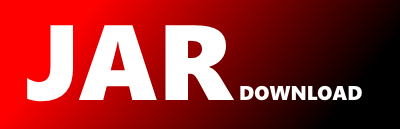
com.huawei.openstack4j.api.gbp.PolicyTargetServiceTest Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright 2016 ContainX and OpenStack4j
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*******************************************************************************/
package com.huawei.openstack4j.api.gbp;
import static org.testng.Assert.assertEquals;
import static org.testng.Assert.assertNotNull;
import static org.testng.Assert.assertTrue;
import java.util.List;
import java.util.logging.Logger;
import org.testng.annotations.Test;
import com.google.common.base.Preconditions;
import com.huawei.openstack4j.api.AbstractTest;
import com.huawei.openstack4j.api.Builders;
import com.huawei.openstack4j.model.common.ActionResponse;
import com.huawei.openstack4j.model.gbp.PolicyTarget;
/**
* Test cases for policy target on GBP
*
* @author vinod borole
*/
@Test(suiteName="grouppolicy/policy_targets")
public class PolicyTargetServiceTest extends AbstractTest {
private static final String POLICY_TARGETS="/network/gbp/policy_targets.json";
private static final String POLICY_TARGET="/network/gbp/policy_target.json";
private static final String POLICY_TARGET_UPDATE="/network/gbp/policy_target_update.json";
@Override
protected Service service() {
return Service.NETWORK;
}
@Test
public void testListPolicyTarget() throws Exception{
respondWith(POLICY_TARGETS);
List extends PolicyTarget> policytargetList = osv2().gbp().policyTarget().list();
assertEquals(10, policytargetList.size());
Preconditions.checkNotNull(policytargetList.get(0));
Logger.getLogger(getClass().getName()).info(getClass().getName() + " : Policy target from List : "+policytargetList.get(0));
assertEquals(policytargetList.get(0).getId(), "0d65eebe-4efe-456e-aec3-7856e4e839b4");
}
@Test
public void testGetPolicyTarget() throws Exception{
respondWith(POLICY_TARGET);
String id = "0d65eebe-4efe-456e-aec3-7856e4e839b4";
PolicyTarget policyTarget = osv2().gbp().policyTarget().get(id);
Logger.getLogger(getClass().getName()).info(getClass().getName() + " : Policy target by ID : "+policyTarget);
assertNotNull(policyTarget);
assertEquals(id, policyTarget.getId());
}
@Test
public void testCreatePolicyTarget() throws Exception{
respondWith(POLICY_TARGET);
PolicyTarget policyTargetCreate= Builders.policyTarget().name("test-policytarget").description("test-policytarget-desc").build();
PolicyTarget policyTarget = osv2().gbp().policyTarget().create(policyTargetCreate);
Logger.getLogger(getClass().getName()).info(getClass().getName() + " : Created Policy Target : "+policyTarget);
assertEquals("test-policytarget", policyTarget.getName());
assertEquals("36af8850-3514-4343-8293-9f9faae980d6", policyTarget.getPortId());
assertEquals("1fb00129-06cf-48e5-8282-d15dbf4be60b", policyTarget.getPolicyTargetGroupId());
}
@Test
public void testUpdatePolicyTarget() throws Exception{
respondWith(POLICY_TARGET_UPDATE);
String id = "0d65eebe-4efe-456e-aec3-7856e4e839b4";
PolicyTarget policyTargetCreate= Builders.policyTarget().name("test-policytarget-update").description("test-policytarget-desc-update").build();
PolicyTarget policyTarget =osv2().gbp().policyTarget().update(id, policyTargetCreate);
Logger.getLogger(getClass().getName()).info(getClass().getName() + " : Updated Policy Target : "+policyTarget);
assertEquals("test-policytarget-desc-update", policyTarget.getDescription());
}
@Test
public void testDeletePolicyTarget() {
respondWith(200);
String id = "0d65eebe-4efe-456e-aec3-7856e4e839b4";
ActionResponse result = osv2().gbp().policyTarget().delete(id);
assertTrue(result.isSuccess());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy