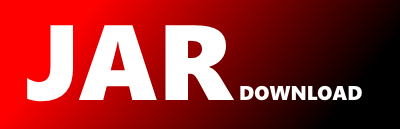
com.huaweicloud.dws.client.exception.DwsClientException Maven / Gradle / Ivy
package com.huaweicloud.dws.client.exception;
import com.huawei.gauss200.jdbc.util.PSQLState;
import lombok.Getter;
import lombok.ToString;
import java.sql.SQLException;
import java.util.Arrays;
import java.util.List;
import java.util.Locale;
import java.util.concurrent.TimeoutException;
/**
* @ProjectName: dws-connector
* @ClassName: DwsClientException
* @Description: dwsClient异常封装
* @Date: 2023/1/9 16:57
* @Version: 1.0
*/
@Getter
@ToString
public class DwsClientException extends Exception {
public static final List CONNECTION_ERROR_KEY = Arrays.asList(
"this connection has been closed",
"connection refused",
"the connection attempt failed",
"can not accept connection in pending mode");
public static final List TOO_MANY_CONNECTIONS_KEY = Arrays.asList(
"already too many clients",
"remaining connection slots are reserved");
public static final List READ_ONLY_KEY = Arrays.asList(
"not allowed in readonly mode",
"read-only transaction",
"read-only",
"readonly");
public static final List LOCK_ERROR_KEY = Arrays.asList(
"lockmode",
"obtain lock",
"deadlock detected",
"lock");
public static final List TIME_OUT_KEY = Arrays.asList(
"canceling statement due to statement timeout",
"timeout");
public static final List ALREADY_EXISTS_KEY = Arrays.asList(
"already exists");
public static final List STARTING_UP_KEY = Arrays.asList("starting up");
/**
* dws解析的异常code
*/
private final ExceptionCode code;
/**
* 原始异常
*/
private Throwable original;
public DwsClientException(ExceptionCode code) {
this.code = code;
}
public DwsClientException() {
this.code = null;
}
public DwsClientException(ExceptionCode code, String message) {
super(message);
this.code = code;
}
public DwsClientException(ExceptionCode code, String message, Throwable cause) {
super(message, cause);
this.code = code;
this.original = cause;
}
public static DwsClientException fromException(SQLException e) {
ExceptionCode exceptionCode = getSqlExceptionCode(e);
return new DwsClientException(exceptionCode, getMsg(e, exceptionCode), e);
}
private static final List DATA_VALUE_ERROR_STATE = Arrays.asList(PSQLState.DATA_ERROR.getState()
, PSQLState.STRING_DATA_RIGHT_TRUNCATION.getState()
, PSQLState.NUMERIC_VALUE_OUT_OF_RANGE.getState(),
PSQLState.BAD_DATETIME_FORMAT.getState(),
PSQLState.DATETIME_OVERFLOW.getState(),
PSQLState.INVALID_PARAMETER_VALUE.getState(),
PSQLState.NUMERIC_CONSTANT_OUT_OF_RANGE.getState()
);
private static ExceptionCode getSqlExceptionCode(SQLException e) {
if (PSQLState.isConnectionError(e.getSQLState())) {
return ExceptionCode.CONNECTION_ERROR;
}
if (e.getCause() instanceof DwsClientException) {
return ((DwsClientException) e.getCause()).getCode();
}
ExceptionCode exceptionCode = getExceptionCodeByState(e);
if (exceptionCode != null) {
return exceptionCode;
}
ExceptionCode connectionError = getExceptionCodeByMsg(e);
if (connectionError != null) {
return connectionError;
}
return ExceptionCode.UNKNOWN_ERROR;
}
public static ExceptionCode getExceptionCodeByMsg(SQLException e) {
if (e.getMessage() != null) {
for (String key : CONNECTION_ERROR_KEY) {
if (e.getMessage().contains(key)) {
return ExceptionCode.CONNECTION_ERROR;
}
}
for (String key : READ_ONLY_KEY) {
if (e.getMessage().toLowerCase(Locale.ROOT).contains(key)) {
return ExceptionCode.READ_ONLY;
}
}
for (String key : TOO_MANY_CONNECTIONS_KEY) {
if (e.getMessage().contains(key)) {
return ExceptionCode.TOO_MANY_CONNECTIONS;
}
}
for (String key : LOCK_ERROR_KEY) {
if (e.getMessage().toLowerCase(Locale.ROOT).contains(key)) {
return ExceptionCode.LOCK_ERROR;
}
}
for (String key : TIME_OUT_KEY) {
if (e.getMessage().toLowerCase(Locale.ROOT).contains(key)) {
return ExceptionCode.TIMEOUT;
}
}
for (String key : STARTING_UP_KEY) {
if (e.getMessage().toLowerCase(Locale.ROOT).contains(key)) {
return ExceptionCode.STARTING_UP;
}
}
for (String key : ALREADY_EXISTS_KEY) {
if (e.getMessage().toLowerCase(Locale.ROOT).contains(key)) {
return ExceptionCode.ALREADY_EXISTS;
}
}
}
return null;
}
public static ExceptionCode getExceptionCodeByState(SQLException e) {
String state = e.getSQLState();
if (PSQLState.SYNTAX_ERROR.getState().equals(state)) {
return ExceptionCode.SYNTAX_ERROR;
} else if (PSQLState.UNDEFINED_TABLE.getState().equals(state)) {
return ExceptionCode.TABLE_NOT_FOUND;
} else if (PSQLState.INVALID_AUTHORIZATION_SPECIFICATION.getState().equals(state) || PSQLState.INVALID_PASSWORD.getState().equals(state)) {
return ExceptionCode.AUTH_FAIL;
} else if (PSQLState.NOT_NULL_VIOLATION.getState().equals(state) || PSQLState.UNIQUE_VIOLATION.getState().equals(state) || PSQLState.CHECK_VIOLATION.getState().equals(state)) {
return ExceptionCode.CONSTRAINT_VIOLATION;
} else if (DATA_VALUE_ERROR_STATE.contains(state)) {
return ExceptionCode.DATA_VALUE_ERROR;
} else if (PSQLState.DATA_TYPE_MISMATCH.getState().equals(state) || PSQLState.INVALID_NAME.getState().equals(state) || PSQLState.DATATYPE_MISMATCH.getState().equals(state) || PSQLState.CANNOT_COERCE.getState().equals(state)) {
return ExceptionCode.DATA_TYPE_ERROR;
}
return null;
}
public static DwsClientException fromException(Throwable exception) {
if (exception == null) {
return new DwsClientException(ExceptionCode.INTERNAL_ERROR);
}
if (exception instanceof DwsClientException) {
return (DwsClientException) exception;
}
if (exception instanceof SQLException) {
return fromException((SQLException) exception);
}
if (exception.getCause() != null && exception.getCause() instanceof DwsClientException) {
return (DwsClientException) exception.getCause();
}
ExceptionCode exceptionCode = ExceptionCode.UNKNOWN_ERROR;
if (exception instanceof InterruptedException) {
exceptionCode = ExceptionCode.INTERRUPTED;
} else if (exception instanceof TimeoutException) {
exceptionCode = ExceptionCode.TIMEOUT;
}
String msg = getMsg(exception, exceptionCode);
return new DwsClientException(exceptionCode, msg, exception);
}
private static String getMsg(Throwable exception, ExceptionCode code) {
String msg;
if (code == ExceptionCode.UNKNOWN_ERROR) {
msg = "[UNKNOWN:]" + exception.getMessage();
} else {
msg = "[" + code.getCode() + "]" + exception.getMessage();
}
return msg;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy