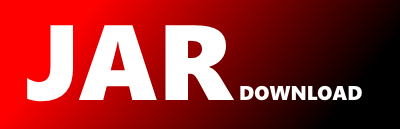
jvmMain.com.huawei.hilink.c2c.integration.helper.api.HiLinkHelperExtensions.kt Maven / Gradle / Ivy
package com.huawei.hilink.c2c.integration.helper.api
import kotlinx.coroutines.runBlocking
/**
* Allows for synchronous use of the helper. Similar to [HiLinkHelper.processRequest] but blocks thread until
* the response is ready.
*/
public fun HiLinkHelper.processRequestSynchronously(authorizationHeader: String, requestBody: String): HttpResponse =
runBlocking {
return@runBlocking processRequest(authorizationHeader, requestBody)
}
/**
* Allows for synchronous use of the helper. Similar to [HiLinkHelper.notifyDeviceStatusChange] but blocks thread until
* the response is ready.
*/
public fun HiLinkHelper.notifyDeviceStatusChangeSynchronously(
userId: String,
huaweiId: String,
deviceSnapshot: DeviceSnapshot
): EventResponse = runBlocking {
return@runBlocking notifyDeviceStatusChange(userId, huaweiId, deviceSnapshot)
}
/**
* Allows for synchronous use of the helper. Similar to [HiLinkHelper.notifyDeviceStatusChangeAuto] but blocks thread until
* the response is ready.
*/
public fun HiLinkHelper.notifyDeviceStatusChangeAutoSynchronously(
accessToken: String?,
userId: String,
huaweiId: String,
deviceId: String
): EventResponse = runBlocking {
return@runBlocking notifyDeviceStatusChangeAuto(accessToken, userId, huaweiId, deviceId)
}
/**
* Allows for synchronous use of the helper. Similar to [HiLinkHelper.notifyDeviceAddition] but blocks thread until
* the response is ready.
*/
public fun HiLinkHelper.notifyDeviceAdditionSynchronously(
userId: String,
huaweiId: String,
deviceInfo: DeviceInformation
): EventResponse = runBlocking {
return@runBlocking notifyDeviceAddition(userId, huaweiId, deviceInfo)
}
/**
* Allows for synchronous use of the helper. Similar to [HiLinkHelper.notifyDeviceAdditionAuto] but blocks thread until
* the response is ready.
*/
public fun HiLinkHelper.notifyDeviceAdditionAutoSynchronously(
accessToken: String?,
userId: String,
huaweiId: String,
deviceId: String
): EventResponse = runBlocking {
return@runBlocking notifyDeviceAdditionAuto(accessToken, userId, huaweiId, deviceId)
}
/**
* Allows for synchronous use of the helper. Similar to [HiLinkHelper.notifyDeviceRemoval] but blocks thread until
* the response is ready.
*/
public fun HiLinkHelper.notifyDeviceRemovalSynchronously(
userId: String,
huaweiId: String,
deviceId: String
): EventResponse = runBlocking {
return@runBlocking notifyDeviceRemoval(userId, huaweiId, deviceId)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy