fun onError(message: String) {
it.resumeWithException(
PartnerImplErrorException(SITE_DISCOVER, message)
)
}
override fun onUnauthorized() {
it.resumeWithException(UnauthorizedException())
}
override fun onIllegalAccess() {
it.resumeWithException(IllegalAccessException())
}
})
} catch (t: Throwable) {
it.resumeWithException(PartnerImplCrashException(SITE_DISCOVER))
}
}
/**
* Called when HiLink cloud needs to obtain the current state of the device.
* It includes the data of the deviceServices (controllable features of the device)
* For example a lamp device might have a "brightness" service, it such a case, the
* level of brightness set at the moment should be returned.
*
* @param userInfo object containing an access token and a HuaweiId of the user.
* If you're implementing [CentralAccessTokenCheck], it will also contain the id of the authorized user.
* @param deviceId id of the device to gather information about.
*/
internal suspend fun DeviceConversion.snapshotSuspending(userInfo: UserInfo, deviceId: String): DeviceSnapshot =
suspendCoroutine {
try {
snapshot(userInfo, deviceId, object : ResultConsumer {
override fun onSuccess(result: DeviceSnapshot) {
result.firstParamViolationOrNull(SITE_SNAPSHOT)
?.let { exception -> return it.resumeWithException(exception) }
it.resume(result)
}
override fun onError(message: String) {
it.resumeWithException(
PartnerImplErrorException(SITE_SNAPSHOT, message)
)
}
override fun onUnauthorized() {
it.resumeWithException(UnauthorizedException())
}
override fun onIllegalAccess() {
it.resumeWithException(IllegalAccessException())
}
})
} catch (t: Throwable) {
it.resumeWithException(PartnerImplCrashException(SITE_SNAPSHOT))
}
}
private const val SITE_EXECUTE_COMMAND = "DeviceConversion.executeCommand()"
private const val SITE_DISCOVER = "DeviceConversion.discover()"
private const val SITE_SNAPSHOT = "DeviceConversion.snapshot()"
© 2015 - 2025 Weber Informatics LLC | Privacy Policy